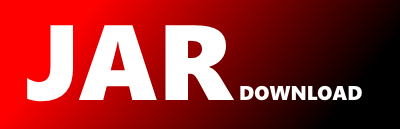
rapture.generated.IndexQueryLexer Maven / Gradle / Ivy
// $ANTLR 3.5.2 /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g 2016-04-15 08:33:46
package rapture.generated;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings("all")
public class IndexQueryLexer extends Lexer {
public static final int EOF=-1;
public static final int AND=4;
public static final int ASC=5;
public static final int BY=6;
public static final int CBRAC=7;
public static final int COMMA=8;
public static final int DESC=9;
public static final int DISTINCT=10;
public static final int EQUAL=11;
public static final int GT=12;
public static final int ID=13;
public static final int LIMIT=14;
public static final int LT=15;
public static final int NOTEQUAL=16;
public static final int NUMBER=17;
public static final int OBRAC=18;
public static final int OR=19;
public static final int ORDER=20;
public static final int SELECT=21;
public static final int STRING=22;
public static final int WHERE=23;
public static final int WS=24;
// delegates
// delegators
public Lexer[] getDelegates() {
return new Lexer[] {};
}
public IndexQueryLexer() {}
public IndexQueryLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public IndexQueryLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
@Override public String getGrammarFileName() { return "/Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g"; }
// $ANTLR start "DISTINCT"
public final void mDISTINCT() throws RecognitionException {
try {
int _type = DISTINCT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:18:10: ( 'DISTINCT' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:18:12: 'DISTINCT'
{
match("DISTINCT");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DISTINCT"
// $ANTLR start "SELECT"
public final void mSELECT() throws RecognitionException {
try {
int _type = SELECT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:19:8: ( 'SELECT' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:19:10: 'SELECT'
{
match("SELECT");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SELECT"
// $ANTLR start "WHERE"
public final void mWHERE() throws RecognitionException {
try {
int _type = WHERE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:20:8: ( 'WHERE' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:20:10: 'WHERE'
{
match("WHERE");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "WHERE"
// $ANTLR start "ORDER"
public final void mORDER() throws RecognitionException {
try {
int _type = ORDER;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:21:8: ( 'ORDER' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:21:10: 'ORDER'
{
match("ORDER");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ORDER"
// $ANTLR start "BY"
public final void mBY() throws RecognitionException {
try {
int _type = BY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:22:8: ( 'BY' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:22:10: 'BY'
{
match("BY");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "BY"
// $ANTLR start "ASC"
public final void mASC() throws RecognitionException {
try {
int _type = ASC;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:23:8: ( 'ASC' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:23:10: 'ASC'
{
match("ASC");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ASC"
// $ANTLR start "DESC"
public final void mDESC() throws RecognitionException {
try {
int _type = DESC;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:24:8: ( 'DESC' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:24:10: 'DESC'
{
match("DESC");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DESC"
// $ANTLR start "EQUAL"
public final void mEQUAL() throws RecognitionException {
try {
int _type = EQUAL;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:25:8: ( '=' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:25:10: '='
{
match('=');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "EQUAL"
// $ANTLR start "GT"
public final void mGT() throws RecognitionException {
try {
int _type = GT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:26:8: ( '>' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:26:10: '>'
{
match('>');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "GT"
// $ANTLR start "LT"
public final void mLT() throws RecognitionException {
try {
int _type = LT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:27:8: ( '<' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:27:10: '<'
{
match('<');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LT"
// $ANTLR start "NOTEQUAL"
public final void mNOTEQUAL() throws RecognitionException {
try {
int _type = NOTEQUAL;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:28:10: ( '!=' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:28:12: '!='
{
match("!=");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NOTEQUAL"
// $ANTLR start "COMMA"
public final void mCOMMA() throws RecognitionException {
try {
int _type = COMMA;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:29:8: ( ',' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:29:10: ','
{
match(',');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COMMA"
// $ANTLR start "OBRAC"
public final void mOBRAC() throws RecognitionException {
try {
int _type = OBRAC;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:30:7: ( '(' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:30:9: '('
{
match('(');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "OBRAC"
// $ANTLR start "CBRAC"
public final void mCBRAC() throws RecognitionException {
try {
int _type = CBRAC;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:31:7: ( ')' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:31:9: ')'
{
match(')');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "CBRAC"
// $ANTLR start "AND"
public final void mAND() throws RecognitionException {
try {
int _type = AND;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:32:7: ( 'AND' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:32:9: 'AND'
{
match("AND");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "AND"
// $ANTLR start "OR"
public final void mOR() throws RecognitionException {
try {
int _type = OR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:33:7: ( 'OR' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:33:9: 'OR'
{
match("OR");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "OR"
// $ANTLR start "LIMIT"
public final void mLIMIT() throws RecognitionException {
try {
int _type = LIMIT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:34:7: ( 'LIMIT' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:34:9: 'LIMIT'
{
match("LIMIT");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LIMIT"
// $ANTLR start "NUMBER"
public final void mNUMBER() throws RecognitionException {
try {
int _type = NUMBER;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:36:8: ( ( '0' .. '9' )+ )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:36:10: ( '0' .. '9' )+
{
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:36:10: ( '0' .. '9' )+
int cnt1=0;
loop1:
while (true) {
int alt1=2;
int LA1_0 = input.LA(1);
if ( ((LA1_0 >= '0' && LA1_0 <= '9')) ) {
alt1=1;
}
switch (alt1) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt1 >= 1 ) break loop1;
EarlyExitException eee = new EarlyExitException(1, input);
throw eee;
}
cnt1++;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NUMBER"
// $ANTLR start "ID"
public final void mID() throws RecognitionException {
try {
int _type = ID;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:37:4: ( ( 'a' .. 'z' | 'A' .. 'Z' | '_' ) ( 'a' .. 'z' | 'A' .. 'Z' | '0' .. '9' | '_' )* )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:37:6: ( 'a' .. 'z' | 'A' .. 'Z' | '_' ) ( 'a' .. 'z' | 'A' .. 'Z' | '0' .. '9' | '_' )*
{
if ( (input.LA(1) >= 'A' && input.LA(1) <= 'Z')||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:37:30: ( 'a' .. 'z' | 'A' .. 'Z' | '0' .. '9' | '_' )*
loop2:
while (true) {
int alt2=2;
int LA2_0 = input.LA(1);
if ( ((LA2_0 >= '0' && LA2_0 <= '9')||(LA2_0 >= 'A' && LA2_0 <= 'Z')||LA2_0=='_'||(LA2_0 >= 'a' && LA2_0 <= 'z')) ) {
alt2=1;
}
switch (alt2) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9')||(input.LA(1) >= 'A' && input.LA(1) <= 'Z')||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop2;
}
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ID"
// $ANTLR start "STRING"
public final void mSTRING() throws RecognitionException {
try {
int _type = STRING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:38:8: ( '\"' (~ '\"' )* '\"' | '\\'' (~ '\\'' )* '\\'' )
int alt5=2;
int LA5_0 = input.LA(1);
if ( (LA5_0=='\"') ) {
alt5=1;
}
else if ( (LA5_0=='\'') ) {
alt5=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 5, 0, input);
throw nvae;
}
switch (alt5) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:38:10: '\"' (~ '\"' )* '\"'
{
match('\"');
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:38:14: (~ '\"' )*
loop3:
while (true) {
int alt3=2;
int LA3_0 = input.LA(1);
if ( ((LA3_0 >= '\u0000' && LA3_0 <= '!')||(LA3_0 >= '#' && LA3_0 <= '\uFFFF')) ) {
alt3=1;
}
switch (alt3) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '!')||(input.LA(1) >= '#' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop3;
}
}
match('\"');
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:39:10: '\\'' (~ '\\'' )* '\\''
{
match('\'');
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:39:15: (~ '\\'' )*
loop4:
while (true) {
int alt4=2;
int LA4_0 = input.LA(1);
if ( ((LA4_0 >= '\u0000' && LA4_0 <= '&')||(LA4_0 >= '(' && LA4_0 <= '\uFFFF')) ) {
alt4=1;
}
switch (alt4) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '&')||(input.LA(1) >= '(' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop4;
}
}
match('\'');
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "STRING"
// $ANTLR start "WS"
public final void mWS() throws RecognitionException {
try {
int _type = WS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:41:4: ( ( ' ' ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:41:6: ( ' ' )
{
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:41:6: ( ' ' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:41:7: ' '
{
match(' ');
}
_channel=HIDDEN;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "WS"
@Override
public void mTokens() throws RecognitionException {
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:8: ( DISTINCT | SELECT | WHERE | ORDER | BY | ASC | DESC | EQUAL | GT | LT | NOTEQUAL | COMMA | OBRAC | CBRAC | AND | OR | LIMIT | NUMBER | ID | STRING | WS )
int alt6=21;
alt6 = dfa6.predict(input);
switch (alt6) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:10: DISTINCT
{
mDISTINCT();
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:19: SELECT
{
mSELECT();
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:26: WHERE
{
mWHERE();
}
break;
case 4 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:32: ORDER
{
mORDER();
}
break;
case 5 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:38: BY
{
mBY();
}
break;
case 6 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:41: ASC
{
mASC();
}
break;
case 7 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:45: DESC
{
mDESC();
}
break;
case 8 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:50: EQUAL
{
mEQUAL();
}
break;
case 9 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:56: GT
{
mGT();
}
break;
case 10 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:59: LT
{
mLT();
}
break;
case 11 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:62: NOTEQUAL
{
mNOTEQUAL();
}
break;
case 12 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:71: COMMA
{
mCOMMA();
}
break;
case 13 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:77: OBRAC
{
mOBRAC();
}
break;
case 14 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:83: CBRAC
{
mCBRAC();
}
break;
case 15 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:89: AND
{
mAND();
}
break;
case 16 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:93: OR
{
mOR();
}
break;
case 17 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:96: LIMIT
{
mLIMIT();
}
break;
case 18 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:102: NUMBER
{
mNUMBER();
}
break;
case 19 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:109: ID
{
mID();
}
break;
case 20 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:112: STRING
{
mSTRING();
}
break;
case 21 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/iqry/IndexQueryLexer.g:1:119: WS
{
mWS();
}
break;
}
}
protected DFA6 dfa6 = new DFA6(this);
static final String DFA6_eotS =
"\1\uffff\6\20\7\uffff\1\20\4\uffff\4\20\1\41\1\42\10\20\2\uffff\1\53\1"+
"\54\2\20\1\57\3\20\2\uffff\2\20\1\uffff\1\20\1\66\1\67\1\70\1\20\1\72"+
"\3\uffff\1\20\1\uffff\1\74\1\uffff";
static final String DFA6_eofS =
"\75\uffff";
static final String DFA6_minS =
"\1\40\2\105\1\110\1\122\1\131\1\116\7\uffff\1\111\4\uffff\2\123\1\114"+
"\1\105\2\60\1\103\1\104\1\115\1\124\1\103\1\105\1\122\1\105\2\uffff\2"+
"\60\2\111\1\60\1\103\1\105\1\122\2\uffff\1\124\1\116\1\uffff\1\124\3\60"+
"\1\103\1\60\3\uffff\1\124\1\uffff\1\60\1\uffff";
static final String DFA6_maxS =
"\1\172\1\111\1\105\1\110\1\122\1\131\1\123\7\uffff\1\111\4\uffff\2\123"+
"\1\114\1\105\2\172\1\103\1\104\1\115\1\124\1\103\1\105\1\122\1\105\2\uffff"+
"\2\172\2\111\1\172\1\103\1\105\1\122\2\uffff\1\124\1\116\1\uffff\1\124"+
"\3\172\1\103\1\172\3\uffff\1\124\1\uffff\1\172\1\uffff";
static final String DFA6_acceptS =
"\7\uffff\1\10\1\11\1\12\1\13\1\14\1\15\1\16\1\uffff\1\22\1\23\1\24\1\25"+
"\16\uffff\1\20\1\5\10\uffff\1\6\1\17\2\uffff\1\7\6\uffff\1\3\1\4\1\21"+
"\1\uffff\1\2\1\uffff\1\1";
static final String DFA6_specialS =
"\75\uffff}>";
static final String[] DFA6_transitionS = {
"\1\22\1\12\1\21\4\uffff\1\21\1\14\1\15\2\uffff\1\13\3\uffff\12\17\2\uffff"+
"\1\11\1\7\1\10\2\uffff\1\6\1\5\1\20\1\1\7\20\1\16\2\20\1\4\3\20\1\2\3"+
"\20\1\3\3\20\4\uffff\1\20\1\uffff\32\20",
"\1\24\3\uffff\1\23",
"\1\25",
"\1\26",
"\1\27",
"\1\30",
"\1\32\4\uffff\1\31",
"",
"",
"",
"",
"",
"",
"",
"\1\33",
"",
"",
"",
"",
"\1\34",
"\1\35",
"\1\36",
"\1\37",
"\12\20\7\uffff\3\20\1\40\26\20\4\uffff\1\20\1\uffff\32\20",
"\12\20\7\uffff\32\20\4\uffff\1\20\1\uffff\32\20",
"\1\43",
"\1\44",
"\1\45",
"\1\46",
"\1\47",
"\1\50",
"\1\51",
"\1\52",
"",
"",
"\12\20\7\uffff\32\20\4\uffff\1\20\1\uffff\32\20",
"\12\20\7\uffff\32\20\4\uffff\1\20\1\uffff\32\20",
"\1\55",
"\1\56",
"\12\20\7\uffff\32\20\4\uffff\1\20\1\uffff\32\20",
"\1\60",
"\1\61",
"\1\62",
"",
"",
"\1\63",
"\1\64",
"",
"\1\65",
"\12\20\7\uffff\32\20\4\uffff\1\20\1\uffff\32\20",
"\12\20\7\uffff\32\20\4\uffff\1\20\1\uffff\32\20",
"\12\20\7\uffff\32\20\4\uffff\1\20\1\uffff\32\20",
"\1\71",
"\12\20\7\uffff\32\20\4\uffff\1\20\1\uffff\32\20",
"",
"",
"",
"\1\73",
"",
"\12\20\7\uffff\32\20\4\uffff\1\20\1\uffff\32\20",
""
};
static final short[] DFA6_eot = DFA.unpackEncodedString(DFA6_eotS);
static final short[] DFA6_eof = DFA.unpackEncodedString(DFA6_eofS);
static final char[] DFA6_min = DFA.unpackEncodedStringToUnsignedChars(DFA6_minS);
static final char[] DFA6_max = DFA.unpackEncodedStringToUnsignedChars(DFA6_maxS);
static final short[] DFA6_accept = DFA.unpackEncodedString(DFA6_acceptS);
static final short[] DFA6_special = DFA.unpackEncodedString(DFA6_specialS);
static final short[][] DFA6_transition;
static {
int numStates = DFA6_transitionS.length;
DFA6_transition = new short[numStates][];
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy