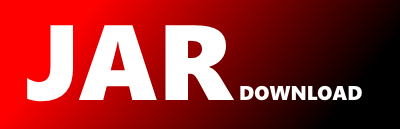
org.fxconnector.helper.SubWindowChecker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scenic-view Show documentation
Show all versions of scenic-view Show documentation
Scenic View is a JavaFX application designed to make it simple to understand the current state of your application scenegraph, and to also easily manipulate properties of the scenegraph without having to keep editing your code. This lets you find bugs, and get things pixel perfect without having to do the compile-check-compile dance.
/*
* Scenic View,
* Copyright (C) 2012 Jonathan Giles, Ander Ruiz, Amy Fowler
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.fxconnector.helper;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import javafx.application.Platform;
import javafx.stage.PopupWindow;
import javafx.stage.Window;
import org.fxconnector.StageControllerImpl;
public class SubWindowChecker extends WindowChecker {
StageControllerImpl model;
public SubWindowChecker(final StageControllerImpl model) {
super(new WindowFilter() {
@Override public boolean accept(final Window window) {
return window instanceof PopupWindow;
}
}, model.getID().toString());
this.model = model;
}
Map previousTree = new HashMap<>();
List windows = new ArrayList<>();
final Map tree = new HashMap<>();
@Override protected void onWindowsFound(final List tempPopups) {
tree.clear();
windows.clear();
for (final Window popupWindow : tempPopups) {
final Map pos = valid((PopupWindow) popupWindow, tree);
if (pos != null) {
pos.put((PopupWindow) popupWindow, new HashMap());
windows.add((PopupWindow) popupWindow);
}
}
if (!tree.equals(previousTree)) {
previousTree.clear();
previousTree.putAll(tree);
final List actualWindows = new ArrayList<>(windows);
Platform.runLater(new Runnable() {
@Override public void run() {
// No need for synchronization here
model.popupWindows.clear();
model.popupWindows.addAll(actualWindows);
model.update();
}
});
}
}
@SuppressWarnings("unchecked") Map valid(final PopupWindow window, final Map tree) {
if (window.getOwnerWindow() == model.targetWindow)
return tree;
for (final Iterator iterator = tree.keySet().iterator(); iterator.hasNext();) {
final PopupWindow type = iterator.next();
if (type == window.getOwnerWindow()) {
return tree.get(type);
} else {
final Map lower = valid(window, tree.get(type));
if (lower != null)
return lower;
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy