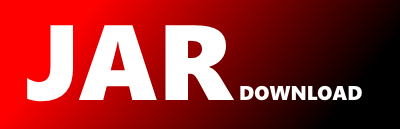
rapture.table.memory.MemoryIndexHandler Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (c) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package rapture.table.memory;
import rapture.common.TableQuery;
import rapture.common.TableQueryResult;
import rapture.common.TableRecord;
import rapture.common.model.DocumentMetadata;
import rapture.dsl.iqry.IndexQuery;
import rapture.dsl.iqry.IndexQueryFactory;
import rapture.dsl.iqry.OrderDirection;
import rapture.dsl.iqry.WhereClause;
import rapture.dsl.iqry.WhereExtension;
import rapture.dsl.iqry.WhereStatement;
import rapture.index.IndexHandler;
import rapture.index.IndexProducer;
import rapture.index.IndexRecord;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.apache.log4j.Logger;
import com.google.common.base.Predicate;
/*
* An in memory table, primarily for testing
*
*/
public class MemoryIndexHandler implements IndexHandler {
protected static Logger log = Logger.getLogger(MemoryIndexHandler.class);
protected Map> memoryView = null;
private IndexProducer indexProducer;
public MemoryIndexHandler() {
reset();
}
@Override
public void deleteTable() {
log.info("Removing index content ");
memoryView.clear();
}
@Override
public void removeAll(String rowId) {
memoryView.put(rowId, new HashMap());
}
private void reset() {
memoryView = new HashMap<>();
}
@Override
public void setConfig(Map config) {
log.info("Creating memory queue with config " + config.toString());
reset();
}
@Override
public void addedRecord(String key, String value, DocumentMetadata mdLatest) {
List records = indexProducer.getIndexRecords(key, value, mdLatest);
for (IndexRecord record : records) {
memoryView.put(key, record.getValues());
memoryView.get(key).put(ROWID, key);
}
}
@Override
public void updateRow(String key, Map recordValues) {
memoryView.put(key, recordValues);
}
@Override
public void ensureIndicesExist() {
//noop
}
@Override
public List queryTable(TableQuery query) {
return new ArrayList();
}
@Override
public void setInstanceName(String instanceName) {
//unused -- ignore
}
@Override
public TableQueryResult query(String query) {
TableQueryResult result = new TableQueryResult();
IndexQuery indexQuery = IndexQueryFactory.parseQuery(query);
Map> data = memoryView;
List>> predicates = predicatesFromQuery(indexQuery);
List columnNames = indexQuery.getSelect().getFieldList();
result.setColumnNames(columnNames);
List> rows = new LinkedList<>();
for (Map.Entry> mainEntry : data.entrySet()) {
boolean isGood = true;
Map body = mainEntry.getValue();
for (Predicate
© 2015 - 2025 Weber Informatics LLC | Privacy Policy