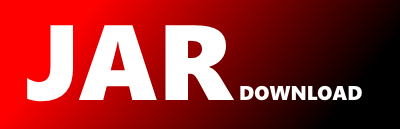
rapture.kernel.script.ScriptDecision Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.kernel.script;
import java.util.List;
import java.util.Map;
import rapture.common.api.DecisionApi;
import rapture.common.api.ScriptDecisionApi;
import org.apache.log4j.Logger;
import rapture.common.exception.RaptureException;
import rapture.common.dp.Step;
import rapture.common.ErrorWrapper;
import rapture.common.dp.Workflow;
import rapture.common.dp.WorkOrderDebug;
import rapture.common.RaptureFolderInfo;
import rapture.common.dp.WorkflowHistoricalMetrics;
import rapture.common.dp.WorkOrder;
import rapture.common.dp.Worker;
import rapture.common.dp.AppStatusDetails;
import rapture.common.dp.WorkOrderStatus;
import rapture.common.LogQueryResponse;
import rapture.common.dp.Transition;
import rapture.common.CreateResponse;
import rapture.common.dp.WorkOrderCancellation;
import rapture.common.AppStatus;
@SuppressWarnings("all")
public class ScriptDecision extends KernelScriptImplBase implements ScriptDecisionApi {
private DecisionApi api;
private static final Logger log = Logger.getLogger(ScriptDecision.class);
public ScriptDecision(DecisionApi api) {
this.api = api;
}
@Override
public List getAllWorkflows() {
List ret = api.getAllWorkflows(callingCtx);
return ret; }
@Override
public List getWorkflowChildren(String workflowURI) {
List ret = api.getWorkflowChildren(callingCtx, workflowURI);
return ret; }
@Override
public List getWorkOrderChildren(String parentPath) {
List ret = api.getWorkOrderChildren(callingCtx, parentPath);
return ret; }
@Override
public void putWorkflow(Workflow workflow) {
api.putWorkflow(callingCtx, workflow); }
@Override
public Workflow getWorkflow(String workflowURI) {
Workflow ret = api.getWorkflow(callingCtx, workflowURI);
return ret; }
@Override
public Step getWorkflowStep(String stepURI) {
Step ret = api.getWorkflowStep(callingCtx, stepURI);
return ret; }
@Override
public String getStepCategory(String stepURI) {
String ret = api.getStepCategory(callingCtx, stepURI);
return ret; }
@Override
public void addStep(String workflowURI,Step step) {
api.addStep(callingCtx, workflowURI, step); }
@Override
public void removeStep(String workflowURI,String stepName) {
api.removeStep(callingCtx, workflowURI, stepName); }
@Override
public void addTransition(String workflowURI,String stepName,Transition transition) {
api.addTransition(callingCtx, workflowURI, stepName, transition); }
@Override
public void removeTransition(String workflowURI,String stepName,String transitionName) {
api.removeTransition(callingCtx, workflowURI, stepName, transitionName); }
@Override
public void deleteWorkflow(String workflowURI) {
api.deleteWorkflow(callingCtx, workflowURI); }
@Override
public String createWorkOrder(String workflowURI,Map argsMap) {
String ret = api.createWorkOrder(callingCtx, workflowURI, argsMap);
return ret; }
@Override
public CreateResponse createWorkOrderP(String workflowURI,Map argsMap,String appStatusUriPattern) {
CreateResponse ret = api.createWorkOrderP(callingCtx, workflowURI, argsMap, appStatusUriPattern);
return ret; }
@Override
public void releaseWorkOrderLock(String workOrderURI) {
api.releaseWorkOrderLock(callingCtx, workOrderURI); }
@Override
public WorkOrderStatus getWorkOrderStatus(String workOrderURI) {
WorkOrderStatus ret = api.getWorkOrderStatus(callingCtx, workOrderURI);
return ret; }
@Override
public void writeWorkflowAuditEntry(String workOrderURI,String message,Boolean error) {
api.writeWorkflowAuditEntry(callingCtx, workOrderURI, message, error); }
@Override
public List getWorkOrdersByDay(Long startTimeInstant) {
List ret = api.getWorkOrdersByDay(callingCtx, startTimeInstant);
return ret; }
@Override
public WorkOrder getWorkOrder(String workOrderURI) {
WorkOrder ret = api.getWorkOrder(callingCtx, workOrderURI);
return ret; }
@Override
public Worker getWorker(String workOrderURI,String workerId) {
Worker ret = api.getWorker(callingCtx, workOrderURI, workerId);
return ret; }
@Override
public void cancelWorkOrder(String workOrderURI) {
api.cancelWorkOrder(callingCtx, workOrderURI); }
@Override
public CreateResponse resumeWorkOrder(String workOrderURI,String resumeStepURI) {
CreateResponse ret = api.resumeWorkOrder(callingCtx, workOrderURI, resumeStepURI);
return ret; }
@Override
public Boolean wasCancelCalled(String workOrderURI) {
Boolean ret = api.wasCancelCalled(callingCtx, workOrderURI);
return ret; }
@Override
public WorkOrderCancellation getCancellationDetails(String workOrderURI) {
WorkOrderCancellation ret = api.getCancellationDetails(callingCtx, workOrderURI);
return ret; }
@Override
public WorkOrderDebug getWorkOrderDebug(String workOrderURI) {
WorkOrderDebug ret = api.getWorkOrderDebug(callingCtx, workOrderURI);
return ret; }
@Override
public void setWorkOrderIdGenConfig(String config,Boolean force) {
api.setWorkOrderIdGenConfig(callingCtx, config, force); }
@Override
public void setContextLiteral(String workerURI,String varAlias,String literalValue) {
api.setContextLiteral(callingCtx, workerURI, varAlias, literalValue); }
@Override
public void setContextLink(String workerURI,String varAlias,String expressionValue) {
api.setContextLink(callingCtx, workerURI, varAlias, expressionValue); }
@Override
public String getContextValue(String workerURI,String varAlias) {
String ret = api.getContextValue(callingCtx, workerURI, varAlias);
return ret; }
@Override
public void addErrorToContext(String workerURI,ErrorWrapper errorWrapper) {
api.addErrorToContext(callingCtx, workerURI, errorWrapper); }
@Override
public List getErrorsFromContext(String workerURI) {
List ret = api.getErrorsFromContext(callingCtx, workerURI);
return ret; }
@Override
public List getExceptionInfo(String workOrderURI) {
List ret = api.getExceptionInfo(callingCtx, workOrderURI);
return ret; }
@Override
public void reportStepProgress(String workerURI,Long stepStartTime,String message,Long progress,Long max) {
api.reportStepProgress(callingCtx, workerURI, stepStartTime, message, progress, max); }
@Override
public List getAppStatuses(String prefix) {
List ret = api.getAppStatuses(callingCtx, prefix);
return ret; }
@Override
public List getAppStatusDetails(String prefix,List extraContextValues) {
List ret = api.getAppStatusDetails(callingCtx, prefix, extraContextValues);
return ret; }
@Override
public WorkflowHistoricalMetrics getMonthlyMetrics(String workflowURI,String jobURI,String argsHashValue,String state) {
WorkflowHistoricalMetrics ret = api.getMonthlyMetrics(callingCtx, workflowURI, jobURI, argsHashValue, state);
return ret; }
@Override
public LogQueryResponse queryLogs(String workOrderURI,Long startTime,Long endTime,Long keepAlive,Long bufferSize,String nextBatchId,String stepName,String stepStartTime) {
LogQueryResponse ret = api.queryLogs(callingCtx, workOrderURI, startTime, endTime, keepAlive, bufferSize, nextBatchId, stepName, stepStartTime);
return ret; }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy