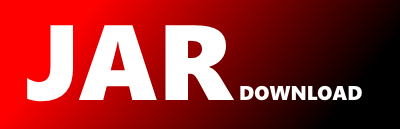
reflex.value.ReflexValue Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (c) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package reflex.value;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.math.MathContext;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonIgnore;
import rapture.common.impl.jackson.JacksonUtil;
import reflex.ReflexException;
public class ReflexValue implements Comparable {
private Object value;
private ReflexValueType valueType;
String NOTNULL = "Argument to ReflexValue cannot be null. Use ReflexNullValue";
public enum Internal {
NULL,
VOID,
BREAK,
CONTINUE,
SUSPEND
}
public Object getValue() {
return value;
}
public void setValue(Object value) {
if (value instanceof List>) {
value = ensureReflexValueList((List>) value);
}
this.value = value;
setTypeBasedOnValue();
}
private Object ensureReflexValueList(List> value) {
List ret = new ArrayList(value.size());
for (Object x : value) {
if (x instanceof ReflexValue) {
ret.add((ReflexValue) x);
} else {
ret.add(new ReflexValue(x));
}
}
return ret;
}
private void setTypeBasedOnValue() {
if (isInternal()) {
valueType = ReflexValueType.INTERNAL;
} else if (value instanceof String) {
valueType = ReflexValueType.STRING;
} else if ((value instanceof Integer) || (value instanceof Long) || (value instanceof BigInteger)) {
valueType = ReflexValueType.INTEGER;
} else if (value instanceof Number) {
valueType = ReflexValueType.NUMBER;
} else if (value instanceof Map, ?>) {
valueType = ReflexValueType.MAP;
} else if (value instanceof Boolean) {
valueType = ReflexValueType.BOOLEAN;
} else if (value instanceof List>) {
valueType = ReflexValueType.LIST;
} else if (value instanceof ReflexDateValue) {
valueType = ReflexValueType.DATE;
} else if (value instanceof ReflexArchiveFileValue) {
valueType = ReflexValueType.ARCHIVE;
} else if (value instanceof ReflexFileValue) {
valueType = ReflexValueType.FILE;
} else if (value instanceof ReflexStringStreamValue) {
valueType = ReflexValueType.STRINGSTREAM;
} else if (value instanceof ReflexStreamValue) {
valueType = ReflexValueType.STREAM;
} else if (value instanceof ReflexLibValue) {
valueType = ReflexValueType.LIB;
} else if (value instanceof ReflexPortValue) {
valueType = ReflexValueType.PORT;
} else if (value instanceof ReflexProcessValue) {
valueType = ReflexValueType.PROCESS;
} else if (value instanceof ReflexTimeValue) {
valueType = ReflexValueType.TIME;
} else if (value instanceof ReflexTimerValue) {
valueType = ReflexValueType.TIMER;
} else if (value instanceof ReflexSparseMatrixValue) {
valueType = ReflexValueType.SPARSEMATRIX;
} else if (value instanceof ReflexByteArrayValue) {
valueType = ReflexValueType.BYTEARRAY;
} else if (value instanceof ReflexMimeValue) {
valueType = ReflexValueType.MIME;
} else if (value instanceof ReflexStructValue) {
valueType = ReflexValueType.STRUCT;
} else if (value instanceof Object[]) {
// maybe we should have an Array type?
Object[] array = (Object[]) value;
List list = new ArrayList();
for (Object obj : array)
list.add(new ReflexValue(obj));
value = list;
valueType = ReflexValueType.LIST;
} else {
valueType = ReflexValueType.COMPLEX;
}
}
private boolean isInternal() {
return false;
}
private boolean isReturn = false;
public ReflexValue(int lineNumber, List v) {
if (v == null) {
throw new ReflexException(lineNumber, NOTNULL);
} else {
setValue(v);
}
}
public ReflexValue(int lineNumber, Object v) {
if (v == null) {
throw new ReflexException(lineNumber, NOTNULL);
} else if (v instanceof ReflexValue) {
// If we're including a value in a value, just include the value. If
// that makes sense.
setValue(((ReflexValue) v).value);
} else {
setValue(v);
}
}
public ReflexValue(List v) {
this(-1, v);
}
public ReflexValue(Object v) {
this(-1, v);
}
// Only used by serializers
public ReflexValue() {
}
private void standardThrow(ReflexValueType expectedType) throws ReflexException {
String message = String.format("Expected %s, have %s", expectedType.toString(), valueType.toString());
throw new ReflexException(-1, message);
}
public Boolean asBoolean() {
switch (valueType) {
case BOOLEAN:
return (Boolean) value;
default:
standardThrow(ReflexValueType.BOOLEAN);
}
return null;
}
public byte[] asByteArray() {
switch (valueType) {
case BYTEARRAY:
return ((ReflexByteArrayValue) value).getBytes();
case STRING:
return value.toString().getBytes();
case MIME:
return ((ReflexMimeValue) value).getData();
default:
standardThrow(ReflexValueType.BYTEARRAY);
}
return null;
}
public ReflexDateValue asDate() {
switch (valueType) {
case DATE:
return (ReflexDateValue) value;
default:
standardThrow(ReflexValueType.DATE);
}
return null;
}
public Double asDouble() {
switch (valueType) {
case INTEGER:
case NUMBER:
return ((Number) value).doubleValue();
case STRING:
return Double.valueOf(value.toString());
default:
standardThrow(ReflexValueType.NUMBER);
}
return null;
}
public BigDecimal asBigDecimal() {
if (value instanceof BigDecimal) return (BigDecimal) value;
switch (valueType) {
case INTEGER:
return new BigDecimal(((Number) value).longValue());
case NUMBER:
return new BigDecimal(((Number) value).doubleValue(), MathContext.DECIMAL64);
case STRING:
return new BigDecimal(value.toString());
default:
standardThrow(ReflexValueType.NUMBER);
}
return null;
}
public Float asFloat() {
switch (valueType) {
case NUMBER:
case INTEGER:
return ((Number) value).floatValue();
default:
standardThrow(ReflexValueType.NUMBER);
}
return null;
}
public ReflexStreamValue asStream() {
switch (valueType) {
case STREAM:
return (ReflexStreamValue) value;
case FILE:
return (ReflexFileValue) value;
case STRINGSTREAM:
return (ReflexStringStreamValue) value;
default:
return null;
}
}
public ReflexSparseMatrixValue asMatrix() {
switch (valueType) {
case SPARSEMATRIX:
return (ReflexSparseMatrixValue) value;
default:
standardThrow(ReflexValueType.SPARSEMATRIX);
}
return null;
}
public ReflexFileValue asFile() {
switch (valueType) {
case FILE:
return (ReflexFileValue) value;
default:
return null;
}
}
public ReflexArchiveFileValue asArchive() {
switch (valueType) {
case ARCHIVE:
return (ReflexArchiveFileValue) value;
default:
return null;
}
}
public Integer asInt() {
switch (valueType) {
case INTEGER:
case NUMBER:
return ((Number) value).intValue();
case STRING:
return Integer.valueOf(value.toString());
default:
return 0;
}
}
public ReflexLibValue asLib() {
switch (valueType) {
case LIB:
return (ReflexLibValue) value;
default:
return null;
}
}
@SuppressWarnings("unchecked")
public List asList() {
if (valueType != ReflexValueType.LIST) return null;
// It's possible for objects other than ReflexValues to get into the list.
List retList = (List) value;
boolean isPure = true;
for (Object o : retList) {
if (!(o instanceof ReflexValue)) {
isPure = false;
break;
}
}
if (!isPure) {
retList = new ArrayList<>();
for (Object o : (List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy