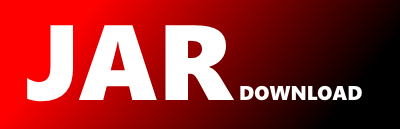
rapture.kernel.UserApiImplWrapper Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.kernel;
import org.apache.log4j.Logger;
import java.util.List;
import java.util.Map;
import rapture.common.api.UserApi;
import rapture.common.CallingContext;
import rapture.common.RaptureEntitlementsContext;
import rapture.common.hooks.CallName;
import rapture.common.model.BasePayload;
import rapture.common.EntitlementSet;
import rapture.kernel.context.ContextValidator;
import rapture.common.version.ApiVersion;
import rapture.common.model.RaptureUser;
import rapture.common.shared.user.GetWhoAmIPayload;
import rapture.common.shared.user.LogoutUserPayload;
import rapture.common.shared.user.StorePreferencePayload;
import rapture.common.shared.user.GetPreferencePayload;
import rapture.common.shared.user.RemovePreferencePayload;
import rapture.common.shared.user.GetPreferenceCategoriesPayload;
import rapture.common.shared.user.GetPreferencesInCategoryPayload;
import rapture.common.shared.user.UpdateMyDescriptionPayload;
import rapture.common.shared.user.ChangeMyPasswordPayload;
import rapture.common.shared.user.ChangeMyEmailPayload;
import rapture.common.shared.user.GetServerApiVersionPayload;
import rapture.common.shared.user.IsPermittedPayload;
/**
* This class is a wrapper around the class {@link UserApiImpl}. This is an auto-generated class that gives us the ability to add hooks such as entitlmeent
* checks before each function call in the implementation. Since we always want to call these hooks, an instance of this class should be used
* (in {@link Kernel}) instead of using the implementation directly.
* implementation directly.
*
*/
@SuppressWarnings("all")
public class UserApiImplWrapper implements UserApi, KernelApi {
private static final Logger log = Logger.getLogger(UserApiImplWrapper.class);
private UserApiImpl apiImpl;
public UserApiImplWrapper(Kernel kernel) {
apiImpl = new UserApiImpl(kernel);
}
/**
* Returns the underlying implementation object. This should be used when a call is deliberately bypassing entitlement checks.
* @return {@link User}
*/
public UserApiImpl getTrusted() {
return apiImpl;
}
@Override
public void start() {
apiImpl.start();
}
/**
*
*/
@Override
public RaptureUser getWhoAmI(CallingContext context) {
long functionStartTime = System.currentTimeMillis();
GetWhoAmIPayload requestObj = new GetWhoAmIPayload();
requestObj.setContext(context);
ContextValidator.validateContext(context, EntitlementSet.User_getWhoAmI, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.User_getWhoAmI);
RaptureUser returnValue = apiImpl.getWhoAmI(context); Kernel.getApiHooksService().post(context, CallName.User_getWhoAmI);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.getWhoAmI.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.getWhoAmI.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public void logoutUser(CallingContext context) {
long functionStartTime = System.currentTimeMillis();
LogoutUserPayload requestObj = new LogoutUserPayload();
requestObj.setContext(context);
ContextValidator.validateContext(context, EntitlementSet.User_logoutUser, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.User_logoutUser);
apiImpl.logoutUser(context); Kernel.getApiHooksService().post(context, CallName.User_logoutUser);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.logoutUser.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.logoutUser.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
}
/**
*
*/
@Override
public void storePreference(CallingContext context, String category, String name, String content) {
long functionStartTime = System.currentTimeMillis();
StorePreferencePayload requestObj = new StorePreferencePayload();
requestObj.setContext(context);
requestObj.setCategory(category);
requestObj.setName(name);
requestObj.setContent(content);
ContextValidator.validateContext(context, EntitlementSet.User_storePreference, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.User_storePreference);
apiImpl.storePreference(context, category, name, content); Kernel.getApiHooksService().post(context, CallName.User_storePreference);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.storePreference.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.storePreference.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
}
/**
*
*/
@Override
public String getPreference(CallingContext context, String category, String name) {
long functionStartTime = System.currentTimeMillis();
GetPreferencePayload requestObj = new GetPreferencePayload();
requestObj.setContext(context);
requestObj.setCategory(category);
requestObj.setName(name);
ContextValidator.validateContext(context, EntitlementSet.User_getPreference, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.User_getPreference);
String returnValue = apiImpl.getPreference(context, category, name); Kernel.getApiHooksService().post(context, CallName.User_getPreference);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.getPreference.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.getPreference.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public void removePreference(CallingContext context, String category, String name) {
long functionStartTime = System.currentTimeMillis();
RemovePreferencePayload requestObj = new RemovePreferencePayload();
requestObj.setContext(context);
requestObj.setCategory(category);
requestObj.setName(name);
ContextValidator.validateContext(context, EntitlementSet.User_removePreference, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.User_removePreference);
apiImpl.removePreference(context, category, name); Kernel.getApiHooksService().post(context, CallName.User_removePreference);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.removePreference.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.removePreference.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
}
/**
*
*/
@Override
public List getPreferenceCategories(CallingContext context) {
long functionStartTime = System.currentTimeMillis();
GetPreferenceCategoriesPayload requestObj = new GetPreferenceCategoriesPayload();
requestObj.setContext(context);
ContextValidator.validateContext(context, EntitlementSet.User_getPreferenceCategories, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.User_getPreferenceCategories);
List returnValue = apiImpl.getPreferenceCategories(context); Kernel.getApiHooksService().post(context, CallName.User_getPreferenceCategories);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.getPreferenceCategories.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.getPreferenceCategories.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public List getPreferencesInCategory(CallingContext context, String category) {
long functionStartTime = System.currentTimeMillis();
GetPreferencesInCategoryPayload requestObj = new GetPreferencesInCategoryPayload();
requestObj.setContext(context);
requestObj.setCategory(category);
ContextValidator.validateContext(context, EntitlementSet.User_getPreferencesInCategory, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.User_getPreferencesInCategory);
List returnValue = apiImpl.getPreferencesInCategory(context, category); Kernel.getApiHooksService().post(context, CallName.User_getPreferencesInCategory);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.getPreferencesInCategory.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.getPreferencesInCategory.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public RaptureUser updateMyDescription(CallingContext context, String description) {
long functionStartTime = System.currentTimeMillis();
UpdateMyDescriptionPayload requestObj = new UpdateMyDescriptionPayload();
requestObj.setContext(context);
requestObj.setDescription(description);
ContextValidator.validateContext(context, EntitlementSet.User_updateMyDescription, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.User_updateMyDescription);
RaptureUser returnValue = apiImpl.updateMyDescription(context, description); Kernel.getApiHooksService().post(context, CallName.User_updateMyDescription);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.updateMyDescription.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.updateMyDescription.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public RaptureUser changeMyPassword(CallingContext context, String oldHashPassword, String newHashPassword) {
long functionStartTime = System.currentTimeMillis();
ChangeMyPasswordPayload requestObj = new ChangeMyPasswordPayload();
requestObj.setContext(context);
requestObj.setOldHashPassword(oldHashPassword);
requestObj.setNewHashPassword(newHashPassword);
ContextValidator.validateContext(context, EntitlementSet.User_changeMyPassword, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.User_changeMyPassword);
RaptureUser returnValue = apiImpl.changeMyPassword(context, oldHashPassword, newHashPassword); Kernel.getApiHooksService().post(context, CallName.User_changeMyPassword);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.changeMyPassword.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.changeMyPassword.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public RaptureUser changeMyEmail(CallingContext context, String newAddress) {
long functionStartTime = System.currentTimeMillis();
ChangeMyEmailPayload requestObj = new ChangeMyEmailPayload();
requestObj.setContext(context);
requestObj.setNewAddress(newAddress);
ContextValidator.validateContext(context, EntitlementSet.User_changeMyEmail, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.User_changeMyEmail);
RaptureUser returnValue = apiImpl.changeMyEmail(context, newAddress); Kernel.getApiHooksService().post(context, CallName.User_changeMyEmail);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.changeMyEmail.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.changeMyEmail.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public ApiVersion getServerApiVersion(CallingContext context) {
long functionStartTime = System.currentTimeMillis();
GetServerApiVersionPayload requestObj = new GetServerApiVersionPayload();
requestObj.setContext(context);
ContextValidator.validateContext(context, EntitlementSet.User_getServerApiVersion, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.User_getServerApiVersion);
ApiVersion returnValue = apiImpl.getServerApiVersion(context); Kernel.getApiHooksService().post(context, CallName.User_getServerApiVersion);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.getServerApiVersion.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.getServerApiVersion.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public Boolean isPermitted(CallingContext context, String apiCall, String callParam) {
long functionStartTime = System.currentTimeMillis();
IsPermittedPayload requestObj = new IsPermittedPayload();
requestObj.setContext(context);
requestObj.setApiCall(apiCall);
requestObj.setCallParam(callParam);
ContextValidator.validateContext(context, EntitlementSet.User_isPermitted, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.User_isPermitted);
Boolean returnValue = apiImpl.isPermitted(context, apiCall, callParam); Kernel.getApiHooksService().post(context, CallName.User_isPermitted);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.isPermitted.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.userApi.isPermitted.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy