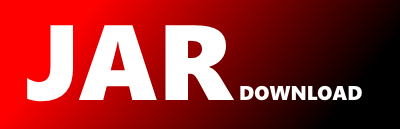
net.ravendb.client.documents.changes.IDatabaseChanges Maven / Gradle / Ivy
package net.ravendb.client.documents.changes;
public interface IDatabaseChanges extends IConnectableChanges {
/**
* Subscribe to changes for specified index only.
* @param indexName The index name
* @return Changes observable
*/
IChangesObservable forIndex(String indexName);
/**
* Subscribe to changes for specified document only.
* @param docId Document identifier
* @return Changes observable
*/
IChangesObservable forDocument(String docId);
/**
* Subscribe to changes for all documents.
* @return Changes observable
*/
IChangesObservable forAllDocuments();
/**
* Subscribe to changes for specified operation only.
* @param operationId Operation id
* @return Changes observable
*/
IChangesObservable forOperationId(long operationId);
/**
* Subscribe to change for all operation statuses.
* @return Changes observable
*/
IChangesObservable forAllOperations();
/**
* Subscribe to changes for all indexes.
* @return Changes observable
*/
IChangesObservable forAllIndexes();
/**
* Subscribe to changes for all documents that Id starts with given prefix.
* @param docIdPrefix The document prefix
* @return Changes observable
*/
IChangesObservable forDocumentsStartingWith(String docIdPrefix);
/**
* Subscribe to changes for all documents that belong to specified collection (Raven-Entity-Name).
* @param collectionName The collection name.
* @return Changes observable
*/
IChangesObservable forDocumentsInCollection(String collectionName);
/**
* Subscribe to changes for all documents that belong to specified collection (Raven-Entity-Name).
* @param clazz The document class
* @return Changes observable
*/
IChangesObservable forDocumentsInCollection(Class> clazz);
/**
* Subscribe for changes for all counters.
* @return Changes observable
*/
IChangesObservable forAllCounters();
/**
* Subscribe to changes for all counters with a given name.
* @param counterName Counter name
* @return Changes observable
*/
IChangesObservable forCounter(String counterName);
/**
* Subscribe to changes for counter from a given document and with given name.
* @param documentId Document identifier
* @param counterName Counter name
* @return Changes observable
*/
IChangesObservable forCounterOfDocument(String documentId, String counterName);
/**
* Subscribe to changes for all counters from a given document.
* @param documentId Document identifier
* @return Changes observable
*/
IChangesObservable forCountersOfDocument(String documentId);
/**
* Subscribe to changes for all timeseries.
* @return Changes observable
*/
IChangesObservable forAllTimeSeries();
/**
* Subscribe to changes for all timeseries with a given name.
* @param timeSeriesName Time series name
* @return Changes observable
*/
IChangesObservable forTimeSeries(String timeSeriesName);
/**
* Subscribe to changes for timeseries from a given document and with given name.
* @param documentId Document identifier
* @param timeSeriesName Time series name
* @return Changes observable
*/
IChangesObservable forTimeSeriesOfDocument(String documentId, String timeSeriesName);
/**
* Subscribe to changes for timeseries from a given document and with given name.
* @param documentId Document identifier
* @return Changes observable
*/
IChangesObservable forTimeSeriesOfDocument(String documentId);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy