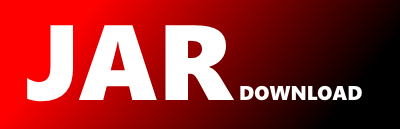
rapture.generated.DParseLexer Maven / Gradle / Ivy
// $ANTLR 3.5.2 /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g 2016-04-15 08:33:46
package rapture.generated;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings("all")
public class DParseLexer extends Lexer {
public static final int EOF=-1;
public static final int AGO=4;
public static final int AND=5;
public static final int COLON=6;
public static final int COMMA=7;
public static final int DASH=8;
public static final int DAY=9;
public static final int DIGIT=10;
public static final int DOLLAR=11;
public static final int DOT=12;
public static final int HASH=13;
public static final int HOUR=14;
public static final int LETTER=15;
public static final int MINUTE=16;
public static final int MONTH=17;
public static final int PLUS=18;
public static final int QUERY=19;
public static final int SECOND=20;
public static final int SINGLE_QUOTE=21;
public static final int SLASH=22;
public static final int SPACE=23;
public static final int STRING=24;
public static final int TODAY=25;
public static final int TOMORROW=26;
public static final int TONIGHT=27;
public static final int UNDER=28;
public static final int WEEK=29;
public static final int YEAR=30;
public static final int YESTERDAY=31;
// delegates
// delegators
public Lexer[] getDelegates() {
return new Lexer[] {};
}
public DParseLexer() {}
public DParseLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public DParseLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
@Override public String getGrammarFileName() { return "/Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g"; }
// $ANTLR start "HOUR"
public final void mHOUR() throws RecognitionException {
try {
int _type = HOUR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:20:8: ( '!hour' | '!hours' | '!hr' ( DOT )? | '!hrs' ( DOT )? )
int alt3=4;
int LA3_0 = input.LA(1);
if ( (LA3_0=='!') ) {
int LA3_1 = input.LA(2);
if ( (LA3_1=='h') ) {
int LA3_2 = input.LA(3);
if ( (LA3_2=='o') ) {
int LA3_3 = input.LA(4);
if ( (LA3_3=='u') ) {
int LA3_5 = input.LA(5);
if ( (LA3_5=='r') ) {
int LA3_8 = input.LA(6);
if ( (LA3_8=='s') ) {
alt3=2;
}
else {
alt3=1;
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 5 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 3, 5, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 4 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 3, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else if ( (LA3_2=='r') ) {
int LA3_4 = input.LA(4);
if ( (LA3_4=='s') ) {
alt3=4;
}
else {
alt3=3;
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 3, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 3, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 3, 0, input);
throw nvae;
}
switch (alt3) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:20:10: '!hour'
{
match("!hour");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:20:22: '!hours'
{
match("!hours");
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:20:35: '!hr' ( DOT )?
{
match("!hr");
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:20:41: ( DOT )?
int alt1=2;
int LA1_0 = input.LA(1);
if ( (LA1_0=='.') ) {
alt1=1;
}
switch (alt1) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:
{
if ( input.LA(1)=='.' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
}
}
break;
case 4 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:20:49: '!hrs' ( DOT )?
{
match("!hrs");
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:20:56: ( DOT )?
int alt2=2;
int LA2_0 = input.LA(1);
if ( (LA2_0=='.') ) {
alt2=1;
}
switch (alt2) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:
{
if ( input.LA(1)=='.' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
}
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "HOUR"
// $ANTLR start "MINUTE"
public final void mMINUTE() throws RecognitionException {
try {
int _type = MINUTE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:21:8: ( '!minute' | '!minutes' | '!min' ( DOT )? | '!mins' ( DOT )? )
int alt6=4;
int LA6_0 = input.LA(1);
if ( (LA6_0=='!') ) {
int LA6_1 = input.LA(2);
if ( (LA6_1=='m') ) {
int LA6_2 = input.LA(3);
if ( (LA6_2=='i') ) {
int LA6_3 = input.LA(4);
if ( (LA6_3=='n') ) {
switch ( input.LA(5) ) {
case 'u':
{
int LA6_5 = input.LA(6);
if ( (LA6_5=='t') ) {
int LA6_8 = input.LA(7);
if ( (LA6_8=='e') ) {
int LA6_9 = input.LA(8);
if ( (LA6_9=='s') ) {
alt6=2;
}
else {
alt6=1;
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 7 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 6, 8, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 6 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 6, 5, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case 's':
{
alt6=4;
}
break;
default:
alt6=3;
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 4 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 6, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 6, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 6, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 6, 0, input);
throw nvae;
}
switch (alt6) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:21:10: '!minute'
{
match("!minute");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:21:22: '!minutes'
{
match("!minutes");
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:21:35: '!min' ( DOT )?
{
match("!min");
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:21:42: ( DOT )?
int alt4=2;
int LA4_0 = input.LA(1);
if ( (LA4_0=='.') ) {
alt4=1;
}
switch (alt4) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:
{
if ( input.LA(1)=='.' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
}
}
break;
case 4 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:21:49: '!mins' ( DOT )?
{
match("!mins");
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:21:57: ( DOT )?
int alt5=2;
int LA5_0 = input.LA(1);
if ( (LA5_0=='.') ) {
alt5=1;
}
switch (alt5) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:
{
if ( input.LA(1)=='.' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
}
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MINUTE"
// $ANTLR start "DAY"
public final void mDAY() throws RecognitionException {
try {
int _type = DAY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:22:8: ( '!day' | '!days' )
int alt7=2;
int LA7_0 = input.LA(1);
if ( (LA7_0=='!') ) {
int LA7_1 = input.LA(2);
if ( (LA7_1=='d') ) {
int LA7_2 = input.LA(3);
if ( (LA7_2=='a') ) {
int LA7_3 = input.LA(4);
if ( (LA7_3=='y') ) {
int LA7_4 = input.LA(5);
if ( (LA7_4=='s') ) {
alt7=2;
}
else {
alt7=1;
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 4 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 7, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 7, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 7, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 7, 0, input);
throw nvae;
}
switch (alt7) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:22:10: '!day'
{
match("!day");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:22:22: '!days'
{
match("!days");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DAY"
// $ANTLR start "WEEK"
public final void mWEEK() throws RecognitionException {
try {
int _type = WEEK;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:23:8: ( '!week' | '!weeks' | '!wks' ( DOT )? )
int alt9=3;
int LA9_0 = input.LA(1);
if ( (LA9_0=='!') ) {
int LA9_1 = input.LA(2);
if ( (LA9_1=='w') ) {
int LA9_2 = input.LA(3);
if ( (LA9_2=='e') ) {
int LA9_3 = input.LA(4);
if ( (LA9_3=='e') ) {
int LA9_5 = input.LA(5);
if ( (LA9_5=='k') ) {
int LA9_6 = input.LA(6);
if ( (LA9_6=='s') ) {
alt9=2;
}
else {
alt9=1;
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 5 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 9, 5, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 4 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 9, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else if ( (LA9_2=='k') ) {
alt9=3;
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 9, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 9, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 9, 0, input);
throw nvae;
}
switch (alt9) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:23:10: '!week'
{
match("!week");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:23:22: '!weeks'
{
match("!weeks");
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:23:35: '!wks' ( DOT )?
{
match("!wks");
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:23:42: ( DOT )?
int alt8=2;
int LA8_0 = input.LA(1);
if ( (LA8_0=='.') ) {
alt8=1;
}
switch (alt8) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:
{
if ( input.LA(1)=='.' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
}
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "WEEK"
// $ANTLR start "MONTH"
public final void mMONTH() throws RecognitionException {
try {
int _type = MONTH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:24:8: ( '!month' | '!months' )
int alt10=2;
int LA10_0 = input.LA(1);
if ( (LA10_0=='!') ) {
int LA10_1 = input.LA(2);
if ( (LA10_1=='m') ) {
int LA10_2 = input.LA(3);
if ( (LA10_2=='o') ) {
int LA10_3 = input.LA(4);
if ( (LA10_3=='n') ) {
int LA10_4 = input.LA(5);
if ( (LA10_4=='t') ) {
int LA10_5 = input.LA(6);
if ( (LA10_5=='h') ) {
int LA10_6 = input.LA(7);
if ( (LA10_6=='s') ) {
alt10=2;
}
else {
alt10=1;
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 6 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 10, 5, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 5 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 10, 4, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 4 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 10, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 10, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 10, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 10, 0, input);
throw nvae;
}
switch (alt10) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:24:10: '!month'
{
match("!month");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:24:22: '!months'
{
match("!months");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MONTH"
// $ANTLR start "YEAR"
public final void mYEAR() throws RecognitionException {
try {
int _type = YEAR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:25:8: ( '!year' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:25:10: '!year'
{
match("!year");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "YEAR"
// $ANTLR start "TODAY"
public final void mTODAY() throws RecognitionException {
try {
int _type = TODAY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:27:11: ( '!today' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:27:13: '!today'
{
match("!today");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "TODAY"
// $ANTLR start "TOMORROW"
public final void mTOMORROW() throws RecognitionException {
try {
int _type = TOMORROW;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:28:11: ( '!tomorow' | '!tomorrow' | '!tommorow' | '!tommorrow' )
int alt11=4;
int LA11_0 = input.LA(1);
if ( (LA11_0=='!') ) {
int LA11_1 = input.LA(2);
if ( (LA11_1=='t') ) {
int LA11_2 = input.LA(3);
if ( (LA11_2=='o') ) {
int LA11_3 = input.LA(4);
if ( (LA11_3=='m') ) {
int LA11_4 = input.LA(5);
if ( (LA11_4=='o') ) {
int LA11_5 = input.LA(6);
if ( (LA11_5=='r') ) {
int LA11_7 = input.LA(7);
if ( (LA11_7=='o') ) {
alt11=1;
}
else if ( (LA11_7=='r') ) {
alt11=2;
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 7 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 11, 7, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 6 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 11, 5, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else if ( (LA11_4=='m') ) {
int LA11_6 = input.LA(6);
if ( (LA11_6=='o') ) {
int LA11_8 = input.LA(7);
if ( (LA11_8=='r') ) {
int LA11_11 = input.LA(8);
if ( (LA11_11=='o') ) {
alt11=3;
}
else if ( (LA11_11=='r') ) {
alt11=4;
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 8 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 11, 11, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 7 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 11, 8, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 6 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 11, 6, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 5 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 11, 4, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 4 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 11, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 11, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 11, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 11, 0, input);
throw nvae;
}
switch (alt11) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:28:13: '!tomorow'
{
match("!tomorow");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:28:28: '!tomorrow'
{
match("!tomorrow");
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:28:44: '!tommorow'
{
match("!tommorow");
}
break;
case 4 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:28:58: '!tommorrow'
{
match("!tommorrow");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "TOMORROW"
// $ANTLR start "TONIGHT"
public final void mTONIGHT() throws RecognitionException {
try {
int _type = TONIGHT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:29:11: ( '!tonight' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:29:13: '!tonight'
{
match("!tonight");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "TONIGHT"
// $ANTLR start "YESTERDAY"
public final void mYESTERDAY() throws RecognitionException {
try {
int _type = YESTERDAY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:30:11: ( '!yesterday' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:30:13: '!yesterday'
{
match("!yesterday");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "YESTERDAY"
// $ANTLR start "SECOND"
public final void mSECOND() throws RecognitionException {
try {
int _type = SECOND;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:34:16: ( '!second' | '!seconds' )
int alt12=2;
int LA12_0 = input.LA(1);
if ( (LA12_0=='!') ) {
int LA12_1 = input.LA(2);
if ( (LA12_1=='s') ) {
int LA12_2 = input.LA(3);
if ( (LA12_2=='e') ) {
int LA12_3 = input.LA(4);
if ( (LA12_3=='c') ) {
int LA12_4 = input.LA(5);
if ( (LA12_4=='o') ) {
int LA12_5 = input.LA(6);
if ( (LA12_5=='n') ) {
int LA12_6 = input.LA(7);
if ( (LA12_6=='d') ) {
int LA12_7 = input.LA(8);
if ( (LA12_7=='s') ) {
alt12=2;
}
else {
alt12=1;
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 7 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 12, 6, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 6 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 12, 5, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 5 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 12, 4, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 4 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 12, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 12, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 12, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 12, 0, input);
throw nvae;
}
switch (alt12) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:34:18: '!second'
{
match("!second");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:34:30: '!seconds'
{
match("!seconds");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SECOND"
// $ANTLR start "HASH"
public final void mHASH() throws RecognitionException {
try {
int _type = HASH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:38:7: ( '#' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:38:9: '#'
{
match('#');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "HASH"
// $ANTLR start "COLON"
public final void mCOLON() throws RecognitionException {
try {
int _type = COLON;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:39:7: ( ':' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:39:9: ':'
{
match(':');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COLON"
// $ANTLR start "COMMA"
public final void mCOMMA() throws RecognitionException {
try {
int _type = COMMA;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:40:7: ( ',' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:40:9: ','
{
match(',');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COMMA"
// $ANTLR start "DASH"
public final void mDASH() throws RecognitionException {
try {
int _type = DASH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:41:7: ( '-' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:41:9: '-'
{
match('-');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DASH"
// $ANTLR start "SLASH"
public final void mSLASH() throws RecognitionException {
try {
int _type = SLASH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:42:7: ( '/' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:42:9: '/'
{
match('/');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SLASH"
// $ANTLR start "DOT"
public final void mDOT() throws RecognitionException {
try {
int _type = DOT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:43:7: ( '.' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:43:9: '.'
{
match('.');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DOT"
// $ANTLR start "PLUS"
public final void mPLUS() throws RecognitionException {
try {
int _type = PLUS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:44:7: ( '+' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:44:9: '+'
{
match('+');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "PLUS"
// $ANTLR start "DOLLAR"
public final void mDOLLAR() throws RecognitionException {
try {
int _type = DOLLAR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:45:8: ( '$' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:45:10: '$'
{
match('$');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DOLLAR"
// $ANTLR start "SINGLE_QUOTE"
public final void mSINGLE_QUOTE() throws RecognitionException {
try {
int _type = SINGLE_QUOTE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:46:14: ( '\\'' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:46:16: '\\''
{
match('\'');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SINGLE_QUOTE"
// $ANTLR start "QUERY"
public final void mQUERY() throws RecognitionException {
try {
int _type = QUERY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:47:7: ( '@' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:47:9: '@'
{
match('@');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "QUERY"
// $ANTLR start "UNDER"
public final void mUNDER() throws RecognitionException {
try {
int _type = UNDER;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:48:7: ( '_' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:48:9: '_'
{
match('_');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "UNDER"
// $ANTLR start "SPACE"
public final void mSPACE() throws RecognitionException {
try {
int _type = SPACE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:49:7: ( ' ' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:49:9: ' '
{
match(' ');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SPACE"
// $ANTLR start "DIGIT"
public final void mDIGIT() throws RecognitionException {
try {
int _type = DIGIT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:51:7: ( '0' .. '9' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DIGIT"
// $ANTLR start "LETTER"
public final void mLETTER() throws RecognitionException {
try {
int _type = LETTER;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:52:8: ( 'a' .. 'z' | 'A' .. 'Z' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:
{
if ( (input.LA(1) >= 'A' && input.LA(1) <= 'Z')||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LETTER"
// $ANTLR start "AGO"
public final void mAGO() throws RecognitionException {
try {
int _type = AGO;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:54:11: ( '!ago' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:54:13: '!ago'
{
match("!ago");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "AGO"
// $ANTLR start "AND"
public final void mAND() throws RecognitionException {
try {
int _type = AND;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:55:11: ( '!and' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:55:13: '!and'
{
match("!and");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "AND"
// $ANTLR start "STRING"
public final void mSTRING() throws RecognitionException {
try {
int _type = STRING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:57:8: ( '\"' (~ '\"' )* '\"' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:57:10: '\"' (~ '\"' )* '\"'
{
match('\"');
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:57:14: (~ '\"' )*
loop13:
while (true) {
int alt13=2;
int LA13_0 = input.LA(1);
if ( ((LA13_0 >= '\u0000' && LA13_0 <= '!')||(LA13_0 >= '#' && LA13_0 <= '\uFFFF')) ) {
alt13=1;
}
switch (alt13) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '!')||(input.LA(1) >= '#' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop13;
}
}
match('\"');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "STRING"
@Override
public void mTokens() throws RecognitionException {
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:8: ( HOUR | MINUTE | DAY | WEEK | MONTH | YEAR | TODAY | TOMORROW | TONIGHT | YESTERDAY | SECOND | HASH | COLON | COMMA | DASH | SLASH | DOT | PLUS | DOLLAR | SINGLE_QUOTE | QUERY | UNDER | SPACE | DIGIT | LETTER | AGO | AND | STRING )
int alt14=28;
switch ( input.LA(1) ) {
case '!':
{
switch ( input.LA(2) ) {
case 'h':
{
alt14=1;
}
break;
case 'm':
{
int LA14_18 = input.LA(3);
if ( (LA14_18=='i') ) {
alt14=2;
}
else if ( (LA14_18=='o') ) {
alt14=5;
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 14, 18, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case 'd':
{
alt14=3;
}
break;
case 'w':
{
alt14=4;
}
break;
case 'y':
{
int LA14_21 = input.LA(3);
if ( (LA14_21=='e') ) {
int LA14_27 = input.LA(4);
if ( (LA14_27=='a') ) {
alt14=6;
}
else if ( (LA14_27=='s') ) {
alt14=10;
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 4 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 14, 27, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 14, 21, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case 't':
{
int LA14_22 = input.LA(3);
if ( (LA14_22=='o') ) {
switch ( input.LA(4) ) {
case 'd':
{
alt14=7;
}
break;
case 'm':
{
alt14=8;
}
break;
case 'n':
{
alt14=9;
}
break;
default:
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 4 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 14, 28, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 14, 22, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case 's':
{
alt14=11;
}
break;
case 'a':
{
int LA14_24 = input.LA(3);
if ( (LA14_24=='g') ) {
alt14=26;
}
else if ( (LA14_24=='n') ) {
alt14=27;
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 14, 24, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
default:
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 14, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case '#':
{
alt14=12;
}
break;
case ':':
{
alt14=13;
}
break;
case ',':
{
alt14=14;
}
break;
case '-':
{
alt14=15;
}
break;
case '/':
{
alt14=16;
}
break;
case '.':
{
alt14=17;
}
break;
case '+':
{
alt14=18;
}
break;
case '$':
{
alt14=19;
}
break;
case '\'':
{
alt14=20;
}
break;
case '@':
{
alt14=21;
}
break;
case '_':
{
alt14=22;
}
break;
case ' ':
{
alt14=23;
}
break;
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
{
alt14=24;
}
break;
case 'A':
case 'B':
case 'C':
case 'D':
case 'E':
case 'F':
case 'G':
case 'H':
case 'I':
case 'J':
case 'K':
case 'L':
case 'M':
case 'N':
case 'O':
case 'P':
case 'Q':
case 'R':
case 'S':
case 'T':
case 'U':
case 'V':
case 'W':
case 'X':
case 'Y':
case 'Z':
case 'a':
case 'b':
case 'c':
case 'd':
case 'e':
case 'f':
case 'g':
case 'h':
case 'i':
case 'j':
case 'k':
case 'l':
case 'm':
case 'n':
case 'o':
case 'p':
case 'q':
case 'r':
case 's':
case 't':
case 'u':
case 'v':
case 'w':
case 'x':
case 'y':
case 'z':
{
alt14=25;
}
break;
case '\"':
{
alt14=28;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 14, 0, input);
throw nvae;
}
switch (alt14) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:10: HOUR
{
mHOUR();
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:15: MINUTE
{
mMINUTE();
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:22: DAY
{
mDAY();
}
break;
case 4 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:26: WEEK
{
mWEEK();
}
break;
case 5 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:31: MONTH
{
mMONTH();
}
break;
case 6 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:37: YEAR
{
mYEAR();
}
break;
case 7 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:42: TODAY
{
mTODAY();
}
break;
case 8 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:48: TOMORROW
{
mTOMORROW();
}
break;
case 9 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:57: TONIGHT
{
mTONIGHT();
}
break;
case 10 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:65: YESTERDAY
{
mYESTERDAY();
}
break;
case 11 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:75: SECOND
{
mSECOND();
}
break;
case 12 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:82: HASH
{
mHASH();
}
break;
case 13 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:87: COLON
{
mCOLON();
}
break;
case 14 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:93: COMMA
{
mCOMMA();
}
break;
case 15 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:99: DASH
{
mDASH();
}
break;
case 16 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:104: SLASH
{
mSLASH();
}
break;
case 17 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:110: DOT
{
mDOT();
}
break;
case 18 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:114: PLUS
{
mPLUS();
}
break;
case 19 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:119: DOLLAR
{
mDOLLAR();
}
break;
case 20 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:126: SINGLE_QUOTE
{
mSINGLE_QUOTE();
}
break;
case 21 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:139: QUERY
{
mQUERY();
}
break;
case 22 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:145: UNDER
{
mUNDER();
}
break;
case 23 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:151: SPACE
{
mSPACE();
}
break;
case 24 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:157: DIGIT
{
mDIGIT();
}
break;
case 25 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:163: LETTER
{
mLETTER();
}
break;
case 26 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:170: AGO
{
mAGO();
}
break;
case 27 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:174: AND
{
mAND();
}
break;
case 28 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/dparse/DParseLexer.g:1:178: STRING
{
mSTRING();
}
break;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy