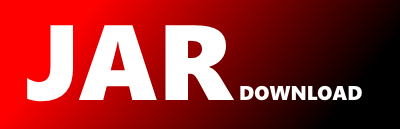
rapture.sheet.memory.InMemorySheet Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (c) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package rapture.sheet.memory;
import rapture.common.RaptureSheetCell;
import rapture.common.RaptureSheetNote;
import rapture.common.RaptureSheetRange;
import rapture.common.RaptureSheetScript;
import rapture.common.RaptureSheetStatus;
import rapture.common.RaptureSheetStyle;
import rapture.common.impl.jackson.JacksonUtil;
import rapture.util.IDGenerator;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.collect.HashBasedTable;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Table;
import com.google.common.collect.Table.Cell;
/**
* The implementation of an in memory Rapture sheet.
*
* @author amkimian
*/
public class InMemorySheet {
@JsonProperty
private Map> dimToData;
private int maxColumn = 0;
private int maxRow = 0;
private Map scriptMap;
private Map noteMap;
private Map rangeMap;
private Map styleMap;
public InMemorySheet() {
dimToData = new HashMap>();
scriptMap = new HashMap();
noteMap = new HashMap();
rangeMap = new HashMap();
styleMap = new HashMap();
}
@SuppressWarnings("unchecked")
public InMemorySheet(InMemorySheet source) {
this.dimToData = new HashMap>();
for (Integer dim : source.dimToData.keySet()) {
Table table = source.dimToData.get(dim);
dimToData.put(dim, HashBasedTable.create(table));
}
this.scriptMap = ((Map) ((Object) JacksonUtil.getMapFromJson(JacksonUtil.jsonFromObject(source.scriptMap))));
this.noteMap = (Map) (Object) JacksonUtil.getMapFromJson(JacksonUtil.jsonFromObject(source.noteMap));
this.rangeMap = (Map) (Object) JacksonUtil.getMapFromJson(JacksonUtil.jsonFromObject(source.rangeMap));
this.styleMap = (Map) (Object) JacksonUtil.getMapFromJson(JacksonUtil.jsonFromObject(source.styleMap));
}
public void setCell(int dim, int row, int col, String val) {
Table table = dimToData.get(dim);
if (table == null) {
table = HashBasedTable.create();
dimToData.put(dim, table);
}
setCell(table, row, col, val);
}
private void setCell(Table table, int row, int column, String value) {
RaptureSheetCell cell = new RaptureSheetCell();
cell.setRow(row);
cell.setColumn(column);
cell.setData(value);
Long epoch = System.currentTimeMillis() / 1000;
cell.setEpoch(epoch);
table.put(row, column, cell);
if (row > maxRow) {
maxRow = row;
}
if (column > maxColumn) {
maxColumn = column;
}
}
public int getMaxRow() {
return maxRow;
}
public int getMaxColumn() {
return maxColumn;
}
public String getCell(int dimension, int row, int col) {
Table table = dimToData.get(dimension);
if (table != null) {
RaptureSheetCell cell = table.get(row, col);
if (cell != null) {
return cell.getData();
} else {
return null;
}
} else {
return null;
}
}
public RaptureSheetStatus getCells(Integer dimension, Long minEpochFilter) {
Table table = dimToData.get(dimension);
Set> cellSet = table.cellSet();
List cells = new LinkedList();
Long maxEpoch = 0L;
for (Cell cell : cellSet) {
RaptureSheetCell sheetCell = cell.getValue();
if (sheetCell.getEpoch() > minEpochFilter) {
cells.add(sheetCell);
if (maxEpoch < sheetCell.getEpoch()) {
maxEpoch = sheetCell.getEpoch();
}
}
}
RaptureSheetStatus ret = new RaptureSheetStatus();
ret.setCells(cells);
ret.setEpoch(maxEpoch);
return ret;
}
public Boolean delete(int row, int column, int dimension) {
Table table = dimToData.get(dimension);
if (table == null) {
return false;
} else {
return table.remove(row, column) != null;
}
}
public Boolean delete(int row, int col) {
for (Integer dimension : dimToData.keySet()) {
delete(row, col, dimension);
}
return true;
}
public Set getDimensions() {
return dimToData.keySet();
}
public RaptureSheetScript getSheetScript(String scriptName) {
return scriptMap.get(scriptName);
}
public List getSheetScripts() {
return ImmutableList.builder().addAll(scriptMap.values()).build();
}
public Boolean deleteSheetScript(String scriptName) {
return scriptMap.remove(scriptName) != null;
}
public RaptureSheetScript putSheetScript(String scriptName, RaptureSheetScript script) {
RaptureSheetScript storedScript = JacksonUtil.objectFromJson(JacksonUtil.jsonFromObject(script), RaptureSheetScript.class);
return scriptMap.put(scriptName, storedScript);
}
public List getSheetNotes() {
return ImmutableList.builder().addAll(noteMap.values()).build();
}
public Boolean deleteSheetNote(String noteId) {
return noteMap.remove(noteId) != null;
}
public RaptureSheetNote putSheetNote(RaptureSheetNote note) {
if (note.getId() == null || note.getId().isEmpty()) {
note.setId(IDGenerator.getUUID());
}
RaptureSheetNote storableNote = JacksonUtil.objectFromJson(JacksonUtil.jsonFromObject(note), RaptureSheetNote.class);
return noteMap.put(storableNote.getId(), storableNote);
}
public RaptureSheetRange putSheetNamedSelection(String rangeName, RaptureSheetRange range) {
RaptureSheetRange storable = JacksonUtil.objectFromJson(JacksonUtil.jsonFromObject(range), RaptureSheetRange.class);
return rangeMap.put(rangeName, storable);
}
public Boolean deleteSheetNamedSelection(String rangeName) {
return rangeMap.remove(rangeName) != null;
}
public RaptureSheetRange getSheetNamedSelection(String rangeName) {
return rangeMap.get(rangeName);
}
public List getSheetNamedSelections() {
return ImmutableList.builder().addAll(rangeMap.values()).build();
}
public RaptureSheetStyle putSheetStyle(RaptureSheetStyle style) {
RaptureSheetStyle storable = JacksonUtil.objectFromJson(JacksonUtil.jsonFromObject(style), RaptureSheetStyle.class);
return styleMap.put(style.getName(), storable);
}
public Boolean deleteSheetStyle(String styleName) {
return styleMap.remove(styleName) != null;
}
public List getSheetStyles() {
return ImmutableList.builder().addAll(styleMap.values()).build();
}
}
|
© 2015 - 2025 Weber Informatics LLC | Privacy Policy