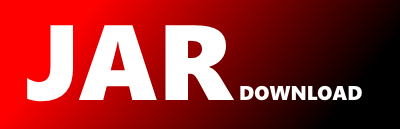
rapture.kernel.script.ScriptPipeline Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.kernel.script;
import java.util.List;
import java.util.Map;
import rapture.common.api.PipelineApi;
import rapture.common.api.ScriptPipelineApi;
import org.apache.log4j.Logger;
import rapture.common.exception.RaptureException;
import rapture.common.RapturePipelineTask;
import rapture.common.model.RaptureExchange;
import rapture.common.TableQuery;
import rapture.common.CategoryQueueBindings;
import rapture.common.PipelineTaskStatus;
@SuppressWarnings("all")
public class ScriptPipeline extends KernelScriptImplBase implements ScriptPipelineApi {
private PipelineApi api;
private static final Logger log = Logger.getLogger(ScriptPipeline.class);
public ScriptPipeline(PipelineApi api) {
this.api = api;
}
@Override
public void removeServerCategory(String category) {
api.removeServerCategory(callingCtx, category); }
@Override
public List getServerCategories() {
List ret = api.getServerCategories(callingCtx);
return ret; }
@Override
public List getBoundExchanges(String category) {
List ret = api.getBoundExchanges(callingCtx, category);
return ret; }
@Override
public void deregisterPipelineExchange(String name) {
api.deregisterPipelineExchange(callingCtx, name); }
@Override
public List getExchanges() {
List ret = api.getExchanges(callingCtx);
return ret; }
@Override
public RaptureExchange getExchange(String name) {
RaptureExchange ret = api.getExchange(callingCtx, name);
return ret; }
@Override
public void publishMessageToCategory(RapturePipelineTask task) {
api.publishMessageToCategory(callingCtx, task); }
@Override
public void broadcastMessageToCategory(RapturePipelineTask task) {
api.broadcastMessageToCategory(callingCtx, task); }
@Override
public void broadcastMessageToAll(RapturePipelineTask task) {
api.broadcastMessageToAll(callingCtx, task); }
@Override
public PipelineTaskStatus getStatus(String taskId) {
PipelineTaskStatus ret = api.getStatus(callingCtx, taskId);
return ret; }
@Override
public List queryTasks(String query) {
List ret = api.queryTasks(callingCtx, query);
return ret; }
@Override
public List queryTasksOld(TableQuery query) {
List ret = api.queryTasksOld(callingCtx, query);
return ret; }
@Override
public Long getLatestTaskEpoch() {
Long ret = api.getLatestTaskEpoch(callingCtx);
return ret; }
@Override
public void drainPipeline(String exchange) {
api.drainPipeline(callingCtx, exchange); }
@Override
public void registerExchangeDomain(String domainURI,String config) {
api.registerExchangeDomain(callingCtx, domainURI, config); }
@Override
public void deregisterExchangeDomain(String domainURI) {
api.deregisterExchangeDomain(callingCtx, domainURI); }
@Override
public List getExchangeDomains() {
List ret = api.getExchangeDomains(callingCtx);
return ret; }
@Override
public void setupStandardCategory(String category) {
api.setupStandardCategory(callingCtx, category); }
@Override
public Map makeRPC(String queueName,String fnName,Map params,Long timeoutInSeconds) {
Map ret = api.makeRPC(callingCtx, queueName, fnName, params, timeoutInSeconds);
return ret; }
@Override
public void createTopicExchange(String domain,String exchange) {
api.createTopicExchange(callingCtx, domain, exchange); }
@Override
public void publishTopicMessage(String domain,String exchange,String topic,String message) {
api.publishTopicMessage(callingCtx, domain, exchange, topic, message); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy