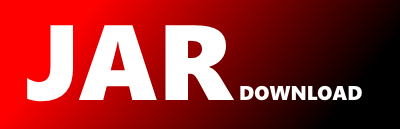
net.ravendb.client.http.ClusterTopology Maven / Gradle / Ivy
package net.ravendb.client.http;
import java.util.HashMap;
import java.util.Map;
public class ClusterTopology {
private String lastNodeId;
private String topologyId;
private long etag;
private Map members;
private Map promotables;
private Map watchers;
public boolean contains(String node) {
if (members != null && members.containsKey(node)) {
return true;
}
if (promotables != null && promotables.containsKey(node)) {
return true;
}
return watchers != null && watchers.containsKey(node);
}
public String getUrlFromTag(String tag) {
if (tag == null) {
return null;
}
if (members != null && members.containsKey(tag)) {
return members.get(tag);
}
if (promotables != null && promotables.containsKey(tag)) {
return promotables.get(tag);
}
if (watchers != null && watchers.containsKey(tag)) {
return watchers.get(tag);
}
return null;
}
public Map getAllNodes() {
Map result = new HashMap<>();
if (members != null) {
for (Map.Entry entry : members.entrySet()) {
result.put(entry.getKey(), entry.getValue());
}
}
if (promotables != null) {
for (Map.Entry entry : promotables.entrySet()) {
result.put(entry.getKey(), entry.getValue());
}
}
if (watchers != null) {
for (Map.Entry entry : watchers.entrySet()) {
result.put(entry.getKey(), entry.getValue());
}
}
return result;
}
public String getLastNodeId() {
return lastNodeId;
}
public void setLastNodeId(String lastNodeId) {
this.lastNodeId = lastNodeId;
}
public String getTopologyId() {
return topologyId;
}
public void setTopologyId(String topologyId) {
this.topologyId = topologyId;
}
public Map getMembers() {
return members;
}
public void setMembers(Map members) {
this.members = members;
}
public Map getPromotables() {
return promotables;
}
public void setPromotables(Map promotables) {
this.promotables = promotables;
}
public Map getWatchers() {
return watchers;
}
public void setWatchers(Map watchers) {
this.watchers = watchers;
}
public long getEtag() {
return etag;
}
public void setEtag(long etag) {
this.etag = etag;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy