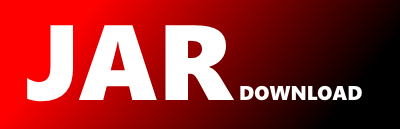
reflex.ReflexTreeWalker Maven / Gradle / Ivy
// $ANTLR 3.5.2 /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g 2016-03-01 15:52:42
package reflex;
import java.io.PrintStream;
import reflex.function.*;
import reflex.node.*;
import reflex.node.io.*;
import reflex.node.functional.*;
import reflex.importer.*;
import reflex.debug.*;
import reflex.util.function.LanguageRegistry;
import reflex.util.*;
import org.antlr.runtime.*;
import org.antlr.runtime.tree.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings("all")
public class ReflexTreeWalker extends TreeParser {
public static final String[] tokenNames = new String[] {
"", "", "", "", "ASSIGNMENT", "Add", "All", "And",
"Any", "Archive", "Assert", "Assign", "AsyncCall", "AsyncCallScript",
"AsyncOneShot", "AsyncStatus", "B64Compress", "B64Decompress", "BLOCK",
"BREAK", "Bool", "Break", "CBrace", "CBracket", "CONSTASSIGNMENT", "CONTINUE",
"CParen", "Call", "Capabilities", "Cast", "Catch", "Chain", "Close", "Collate",
"Colon", "Comma", "Comment", "Const", "Continue", "Copy", "DOTTEDASSIGNMENT",
"Date", "Debug", "Def", "Defined", "Delete", "Difference", "Digit", "Divide",
"Do", "DottedIdentifier", "DropWhile", "ESC", "EXP", "EXPORT", "EXP_LIST",
"Else", "End", "Equals", "Evals", "Excl", "Export", "FORLIST", "FORTO",
"FUNCTION", "FUNC_CALL", "File", "FilterFn", "Fold", "For", "Format",
"FromJson", "GT", "GTEquals", "GenSchema", "GenStruct", "GetCache", "GetCh",
"GetLine", "HasCapability", "ID_LIST", "IF", "IMPORT", "IMPORTAS", "IMPORTPARAMS",
"INCLUDE", "INDEXES", "Identifier", "If", "Import", "In", "Int", "Integer",
"IsFile", "IsFolder", "Join", "Json", "KERNEL_CALL", "KEYVAL", "KEYVAL_LIST",
"KernelIdentifier", "Keys", "LIST", "LOOKUP", "LT", "LTEquals", "Lib",
"Long", "MAPDEF", "MD5", "METABLOCK", "METAPULL", "MapFn", "Matches",
"Merge", "MergeIf", "Message", "MkDir", "Modulus", "Multiply", "NEGATE",
"NEquals", "New", "Null", "Number", "OBrace", "OBracket", "OParen", "Or",
"PATCH", "PFORLIST", "PFORTO", "PFor", "PLUSASSIGNMENT", "PORTF", "PORTR",
"PULL", "PUSH", "PackageIdentifier", "Patch", "Port", "PortA", "Pow",
"Print", "Println", "PropertyPlaceholder", "PullVal", "PushVal", "PutCache",
"QMark", "QUALIFIED_FUNC_CALL", "QuotedString", "RANGEINDEX", "RANGELOOKUP",
"REQUIRE", "RETURN", "RPull", "RPush", "Rand", "ReadDir", "Remove", "Replace",
"Return", "Round", "SColon", "SPARSE", "SPARSELOOKUP", "STATEMENTS", "Signal",
"Size", "Sleep", "Sort", "Space", "Spawn", "Split", "SplitWith", "String",
"Structure", "Subtract", "Suspend", "SuspendWait", "TERNARY", "TakeWhile",
"Template", "Throw", "Time", "Timer", "To", "Transpose", "Try", "TypeOf",
"UNARY_MIN", "Unique", "UrlDecode", "UrlEncode", "Use", "Uuid", "Vars",
"Wait", "While", "'+='", "'..'", "'<<--'", "'FIXEDLIST'", "'FREESTRING'",
"'SCRIPTLIST'", "'array'", "'as'", "'integer'", "'list'", "'meta'", "'number'",
"'of'", "'param'", "'property'", "'string'", "'with'"
};
public static final int EOF=-1;
public static final int T__200=200;
public static final int T__201=201;
public static final int T__202=202;
public static final int T__203=203;
public static final int T__204=204;
public static final int T__205=205;
public static final int T__206=206;
public static final int T__207=207;
public static final int T__208=208;
public static final int T__209=209;
public static final int T__210=210;
public static final int T__211=211;
public static final int T__212=212;
public static final int T__213=213;
public static final int T__214=214;
public static final int T__215=215;
public static final int T__216=216;
public static final int ASSIGNMENT=4;
public static final int Add=5;
public static final int All=6;
public static final int And=7;
public static final int Any=8;
public static final int Archive=9;
public static final int Assert=10;
public static final int Assign=11;
public static final int AsyncCall=12;
public static final int AsyncCallScript=13;
public static final int AsyncOneShot=14;
public static final int AsyncStatus=15;
public static final int B64Compress=16;
public static final int B64Decompress=17;
public static final int BLOCK=18;
public static final int BREAK=19;
public static final int Bool=20;
public static final int Break=21;
public static final int CBrace=22;
public static final int CBracket=23;
public static final int CONSTASSIGNMENT=24;
public static final int CONTINUE=25;
public static final int CParen=26;
public static final int Call=27;
public static final int Capabilities=28;
public static final int Cast=29;
public static final int Catch=30;
public static final int Chain=31;
public static final int Close=32;
public static final int Collate=33;
public static final int Colon=34;
public static final int Comma=35;
public static final int Comment=36;
public static final int Const=37;
public static final int Continue=38;
public static final int Copy=39;
public static final int DOTTEDASSIGNMENT=40;
public static final int Date=41;
public static final int Debug=42;
public static final int Def=43;
public static final int Defined=44;
public static final int Delete=45;
public static final int Difference=46;
public static final int Digit=47;
public static final int Divide=48;
public static final int Do=49;
public static final int DottedIdentifier=50;
public static final int DropWhile=51;
public static final int ESC=52;
public static final int EXP=53;
public static final int EXPORT=54;
public static final int EXP_LIST=55;
public static final int Else=56;
public static final int End=57;
public static final int Equals=58;
public static final int Evals=59;
public static final int Excl=60;
public static final int Export=61;
public static final int FORLIST=62;
public static final int FORTO=63;
public static final int FUNCTION=64;
public static final int FUNC_CALL=65;
public static final int File=66;
public static final int FilterFn=67;
public static final int Fold=68;
public static final int For=69;
public static final int Format=70;
public static final int FromJson=71;
public static final int GT=72;
public static final int GTEquals=73;
public static final int GenSchema=74;
public static final int GenStruct=75;
public static final int GetCache=76;
public static final int GetCh=77;
public static final int GetLine=78;
public static final int HasCapability=79;
public static final int ID_LIST=80;
public static final int IF=81;
public static final int IMPORT=82;
public static final int IMPORTAS=83;
public static final int IMPORTPARAMS=84;
public static final int INCLUDE=85;
public static final int INDEXES=86;
public static final int Identifier=87;
public static final int If=88;
public static final int Import=89;
public static final int In=90;
public static final int Int=91;
public static final int Integer=92;
public static final int IsFile=93;
public static final int IsFolder=94;
public static final int Join=95;
public static final int Json=96;
public static final int KERNEL_CALL=97;
public static final int KEYVAL=98;
public static final int KEYVAL_LIST=99;
public static final int KernelIdentifier=100;
public static final int Keys=101;
public static final int LIST=102;
public static final int LOOKUP=103;
public static final int LT=104;
public static final int LTEquals=105;
public static final int Lib=106;
public static final int Long=107;
public static final int MAPDEF=108;
public static final int MD5=109;
public static final int METABLOCK=110;
public static final int METAPULL=111;
public static final int MapFn=112;
public static final int Matches=113;
public static final int Merge=114;
public static final int MergeIf=115;
public static final int Message=116;
public static final int MkDir=117;
public static final int Modulus=118;
public static final int Multiply=119;
public static final int NEGATE=120;
public static final int NEquals=121;
public static final int New=122;
public static final int Null=123;
public static final int Number=124;
public static final int OBrace=125;
public static final int OBracket=126;
public static final int OParen=127;
public static final int Or=128;
public static final int PATCH=129;
public static final int PFORLIST=130;
public static final int PFORTO=131;
public static final int PFor=132;
public static final int PLUSASSIGNMENT=133;
public static final int PORTF=134;
public static final int PORTR=135;
public static final int PULL=136;
public static final int PUSH=137;
public static final int PackageIdentifier=138;
public static final int Patch=139;
public static final int Port=140;
public static final int PortA=141;
public static final int Pow=142;
public static final int Print=143;
public static final int Println=144;
public static final int PropertyPlaceholder=145;
public static final int PullVal=146;
public static final int PushVal=147;
public static final int PutCache=148;
public static final int QMark=149;
public static final int QUALIFIED_FUNC_CALL=150;
public static final int QuotedString=151;
public static final int RANGEINDEX=152;
public static final int RANGELOOKUP=153;
public static final int REQUIRE=154;
public static final int RETURN=155;
public static final int RPull=156;
public static final int RPush=157;
public static final int Rand=158;
public static final int ReadDir=159;
public static final int Remove=160;
public static final int Replace=161;
public static final int Return=162;
public static final int Round=163;
public static final int SColon=164;
public static final int SPARSE=165;
public static final int SPARSELOOKUP=166;
public static final int STATEMENTS=167;
public static final int Signal=168;
public static final int Size=169;
public static final int Sleep=170;
public static final int Sort=171;
public static final int Space=172;
public static final int Spawn=173;
public static final int Split=174;
public static final int SplitWith=175;
public static final int String=176;
public static final int Structure=177;
public static final int Subtract=178;
public static final int Suspend=179;
public static final int SuspendWait=180;
public static final int TERNARY=181;
public static final int TakeWhile=182;
public static final int Template=183;
public static final int Throw=184;
public static final int Time=185;
public static final int Timer=186;
public static final int To=187;
public static final int Transpose=188;
public static final int Try=189;
public static final int TypeOf=190;
public static final int UNARY_MIN=191;
public static final int Unique=192;
public static final int UrlDecode=193;
public static final int UrlEncode=194;
public static final int Use=195;
public static final int Uuid=196;
public static final int Vars=197;
public static final int Wait=198;
public static final int While=199;
// delegates
public TreeParser[] getDelegates() {
return new TreeParser[] {};
}
// delegators
public ReflexTreeWalker(TreeNodeStream input) {
this(input, new RecognizerSharedState());
}
public ReflexTreeWalker(TreeNodeStream input, RecognizerSharedState state) {
super(input, state);
}
@Override public String[] getTokenNames() { return ReflexTreeWalker.tokenNames; }
@Override public String getGrammarFileName() { return "/Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g"; }
public LanguageRegistry languageRegistry = null;
public Scope currentScope = null;
private IReflexHandler handler = new DummyReflexHandler();
public ImportHandler importHandler = new ImportHandler();
private NamespaceStack namespaceStack;
public ReflexTreeWalker(CommonTreeNodeStream nodes, LanguageRegistry languageRegistry) {
this(nodes, null, languageRegistry);
}
public ReflexTreeWalker(CommonTreeNodeStream nds, Scope sc, LanguageRegistry languageRegistry) {
this(nds, sc, languageRegistry, languageRegistry.getNamespaceStack());
}
public ReflexTreeWalker(CommonTreeNodeStream nds, Scope sc, LanguageRegistry languageRegistry, NamespaceStack namespaceStack) {
super(nds);
if (sc == null) {
currentScope = Scope.getInitialScope();
} else {
currentScope = sc;
}
this.languageRegistry = languageRegistry;
importHandler.setReflexHandler(handler);
this.namespaceStack = namespaceStack;
}
public void setReflexHandler(IReflexHandler handler) {
this.handler = handler;
importHandler.setReflexHandler(handler);
}
public IReflexHandler getReflexHandler() {
return handler;
}
public void setImportHandler(ImportHandler importHandler) {
this.importHandler = importHandler;
}
public ImportHandler getImportHandler() {
return importHandler;
}
@Override
public void reportError(RecognitionException e) {
super.reportError(e);
}
// $ANTLR start "walk"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:73:1: walk returns [ReflexNode node] : ( metaBlock )? block ;
public final ReflexNode walk() throws RecognitionException {
ReflexNode node = null;
ReflexNode block1 =null;
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:74:3: ( ( metaBlock )? block )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:74:6: ( metaBlock )? block
{
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:74:6: ( metaBlock )?
int alt1=2;
int LA1_0 = input.LA(1);
if ( (LA1_0==METABLOCK) ) {
alt1=1;
}
switch (alt1) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:74:6: metaBlock
{
pushFollow(FOLLOW_metaBlock_in_walk50);
metaBlock();
state._fsp--;
}
break;
}
pushFollow(FOLLOW_block_in_walk53);
block1=block();
state._fsp--;
node = block1;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "walk"
// $ANTLR start "metaBlock"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:78:1: metaBlock : METABLOCK ;
public final void metaBlock() throws RecognitionException {
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:79:3: ( METABLOCK )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:79:5: METABLOCK
{
match(input,METABLOCK,FOLLOW_METABLOCK_in_metaBlock69);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "metaBlock"
// $ANTLR start "block"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:82:1: block returns [ReflexNode node] : ^( BLOCK ^( STATEMENTS ( statement )* ) ^( RETURN ( expression )? ) ) ;
public final ReflexNode block() throws RecognitionException {
ReflexNode node = null;
ReflexNode statement2 =null;
ReflexNode expression3 =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
Scope scope = new Scope(currentScope);
currentScope = scope;
BlockNode bn = new BlockNode(line, handler, currentScope);
node = bn;
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:94:3: ( ^( BLOCK ^( STATEMENTS ( statement )* ) ^( RETURN ( expression )? ) ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:94:6: ^( BLOCK ^( STATEMENTS ( statement )* ) ^( RETURN ( expression )? ) )
{
match(input,BLOCK,FOLLOW_BLOCK_in_block98);
match(input, Token.DOWN, null);
match(input,STATEMENTS,FOLLOW_STATEMENTS_in_block109);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:95:22: ( statement )*
loop2:
while (true) {
int alt2=2;
int LA2_0 = input.LA(1);
if ( (LA2_0==ASSIGNMENT||LA2_0==BREAK||(LA2_0 >= CONSTASSIGNMENT && LA2_0 <= CONTINUE)||LA2_0==EXPORT||(LA2_0 >= FORLIST && LA2_0 <= FORTO)||LA2_0==FUNC_CALL||(LA2_0 >= IF && LA2_0 <= IMPORT)||LA2_0==KERNEL_CALL||LA2_0==METAPULL||(LA2_0 >= PATCH && LA2_0 <= PFORTO)||(LA2_0 >= PLUSASSIGNMENT && LA2_0 <= PUSH)||LA2_0==QUALIFIED_FUNC_CALL||LA2_0==Throw||LA2_0==Try||LA2_0==While) ) {
alt2=1;
}
switch (alt2) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:95:23: statement
{
pushFollow(FOLLOW_statement_in_block112);
statement2=statement();
state._fsp--;
bn.addStatement(statement2);
}
break;
default :
break loop2;
}
}
match(input, Token.UP, null);
}
match(input,RETURN,FOLLOW_RETURN_in_block129);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:96:22: ( expression )?
int alt3=2;
int LA3_0 = input.LA(1);
if ( (LA3_0==Add||LA3_0==And||LA3_0==Bool||LA3_0==Divide||LA3_0==Equals||(LA3_0 >= GT && LA3_0 <= GTEquals)||LA3_0==In||LA3_0==Integer||(LA3_0 >= LOOKUP && LA3_0 <= LTEquals)||LA3_0==Long||(LA3_0 >= Modulus && LA3_0 <= NEquals)||(LA3_0 >= Null && LA3_0 <= Number)||LA3_0==Or||LA3_0==Pow||LA3_0==RANGELOOKUP||LA3_0==SPARSE||LA3_0==Subtract||LA3_0==TERNARY||LA3_0==UNARY_MIN) ) {
alt3=1;
}
switch (alt3) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:96:23: expression
{
pushFollow(FOLLOW_expression_in_block136);
expression3=expression();
state._fsp--;
bn.addReturn(expression3);
}
break;
}
match(input, Token.UP, null);
}
match(input, Token.UP, null);
}
currentScope = currentScope.parent();
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "block"
// $ANTLR start "exportStatement"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:100:1: exportStatement returns [ReflexNode node] : ^( EXPORT i= Identifier b= block ) ;
public final ReflexNode exportStatement() throws RecognitionException {
ReflexNode node = null;
CommonTree i=null;
ReflexNode b =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:108:3: ( ^( EXPORT i= Identifier b= block ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:108:5: ^( EXPORT i= Identifier b= block )
{
match(input,EXPORT,FOLLOW_EXPORT_in_exportStatement177);
match(input, Token.DOWN, null);
i=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_exportStatement181);
namespaceStack.push((i!=null?i.getText():null));
pushFollow(FOLLOW_block_in_exportStatement187);
b=block();
state._fsp--;
match(input, Token.UP, null);
node = new ExportStatementNode(line, handler, currentScope, b );
}
namespaceStack.pop();
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "exportStatement"
// $ANTLR start "statement"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:112:1: statement returns [ReflexNode node] : ( assignment | pull | metapull | push | patchStatement | port | importStatement | exportStatement | breakStatement | continueStatement | functionCall | throwStatement | ifStatement | forStatement | pforStatement | whileStatement | guardedStatement );
public final ReflexNode statement() throws RecognitionException {
ReflexNode node = null;
ReflexNode assignment4 =null;
ReflexNode pull5 =null;
ReflexNode metapull6 =null;
ReflexNode push7 =null;
ReflexNode patchStatement8 =null;
ReflexNode port9 =null;
ReflexNode importStatement10 =null;
ReflexNode exportStatement11 =null;
ReflexNode breakStatement12 =null;
ReflexNode continueStatement13 =null;
ReflexNode functionCall14 =null;
ReflexNode throwStatement15 =null;
ReflexNode ifStatement16 =null;
ReflexNode forStatement17 =null;
ReflexNode pforStatement18 =null;
ReflexNode whileStatement19 =null;
ReflexNode guardedStatement20 =null;
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:113:3: ( assignment | pull | metapull | push | patchStatement | port | importStatement | exportStatement | breakStatement | continueStatement | functionCall | throwStatement | ifStatement | forStatement | pforStatement | whileStatement | guardedStatement )
int alt4=17;
switch ( input.LA(1) ) {
case ASSIGNMENT:
case CONSTASSIGNMENT:
case PLUSASSIGNMENT:
{
alt4=1;
}
break;
case PULL:
{
alt4=2;
}
break;
case METAPULL:
{
alt4=3;
}
break;
case PUSH:
{
alt4=4;
}
break;
case PATCH:
{
alt4=5;
}
break;
case PORTF:
case PORTR:
{
alt4=6;
}
break;
case IMPORT:
{
alt4=7;
}
break;
case EXPORT:
{
alt4=8;
}
break;
case BREAK:
{
alt4=9;
}
break;
case CONTINUE:
{
alt4=10;
}
break;
case FUNC_CALL:
case KERNEL_CALL:
case QUALIFIED_FUNC_CALL:
{
alt4=11;
}
break;
case Throw:
{
alt4=12;
}
break;
case IF:
{
alt4=13;
}
break;
case FORLIST:
case FORTO:
{
alt4=14;
}
break;
case PFORLIST:
case PFORTO:
{
alt4=15;
}
break;
case While:
{
alt4=16;
}
break;
case Try:
{
alt4=17;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 4, 0, input);
throw nvae;
}
switch (alt4) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:113:6: assignment
{
pushFollow(FOLLOW_assignment_in_statement209);
assignment4=assignment();
state._fsp--;
node = assignment4;
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:114:6: pull
{
pushFollow(FOLLOW_pull_in_statement218);
pull5=pull();
state._fsp--;
node = pull5;
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:115:6: metapull
{
pushFollow(FOLLOW_metapull_in_statement227);
metapull6=metapull();
state._fsp--;
node = metapull6;
}
break;
case 4 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:116:6: push
{
pushFollow(FOLLOW_push_in_statement236);
push7=push();
state._fsp--;
node = push7;
}
break;
case 5 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:117:6: patchStatement
{
pushFollow(FOLLOW_patchStatement_in_statement245);
patchStatement8=patchStatement();
state._fsp--;
node = patchStatement8;
}
break;
case 6 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:118:6: port
{
pushFollow(FOLLOW_port_in_statement254);
port9=port();
state._fsp--;
node = port9;
}
break;
case 7 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:119:6: importStatement
{
pushFollow(FOLLOW_importStatement_in_statement263);
importStatement10=importStatement();
state._fsp--;
node = importStatement10;
}
break;
case 8 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:120:6: exportStatement
{
pushFollow(FOLLOW_exportStatement_in_statement272);
exportStatement11=exportStatement();
state._fsp--;
node = exportStatement11;
}
break;
case 9 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:121:6: breakStatement
{
pushFollow(FOLLOW_breakStatement_in_statement281);
breakStatement12=breakStatement();
state._fsp--;
node = breakStatement12;
}
break;
case 10 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:122:6: continueStatement
{
pushFollow(FOLLOW_continueStatement_in_statement290);
continueStatement13=continueStatement();
state._fsp--;
node = continueStatement13;
}
break;
case 11 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:123:6: functionCall
{
pushFollow(FOLLOW_functionCall_in_statement299);
functionCall14=functionCall();
state._fsp--;
node = functionCall14;
}
break;
case 12 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:124:6: throwStatement
{
pushFollow(FOLLOW_throwStatement_in_statement308);
throwStatement15=throwStatement();
state._fsp--;
node = throwStatement15;
}
break;
case 13 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:125:6: ifStatement
{
pushFollow(FOLLOW_ifStatement_in_statement317);
ifStatement16=ifStatement();
state._fsp--;
node = ifStatement16;
}
break;
case 14 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:126:6: forStatement
{
pushFollow(FOLLOW_forStatement_in_statement326);
forStatement17=forStatement();
state._fsp--;
node = forStatement17;
}
break;
case 15 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:127:6: pforStatement
{
pushFollow(FOLLOW_pforStatement_in_statement335);
pforStatement18=pforStatement();
state._fsp--;
node = pforStatement18;
}
break;
case 16 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:128:6: whileStatement
{
pushFollow(FOLLOW_whileStatement_in_statement344);
whileStatement19=whileStatement();
state._fsp--;
node = whileStatement19;
}
break;
case 17 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:129:6: guardedStatement
{
pushFollow(FOLLOW_guardedStatement_in_statement353);
guardedStatement20=guardedStatement();
state._fsp--;
node = guardedStatement20;
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "statement"
// $ANTLR start "assignment"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:132:1: assignment returns [ReflexNode node] : ( ^( CONSTASSIGNMENT i= Identifier e= expression ) | ^( ASSIGNMENT i= ( Identifier | DottedIdentifier ) (x= indexes )? e= expression ) | ^( PLUSASSIGNMENT i= Identifier e= expression ) );
public final ReflexNode assignment() throws RecognitionException {
ReflexNode node = null;
CommonTree i=null;
ReflexNode e =null;
java.util.List> x =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:137:3: ( ^( CONSTASSIGNMENT i= Identifier e= expression ) | ^( ASSIGNMENT i= ( Identifier | DottedIdentifier ) (x= indexes )? e= expression ) | ^( PLUSASSIGNMENT i= Identifier e= expression ) )
int alt6=3;
switch ( input.LA(1) ) {
case CONSTASSIGNMENT:
{
alt6=1;
}
break;
case ASSIGNMENT:
{
alt6=2;
}
break;
case PLUSASSIGNMENT:
{
alt6=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 6, 0, input);
throw nvae;
}
switch (alt6) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:137:6: ^( CONSTASSIGNMENT i= Identifier e= expression )
{
match(input,CONSTASSIGNMENT,FOLLOW_CONSTASSIGNMENT_in_assignment379);
match(input, Token.DOWN, null);
i=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_assignment383);
pushFollow(FOLLOW_expression_in_assignment387);
e=expression();
state._fsp--;
match(input, Token.UP, null);
node = new ConstAssignmentNode(line, handler, currentScope, (i!=null?i.getText():null), e,
namespaceStack.asPrefix());
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:139:5: ^( ASSIGNMENT i= ( Identifier | DottedIdentifier ) (x= indexes )? e= expression )
{
match(input,ASSIGNMENT,FOLLOW_ASSIGNMENT_in_assignment397);
match(input, Token.DOWN, null);
i=(CommonTree)input.LT(1);
if ( input.LA(1)==DottedIdentifier||input.LA(1)==Identifier ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:139:53: (x= indexes )?
int alt5=2;
int LA5_0 = input.LA(1);
if ( (LA5_0==INDEXES) ) {
alt5=1;
}
switch (alt5) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:139:53: x= indexes
{
pushFollow(FOLLOW_indexes_in_assignment411);
x=indexes();
state._fsp--;
}
break;
}
pushFollow(FOLLOW_expression_in_assignment416);
e=expression();
state._fsp--;
match(input, Token.UP, null);
node = new AssignmentNode(line, handler, currentScope, (i!=null?i.getText():null), x, e);
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:141:5: ^( PLUSASSIGNMENT i= Identifier e= expression )
{
match(input,PLUSASSIGNMENT,FOLLOW_PLUSASSIGNMENT_in_assignment431);
match(input, Token.DOWN, null);
i=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_assignment435);
pushFollow(FOLLOW_expression_in_assignment439);
e=expression();
state._fsp--;
match(input, Token.UP, null);
node = new PlusAssignmentNode(line, handler, currentScope, (i!=null?i.getText():null), e);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "assignment"
// $ANTLR start "breakStatement"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:145:1: breakStatement returns [ReflexNode node] : BREAK ;
public final ReflexNode breakStatement() throws RecognitionException {
ReflexNode node = null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:150:3: ( BREAK )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:150:5: BREAK
{
match(input,BREAK,FOLLOW_BREAK_in_breakStatement469);
node = new BreakNode(line, handler, currentScope);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "breakStatement"
// $ANTLR start "continueStatement"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:153:1: continueStatement returns [ReflexNode node] : CONTINUE ;
public final ReflexNode continueStatement() throws RecognitionException {
ReflexNode node = null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:158:3: ( CONTINUE )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:158:5: CONTINUE
{
match(input,CONTINUE,FOLLOW_CONTINUE_in_continueStatement493);
node = new ContinueNode(line, handler, currentScope);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "continueStatement"
// $ANTLR start "importStatement"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:161:1: importStatement returns [ReflexNode node] : ^( IMPORT l= Identifier ^( IMPORTAS (alias= Identifier )? ) ^( IMPORTPARAMS (params= exprList )? ) ) ;
public final ReflexNode importStatement() throws RecognitionException {
ReflexNode node = null;
CommonTree l=null;
CommonTree alias=null;
java.util.List params =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:166:3: ( ^( IMPORT l= Identifier ^( IMPORTAS (alias= Identifier )? ) ^( IMPORTPARAMS (params= exprList )? ) ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:166:5: ^( IMPORT l= Identifier ^( IMPORTAS (alias= Identifier )? ) ^( IMPORTPARAMS (params= exprList )? ) )
{
match(input,IMPORT,FOLLOW_IMPORT_in_importStatement518);
match(input, Token.DOWN, null);
l=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_importStatement522);
match(input,IMPORTAS,FOLLOW_IMPORTAS_in_importStatement525);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:166:43: (alias= Identifier )?
int alt7=2;
int LA7_0 = input.LA(1);
if ( (LA7_0==Identifier) ) {
alt7=1;
}
switch (alt7) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:166:43: alias= Identifier
{
alias=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_importStatement529);
}
break;
}
match(input, Token.UP, null);
}
match(input,IMPORTPARAMS,FOLLOW_IMPORTPARAMS_in_importStatement534);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:166:78: (params= exprList )?
int alt8=2;
int LA8_0 = input.LA(1);
if ( (LA8_0==EXP_LIST) ) {
alt8=1;
}
switch (alt8) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:166:78: params= exprList
{
pushFollow(FOLLOW_exprList_in_importStatement538);
params=exprList();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
}
match(input, Token.UP, null);
node = new ImportNode(line, handler, currentScope, importHandler, (l!=null?l.getText():null), (alias!=null?alias.getText():null), params);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "importStatement"
// $ANTLR start "port"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:169:1: port returns [ReflexNode node] : ( ^( PORTF l= expression r= expression ) | ^( PORTR expression ) );
public final ReflexNode port() throws RecognitionException {
ReflexNode node = null;
ReflexNode l =null;
ReflexNode r =null;
ReflexNode expression21 =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:174:4: ( ^( PORTF l= expression r= expression ) | ^( PORTR expression ) )
int alt9=2;
int LA9_0 = input.LA(1);
if ( (LA9_0==PORTF) ) {
alt9=1;
}
else if ( (LA9_0==PORTR) ) {
alt9=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 9, 0, input);
throw nvae;
}
switch (alt9) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:174:6: ^( PORTF l= expression r= expression )
{
match(input,PORTF,FOLLOW_PORTF_in_port567);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_port571);
l=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_port575);
r=expression();
state._fsp--;
match(input, Token.UP, null);
node = new PortANode(line, handler, currentScope, l, r);
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:176:6: ^( PORTR expression )
{
match(input,PORTR,FOLLOW_PORTR_in_port592);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_port594);
expression21=expression();
state._fsp--;
match(input, Token.UP, null);
node = new PortANode(line, handler, currentScope, null, expression21);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "port"
// $ANTLR start "patchStatement"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:180:1: patchStatement returns [ReflexNode node] : ^( PATCH l= expression i= Identifier b= block ) ;
public final ReflexNode patchStatement() throws RecognitionException {
ReflexNode node = null;
CommonTree i=null;
ReflexNode l =null;
ReflexNode b =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:185:2: ( ^( PATCH l= expression i= Identifier b= block ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:185:4: ^( PATCH l= expression i= Identifier b= block )
{
match(input,PATCH,FOLLOW_PATCH_in_patchStatement626);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_patchStatement630);
l=expression();
state._fsp--;
i=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_patchStatement634);
pushFollow(FOLLOW_block_in_patchStatement638);
b=block();
state._fsp--;
match(input, Token.UP, null);
node = new PatchNode(line, handler, currentScope, l, (i!=null?i.getText():null), b);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "patchStatement"
// $ANTLR start "pull"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:189:1: pull returns [ReflexNode node] : ^( PULL i= Identifier e= expression ) ;
public final ReflexNode pull() throws RecognitionException {
ReflexNode node = null;
CommonTree i=null;
ReflexNode e =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:194:3: ( ^( PULL i= Identifier e= expression ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:194:5: ^( PULL i= Identifier e= expression )
{
match(input,PULL,FOLLOW_PULL_in_pull667);
match(input, Token.DOWN, null);
i=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_pull671);
pushFollow(FOLLOW_expression_in_pull675);
e=expression();
state._fsp--;
match(input, Token.UP, null);
node = new PullNode(line, handler, currentScope, (i!=null?i.getText():null), e);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "pull"
// $ANTLR start "metapull"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:198:1: metapull returns [ReflexNode node] : ^( METAPULL i= Identifier e= expression ) ;
public final ReflexNode metapull() throws RecognitionException {
ReflexNode node = null;
CommonTree i=null;
ReflexNode e =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:203:3: ( ^( METAPULL i= Identifier e= expression ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:203:5: ^( METAPULL i= Identifier e= expression )
{
match(input,METAPULL,FOLLOW_METAPULL_in_metapull706);
match(input, Token.DOWN, null);
i=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_metapull710);
pushFollow(FOLLOW_expression_in_metapull714);
e=expression();
state._fsp--;
match(input, Token.UP, null);
node = new MetaPullNode(line, handler, currentScope, (i!=null?i.getText():null), e);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "metapull"
// $ANTLR start "push"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:208:1: push returns [ReflexNode node] : ^( PUSH l= expression r= expression ) ;
public final ReflexNode push() throws RecognitionException {
ReflexNode node = null;
ReflexNode l =null;
ReflexNode r =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:213:3: ( ^( PUSH l= expression r= expression ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:213:5: ^( PUSH l= expression r= expression )
{
match(input,PUSH,FOLLOW_PUSH_in_push746);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_push750);
l=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_push754);
r=expression();
state._fsp--;
match(input, Token.UP, null);
node = new PushNode(line, handler, currentScope, l, r);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "push"
// $ANTLR start "throwStatement"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:217:1: throwStatement returns [ReflexNode node] : ^( Throw e= expression ) ;
public final ReflexNode throwStatement() throws RecognitionException {
ReflexNode node = null;
ReflexNode e =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:222:3: ( ^( Throw e= expression ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:222:5: ^( Throw e= expression )
{
match(input,Throw,FOLLOW_Throw_in_throwStatement785);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_throwStatement789);
e=expression();
state._fsp--;
match(input, Token.UP, null);
node = new ThrowNode(line, handler, currentScope, e);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "throwStatement"
// $ANTLR start "functionCall"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:226:1: functionCall returns [ReflexNode node] : ( ^( FUNC_CALL Identifier ( exprList )? ) | ^( FUNC_CALL PackageIdentifier ( exprList )? ) | ^( FUNC_CALL Println ( expression )? ) | ^( FUNC_CALL GetLine ( expression )? ) | ^( FUNC_CALL GetCh ( expression )? ) | ^( FUNC_CALL Capabilities ) | ^( FUNC_CALL HasCapability expression ) | ^( FUNC_CALL Print expression ) | ^( FUNC_CALL MapFn Identifier expression ) | ^( FUNC_CALL FilterFn Identifier expression ) | ^( FUNC_CALL Fold Identifier a= expression b= expression ) | ^( FUNC_CALL Any Identifier expression ) | ^( FUNC_CALL All Identifier expression ) | ^( FUNC_CALL TakeWhile Identifier expression ) | ^( FUNC_CALL DropWhile Identifier expression ) | ^( FUNC_CALL SplitWith Identifier expression ) | ^( FUNC_CALL Split str= expression sep= expression quoter= expression ) | ^( FUNC_CALL TypeOf expression ) | ^( FUNC_CALL Assert expression ) | ^( FUNC_CALL Size expression ) | ^( FUNC_CALL RPull u= expression ) | ^( FUNC_CALL RPush u= expression v= expression (o= expression )? ) | ^( FUNC_CALL Transpose expression ) | ^( FUNC_CALL Keys expression ) | ^( FUNC_CALL Sort arg= expression asc= expression ) | ^( FUNC_CALL Collate arg= expression locale= expression ) | ^( FUNC_CALL B64Compress expression ) | ^( FUNC_CALL B64Decompress expression ) | ^( FUNC_CALL Debug expression ) | ^( FUNC_CALL Date ( exprList )? ) | ^( FUNC_CALL Time ( expression )? ) | ^( FUNC_CALL Evals expression ) | ^( FUNC_CALL Vars ) | ^( FUNC_CALL ReadDir expression ) | ^( FUNC_CALL MkDir expression ) | ^( FUNC_CALL IsFile expression ) | ^( FUNC_CALL IsFolder expression ) | ^( FUNC_CALL File exprList ) | ^( FUNC_CALL Copy s= expression t= expression ) | ^( FUNC_CALL Archive expression ) | ^( FUNC_CALL Delete expression ) | ^( FUNC_CALL Port expression ) | ^( FUNC_CALL Suspend expression ) | ^( FUNC_CALL Close expression ) | ^( FUNC_CALL Timer ( expression )? ) | ^( FUNC_CALL Merge exprList ) | ^( FUNC_CALL Format exprList ) | ^( FUNC_CALL MergeIf exprList ) | ^( FUNC_CALL Replace v= expression s= expression t= expression ) | ^( FUNC_CALL Message a= expression m= expression ) | ^( FUNC_CALL PutCache v= expression n= expression (exp= expression )? ) | ^( FUNC_CALL GetCache n= expression ) | ^( FUNC_CALL Difference exprList ) | ^( FUNC_CALL Join exprList ) | ^( FUNC_CALL Unique exprList ) | ^( FUNC_CALL Json expression ) | ^( FUNC_CALL MD5 expression ) | ^( FUNC_CALL FromJson expression ) | ^( FUNC_CALL UrlEncode expression ) | ^( FUNC_CALL UrlDecode expression ) | ^( FUNC_CALL Uuid ) | ^( FUNC_CALL Remove Identifier k= expression ) | ^( FUNC_CALL AsyncCall s= expression (p= expression )? ) | ^( FUNC_CALL AsyncCallScript r= expression s= expression (p= expression )? ) | ^( FUNC_CALL AsyncStatus expression ) | ^( FUNC_CALL SuspendWait exprList ) | ^( FUNC_CALL Wait d= expression (in= expression retry= expression )? ) | ^( FUNC_CALL Signal d= expression v= expression ) | ^( FUNC_CALL Chain s= expression (p= expression )? ) | ^( FUNC_CALL Sleep expression ) | ^( FUNC_CALL Matches s= expression r= expression ) | ^( FUNC_CALL Cast a= expression b= expression ) | ^( FUNC_CALL Rand expression ) | ^( FUNC_CALL Round v= expression dp= expression ) | ^( FUNC_CALL Lib expression ) | ^( FUNC_CALL Call a= expression b= expression c= expression ) | ^( FUNC_CALL New a= expression ) | ^( FUNC_CALL GenSchema a= expression ) | ^( FUNC_CALL GenStruct Identifier a= expression ) | ^( FUNC_CALL Template t= expression p= expression ) | ^( FUNC_CALL Spawn p= expression (e= expression f= expression )? ) | ^( FUNC_CALL Defined Identifier ) | ^( KERNEL_CALL KernelIdentifier ( exprList )? ) | ^( QUALIFIED_FUNC_CALL DottedIdentifier ( exprList )? ) );
public final ReflexNode functionCall() throws RecognitionException {
ReflexNode node = null;
CommonTree Identifier22=null;
CommonTree PackageIdentifier24=null;
CommonTree Identifier31=null;
CommonTree Identifier33=null;
CommonTree Identifier35=null;
CommonTree Identifier36=null;
CommonTree Identifier38=null;
CommonTree Identifier40=null;
CommonTree Identifier42=null;
CommonTree Identifier44=null;
CommonTree Identifier79=null;
CommonTree Identifier85=null;
CommonTree Identifier86=null;
CommonTree KernelIdentifier87=null;
CommonTree DottedIdentifier89=null;
ReflexNode a =null;
ReflexNode b =null;
ReflexNode str =null;
ReflexNode sep =null;
ReflexNode quoter =null;
ReflexNode u =null;
ReflexNode v =null;
ReflexNode o =null;
ReflexNode arg =null;
ReflexNode asc =null;
ReflexNode locale =null;
ReflexNode s =null;
ReflexNode t =null;
ReflexNode m =null;
ReflexNode n =null;
ReflexNode exp =null;
ReflexNode k =null;
ReflexNode p =null;
ReflexNode r =null;
ReflexNode d =null;
ReflexNode in =null;
ReflexNode retry =null;
ReflexNode dp =null;
ReflexNode c =null;
ReflexNode e =null;
ReflexNode f =null;
java.util.List exprList23 =null;
java.util.List exprList25 =null;
ReflexNode expression26 =null;
ReflexNode expression27 =null;
ReflexNode expression28 =null;
ReflexNode expression29 =null;
ReflexNode expression30 =null;
ReflexNode expression32 =null;
ReflexNode expression34 =null;
ReflexNode expression37 =null;
ReflexNode expression39 =null;
ReflexNode expression41 =null;
ReflexNode expression43 =null;
ReflexNode expression45 =null;
ReflexNode expression46 =null;
ReflexNode expression47 =null;
ReflexNode expression48 =null;
ReflexNode expression49 =null;
ReflexNode expression50 =null;
ReflexNode expression51 =null;
ReflexNode expression52 =null;
ReflexNode expression53 =null;
java.util.List exprList54 =null;
ReflexNode expression55 =null;
ReflexNode expression56 =null;
ReflexNode expression57 =null;
ReflexNode expression58 =null;
ReflexNode expression59 =null;
ReflexNode expression60 =null;
java.util.List exprList61 =null;
ReflexNode expression62 =null;
ReflexNode expression63 =null;
ReflexNode expression64 =null;
ReflexNode expression65 =null;
ReflexNode expression66 =null;
ReflexNode expression67 =null;
java.util.List exprList68 =null;
java.util.List exprList69 =null;
java.util.List exprList70 =null;
java.util.List exprList71 =null;
java.util.List exprList72 =null;
java.util.List exprList73 =null;
ReflexNode expression74 =null;
ReflexNode expression75 =null;
ReflexNode expression76 =null;
ReflexNode expression77 =null;
ReflexNode expression78 =null;
ReflexNode expression80 =null;
java.util.List exprList81 =null;
ReflexNode expression82 =null;
ReflexNode expression83 =null;
ReflexNode expression84 =null;
java.util.List exprList88 =null;
java.util.List exprList90 =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:231:3: ( ^( FUNC_CALL Identifier ( exprList )? ) | ^( FUNC_CALL PackageIdentifier ( exprList )? ) | ^( FUNC_CALL Println ( expression )? ) | ^( FUNC_CALL GetLine ( expression )? ) | ^( FUNC_CALL GetCh ( expression )? ) | ^( FUNC_CALL Capabilities ) | ^( FUNC_CALL HasCapability expression ) | ^( FUNC_CALL Print expression ) | ^( FUNC_CALL MapFn Identifier expression ) | ^( FUNC_CALL FilterFn Identifier expression ) | ^( FUNC_CALL Fold Identifier a= expression b= expression ) | ^( FUNC_CALL Any Identifier expression ) | ^( FUNC_CALL All Identifier expression ) | ^( FUNC_CALL TakeWhile Identifier expression ) | ^( FUNC_CALL DropWhile Identifier expression ) | ^( FUNC_CALL SplitWith Identifier expression ) | ^( FUNC_CALL Split str= expression sep= expression quoter= expression ) | ^( FUNC_CALL TypeOf expression ) | ^( FUNC_CALL Assert expression ) | ^( FUNC_CALL Size expression ) | ^( FUNC_CALL RPull u= expression ) | ^( FUNC_CALL RPush u= expression v= expression (o= expression )? ) | ^( FUNC_CALL Transpose expression ) | ^( FUNC_CALL Keys expression ) | ^( FUNC_CALL Sort arg= expression asc= expression ) | ^( FUNC_CALL Collate arg= expression locale= expression ) | ^( FUNC_CALL B64Compress expression ) | ^( FUNC_CALL B64Decompress expression ) | ^( FUNC_CALL Debug expression ) | ^( FUNC_CALL Date ( exprList )? ) | ^( FUNC_CALL Time ( expression )? ) | ^( FUNC_CALL Evals expression ) | ^( FUNC_CALL Vars ) | ^( FUNC_CALL ReadDir expression ) | ^( FUNC_CALL MkDir expression ) | ^( FUNC_CALL IsFile expression ) | ^( FUNC_CALL IsFolder expression ) | ^( FUNC_CALL File exprList ) | ^( FUNC_CALL Copy s= expression t= expression ) | ^( FUNC_CALL Archive expression ) | ^( FUNC_CALL Delete expression ) | ^( FUNC_CALL Port expression ) | ^( FUNC_CALL Suspend expression ) | ^( FUNC_CALL Close expression ) | ^( FUNC_CALL Timer ( expression )? ) | ^( FUNC_CALL Merge exprList ) | ^( FUNC_CALL Format exprList ) | ^( FUNC_CALL MergeIf exprList ) | ^( FUNC_CALL Replace v= expression s= expression t= expression ) | ^( FUNC_CALL Message a= expression m= expression ) | ^( FUNC_CALL PutCache v= expression n= expression (exp= expression )? ) | ^( FUNC_CALL GetCache n= expression ) | ^( FUNC_CALL Difference exprList ) | ^( FUNC_CALL Join exprList ) | ^( FUNC_CALL Unique exprList ) | ^( FUNC_CALL Json expression ) | ^( FUNC_CALL MD5 expression ) | ^( FUNC_CALL FromJson expression ) | ^( FUNC_CALL UrlEncode expression ) | ^( FUNC_CALL UrlDecode expression ) | ^( FUNC_CALL Uuid ) | ^( FUNC_CALL Remove Identifier k= expression ) | ^( FUNC_CALL AsyncCall s= expression (p= expression )? ) | ^( FUNC_CALL AsyncCallScript r= expression s= expression (p= expression )? ) | ^( FUNC_CALL AsyncStatus expression ) | ^( FUNC_CALL SuspendWait exprList ) | ^( FUNC_CALL Wait d= expression (in= expression retry= expression )? ) | ^( FUNC_CALL Signal d= expression v= expression ) | ^( FUNC_CALL Chain s= expression (p= expression )? ) | ^( FUNC_CALL Sleep expression ) | ^( FUNC_CALL Matches s= expression r= expression ) | ^( FUNC_CALL Cast a= expression b= expression ) | ^( FUNC_CALL Rand expression ) | ^( FUNC_CALL Round v= expression dp= expression ) | ^( FUNC_CALL Lib expression ) | ^( FUNC_CALL Call a= expression b= expression c= expression ) | ^( FUNC_CALL New a= expression ) | ^( FUNC_CALL GenSchema a= expression ) | ^( FUNC_CALL GenStruct Identifier a= expression ) | ^( FUNC_CALL Template t= expression p= expression ) | ^( FUNC_CALL Spawn p= expression (e= expression f= expression )? ) | ^( FUNC_CALL Defined Identifier ) | ^( KERNEL_CALL KernelIdentifier ( exprList )? ) | ^( QUALIFIED_FUNC_CALL DottedIdentifier ( exprList )? ) )
int alt27=84;
alt27 = dfa27.predict(input);
switch (alt27) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:231:6: ^( FUNC_CALL Identifier ( exprList )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall821);
match(input, Token.DOWN, null);
Identifier22=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_functionCall823);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:231:29: ( exprList )?
int alt10=2;
int LA10_0 = input.LA(1);
if ( (LA10_0==EXP_LIST) ) {
alt10=1;
}
switch (alt10) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:231:29: exprList
{
pushFollow(FOLLOW_exprList_in_functionCall825);
exprList23=exprList();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new FunctionCallNode(line, handler, currentScope, (Identifier22!=null?Identifier22.getText():null), exprList23, languageRegistry, importHandler, namespaceStack.asPrefix());
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:232:6: ^( FUNC_CALL PackageIdentifier ( exprList )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall837);
match(input, Token.DOWN, null);
PackageIdentifier24=(CommonTree)match(input,PackageIdentifier,FOLLOW_PackageIdentifier_in_functionCall839);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:232:36: ( exprList )?
int alt11=2;
int LA11_0 = input.LA(1);
if ( (LA11_0==EXP_LIST) ) {
alt11=1;
}
switch (alt11) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:232:36: exprList
{
pushFollow(FOLLOW_exprList_in_functionCall841);
exprList25=exprList();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new PackageCallNode(line, handler, currentScope, importHandler, (PackageIdentifier24!=null?PackageIdentifier24.getText():null), exprList25);
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:233:6: ^( FUNC_CALL Println ( expression )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall853);
match(input, Token.DOWN, null);
match(input,Println,FOLLOW_Println_in_functionCall855);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:233:26: ( expression )?
int alt12=2;
int LA12_0 = input.LA(1);
if ( (LA12_0==Add||LA12_0==And||LA12_0==Bool||LA12_0==Divide||LA12_0==Equals||(LA12_0 >= GT && LA12_0 <= GTEquals)||LA12_0==In||LA12_0==Integer||(LA12_0 >= LOOKUP && LA12_0 <= LTEquals)||LA12_0==Long||(LA12_0 >= Modulus && LA12_0 <= NEquals)||(LA12_0 >= Null && LA12_0 <= Number)||LA12_0==Or||LA12_0==Pow||LA12_0==RANGELOOKUP||LA12_0==SPARSE||LA12_0==Subtract||LA12_0==TERNARY||LA12_0==UNARY_MIN) ) {
alt12=1;
}
switch (alt12) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:233:26: expression
{
pushFollow(FOLLOW_expression_in_functionCall857);
expression26=expression();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new PrintlnNode(line, handler, currentScope, expression26);
}
break;
case 4 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:234:6: ^( FUNC_CALL GetLine ( expression )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall869);
match(input, Token.DOWN, null);
match(input,GetLine,FOLLOW_GetLine_in_functionCall871);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:234:26: ( expression )?
int alt13=2;
int LA13_0 = input.LA(1);
if ( (LA13_0==Add||LA13_0==And||LA13_0==Bool||LA13_0==Divide||LA13_0==Equals||(LA13_0 >= GT && LA13_0 <= GTEquals)||LA13_0==In||LA13_0==Integer||(LA13_0 >= LOOKUP && LA13_0 <= LTEquals)||LA13_0==Long||(LA13_0 >= Modulus && LA13_0 <= NEquals)||(LA13_0 >= Null && LA13_0 <= Number)||LA13_0==Or||LA13_0==Pow||LA13_0==RANGELOOKUP||LA13_0==SPARSE||LA13_0==Subtract||LA13_0==TERNARY||LA13_0==UNARY_MIN) ) {
alt13=1;
}
switch (alt13) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:234:26: expression
{
pushFollow(FOLLOW_expression_in_functionCall873);
expression27=expression();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new GetLineNode(line, handler, currentScope, expression27);
}
break;
case 5 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:235:6: ^( FUNC_CALL GetCh ( expression )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall885);
match(input, Token.DOWN, null);
match(input,GetCh,FOLLOW_GetCh_in_functionCall887);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:235:24: ( expression )?
int alt14=2;
int LA14_0 = input.LA(1);
if ( (LA14_0==Add||LA14_0==And||LA14_0==Bool||LA14_0==Divide||LA14_0==Equals||(LA14_0 >= GT && LA14_0 <= GTEquals)||LA14_0==In||LA14_0==Integer||(LA14_0 >= LOOKUP && LA14_0 <= LTEquals)||LA14_0==Long||(LA14_0 >= Modulus && LA14_0 <= NEquals)||(LA14_0 >= Null && LA14_0 <= Number)||LA14_0==Or||LA14_0==Pow||LA14_0==RANGELOOKUP||LA14_0==SPARSE||LA14_0==Subtract||LA14_0==TERNARY||LA14_0==UNARY_MIN) ) {
alt14=1;
}
switch (alt14) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:235:24: expression
{
pushFollow(FOLLOW_expression_in_functionCall889);
expression28=expression();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new GetChNode(line, handler, currentScope, expression28);
}
break;
case 6 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:236:6: ^( FUNC_CALL Capabilities )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall901);
match(input, Token.DOWN, null);
match(input,Capabilities,FOLLOW_Capabilities_in_functionCall903);
match(input, Token.UP, null);
node = new CapabilitiesNode(line, handler, currentScope);
}
break;
case 7 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:237:6: ^( FUNC_CALL HasCapability expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall915);
match(input, Token.DOWN, null);
match(input,HasCapability,FOLLOW_HasCapability_in_functionCall917);
pushFollow(FOLLOW_expression_in_functionCall919);
expression29=expression();
state._fsp--;
match(input, Token.UP, null);
node = new HasCapabilityNode(line, handler, currentScope, expression29);
}
break;
case 8 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:238:6: ^( FUNC_CALL Print expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall931);
match(input, Token.DOWN, null);
match(input,Print,FOLLOW_Print_in_functionCall933);
pushFollow(FOLLOW_expression_in_functionCall935);
expression30=expression();
state._fsp--;
match(input, Token.UP, null);
node = new PrintNode(line, handler, currentScope, expression30);
}
break;
case 9 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:239:6: ^( FUNC_CALL MapFn Identifier expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall946);
match(input, Token.DOWN, null);
match(input,MapFn,FOLLOW_MapFn_in_functionCall948);
Identifier31=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_functionCall950);
pushFollow(FOLLOW_expression_in_functionCall952);
expression32=expression();
state._fsp--;
match(input, Token.UP, null);
node = new MapFnNode(line, handler, currentScope, (Identifier31!=null?Identifier31.getText():null), expression32, languageRegistry, importHandler);
}
break;
case 10 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:240:6: ^( FUNC_CALL FilterFn Identifier expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall963);
match(input, Token.DOWN, null);
match(input,FilterFn,FOLLOW_FilterFn_in_functionCall965);
Identifier33=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_functionCall967);
pushFollow(FOLLOW_expression_in_functionCall969);
expression34=expression();
state._fsp--;
match(input, Token.UP, null);
node = new FilterFnNode(line, handler, currentScope, (Identifier33!=null?Identifier33.getText():null), expression34, languageRegistry, importHandler);
}
break;
case 11 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:241:6: ^( FUNC_CALL Fold Identifier a= expression b= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall980);
match(input, Token.DOWN, null);
match(input,Fold,FOLLOW_Fold_in_functionCall982);
Identifier35=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_functionCall984);
pushFollow(FOLLOW_expression_in_functionCall988);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall992);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new FoldNode(line, handler, currentScope, (Identifier35!=null?Identifier35.getText():null), a, b, languageRegistry, importHandler);
}
break;
case 12 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:242:6: ^( FUNC_CALL Any Identifier expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1003);
match(input, Token.DOWN, null);
match(input,Any,FOLLOW_Any_in_functionCall1005);
Identifier36=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_functionCall1007);
pushFollow(FOLLOW_expression_in_functionCall1009);
expression37=expression();
state._fsp--;
match(input, Token.UP, null);
node = new AnyNode(line, handler, currentScope, (Identifier36!=null?Identifier36.getText():null), expression37, languageRegistry, importHandler);
}
break;
case 13 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:243:6: ^( FUNC_CALL All Identifier expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1020);
match(input, Token.DOWN, null);
match(input,All,FOLLOW_All_in_functionCall1022);
Identifier38=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_functionCall1024);
pushFollow(FOLLOW_expression_in_functionCall1026);
expression39=expression();
state._fsp--;
match(input, Token.UP, null);
node = new AllNode(line, handler, currentScope, (Identifier38!=null?Identifier38.getText():null), expression39, languageRegistry, importHandler);
}
break;
case 14 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:244:6: ^( FUNC_CALL TakeWhile Identifier expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1037);
match(input, Token.DOWN, null);
match(input,TakeWhile,FOLLOW_TakeWhile_in_functionCall1039);
Identifier40=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_functionCall1041);
pushFollow(FOLLOW_expression_in_functionCall1043);
expression41=expression();
state._fsp--;
match(input, Token.UP, null);
node = new TakeWhileNode(line, handler, currentScope, (Identifier40!=null?Identifier40.getText():null), expression41, languageRegistry, importHandler);
}
break;
case 15 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:245:6: ^( FUNC_CALL DropWhile Identifier expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1054);
match(input, Token.DOWN, null);
match(input,DropWhile,FOLLOW_DropWhile_in_functionCall1056);
Identifier42=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_functionCall1058);
pushFollow(FOLLOW_expression_in_functionCall1060);
expression43=expression();
state._fsp--;
match(input, Token.UP, null);
node = new DropWhileNode(line, handler, currentScope, (Identifier42!=null?Identifier42.getText():null), expression43, languageRegistry, importHandler);
}
break;
case 16 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:246:6: ^( FUNC_CALL SplitWith Identifier expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1071);
match(input, Token.DOWN, null);
match(input,SplitWith,FOLLOW_SplitWith_in_functionCall1073);
Identifier44=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_functionCall1075);
pushFollow(FOLLOW_expression_in_functionCall1077);
expression45=expression();
state._fsp--;
match(input, Token.UP, null);
node = new SplitWithNode(line, handler, currentScope, (Identifier44!=null?Identifier44.getText():null), expression45, languageRegistry, importHandler);
}
break;
case 17 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:247:6: ^( FUNC_CALL Split str= expression sep= expression quoter= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1088);
match(input, Token.DOWN, null);
match(input,Split,FOLLOW_Split_in_functionCall1090);
pushFollow(FOLLOW_expression_in_functionCall1094);
str=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall1098);
sep=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall1102);
quoter=expression();
state._fsp--;
match(input, Token.UP, null);
node = new SplitNode(line, handler, currentScope, str, sep, quoter, languageRegistry, importHandler);
}
break;
case 18 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:248:6: ^( FUNC_CALL TypeOf expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1113);
match(input, Token.DOWN, null);
match(input,TypeOf,FOLLOW_TypeOf_in_functionCall1115);
pushFollow(FOLLOW_expression_in_functionCall1117);
expression46=expression();
state._fsp--;
match(input, Token.UP, null);
node = new TypeOfNode(line, handler, currentScope, expression46);
}
break;
case 19 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:249:6: ^( FUNC_CALL Assert expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1128);
match(input, Token.DOWN, null);
match(input,Assert,FOLLOW_Assert_in_functionCall1130);
pushFollow(FOLLOW_expression_in_functionCall1132);
expression47=expression();
state._fsp--;
match(input, Token.UP, null);
node = new AssertNode(line, handler, currentScope, expression47);
}
break;
case 20 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:250:6: ^( FUNC_CALL Size expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1143);
match(input, Token.DOWN, null);
match(input,Size,FOLLOW_Size_in_functionCall1145);
pushFollow(FOLLOW_expression_in_functionCall1147);
expression48=expression();
state._fsp--;
match(input, Token.UP, null);
node = new SizeNode(line, handler,currentScope, expression48);
}
break;
case 21 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:251:6: ^( FUNC_CALL RPull u= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1158);
match(input, Token.DOWN, null);
match(input,RPull,FOLLOW_RPull_in_functionCall1160);
pushFollow(FOLLOW_expression_in_functionCall1164);
u=expression();
state._fsp--;
match(input, Token.UP, null);
node = new RapturePullNode(line, handler, currentScope, u, null);
}
break;
case 22 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:252:6: ^( FUNC_CALL RPush u= expression v= expression (o= expression )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1175);
match(input, Token.DOWN, null);
match(input,RPush,FOLLOW_RPush_in_functionCall1177);
pushFollow(FOLLOW_expression_in_functionCall1181);
u=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall1185);
v=expression();
state._fsp--;
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:252:51: (o= expression )?
int alt15=2;
int LA15_0 = input.LA(1);
if ( (LA15_0==Add||LA15_0==And||LA15_0==Bool||LA15_0==Divide||LA15_0==Equals||(LA15_0 >= GT && LA15_0 <= GTEquals)||LA15_0==In||LA15_0==Integer||(LA15_0 >= LOOKUP && LA15_0 <= LTEquals)||LA15_0==Long||(LA15_0 >= Modulus && LA15_0 <= NEquals)||(LA15_0 >= Null && LA15_0 <= Number)||LA15_0==Or||LA15_0==Pow||LA15_0==RANGELOOKUP||LA15_0==SPARSE||LA15_0==Subtract||LA15_0==TERNARY||LA15_0==UNARY_MIN) ) {
alt15=1;
}
switch (alt15) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:252:51: o= expression
{
pushFollow(FOLLOW_expression_in_functionCall1189);
o=expression();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new RapturePushNode(line, handler, currentScope, u, v, o);
}
break;
case 23 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:253:6: ^( FUNC_CALL Transpose expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1201);
match(input, Token.DOWN, null);
match(input,Transpose,FOLLOW_Transpose_in_functionCall1203);
pushFollow(FOLLOW_expression_in_functionCall1205);
expression49=expression();
state._fsp--;
match(input, Token.UP, null);
node = new TransposeNode(line, handler, currentScope, expression49);
}
break;
case 24 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:254:6: ^( FUNC_CALL Keys expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1216);
match(input, Token.DOWN, null);
match(input,Keys,FOLLOW_Keys_in_functionCall1218);
pushFollow(FOLLOW_expression_in_functionCall1220);
expression50=expression();
state._fsp--;
match(input, Token.UP, null);
node = new KeysNode(line, handler, currentScope, expression50);
}
break;
case 25 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:255:6: ^( FUNC_CALL Sort arg= expression asc= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1231);
match(input, Token.DOWN, null);
match(input,Sort,FOLLOW_Sort_in_functionCall1233);
pushFollow(FOLLOW_expression_in_functionCall1237);
arg=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall1241);
asc=expression();
state._fsp--;
match(input, Token.UP, null);
node = new SortNode(line, handler, currentScope, arg, asc);
}
break;
case 26 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:256:6: ^( FUNC_CALL Collate arg= expression locale= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1252);
match(input, Token.DOWN, null);
match(input,Collate,FOLLOW_Collate_in_functionCall1254);
pushFollow(FOLLOW_expression_in_functionCall1258);
arg=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall1262);
locale=expression();
state._fsp--;
match(input, Token.UP, null);
node = new CollateNode(line, handler, currentScope, arg, locale);
}
break;
case 27 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:257:6: ^( FUNC_CALL B64Compress expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1273);
match(input, Token.DOWN, null);
match(input,B64Compress,FOLLOW_B64Compress_in_functionCall1275);
pushFollow(FOLLOW_expression_in_functionCall1277);
expression51=expression();
state._fsp--;
match(input, Token.UP, null);
node = new B64Compress(line, handler, currentScope, expression51);
}
break;
case 28 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:258:6: ^( FUNC_CALL B64Decompress expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1288);
match(input, Token.DOWN, null);
match(input,B64Decompress,FOLLOW_B64Decompress_in_functionCall1290);
pushFollow(FOLLOW_expression_in_functionCall1292);
expression52=expression();
state._fsp--;
match(input, Token.UP, null);
node = new B64Decompress(line, handler, currentScope, expression52);
}
break;
case 29 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:259:6: ^( FUNC_CALL Debug expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1303);
match(input, Token.DOWN, null);
match(input,Debug,FOLLOW_Debug_in_functionCall1305);
pushFollow(FOLLOW_expression_in_functionCall1307);
expression53=expression();
state._fsp--;
match(input, Token.UP, null);
node = new DebugNode(line, handler, currentScope, expression53);
}
break;
case 30 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:260:6: ^( FUNC_CALL Date ( exprList )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1318);
match(input, Token.DOWN, null);
match(input,Date,FOLLOW_Date_in_functionCall1320);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:260:23: ( exprList )?
int alt16=2;
int LA16_0 = input.LA(1);
if ( (LA16_0==EXP_LIST) ) {
alt16=1;
}
switch (alt16) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:260:23: exprList
{
pushFollow(FOLLOW_exprList_in_functionCall1322);
exprList54=exprList();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new DateNode(line, handler, currentScope, exprList54);
}
break;
case 31 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:261:6: ^( FUNC_CALL Time ( expression )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1335);
match(input, Token.DOWN, null);
match(input,Time,FOLLOW_Time_in_functionCall1337);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:261:23: ( expression )?
int alt17=2;
int LA17_0 = input.LA(1);
if ( (LA17_0==Add||LA17_0==And||LA17_0==Bool||LA17_0==Divide||LA17_0==Equals||(LA17_0 >= GT && LA17_0 <= GTEquals)||LA17_0==In||LA17_0==Integer||(LA17_0 >= LOOKUP && LA17_0 <= LTEquals)||LA17_0==Long||(LA17_0 >= Modulus && LA17_0 <= NEquals)||(LA17_0 >= Null && LA17_0 <= Number)||LA17_0==Or||LA17_0==Pow||LA17_0==RANGELOOKUP||LA17_0==SPARSE||LA17_0==Subtract||LA17_0==TERNARY||LA17_0==UNARY_MIN) ) {
alt17=1;
}
switch (alt17) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:261:23: expression
{
pushFollow(FOLLOW_expression_in_functionCall1339);
expression55=expression();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new TimeNode(line, handler, currentScope, expression55);
}
break;
case 32 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:262:6: ^( FUNC_CALL Evals expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1352);
match(input, Token.DOWN, null);
match(input,Evals,FOLLOW_Evals_in_functionCall1354);
pushFollow(FOLLOW_expression_in_functionCall1356);
expression56=expression();
state._fsp--;
match(input, Token.UP, null);
node = new QuotedStringNode(line, handler, currentScope, expression56);
}
break;
case 33 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:263:6: ^( FUNC_CALL Vars )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1368);
match(input, Token.DOWN, null);
match(input,Vars,FOLLOW_Vars_in_functionCall1370);
match(input, Token.UP, null);
node = new VarsNode(line, handler, currentScope);
}
break;
case 34 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:264:6: ^( FUNC_CALL ReadDir expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1381);
match(input, Token.DOWN, null);
match(input,ReadDir,FOLLOW_ReadDir_in_functionCall1383);
pushFollow(FOLLOW_expression_in_functionCall1385);
expression57=expression();
state._fsp--;
match(input, Token.UP, null);
node = new ReadDirNode(line, handler, currentScope, expression57);
}
break;
case 35 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:265:6: ^( FUNC_CALL MkDir expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1396);
match(input, Token.DOWN, null);
match(input,MkDir,FOLLOW_MkDir_in_functionCall1398);
pushFollow(FOLLOW_expression_in_functionCall1400);
expression58=expression();
state._fsp--;
match(input, Token.UP, null);
node = new MkDirNode(line, handler, currentScope, expression58);
}
break;
case 36 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:266:6: ^( FUNC_CALL IsFile expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1411);
match(input, Token.DOWN, null);
match(input,IsFile,FOLLOW_IsFile_in_functionCall1413);
pushFollow(FOLLOW_expression_in_functionCall1415);
expression59=expression();
state._fsp--;
match(input, Token.UP, null);
node = new IsFileNode(line, handler, currentScope, expression59);
}
break;
case 37 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:267:6: ^( FUNC_CALL IsFolder expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1426);
match(input, Token.DOWN, null);
match(input,IsFolder,FOLLOW_IsFolder_in_functionCall1428);
pushFollow(FOLLOW_expression_in_functionCall1430);
expression60=expression();
state._fsp--;
match(input, Token.UP, null);
node = new IsFolderNode(line, handler, currentScope, expression60);
}
break;
case 38 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:268:6: ^( FUNC_CALL File exprList )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1441);
match(input, Token.DOWN, null);
match(input,File,FOLLOW_File_in_functionCall1443);
pushFollow(FOLLOW_exprList_in_functionCall1445);
exprList61=exprList();
state._fsp--;
match(input, Token.UP, null);
node = new FileNode(line, handler, currentScope, exprList61);
}
break;
case 39 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:269:6: ^( FUNC_CALL Copy s= expression t= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1456);
match(input, Token.DOWN, null);
match(input,Copy,FOLLOW_Copy_in_functionCall1458);
pushFollow(FOLLOW_expression_in_functionCall1462);
s=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall1466);
t=expression();
state._fsp--;
match(input, Token.UP, null);
node = new CopyNode(line, handler, currentScope, s, t);
}
break;
case 40 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:270:6: ^( FUNC_CALL Archive expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1477);
match(input, Token.DOWN, null);
match(input,Archive,FOLLOW_Archive_in_functionCall1479);
pushFollow(FOLLOW_expression_in_functionCall1481);
expression62=expression();
state._fsp--;
match(input, Token.UP, null);
node = new ArchiveNode(line, handler, currentScope, expression62);
}
break;
case 41 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:271:6: ^( FUNC_CALL Delete expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1492);
match(input, Token.DOWN, null);
match(input,Delete,FOLLOW_Delete_in_functionCall1494);
pushFollow(FOLLOW_expression_in_functionCall1496);
expression63=expression();
state._fsp--;
match(input, Token.UP, null);
node = new DeleteNode(line, handler, currentScope, expression63);
}
break;
case 42 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:272:6: ^( FUNC_CALL Port expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1507);
match(input, Token.DOWN, null);
match(input,Port,FOLLOW_Port_in_functionCall1509);
pushFollow(FOLLOW_expression_in_functionCall1511);
expression64=expression();
state._fsp--;
match(input, Token.UP, null);
node = new PortNode(line, handler, currentScope, expression64);
}
break;
case 43 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:273:6: ^( FUNC_CALL Suspend expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1522);
match(input, Token.DOWN, null);
match(input,Suspend,FOLLOW_Suspend_in_functionCall1524);
pushFollow(FOLLOW_expression_in_functionCall1526);
expression65=expression();
state._fsp--;
match(input, Token.UP, null);
node = new SuspendNode(line, handler, currentScope, expression65);
}
break;
case 44 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:274:6: ^( FUNC_CALL Close expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1537);
match(input, Token.DOWN, null);
match(input,Close,FOLLOW_Close_in_functionCall1539);
pushFollow(FOLLOW_expression_in_functionCall1541);
expression66=expression();
state._fsp--;
match(input, Token.UP, null);
node = new CloseNode(line, handler, currentScope, expression66);
}
break;
case 45 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:275:6: ^( FUNC_CALL Timer ( expression )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1552);
match(input, Token.DOWN, null);
match(input,Timer,FOLLOW_Timer_in_functionCall1554);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:275:24: ( expression )?
int alt18=2;
int LA18_0 = input.LA(1);
if ( (LA18_0==Add||LA18_0==And||LA18_0==Bool||LA18_0==Divide||LA18_0==Equals||(LA18_0 >= GT && LA18_0 <= GTEquals)||LA18_0==In||LA18_0==Integer||(LA18_0 >= LOOKUP && LA18_0 <= LTEquals)||LA18_0==Long||(LA18_0 >= Modulus && LA18_0 <= NEquals)||(LA18_0 >= Null && LA18_0 <= Number)||LA18_0==Or||LA18_0==Pow||LA18_0==RANGELOOKUP||LA18_0==SPARSE||LA18_0==Subtract||LA18_0==TERNARY||LA18_0==UNARY_MIN) ) {
alt18=1;
}
switch (alt18) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:275:24: expression
{
pushFollow(FOLLOW_expression_in_functionCall1556);
expression67=expression();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new TimerNode(line, handler, currentScope, expression67);
}
break;
case 46 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:276:6: ^( FUNC_CALL Merge exprList )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1568);
match(input, Token.DOWN, null);
match(input,Merge,FOLLOW_Merge_in_functionCall1570);
pushFollow(FOLLOW_exprList_in_functionCall1572);
exprList68=exprList();
state._fsp--;
match(input, Token.UP, null);
node = new MergeNode(line, handler, currentScope, exprList68);
}
break;
case 47 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:277:6: ^( FUNC_CALL Format exprList )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1583);
match(input, Token.DOWN, null);
match(input,Format,FOLLOW_Format_in_functionCall1585);
pushFollow(FOLLOW_exprList_in_functionCall1587);
exprList69=exprList();
state._fsp--;
match(input, Token.UP, null);
node = new FormatNode(line, handler, currentScope, exprList69);
}
break;
case 48 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:278:6: ^( FUNC_CALL MergeIf exprList )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1598);
match(input, Token.DOWN, null);
match(input,MergeIf,FOLLOW_MergeIf_in_functionCall1600);
pushFollow(FOLLOW_exprList_in_functionCall1602);
exprList70=exprList();
state._fsp--;
match(input, Token.UP, null);
node = new MergeIfNode(line, handler, currentScope, exprList70);
}
break;
case 49 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:279:6: ^( FUNC_CALL Replace v= expression s= expression t= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1613);
match(input, Token.DOWN, null);
match(input,Replace,FOLLOW_Replace_in_functionCall1615);
pushFollow(FOLLOW_expression_in_functionCall1619);
v=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall1623);
s=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall1627);
t=expression();
state._fsp--;
match(input, Token.UP, null);
node = new ReplaceNode(line, handler, currentScope, v, s, t);
}
break;
case 50 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:280:6: ^( FUNC_CALL Message a= expression m= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1638);
match(input, Token.DOWN, null);
match(input,Message,FOLLOW_Message_in_functionCall1640);
pushFollow(FOLLOW_expression_in_functionCall1644);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall1648);
m=expression();
state._fsp--;
match(input, Token.UP, null);
node = new MessageNode(line, handler, currentScope, a, m);
}
break;
case 51 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:281:6: ^( FUNC_CALL PutCache v= expression n= expression (exp= expression )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1659);
match(input, Token.DOWN, null);
match(input,PutCache,FOLLOW_PutCache_in_functionCall1661);
pushFollow(FOLLOW_expression_in_functionCall1665);
v=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall1669);
n=expression();
state._fsp--;
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:281:56: (exp= expression )?
int alt19=2;
int LA19_0 = input.LA(1);
if ( (LA19_0==Add||LA19_0==And||LA19_0==Bool||LA19_0==Divide||LA19_0==Equals||(LA19_0 >= GT && LA19_0 <= GTEquals)||LA19_0==In||LA19_0==Integer||(LA19_0 >= LOOKUP && LA19_0 <= LTEquals)||LA19_0==Long||(LA19_0 >= Modulus && LA19_0 <= NEquals)||(LA19_0 >= Null && LA19_0 <= Number)||LA19_0==Or||LA19_0==Pow||LA19_0==RANGELOOKUP||LA19_0==SPARSE||LA19_0==Subtract||LA19_0==TERNARY||LA19_0==UNARY_MIN) ) {
alt19=1;
}
switch (alt19) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:281:56: exp= expression
{
pushFollow(FOLLOW_expression_in_functionCall1673);
exp=expression();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new PutCacheNode(line, handler, currentScope, v, n, exp);
}
break;
case 52 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:282:6: ^( FUNC_CALL GetCache n= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1685);
match(input, Token.DOWN, null);
match(input,GetCache,FOLLOW_GetCache_in_functionCall1687);
pushFollow(FOLLOW_expression_in_functionCall1691);
n=expression();
state._fsp--;
match(input, Token.UP, null);
node = new GetCacheNode(line, handler, currentScope, n);
}
break;
case 53 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:283:6: ^( FUNC_CALL Difference exprList )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1702);
match(input, Token.DOWN, null);
match(input,Difference,FOLLOW_Difference_in_functionCall1704);
pushFollow(FOLLOW_exprList_in_functionCall1706);
exprList71=exprList();
state._fsp--;
match(input, Token.UP, null);
node = new DifferenceNode(line, handler, currentScope, exprList71);
}
break;
case 54 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:284:6: ^( FUNC_CALL Join exprList )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1717);
match(input, Token.DOWN, null);
match(input,Join,FOLLOW_Join_in_functionCall1719);
pushFollow(FOLLOW_exprList_in_functionCall1721);
exprList72=exprList();
state._fsp--;
match(input, Token.UP, null);
node = new JoinNode(line, handler, currentScope, exprList72);
}
break;
case 55 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:285:6: ^( FUNC_CALL Unique exprList )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1732);
match(input, Token.DOWN, null);
match(input,Unique,FOLLOW_Unique_in_functionCall1734);
pushFollow(FOLLOW_exprList_in_functionCall1736);
exprList73=exprList();
state._fsp--;
match(input, Token.UP, null);
node = new UniqueNode(line, handler, currentScope, exprList73);
}
break;
case 56 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:286:6: ^( FUNC_CALL Json expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1747);
match(input, Token.DOWN, null);
match(input,Json,FOLLOW_Json_in_functionCall1749);
pushFollow(FOLLOW_expression_in_functionCall1751);
expression74=expression();
state._fsp--;
match(input, Token.UP, null);
node = new JsonNode(line, handler, currentScope, expression74);
}
break;
case 57 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:288:6: ^( FUNC_CALL MD5 expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1763);
match(input, Token.DOWN, null);
match(input,MD5,FOLLOW_MD5_in_functionCall1765);
pushFollow(FOLLOW_expression_in_functionCall1768);
expression75=expression();
state._fsp--;
match(input, Token.UP, null);
node = new MD5Node(line, handler, currentScope, expression75);
}
break;
case 58 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:289:6: ^( FUNC_CALL FromJson expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1779);
match(input, Token.DOWN, null);
match(input,FromJson,FOLLOW_FromJson_in_functionCall1781);
pushFollow(FOLLOW_expression_in_functionCall1783);
expression76=expression();
state._fsp--;
match(input, Token.UP, null);
node = new FromJsonNode(line, handler, currentScope, expression76);
}
break;
case 59 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:290:6: ^( FUNC_CALL UrlEncode expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1794);
match(input, Token.DOWN, null);
match(input,UrlEncode,FOLLOW_UrlEncode_in_functionCall1796);
pushFollow(FOLLOW_expression_in_functionCall1798);
expression77=expression();
state._fsp--;
match(input, Token.UP, null);
node = new UrlEncodeNode(line, handler, currentScope, expression77);
}
break;
case 60 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:291:6: ^( FUNC_CALL UrlDecode expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1809);
match(input, Token.DOWN, null);
match(input,UrlDecode,FOLLOW_UrlDecode_in_functionCall1811);
pushFollow(FOLLOW_expression_in_functionCall1813);
expression78=expression();
state._fsp--;
match(input, Token.UP, null);
node = new UrlDecodeNode(line, handler, currentScope, expression78);
}
break;
case 61 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:292:6: ^( FUNC_CALL Uuid )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1824);
match(input, Token.DOWN, null);
match(input,Uuid,FOLLOW_Uuid_in_functionCall1826);
match(input, Token.UP, null);
node = new UuidNode(line, handler, currentScope);
}
break;
case 62 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:293:6: ^( FUNC_CALL Remove Identifier k= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1837);
match(input, Token.DOWN, null);
match(input,Remove,FOLLOW_Remove_in_functionCall1839);
Identifier79=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_functionCall1841);
pushFollow(FOLLOW_expression_in_functionCall1845);
k=expression();
state._fsp--;
match(input, Token.UP, null);
node = new RemoveNode(line, handler, currentScope, (Identifier79!=null?Identifier79.getText():null), k);
}
break;
case 63 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:294:6: ^( FUNC_CALL AsyncCall s= expression (p= expression )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1856);
match(input, Token.DOWN, null);
match(input,AsyncCall,FOLLOW_AsyncCall_in_functionCall1858);
pushFollow(FOLLOW_expression_in_functionCall1862);
s=expression();
state._fsp--;
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:294:42: (p= expression )?
int alt20=2;
int LA20_0 = input.LA(1);
if ( (LA20_0==Add||LA20_0==And||LA20_0==Bool||LA20_0==Divide||LA20_0==Equals||(LA20_0 >= GT && LA20_0 <= GTEquals)||LA20_0==In||LA20_0==Integer||(LA20_0 >= LOOKUP && LA20_0 <= LTEquals)||LA20_0==Long||(LA20_0 >= Modulus && LA20_0 <= NEquals)||(LA20_0 >= Null && LA20_0 <= Number)||LA20_0==Or||LA20_0==Pow||LA20_0==RANGELOOKUP||LA20_0==SPARSE||LA20_0==Subtract||LA20_0==TERNARY||LA20_0==UNARY_MIN) ) {
alt20=1;
}
switch (alt20) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:294:42: p= expression
{
pushFollow(FOLLOW_expression_in_functionCall1866);
p=expression();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new AsyncCallNode(line, handler, currentScope, s, p);
}
break;
case 64 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:295:6: ^( FUNC_CALL AsyncCallScript r= expression s= expression (p= expression )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1878);
match(input, Token.DOWN, null);
match(input,AsyncCallScript,FOLLOW_AsyncCallScript_in_functionCall1880);
pushFollow(FOLLOW_expression_in_functionCall1884);
r=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall1888);
s=expression();
state._fsp--;
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:295:61: (p= expression )?
int alt21=2;
int LA21_0 = input.LA(1);
if ( (LA21_0==Add||LA21_0==And||LA21_0==Bool||LA21_0==Divide||LA21_0==Equals||(LA21_0 >= GT && LA21_0 <= GTEquals)||LA21_0==In||LA21_0==Integer||(LA21_0 >= LOOKUP && LA21_0 <= LTEquals)||LA21_0==Long||(LA21_0 >= Modulus && LA21_0 <= NEquals)||(LA21_0 >= Null && LA21_0 <= Number)||LA21_0==Or||LA21_0==Pow||LA21_0==RANGELOOKUP||LA21_0==SPARSE||LA21_0==Subtract||LA21_0==TERNARY||LA21_0==UNARY_MIN) ) {
alt21=1;
}
switch (alt21) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:295:61: p= expression
{
pushFollow(FOLLOW_expression_in_functionCall1892);
p=expression();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new AsyncCallScriptNode(line, handler, currentScope, r, s, p);
}
break;
case 65 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:296:6: ^( FUNC_CALL AsyncStatus expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1904);
match(input, Token.DOWN, null);
match(input,AsyncStatus,FOLLOW_AsyncStatus_in_functionCall1906);
pushFollow(FOLLOW_expression_in_functionCall1908);
expression80=expression();
state._fsp--;
match(input, Token.UP, null);
node = new AsyncStatusNode(line, handler, currentScope, expression80);
}
break;
case 66 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:297:6: ^( FUNC_CALL SuspendWait exprList )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1919);
match(input, Token.DOWN, null);
match(input,SuspendWait,FOLLOW_SuspendWait_in_functionCall1921);
pushFollow(FOLLOW_exprList_in_functionCall1923);
exprList81=exprList();
state._fsp--;
match(input, Token.UP, null);
node = new SuspendWaitNode(line, handler, currentScope, exprList81);
}
break;
case 67 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:298:6: ^( FUNC_CALL Wait d= expression (in= expression retry= expression )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1934);
match(input, Token.DOWN, null);
match(input,Wait,FOLLOW_Wait_in_functionCall1936);
pushFollow(FOLLOW_expression_in_functionCall1940);
d=expression();
state._fsp--;
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:298:36: (in= expression retry= expression )?
int alt22=2;
int LA22_0 = input.LA(1);
if ( (LA22_0==Add||LA22_0==And||LA22_0==Bool||LA22_0==Divide||LA22_0==Equals||(LA22_0 >= GT && LA22_0 <= GTEquals)||LA22_0==In||LA22_0==Integer||(LA22_0 >= LOOKUP && LA22_0 <= LTEquals)||LA22_0==Long||(LA22_0 >= Modulus && LA22_0 <= NEquals)||(LA22_0 >= Null && LA22_0 <= Number)||LA22_0==Or||LA22_0==Pow||LA22_0==RANGELOOKUP||LA22_0==SPARSE||LA22_0==Subtract||LA22_0==TERNARY||LA22_0==UNARY_MIN) ) {
alt22=1;
}
switch (alt22) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:298:37: in= expression retry= expression
{
pushFollow(FOLLOW_expression_in_functionCall1945);
in=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall1949);
retry=expression();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new WaitNode(line, handler, currentScope, d, in, retry);
}
break;
case 68 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:299:6: ^( FUNC_CALL Signal d= expression v= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1962);
match(input, Token.DOWN, null);
match(input,Signal,FOLLOW_Signal_in_functionCall1964);
pushFollow(FOLLOW_expression_in_functionCall1968);
d=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall1972);
v=expression();
state._fsp--;
match(input, Token.UP, null);
node = new SignalNode(line, handler, currentScope, d, v);
}
break;
case 69 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:300:6: ^( FUNC_CALL Chain s= expression (p= expression )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall1983);
match(input, Token.DOWN, null);
match(input,Chain,FOLLOW_Chain_in_functionCall1985);
pushFollow(FOLLOW_expression_in_functionCall1989);
s=expression();
state._fsp--;
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:300:38: (p= expression )?
int alt23=2;
int LA23_0 = input.LA(1);
if ( (LA23_0==Add||LA23_0==And||LA23_0==Bool||LA23_0==Divide||LA23_0==Equals||(LA23_0 >= GT && LA23_0 <= GTEquals)||LA23_0==In||LA23_0==Integer||(LA23_0 >= LOOKUP && LA23_0 <= LTEquals)||LA23_0==Long||(LA23_0 >= Modulus && LA23_0 <= NEquals)||(LA23_0 >= Null && LA23_0 <= Number)||LA23_0==Or||LA23_0==Pow||LA23_0==RANGELOOKUP||LA23_0==SPARSE||LA23_0==Subtract||LA23_0==TERNARY||LA23_0==UNARY_MIN) ) {
alt23=1;
}
switch (alt23) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:300:38: p= expression
{
pushFollow(FOLLOW_expression_in_functionCall1993);
p=expression();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new ChainNode(line, handler, currentScope, s, p);
}
break;
case 70 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:301:6: ^( FUNC_CALL Sleep expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall2005);
match(input, Token.DOWN, null);
match(input,Sleep,FOLLOW_Sleep_in_functionCall2007);
pushFollow(FOLLOW_expression_in_functionCall2009);
expression82=expression();
state._fsp--;
match(input, Token.UP, null);
node = new SleepNode(line, handler,currentScope, expression82);
}
break;
case 71 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:302:6: ^( FUNC_CALL Matches s= expression r= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall2020);
match(input, Token.DOWN, null);
match(input,Matches,FOLLOW_Matches_in_functionCall2022);
pushFollow(FOLLOW_expression_in_functionCall2026);
s=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall2030);
r=expression();
state._fsp--;
match(input, Token.UP, null);
node = new MatchesNode(line, handler, currentScope, s, r);
}
break;
case 72 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:303:6: ^( FUNC_CALL Cast a= expression b= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall2041);
match(input, Token.DOWN, null);
match(input,Cast,FOLLOW_Cast_in_functionCall2043);
pushFollow(FOLLOW_expression_in_functionCall2047);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall2051);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new CastNode(line, handler, currentScope, a, b, languageRegistry, namespaceStack.asPrefix());
}
break;
case 73 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:304:6: ^( FUNC_CALL Rand expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall2062);
match(input, Token.DOWN, null);
match(input,Rand,FOLLOW_Rand_in_functionCall2064);
pushFollow(FOLLOW_expression_in_functionCall2066);
expression83=expression();
state._fsp--;
match(input, Token.UP, null);
node = new RandNode(line, handler, currentScope, expression83);
}
break;
case 74 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:305:6: ^( FUNC_CALL Round v= expression dp= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall2077);
match(input, Token.DOWN, null);
match(input,Round,FOLLOW_Round_in_functionCall2079);
pushFollow(FOLLOW_expression_in_functionCall2083);
v=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall2087);
dp=expression();
state._fsp--;
match(input, Token.UP, null);
node = new RoundNode(line, handler, currentScope, v, dp);
}
break;
case 75 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:306:6: ^( FUNC_CALL Lib expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall2098);
match(input, Token.DOWN, null);
match(input,Lib,FOLLOW_Lib_in_functionCall2100);
pushFollow(FOLLOW_expression_in_functionCall2102);
expression84=expression();
state._fsp--;
match(input, Token.UP, null);
node = new LibNode(line, handler, currentScope, expression84);
}
break;
case 76 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:307:6: ^( FUNC_CALL Call a= expression b= expression c= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall2113);
match(input, Token.DOWN, null);
match(input,Call,FOLLOW_Call_in_functionCall2115);
pushFollow(FOLLOW_expression_in_functionCall2119);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall2123);
b=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall2127);
c=expression();
state._fsp--;
match(input, Token.UP, null);
node = new CallNode(line, handler, currentScope, a, b, c);
}
break;
case 77 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:308:6: ^( FUNC_CALL New a= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall2138);
match(input, Token.DOWN, null);
match(input,New,FOLLOW_New_in_functionCall2140);
pushFollow(FOLLOW_expression_in_functionCall2145);
a=expression();
state._fsp--;
match(input, Token.UP, null);
node = new NewNode(line, handler, currentScope, a, languageRegistry, namespaceStack.asPrefix());
}
break;
case 78 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:309:6: ^( FUNC_CALL GenSchema a= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall2156);
match(input, Token.DOWN, null);
match(input,GenSchema,FOLLOW_GenSchema_in_functionCall2158);
pushFollow(FOLLOW_expression_in_functionCall2162);
a=expression();
state._fsp--;
match(input, Token.UP, null);
node = new GenSchemaNode(line, handler, currentScope, a, languageRegistry, namespaceStack.asPrefix());
}
break;
case 79 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:310:6: ^( FUNC_CALL GenStruct Identifier a= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall2173);
match(input, Token.DOWN, null);
match(input,GenStruct,FOLLOW_GenStruct_in_functionCall2175);
Identifier85=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_functionCall2177);
pushFollow(FOLLOW_expression_in_functionCall2181);
a=expression();
state._fsp--;
match(input, Token.UP, null);
node = new GenStructNode(line, handler, currentScope, (Identifier85!=null?Identifier85.getText():null), a, languageRegistry, namespaceStack.asPrefix());
}
break;
case 80 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:311:6: ^( FUNC_CALL Template t= expression p= expression )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall2192);
match(input, Token.DOWN, null);
match(input,Template,FOLLOW_Template_in_functionCall2194);
pushFollow(FOLLOW_expression_in_functionCall2198);
t=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall2202);
p=expression();
state._fsp--;
match(input, Token.UP, null);
node = new TemplateNode(line, handler, currentScope, t, p);
}
break;
case 81 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:312:6: ^( FUNC_CALL Spawn p= expression (e= expression f= expression )? )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall2213);
match(input, Token.DOWN, null);
match(input,Spawn,FOLLOW_Spawn_in_functionCall2215);
pushFollow(FOLLOW_expression_in_functionCall2219);
p=expression();
state._fsp--;
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:312:37: (e= expression f= expression )?
int alt24=2;
int LA24_0 = input.LA(1);
if ( (LA24_0==Add||LA24_0==And||LA24_0==Bool||LA24_0==Divide||LA24_0==Equals||(LA24_0 >= GT && LA24_0 <= GTEquals)||LA24_0==In||LA24_0==Integer||(LA24_0 >= LOOKUP && LA24_0 <= LTEquals)||LA24_0==Long||(LA24_0 >= Modulus && LA24_0 <= NEquals)||(LA24_0 >= Null && LA24_0 <= Number)||LA24_0==Or||LA24_0==Pow||LA24_0==RANGELOOKUP||LA24_0==SPARSE||LA24_0==Subtract||LA24_0==TERNARY||LA24_0==UNARY_MIN) ) {
alt24=1;
}
switch (alt24) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:312:38: e= expression f= expression
{
pushFollow(FOLLOW_expression_in_functionCall2224);
e=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_functionCall2228);
f=expression();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new SpawnNode(line, handler, currentScope, p, e, f);
}
break;
case 82 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:313:6: ^( FUNC_CALL Defined Identifier )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall2241);
match(input, Token.DOWN, null);
match(input,Defined,FOLLOW_Defined_in_functionCall2243);
Identifier86=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_functionCall2245);
match(input, Token.UP, null);
node = new DefinedNode(line, handler, currentScope, (Identifier86!=null?Identifier86.getText():null), namespaceStack.asPrefix()) ;
}
break;
case 83 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:314:6: ^( KERNEL_CALL KernelIdentifier ( exprList )? )
{
match(input,KERNEL_CALL,FOLLOW_KERNEL_CALL_in_functionCall2256);
match(input, Token.DOWN, null);
KernelIdentifier87=(CommonTree)match(input,KernelIdentifier,FOLLOW_KernelIdentifier_in_functionCall2258);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:314:37: ( exprList )?
int alt25=2;
int LA25_0 = input.LA(1);
if ( (LA25_0==EXP_LIST) ) {
alt25=1;
}
switch (alt25) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:314:37: exprList
{
pushFollow(FOLLOW_exprList_in_functionCall2260);
exprList88=exprList();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new KernelCallNode(line, handler, currentScope, (KernelIdentifier87!=null?KernelIdentifier87.getText():null), exprList88);
}
break;
case 84 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:315:6: ^( QUALIFIED_FUNC_CALL DottedIdentifier ( exprList )? )
{
match(input,QUALIFIED_FUNC_CALL,FOLLOW_QUALIFIED_FUNC_CALL_in_functionCall2272);
match(input, Token.DOWN, null);
DottedIdentifier89=(CommonTree)match(input,DottedIdentifier,FOLLOW_DottedIdentifier_in_functionCall2274);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:315:45: ( exprList )?
int alt26=2;
int LA26_0 = input.LA(1);
if ( (LA26_0==EXP_LIST) ) {
alt26=1;
}
switch (alt26) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:315:45: exprList
{
pushFollow(FOLLOW_exprList_in_functionCall2276);
exprList90=exprList();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = new QualifiedFuncCallNode(line, handler, currentScope, (DottedIdentifier89!=null?DottedIdentifier89.getText():null),
exprList90, languageRegistry, importHandler, namespaceStack.asPrefix());
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "functionCall"
// $ANTLR start "ifStatement"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:319:1: ifStatement returns [ReflexNode node] : ^( IF ( ^( EXP expression b1= block ) )+ ( ^( EXP b2= block ) )? ) ;
public final ReflexNode ifStatement() throws RecognitionException {
ReflexNode node = null;
ReflexNode b1 =null;
ReflexNode b2 =null;
ReflexNode expression91 =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
IfNode ifNode = new IfNode(line, handler, currentScope);
node = ifNode;
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:326:3: ( ^( IF ( ^( EXP expression b1= block ) )+ ( ^( EXP b2= block ) )? ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:326:6: ^( IF ( ^( EXP expression b1= block ) )+ ( ^( EXP b2= block ) )? )
{
match(input,IF,FOLLOW_IF_in_ifStatement2305);
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:327:8: ( ^( EXP expression b1= block ) )+
int cnt28=0;
loop28:
while (true) {
int alt28=2;
int LA28_0 = input.LA(1);
if ( (LA28_0==EXP) ) {
int LA28_1 = input.LA(2);
if ( (LA28_1==DOWN) ) {
int LA28_3 = input.LA(3);
if ( (LA28_3==Add||LA28_3==And||LA28_3==Bool||LA28_3==Divide||LA28_3==Equals||(LA28_3 >= GT && LA28_3 <= GTEquals)||LA28_3==In||LA28_3==Integer||(LA28_3 >= LOOKUP && LA28_3 <= LTEquals)||LA28_3==Long||(LA28_3 >= Modulus && LA28_3 <= NEquals)||(LA28_3 >= Null && LA28_3 <= Number)||LA28_3==Or||LA28_3==Pow||LA28_3==RANGELOOKUP||LA28_3==SPARSE||LA28_3==Subtract||LA28_3==TERNARY||LA28_3==UNARY_MIN) ) {
alt28=1;
}
}
}
switch (alt28) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:327:9: ^( EXP expression b1= block )
{
match(input,EXP,FOLLOW_EXP_in_ifStatement2316);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_ifStatement2318);
expression91=expression();
state._fsp--;
pushFollow(FOLLOW_block_in_ifStatement2322);
b1=block();
state._fsp--;
match(input, Token.UP, null);
ifNode.addChoice(expression91,b1);
}
break;
default :
if ( cnt28 >= 1 ) break loop28;
EarlyExitException eee = new EarlyExitException(28, input);
throw eee;
}
cnt28++;
}
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:328:8: ( ^( EXP b2= block ) )?
int alt29=2;
int LA29_0 = input.LA(1);
if ( (LA29_0==EXP) ) {
alt29=1;
}
switch (alt29) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:328:9: ^( EXP b2= block )
{
match(input,EXP,FOLLOW_EXP_in_ifStatement2337);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_block_in_ifStatement2341);
b2=block();
state._fsp--;
match(input, Token.UP, null);
ifNode.addChoice(new AtomNode(line, handler, currentScope, true),b2);
}
break;
}
match(input, Token.UP, null);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "ifStatement"
// $ANTLR start "forStatement"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:333:1: forStatement returns [ReflexNode node] : ( ^( FORLIST Identifier a= expression block ) | ^( FORTO Identifier a= expression b= expression block ) );
public final ReflexNode forStatement() throws RecognitionException {
ReflexNode node = null;
CommonTree Identifier92=null;
CommonTree Identifier94=null;
ReflexNode a =null;
ReflexNode b =null;
ReflexNode block93 =null;
ReflexNode block95 =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:338:3: ( ^( FORLIST Identifier a= expression block ) | ^( FORTO Identifier a= expression b= expression block ) )
int alt30=2;
int LA30_0 = input.LA(1);
if ( (LA30_0==FORLIST) ) {
alt30=1;
}
else if ( (LA30_0==FORTO) ) {
alt30=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 30, 0, input);
throw nvae;
}
switch (alt30) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:339:6: ^( FORLIST Identifier a= expression block )
{
match(input,FORLIST,FOLLOW_FORLIST_in_forStatement2392);
match(input, Token.DOWN, null);
Identifier92=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_forStatement2394);
pushFollow(FOLLOW_expression_in_forStatement2398);
a=expression();
state._fsp--;
pushFollow(FOLLOW_block_in_forStatement2400);
block93=block();
state._fsp--;
match(input, Token.UP, null);
node = new ForInStatementNode(line, handler, currentScope, (Identifier92!=null?Identifier92.getText():null), a, block93);
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:340:6: ^( FORTO Identifier a= expression b= expression block )
{
match(input,FORTO,FOLLOW_FORTO_in_forStatement2411);
match(input, Token.DOWN, null);
Identifier94=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_forStatement2413);
pushFollow(FOLLOW_expression_in_forStatement2417);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_forStatement2421);
b=expression();
state._fsp--;
pushFollow(FOLLOW_block_in_forStatement2423);
block95=block();
state._fsp--;
match(input, Token.UP, null);
node = new ForStatementNode(line, handler, currentScope, (Identifier94!=null?Identifier94.getText():null), a, b, block95);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "forStatement"
// $ANTLR start "pforStatement"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:343:1: pforStatement returns [ReflexNode node] : ( ^( PFORLIST Identifier a= expression block ) | ^( PFORTO Identifier a= expression b= expression block ) );
public final ReflexNode pforStatement() throws RecognitionException {
ReflexNode node = null;
CommonTree Identifier96=null;
CommonTree Identifier98=null;
ReflexNode a =null;
ReflexNode b =null;
ReflexNode block97 =null;
ReflexNode block99 =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:348:3: ( ^( PFORLIST Identifier a= expression block ) | ^( PFORTO Identifier a= expression b= expression block ) )
int alt31=2;
int LA31_0 = input.LA(1);
if ( (LA31_0==PFORLIST) ) {
alt31=1;
}
else if ( (LA31_0==PFORTO) ) {
alt31=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 31, 0, input);
throw nvae;
}
switch (alt31) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:349:6: ^( PFORLIST Identifier a= expression block )
{
match(input,PFORLIST,FOLLOW_PFORLIST_in_pforStatement2454);
match(input, Token.DOWN, null);
Identifier96=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_pforStatement2456);
pushFollow(FOLLOW_expression_in_pforStatement2460);
a=expression();
state._fsp--;
pushFollow(FOLLOW_block_in_pforStatement2462);
block97=block();
state._fsp--;
match(input, Token.UP, null);
node = new PForInStatementNode(line, handler, currentScope, (Identifier96!=null?Identifier96.getText():null), a, block97);
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:350:6: ^( PFORTO Identifier a= expression b= expression block )
{
match(input,PFORTO,FOLLOW_PFORTO_in_pforStatement2473);
match(input, Token.DOWN, null);
Identifier98=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_pforStatement2475);
pushFollow(FOLLOW_expression_in_pforStatement2479);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_pforStatement2483);
b=expression();
state._fsp--;
pushFollow(FOLLOW_block_in_pforStatement2485);
block99=block();
state._fsp--;
match(input, Token.UP, null);
node = new PForStatementNode(line, handler, currentScope, (Identifier98!=null?Identifier98.getText():null), a, b, block99);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "pforStatement"
// $ANTLR start "whileStatement"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:353:1: whileStatement returns [ReflexNode node] : ^( While expression block ) ;
public final ReflexNode whileStatement() throws RecognitionException {
ReflexNode node = null;
ReflexNode expression100 =null;
ReflexNode block101 =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:358:3: ( ^( While expression block ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:358:6: ^( While expression block )
{
match(input,While,FOLLOW_While_in_whileStatement2512);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_whileStatement2514);
expression100=expression();
state._fsp--;
pushFollow(FOLLOW_block_in_whileStatement2516);
block101=block();
state._fsp--;
match(input, Token.UP, null);
node = new WhileStatementNode(line, handler, currentScope, expression100, block101);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "whileStatement"
// $ANTLR start "guardedStatement"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:361:1: guardedStatement returns [ReflexNode node] : ^( Try g= block Identifier c= block ) ;
public final ReflexNode guardedStatement() throws RecognitionException {
ReflexNode node = null;
CommonTree Identifier102=null;
ReflexNode g =null;
ReflexNode c =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:366:3: ( ^( Try g= block Identifier c= block ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:366:5: ^( Try g= block Identifier c= block )
{
match(input,Try,FOLLOW_Try_in_guardedStatement2542);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_block_in_guardedStatement2546);
g=block();
state._fsp--;
Identifier102=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_guardedStatement2548);
pushFollow(FOLLOW_block_in_guardedStatement2552);
c=block();
state._fsp--;
match(input, Token.UP, null);
node = new GuardedNode(line, handler, currentScope, g, (Identifier102!=null?Identifier102.getText():null), c);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "guardedStatement"
// $ANTLR start "sparsesep"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:369:1: sparsesep : '-' ;
public final void sparsesep() throws RecognitionException {
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:369:10: ( '-' )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:370:4: '-'
{
match(input,Subtract,FOLLOW_Subtract_in_sparsesep2568);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "sparsesep"
// $ANTLR start "sparsematrix"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:372:1: sparsematrix returns [int dim] : ^( SPARSE ( sparsesep )+ ) ;
public final int sparsematrix() throws RecognitionException {
int dim = 0;
dim = 1;
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:376:4: ( ^( SPARSE ( sparsesep )+ ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:376:6: ^( SPARSE ( sparsesep )+ )
{
match(input,SPARSE,FOLLOW_SPARSE_in_sparsematrix2589);
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:376:15: ( sparsesep )+
int cnt32=0;
loop32:
while (true) {
int alt32=2;
int LA32_0 = input.LA(1);
if ( (LA32_0==Subtract) ) {
alt32=1;
}
switch (alt32) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:376:16: sparsesep
{
pushFollow(FOLLOW_sparsesep_in_sparsematrix2592);
sparsesep();
state._fsp--;
dim++;
}
break;
default :
if ( cnt32 >= 1 ) break loop32;
EarlyExitException eee = new EarlyExitException(32, input);
throw eee;
}
cnt32++;
}
match(input, Token.UP, null);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return dim;
}
// $ANTLR end "sparsematrix"
// $ANTLR start "idList"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:379:1: idList returns [java.util.List i] : ^( ID_LIST ( Identifier )+ ) ;
public final java.util.List idList() throws RecognitionException {
java.util.List i = null;
CommonTree Identifier103=null;
i = new java.util.ArrayList();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:383:3: ( ^( ID_LIST ( Identifier )+ ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:383:6: ^( ID_LIST ( Identifier )+ )
{
match(input,ID_LIST,FOLLOW_ID_LIST_in_idList2623);
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:383:16: ( Identifier )+
int cnt33=0;
loop33:
while (true) {
int alt33=2;
int LA33_0 = input.LA(1);
if ( (LA33_0==Identifier) ) {
alt33=1;
}
switch (alt33) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:383:17: Identifier
{
Identifier103=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_idList2626);
i.add((Identifier103!=null?Identifier103.getText():null));
}
break;
default :
if ( cnt33 >= 1 ) break loop33;
EarlyExitException eee = new EarlyExitException(33, input);
throw eee;
}
cnt33++;
}
match(input, Token.UP, null);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return i;
}
// $ANTLR end "idList"
// $ANTLR start "exprList"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:386:1: exprList returns [java.util.List e] : ^( EXP_LIST ( expression )+ ) ;
public final java.util.List exprList() throws RecognitionException {
java.util.List e = null;
ReflexNode expression104 =null;
e = new java.util.ArrayList();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:390:3: ( ^( EXP_LIST ( expression )+ ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:390:6: ^( EXP_LIST ( expression )+ )
{
match(input,EXP_LIST,FOLLOW_EXP_LIST_in_exprList2655);
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:390:17: ( expression )+
int cnt34=0;
loop34:
while (true) {
int alt34=2;
int LA34_0 = input.LA(1);
if ( (LA34_0==Add||LA34_0==And||LA34_0==Bool||LA34_0==Divide||LA34_0==Equals||(LA34_0 >= GT && LA34_0 <= GTEquals)||LA34_0==In||LA34_0==Integer||(LA34_0 >= LOOKUP && LA34_0 <= LTEquals)||LA34_0==Long||(LA34_0 >= Modulus && LA34_0 <= NEquals)||(LA34_0 >= Null && LA34_0 <= Number)||LA34_0==Or||LA34_0==Pow||LA34_0==RANGELOOKUP||LA34_0==SPARSE||LA34_0==Subtract||LA34_0==TERNARY||LA34_0==UNARY_MIN) ) {
alt34=1;
}
switch (alt34) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:390:18: expression
{
pushFollow(FOLLOW_expression_in_exprList2658);
expression104=expression();
state._fsp--;
e.add(expression104);
}
break;
default :
if ( cnt34 >= 1 ) break loop34;
EarlyExitException eee = new EarlyExitException(34, input);
throw eee;
}
cnt34++;
}
match(input, Token.UP, null);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return e;
}
// $ANTLR end "exprList"
// $ANTLR start "expression"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:393:1: expression returns [ReflexNode node] : ( ^( TERNARY a= expression b= expression c= expression ) | ^( In a= expression b= expression ) | ^( '||' a= expression b= expression ) | ^( '&&' a= expression b= expression ) | ^( '==' a= expression b= expression ) | ^( '!=' a= expression b= expression ) | ^( '>=' a= expression b= expression ) | ^( '<=' a= expression b= expression ) | ^( '>' a= expression b= expression ) | ^( '<' a= expression b= expression ) | ^( '+' a= expression b= expression ) | ^( '-' a= expression b= expression ) | ^( '*' a= expression b= expression ) | ^( '/' a= expression b= expression ) | ^( '%' a= expression b= expression ) | ^( '^' a= expression b= expression ) | ^( UNARY_MIN a= expression ) | ^( NEGATE a= expression ) | Number | Integer | Long | Bool | Null | sparsematrix | lookup );
public final ReflexNode expression() throws RecognitionException {
ReflexNode node = null;
CommonTree Number105=null;
CommonTree Integer106=null;
CommonTree Long107=null;
CommonTree Bool108=null;
ReflexNode a =null;
ReflexNode b =null;
ReflexNode c =null;
int sparsematrix109 =0;
ReflexNode lookup110 =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:398:3: ( ^( TERNARY a= expression b= expression c= expression ) | ^( In a= expression b= expression ) | ^( '||' a= expression b= expression ) | ^( '&&' a= expression b= expression ) | ^( '==' a= expression b= expression ) | ^( '!=' a= expression b= expression ) | ^( '>=' a= expression b= expression ) | ^( '<=' a= expression b= expression ) | ^( '>' a= expression b= expression ) | ^( '<' a= expression b= expression ) | ^( '+' a= expression b= expression ) | ^( '-' a= expression b= expression ) | ^( '*' a= expression b= expression ) | ^( '/' a= expression b= expression ) | ^( '%' a= expression b= expression ) | ^( '^' a= expression b= expression ) | ^( UNARY_MIN a= expression ) | ^( NEGATE a= expression ) | Number | Integer | Long | Bool | Null | sparsematrix | lookup )
int alt35=25;
switch ( input.LA(1) ) {
case TERNARY:
{
alt35=1;
}
break;
case In:
{
alt35=2;
}
break;
case Or:
{
alt35=3;
}
break;
case And:
{
alt35=4;
}
break;
case Equals:
{
alt35=5;
}
break;
case NEquals:
{
alt35=6;
}
break;
case GTEquals:
{
alt35=7;
}
break;
case LTEquals:
{
alt35=8;
}
break;
case GT:
{
alt35=9;
}
break;
case LT:
{
alt35=10;
}
break;
case Add:
{
alt35=11;
}
break;
case Subtract:
{
alt35=12;
}
break;
case Multiply:
{
alt35=13;
}
break;
case Divide:
{
alt35=14;
}
break;
case Modulus:
{
alt35=15;
}
break;
case Pow:
{
alt35=16;
}
break;
case UNARY_MIN:
{
alt35=17;
}
break;
case NEGATE:
{
alt35=18;
}
break;
case Number:
{
alt35=19;
}
break;
case Integer:
{
alt35=20;
}
break;
case Long:
{
alt35=21;
}
break;
case Bool:
{
alt35=22;
}
break;
case Null:
{
alt35=23;
}
break;
case SPARSE:
{
alt35=24;
}
break;
case LOOKUP:
case RANGELOOKUP:
{
alt35=25;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 35, 0, input);
throw nvae;
}
switch (alt35) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:398:6: ^( TERNARY a= expression b= expression c= expression )
{
match(input,TERNARY,FOLLOW_TERNARY_in_expression2687);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2691);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2695);
b=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2699);
c=expression();
state._fsp--;
match(input, Token.UP, null);
node = new TernaryNode(line, handler, currentScope, a, b, c);
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:399:6: ^( In a= expression b= expression )
{
match(input,In,FOLLOW_In_in_expression2710);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2714);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2718);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new InNode(line, handler, currentScope, a, b);
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:400:6: ^( '||' a= expression b= expression )
{
match(input,Or,FOLLOW_Or_in_expression2729);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2733);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2737);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new OrNode(line, handler, currentScope, a, b);
}
break;
case 4 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:401:6: ^( '&&' a= expression b= expression )
{
match(input,And,FOLLOW_And_in_expression2748);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2752);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2756);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new AndNode(line, handler, currentScope, a, b);
}
break;
case 5 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:402:6: ^( '==' a= expression b= expression )
{
match(input,Equals,FOLLOW_Equals_in_expression2767);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2771);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2775);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new EqualsNode(line, handler, currentScope, a, b);
}
break;
case 6 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:403:6: ^( '!=' a= expression b= expression )
{
match(input,NEquals,FOLLOW_NEquals_in_expression2786);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2790);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2794);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new NotEqualsNode(line,handler, currentScope, a, b);
}
break;
case 7 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:404:6: ^( '>=' a= expression b= expression )
{
match(input,GTEquals,FOLLOW_GTEquals_in_expression2805);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2809);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2813);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new GTEqualsNode(line, handler, currentScope, a, b);
}
break;
case 8 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:405:6: ^( '<=' a= expression b= expression )
{
match(input,LTEquals,FOLLOW_LTEquals_in_expression2824);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2828);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2832);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new LTEqualsNode(line, handler, currentScope, a, b);
}
break;
case 9 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:406:6: ^( '>' a= expression b= expression )
{
match(input,GT,FOLLOW_GT_in_expression2843);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2847);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2851);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new GTNode(line, handler, currentScope, a, b);
}
break;
case 10 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:407:6: ^( '<' a= expression b= expression )
{
match(input,LT,FOLLOW_LT_in_expression2862);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2866);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2870);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new LTNode(line, handler, currentScope, a, b);
}
break;
case 11 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:408:6: ^( '+' a= expression b= expression )
{
match(input,Add,FOLLOW_Add_in_expression2881);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2885);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2889);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new AddNode(line, handler,currentScope, a, b);
}
break;
case 12 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:409:6: ^( '-' a= expression b= expression )
{
match(input,Subtract,FOLLOW_Subtract_in_expression2900);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2904);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2908);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new SubNode(line, handler, currentScope, a, b);
}
break;
case 13 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:410:6: ^( '*' a= expression b= expression )
{
match(input,Multiply,FOLLOW_Multiply_in_expression2919);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2923);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2927);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new MulNode(line, handler, currentScope, a, b);
}
break;
case 14 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:411:6: ^( '/' a= expression b= expression )
{
match(input,Divide,FOLLOW_Divide_in_expression2938);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2942);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2946);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new DivNode(line, handler, currentScope, a, b);
}
break;
case 15 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:412:6: ^( '%' a= expression b= expression )
{
match(input,Modulus,FOLLOW_Modulus_in_expression2957);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2961);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2965);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new ModNode(line, handler, currentScope, a, b);
}
break;
case 16 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:413:6: ^( '^' a= expression b= expression )
{
match(input,Pow,FOLLOW_Pow_in_expression2976);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2980);
a=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2984);
b=expression();
state._fsp--;
match(input, Token.UP, null);
node = new PowNode(line, handler, currentScope, a, b);
}
break;
case 17 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:414:6: ^( UNARY_MIN a= expression )
{
match(input,UNARY_MIN,FOLLOW_UNARY_MIN_in_expression2995);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2999);
a=expression();
state._fsp--;
match(input, Token.UP, null);
node = new UnaryMinusNode(line, handler, currentScope, a);
}
break;
case 18 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:415:6: ^( NEGATE a= expression )
{
match(input,NEGATE,FOLLOW_NEGATE_in_expression3010);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression3014);
a=expression();
state._fsp--;
match(input, Token.UP, null);
node = new NegateNode(line, handler, currentScope, a);
}
break;
case 19 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:416:6: Number
{
Number105=(CommonTree)match(input,Number,FOLLOW_Number_in_expression3024);
node = new AtomNode(line, handler, currentScope, Double.parseDouble((Number105!=null?Number105.getText():null)));
}
break;
case 20 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:417:6: Integer
{
Integer106=(CommonTree)match(input,Integer,FOLLOW_Integer_in_expression3033);
node = AtomNode.getIntegerAtom(line, handler, currentScope, (Integer106!=null?Integer106.getText():null));
}
break;
case 21 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:418:6: Long
{
Long107=(CommonTree)match(input,Long,FOLLOW_Long_in_expression3042);
node = new AtomNode(line, handler, currentScope, java.lang.Long.parseLong((Long107!=null?Long107.getText():null)));
}
break;
case 22 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:419:6: Bool
{
Bool108=(CommonTree)match(input,Bool,FOLLOW_Bool_in_expression3051);
node = new AtomNode(line, handler, currentScope, Boolean.parseBoolean((Bool108!=null?Bool108.getText():null)));
}
break;
case 23 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:420:6: Null
{
match(input,Null,FOLLOW_Null_in_expression3060);
node = new AtomNode(line, handler, currentScope);
}
break;
case 24 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:421:6: sparsematrix
{
pushFollow(FOLLOW_sparsematrix_in_expression3069);
sparsematrix109=sparsematrix();
state._fsp--;
node = new AtomNode(line, handler, currentScope, new MatrixDim(sparsematrix109));
}
break;
case 25 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:422:6: lookup
{
pushFollow(FOLLOW_lookup_in_expression3078);
lookup110=lookup();
state._fsp--;
node = lookup110;
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "expression"
// $ANTLR start "list"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:426:1: list returns [ReflexNode node] : ^( LIST ( exprList )? ) ;
public final ReflexNode list() throws RecognitionException {
ReflexNode node = null;
java.util.List exprList111 =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:431:3: ( ^( LIST ( exprList )? ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:431:5: ^( LIST ( exprList )? )
{
match(input,LIST,FOLLOW_LIST_in_list3104);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:431:12: ( exprList )?
int alt36=2;
int LA36_0 = input.LA(1);
if ( (LA36_0==EXP_LIST) ) {
alt36=1;
}
switch (alt36) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:431:12: exprList
{
pushFollow(FOLLOW_exprList_in_list3106);
exprList111=exprList();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
}
node = new ListNode(line, handler, currentScope, exprList111);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "list"
// $ANTLR start "mapdef"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:434:1: mapdef returns [ReflexNode node] : ^( MAPDEF ( keyValList )? ) ;
public final ReflexNode mapdef() throws RecognitionException {
ReflexNode node = null;
java.util.List keyValList112 =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:439:3: ( ^( MAPDEF ( keyValList )? ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:439:5: ^( MAPDEF ( keyValList )? )
{
match(input,MAPDEF,FOLLOW_MAPDEF_in_mapdef3133);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:439:14: ( keyValList )?
int alt37=2;
int LA37_0 = input.LA(1);
if ( (LA37_0==KEYVAL_LIST) ) {
alt37=1;
}
switch (alt37) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:439:14: keyValList
{
pushFollow(FOLLOW_keyValList_in_mapdef3135);
keyValList112=keyValList();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
}
node = new MapNode(line, handler,currentScope, keyValList112);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "mapdef"
// $ANTLR start "keyValList"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:442:1: keyValList returns [java.util.List e] : ^( KEYVAL_LIST ( keyval )+ ) ;
public final java.util.List keyValList() throws RecognitionException {
java.util.List e = null;
ReflexNode keyval113 =null;
e = new java.util.ArrayList();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:446:3: ( ^( KEYVAL_LIST ( keyval )+ ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:446:6: ^( KEYVAL_LIST ( keyval )+ )
{
match(input,KEYVAL_LIST,FOLLOW_KEYVAL_LIST_in_keyValList3163);
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:446:20: ( keyval )+
int cnt38=0;
loop38:
while (true) {
int alt38=2;
int LA38_0 = input.LA(1);
if ( (LA38_0==KEYVAL) ) {
alt38=1;
}
switch (alt38) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:446:21: keyval
{
pushFollow(FOLLOW_keyval_in_keyValList3166);
keyval113=keyval();
state._fsp--;
e.add(keyval113);
}
break;
default :
if ( cnt38 >= 1 ) break loop38;
EarlyExitException eee = new EarlyExitException(38, input);
throw eee;
}
cnt38++;
}
match(input, Token.UP, null);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return e;
}
// $ANTLR end "keyValList"
// $ANTLR start "keyval"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:449:1: keyval returns [ReflexNode node] : ^( KEYVAL k= expression v= expression ) ;
public final ReflexNode keyval() throws RecognitionException {
ReflexNode node = null;
ReflexNode k =null;
ReflexNode v =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:454:3: ( ^( KEYVAL k= expression v= expression ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:454:6: ^( KEYVAL k= expression v= expression )
{
match(input,KEYVAL,FOLLOW_KEYVAL_in_keyval3195);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_keyval3199);
k=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_keyval3203);
v=expression();
state._fsp--;
match(input, Token.UP, null);
node = new KeyValNode(line, handler, currentScope, k, v);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "keyval"
// $ANTLR start "lookup"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:457:1: lookup returns [ReflexNode node] : ( ^( LOOKUP functionCall (i= indexes )? ) | ^( LOOKUP PropertyPlaceholder ) | ^( RANGELOOKUP Identifier rangeindex ) | ^( RANGELOOKUP DottedIdentifier rangeindex ) | ^( LOOKUP list (i= indexes )? ) | ^( LOOKUP mapdef (i= indexes )? ) | ^( LOOKUP expression (i= indexes )? ) | ^( LOOKUP DottedIdentifier (x= indexes )? ) | ^( LOOKUP Identifier (x= indexes )? ) | ^( LOOKUP String (x= indexes )? ) | ^( LOOKUP QuotedString (x= indexes )? ) );
public final ReflexNode lookup() throws RecognitionException {
ReflexNode node = null;
CommonTree PropertyPlaceholder115=null;
CommonTree Identifier116=null;
CommonTree DottedIdentifier118=null;
CommonTree DottedIdentifier123=null;
CommonTree Identifier124=null;
CommonTree String125=null;
CommonTree QuotedString126=null;
java.util.List> i =null;
java.util.List> x =null;
ReflexNode functionCall114 =null;
TreeRuleReturnScope rangeindex117 =null;
TreeRuleReturnScope rangeindex119 =null;
ReflexNode list120 =null;
ReflexNode mapdef121 =null;
ReflexNode expression122 =null;
CommonTree ahead = (CommonTree) input.LT(1);
int line = ahead.getToken().getLine();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:462:3: ( ^( LOOKUP functionCall (i= indexes )? ) | ^( LOOKUP PropertyPlaceholder ) | ^( RANGELOOKUP Identifier rangeindex ) | ^( RANGELOOKUP DottedIdentifier rangeindex ) | ^( LOOKUP list (i= indexes )? ) | ^( LOOKUP mapdef (i= indexes )? ) | ^( LOOKUP expression (i= indexes )? ) | ^( LOOKUP DottedIdentifier (x= indexes )? ) | ^( LOOKUP Identifier (x= indexes )? ) | ^( LOOKUP String (x= indexes )? ) | ^( LOOKUP QuotedString (x= indexes )? ) )
int alt47=11;
int LA47_0 = input.LA(1);
if ( (LA47_0==LOOKUP) ) {
int LA47_1 = input.LA(2);
if ( (LA47_1==DOWN) ) {
switch ( input.LA(3) ) {
case PropertyPlaceholder:
{
alt47=2;
}
break;
case DottedIdentifier:
{
alt47=8;
}
break;
case Identifier:
{
alt47=9;
}
break;
case String:
{
alt47=10;
}
break;
case QuotedString:
{
alt47=11;
}
break;
case FUNC_CALL:
case KERNEL_CALL:
case QUALIFIED_FUNC_CALL:
{
alt47=1;
}
break;
case LIST:
{
alt47=5;
}
break;
case MAPDEF:
{
alt47=6;
}
break;
case Add:
case And:
case Bool:
case Divide:
case Equals:
case GT:
case GTEquals:
case In:
case Integer:
case LOOKUP:
case LT:
case LTEquals:
case Long:
case Modulus:
case Multiply:
case NEGATE:
case NEquals:
case Null:
case Number:
case Or:
case Pow:
case RANGELOOKUP:
case SPARSE:
case Subtract:
case TERNARY:
case UNARY_MIN:
{
alt47=7;
}
break;
default:
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 47, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 47, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else if ( (LA47_0==RANGELOOKUP) ) {
int LA47_2 = input.LA(2);
if ( (LA47_2==DOWN) ) {
int LA47_4 = input.LA(3);
if ( (LA47_4==Identifier) ) {
alt47=3;
}
else if ( (LA47_4==DottedIdentifier) ) {
alt47=4;
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 47, 4, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 47, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 47, 0, input);
throw nvae;
}
switch (alt47) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:462:6: ^( LOOKUP functionCall (i= indexes )? )
{
match(input,LOOKUP,FOLLOW_LOOKUP_in_lookup3230);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_functionCall_in_lookup3232);
functionCall114=functionCall();
state._fsp--;
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:462:29: (i= indexes )?
int alt39=2;
int LA39_0 = input.LA(1);
if ( (LA39_0==INDEXES) ) {
alt39=1;
}
switch (alt39) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:462:29: i= indexes
{
pushFollow(FOLLOW_indexes_in_lookup3236);
i=indexes();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = i != null ? new LookupNode(line, handler, currentScope, functionCall114, i) : functionCall114;
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:463:6: ^( LOOKUP PropertyPlaceholder )
{
match(input,LOOKUP,FOLLOW_LOOKUP_in_lookup3248);
match(input, Token.DOWN, null);
PropertyPlaceholder115=(CommonTree)match(input,PropertyPlaceholder,FOLLOW_PropertyPlaceholder_in_lookup3250);
match(input, Token.UP, null);
node = new PropertyPlaceholderNode(line, handler, currentScope, (PropertyPlaceholder115!=null?PropertyPlaceholder115.getText():null));
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:464:6: ^( RANGELOOKUP Identifier rangeindex )
{
match(input,RANGELOOKUP,FOLLOW_RANGELOOKUP_in_lookup3262);
match(input, Token.DOWN, null);
Identifier116=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_lookup3264);
pushFollow(FOLLOW_rangeindex_in_lookup3266);
rangeindex117=rangeindex();
state._fsp--;
match(input, Token.UP, null);
node = new RangeLookupNode(line, handler, currentScope, new IdentifierNode(line, handler, currentScope, (Identifier116!=null?Identifier116.getText():null), namespaceStack.asPrefix()), (rangeindex117!=null?((ReflexTreeWalker.rangeindex_return)rangeindex117).ste:null), (rangeindex117!=null?((ReflexTreeWalker.rangeindex_return)rangeindex117).ed:null));
}
break;
case 4 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:465:6: ^( RANGELOOKUP DottedIdentifier rangeindex )
{
match(input,RANGELOOKUP,FOLLOW_RANGELOOKUP_in_lookup3277);
match(input, Token.DOWN, null);
DottedIdentifier118=(CommonTree)match(input,DottedIdentifier,FOLLOW_DottedIdentifier_in_lookup3279);
pushFollow(FOLLOW_rangeindex_in_lookup3281);
rangeindex119=rangeindex();
state._fsp--;
match(input, Token.UP, null);
node = new RangeLookupNode(line, handler, currentScope, new IdentifierNode(line, handler, currentScope, (DottedIdentifier118!=null?DottedIdentifier118.getText():null), namespaceStack.asPrefix()), (rangeindex119!=null?((ReflexTreeWalker.rangeindex_return)rangeindex119).ste:null), (rangeindex119!=null?((ReflexTreeWalker.rangeindex_return)rangeindex119).ed:null));
}
break;
case 5 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:466:6: ^( LOOKUP list (i= indexes )? )
{
match(input,LOOKUP,FOLLOW_LOOKUP_in_lookup3292);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_list_in_lookup3294);
list120=list();
state._fsp--;
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:466:21: (i= indexes )?
int alt40=2;
int LA40_0 = input.LA(1);
if ( (LA40_0==INDEXES) ) {
alt40=1;
}
switch (alt40) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:466:21: i= indexes
{
pushFollow(FOLLOW_indexes_in_lookup3298);
i=indexes();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = i != null ? new LookupNode(line, handler, currentScope, list120, i) : list120;
}
break;
case 6 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:467:6: ^( LOOKUP mapdef (i= indexes )? )
{
match(input,LOOKUP,FOLLOW_LOOKUP_in_lookup3310);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_mapdef_in_lookup3312);
mapdef121=mapdef();
state._fsp--;
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:467:23: (i= indexes )?
int alt41=2;
int LA41_0 = input.LA(1);
if ( (LA41_0==INDEXES) ) {
alt41=1;
}
switch (alt41) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:467:23: i= indexes
{
pushFollow(FOLLOW_indexes_in_lookup3316);
i=indexes();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = i != null ? new LookupNode(line, handler, currentScope, mapdef121, i) : mapdef121;
}
break;
case 7 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:468:6: ^( LOOKUP expression (i= indexes )? )
{
match(input,LOOKUP,FOLLOW_LOOKUP_in_lookup3328);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_lookup3330);
expression122=expression();
state._fsp--;
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:468:27: (i= indexes )?
int alt42=2;
int LA42_0 = input.LA(1);
if ( (LA42_0==INDEXES) ) {
alt42=1;
}
switch (alt42) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:468:27: i= indexes
{
pushFollow(FOLLOW_indexes_in_lookup3334);
i=indexes();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = i != null ? new LookupNode(line, handler, currentScope, expression122, i) : expression122;
}
break;
case 8 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:469:6: ^( LOOKUP DottedIdentifier (x= indexes )? )
{
match(input,LOOKUP,FOLLOW_LOOKUP_in_lookup3346);
match(input, Token.DOWN, null);
DottedIdentifier123=(CommonTree)match(input,DottedIdentifier,FOLLOW_DottedIdentifier_in_lookup3348);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:469:33: (x= indexes )?
int alt43=2;
int LA43_0 = input.LA(1);
if ( (LA43_0==INDEXES) ) {
alt43=1;
}
switch (alt43) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:469:33: x= indexes
{
pushFollow(FOLLOW_indexes_in_lookup3352);
x=indexes();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = (x != null)
? new LookupNode(line, handler, currentScope, new IdentifierNode(line, handler, currentScope, (DottedIdentifier123!=null?DottedIdentifier123.getText():null), namespaceStack.asPrefix()), x)
: new IdentifierNode(line, handler, currentScope, (DottedIdentifier123!=null?DottedIdentifier123.getText():null), namespaceStack.asPrefix());
}
break;
case 9 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:475:6: ^( LOOKUP Identifier (x= indexes )? )
{
match(input,LOOKUP,FOLLOW_LOOKUP_in_lookup3370);
match(input, Token.DOWN, null);
Identifier124=(CommonTree)match(input,Identifier,FOLLOW_Identifier_in_lookup3372);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:475:27: (x= indexes )?
int alt44=2;
int LA44_0 = input.LA(1);
if ( (LA44_0==INDEXES) ) {
alt44=1;
}
switch (alt44) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:475:27: x= indexes
{
pushFollow(FOLLOW_indexes_in_lookup3376);
x=indexes();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = (x != null)
? new LookupNode(line, handler, currentScope, new IdentifierNode(line, handler, currentScope, (Identifier124!=null?Identifier124.getText():null), namespaceStack.asPrefix()), x)
: new IdentifierNode(line, handler, currentScope, (Identifier124!=null?Identifier124.getText():null), namespaceStack.asPrefix());
}
break;
case 10 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:481:6: ^( LOOKUP String (x= indexes )? )
{
match(input,LOOKUP,FOLLOW_LOOKUP_in_lookup3394);
match(input, Token.DOWN, null);
String125=(CommonTree)match(input,String,FOLLOW_String_in_lookup3396);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:481:23: (x= indexes )?
int alt45=2;
int LA45_0 = input.LA(1);
if ( (LA45_0==INDEXES) ) {
alt45=1;
}
switch (alt45) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:481:23: x= indexes
{
pushFollow(FOLLOW_indexes_in_lookup3400);
x=indexes();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = (x != null)
? new LookupNode(line, handler, currentScope, new AtomNode(line, handler, currentScope, (String125!=null?String125.getText():null)), x)
: AtomNode.getStringAtom(line, handler,currentScope, (String125!=null?String125.getText():null));
}
break;
case 11 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:487:5: ^( LOOKUP QuotedString (x= indexes )? )
{
match(input,LOOKUP,FOLLOW_LOOKUP_in_lookup3415);
match(input, Token.DOWN, null);
QuotedString126=(CommonTree)match(input,QuotedString,FOLLOW_QuotedString_in_lookup3417);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:487:28: (x= indexes )?
int alt46=2;
int LA46_0 = input.LA(1);
if ( (LA46_0==INDEXES) ) {
alt46=1;
}
switch (alt46) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:487:28: x= indexes
{
pushFollow(FOLLOW_indexes_in_lookup3421);
x=indexes();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
node = (x != null)
? new LookupNode(line, handler, currentScope, new QuotedStringNode(line, handler, currentScope, (QuotedString126!=null?QuotedString126.getText():null)), x)
: new QuotedStringNode(line, handler, currentScope, (QuotedString126!=null?QuotedString126.getText():null));
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return node;
}
// $ANTLR end "lookup"
public static class rangeindex_return extends TreeRuleReturnScope {
public ReflexNode ste;
public ReflexNode ed;
};
// $ANTLR start "rangeindex"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:495:1: rangeindex returns [ReflexNode ste, ReflexNode ed] : ^( RANGEINDEX lhs= expression rhs= expression ) ;
public final ReflexTreeWalker.rangeindex_return rangeindex() throws RecognitionException {
ReflexTreeWalker.rangeindex_return retval = new ReflexTreeWalker.rangeindex_return();
retval.start = input.LT(1);
ReflexNode lhs =null;
ReflexNode rhs =null;
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:496:3: ( ^( RANGEINDEX lhs= expression rhs= expression ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:496:3: ^( RANGEINDEX lhs= expression rhs= expression )
{
match(input,RANGEINDEX,FOLLOW_RANGEINDEX_in_rangeindex3445);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_rangeindex3449);
lhs=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_rangeindex3453);
rhs=expression();
state._fsp--;
match(input, Token.UP, null);
retval.ste = lhs; retval.ed = rhs;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "rangeindex"
// $ANTLR start "indexes"
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:499:1: indexes returns [java.util.List> e] : ^( INDEXES ( exprList )+ ) ;
public final java.util.List> indexes() throws RecognitionException {
java.util.List> e = null;
java.util.List exprList127 =null;
e = new ArrayList>();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:503:3: ( ^( INDEXES ( exprList )+ ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:503:6: ^( INDEXES ( exprList )+ )
{
match(input,INDEXES,FOLLOW_INDEXES_in_indexes3478);
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:503:16: ( exprList )+
int cnt48=0;
loop48:
while (true) {
int alt48=2;
int LA48_0 = input.LA(1);
if ( (LA48_0==EXP_LIST) ) {
alt48=1;
}
switch (alt48) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/Reflex/src/main/antlr3/reflex/ReflexTreeWalker.g:503:17: exprList
{
pushFollow(FOLLOW_exprList_in_indexes3481);
exprList127=exprList();
state._fsp--;
e.add(exprList127);
}
break;
default :
if ( cnt48 >= 1 ) break loop48;
EarlyExitException eee = new EarlyExitException(48, input);
throw eee;
}
cnt48++;
}
match(input, Token.UP, null);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return e;
}
// $ANTLR end "indexes"
// Delegated rules
protected DFA27 dfa27 = new DFA27(this);
static final String DFA27_eotS =
"\127\uffff";
static final String DFA27_eofS =
"\127\uffff";
static final String DFA27_minS =
"\1\101\1\2\2\uffff\1\6\122\uffff";
static final String DFA27_maxS =
"\1\u0096\1\2\2\uffff\1\u00c6\122\uffff";
static final String DFA27_acceptS =
"\2\uffff\1\123\1\124\1\uffff\1\1\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11\1\12"+
"\1\13\1\14\1\15\1\16\1\17\1\20\1\21\1\22\1\23\1\24\1\25\1\26\1\27\1\30"+
"\1\31\1\32\1\33\1\34\1\35\1\36\1\37\1\40\1\41\1\42\1\43\1\44\1\45\1\46"+
"\1\47\1\50\1\51\1\52\1\53\1\54\1\55\1\56\1\57\1\60\1\61\1\62\1\63\1\64"+
"\1\65\1\66\1\67\1\70\1\71\1\72\1\73\1\74\1\75\1\76\1\77\1\100\1\101\1"+
"\102\1\103\1\104\1\105\1\106\1\107\1\110\1\111\1\112\1\113\1\114\1\115"+
"\1\116\1\117\1\120\1\121\1\122";
static final String DFA27_specialS =
"\127\uffff}>";
static final String[] DFA27_transitionS = {
"\1\1\37\uffff\1\2\64\uffff\1\3",
"\1\4",
"",
"",
"\1\21\1\uffff\1\20\1\54\1\27\1\uffff\1\103\1\104\1\uffff\1\105\1\37"+
"\1\40\11\uffff\1\120\1\12\1\114\1\uffff\1\111\1\60\1\36\5\uffff\1\53"+
"\1\uffff\1\42\1\41\1\uffff\1\126\1\55\1\71\4\uffff\1\23\7\uffff\1\44"+
"\6\uffff\1\52\1\16\1\17\1\uffff\1\63\1\76\2\uffff\1\122\1\123\1\70\1"+
"\11\1\10\1\13\7\uffff\1\5\5\uffff\1\50\1\51\1\72\1\74\4\uffff\1\34\4"+
"\uffff\1\117\2\uffff\1\75\2\uffff\1\15\1\113\1\62\1\64\1\66\1\47\4\uffff"+
"\1\121\17\uffff\1\6\1\uffff\1\56\2\uffff\1\14\1\7\3\uffff\1\67\7\uffff"+
"\1\31\1\32\1\115\1\46\1\102\1\65\1\uffff\1\116\4\uffff\1\110\1\30\1\112"+
"\1\35\1\uffff\1\125\1\25\1\24\3\uffff\1\57\1\106\1\uffff\1\22\1\124\1"+
"\uffff\1\43\1\61\1\uffff\1\33\1\uffff\1\26\1\uffff\1\73\1\100\1\77\1"+
"\uffff\1\101\1\45\1\107",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA27_eot = DFA.unpackEncodedString(DFA27_eotS);
static final short[] DFA27_eof = DFA.unpackEncodedString(DFA27_eofS);
static final char[] DFA27_min = DFA.unpackEncodedStringToUnsignedChars(DFA27_minS);
static final char[] DFA27_max = DFA.unpackEncodedStringToUnsignedChars(DFA27_maxS);
static final short[] DFA27_accept = DFA.unpackEncodedString(DFA27_acceptS);
static final short[] DFA27_special = DFA.unpackEncodedString(DFA27_specialS);
static final short[][] DFA27_transition;
static {
int numStates = DFA27_transitionS.length;
DFA27_transition = new short[numStates][];
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy