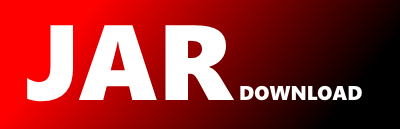
net.ravendb.client.documents.indexes.AbstractIndexDefinitionBuilder Maven / Gradle / Ivy
package net.ravendb.client.documents.indexes;
import net.ravendb.client.documents.conventions.DocumentConventions;
import net.ravendb.client.documents.indexes.spatial.SpatialOptions;
import net.ravendb.client.exceptions.documents.compilation.IndexCompilationException;
import org.apache.commons.lang3.ObjectUtils;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.function.BiConsumer;
public abstract class AbstractIndexDefinitionBuilder {
protected final String _indexName;
private String reduce;
private Map storesStrings;
private Map indexesStrings;
private Map analyzersStrings;
private Set suggestionsOptions;
private Map termVectorsStrings;
private Map spatialIndexesStrings;
private IndexLockMode lockMode;
private IndexPriority priority;
private IndexState state;
private IndexDeploymentMode deploymentMode;
private String outputReduceToCollection;
private String patternForOutputReduceToCollectionReferences;
private String patternReferencesCollectionName;
private Map additionalSources;
private Set additionalAssemblies;
private IndexConfiguration configuration;
protected AbstractIndexDefinitionBuilder(String indexName) {
_indexName = ObjectUtils.firstNonNull(indexName, getClass().getSimpleName());
if (_indexName.length() > 256) {
throw new IllegalArgumentException("The index name is limited to 256 characters, but was: " + _indexName);
}
storesStrings = new HashMap<>();
indexesStrings = new HashMap<>();
suggestionsOptions = new HashSet<>();
analyzersStrings = new HashMap<>();
termVectorsStrings = new HashMap<>();
spatialIndexesStrings = new HashMap<>();
configuration = new IndexConfiguration();
}
public TIndexDefinition toIndexDefinition(DocumentConventions conventions) {
return toIndexDefinition(conventions, true);
}
public TIndexDefinition toIndexDefinition(DocumentConventions conventions, boolean validateMap) {
try {
TIndexDefinition indexDefinition = newIndexDefinition();
indexDefinition.setName(_indexName);
indexDefinition.setReduce(reduce);
indexDefinition.setLockMode(lockMode);
indexDefinition.setPriority(priority);
indexDefinition.setDeploymentMode(deploymentMode);
indexDefinition.setState(state);
indexDefinition.setOutputReduceToCollection(outputReduceToCollection);
indexDefinition.setPatternForOutputReduceToCollectionReferences(patternForOutputReduceToCollectionReferences);
indexDefinition.setPatternReferencesCollectionName(patternReferencesCollectionName);
Map suggestions = new HashMap<>();
for (String suggestionsOption : suggestionsOptions) {
suggestions.put(suggestionsOption, true);
}
applyValues(indexDefinition, indexesStrings, (options, value) -> options.setIndexing(value));
applyValues(indexDefinition, storesStrings, (options, value) -> options.setStorage(value));
applyValues(indexDefinition, analyzersStrings, (options, value) -> options.setAnalyzer(value));
applyValues(indexDefinition, termVectorsStrings, (options, value) -> options.setTermVector(value));
applyValues(indexDefinition, spatialIndexesStrings, (options, value) -> options.setSpatial(value));
applyValues(indexDefinition, suggestions, (options, value) -> options.setSuggestions(value));
indexDefinition.setAdditionalSources(additionalSources);
indexDefinition.setAdditionalAssemblies(additionalAssemblies);
indexDefinition.setConfiguration(configuration);
toIndexDefinition(indexDefinition, conventions);
return indexDefinition;
} catch (Exception e) {
throw new IndexCompilationException("Failed to create index " + _indexName, e);
}
}
protected abstract TIndexDefinition newIndexDefinition();
protected abstract void toIndexDefinition(TIndexDefinition indexDefinition, DocumentConventions conventions);
private void applyValues(IndexDefinition indexDefinition, Map values, BiConsumer action) {
for (Map.Entry kvp : values.entrySet()) {
IndexFieldOptions field = indexDefinition.getFields().computeIfAbsent(kvp.getKey(), x -> new IndexFieldOptions());
action.accept(field, kvp.getValue());
}
}
public String getReduce() {
return reduce;
}
public void setReduce(String reduce) {
this.reduce = reduce;
}
public Map getStoresStrings() {
return storesStrings;
}
public void setStoresStrings(Map storesStrings) {
this.storesStrings = storesStrings;
}
public Map getIndexesStrings() {
return indexesStrings;
}
public void setIndexesStrings(Map indexesStrings) {
this.indexesStrings = indexesStrings;
}
public Map getAnalyzersStrings() {
return analyzersStrings;
}
public void setAnalyzersStrings(Map analyzersStrings) {
this.analyzersStrings = analyzersStrings;
}
public Set getSuggestionsOptions() {
return suggestionsOptions;
}
public void setSuggestionsOptions(Set suggestionsOptions) {
this.suggestionsOptions = suggestionsOptions;
}
public Map getTermVectorsStrings() {
return termVectorsStrings;
}
public void setTermVectorsStrings(Map termVectorsStrings) {
this.termVectorsStrings = termVectorsStrings;
}
public Map getSpatialIndexesStrings() {
return spatialIndexesStrings;
}
public void setSpatialIndexesStrings(Map spatialIndexesStrings) {
this.spatialIndexesStrings = spatialIndexesStrings;
}
public IndexLockMode getLockMode() {
return lockMode;
}
public void setLockMode(IndexLockMode lockMode) {
this.lockMode = lockMode;
}
public IndexPriority getPriority() {
return priority;
}
public void setPriority(IndexPriority priority) {
this.priority = priority;
}
public IndexState getState() {
return state;
}
public void setState(IndexState state) {
this.state = state;
}
public IndexDeploymentMode getDeploymentMode() {
return deploymentMode;
}
public void setDeploymentMode(IndexDeploymentMode deploymentMode) {
this.deploymentMode = deploymentMode;
}
public String getOutputReduceToCollection() {
return outputReduceToCollection;
}
public void setOutputReduceToCollection(String outputReduceToCollection) {
this.outputReduceToCollection = outputReduceToCollection;
}
public String getPatternForOutputReduceToCollectionReferences() {
return patternForOutputReduceToCollectionReferences;
}
public void setPatternForOutputReduceToCollectionReferences(String patternForOutputReduceToCollectionReferences) {
this.patternForOutputReduceToCollectionReferences = patternForOutputReduceToCollectionReferences;
}
public String getPatternReferencesCollectionName() {
return patternReferencesCollectionName;
}
public void setPatternReferencesCollectionName(String patternReferencesCollectionName) {
this.patternReferencesCollectionName = patternReferencesCollectionName;
}
public Map getAdditionalSources() {
return additionalSources;
}
public void setAdditionalSources(Map additionalSources) {
this.additionalSources = additionalSources;
}
public Set getAdditionalAssemblies() {
return additionalAssemblies;
}
public void setAdditionalAssemblies(Set additionalAssemblies) {
this.additionalAssemblies = additionalAssemblies;
}
public IndexConfiguration getConfiguration() {
return configuration;
}
public void setConfiguration(IndexConfiguration configuration) {
this.configuration = configuration;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy