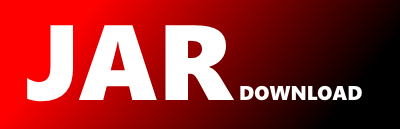
net.rationalminds.util.TextFileUtility Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of DateParser Show documentation
Show all versions of DateParser Show documentation
The maven main core project description
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy