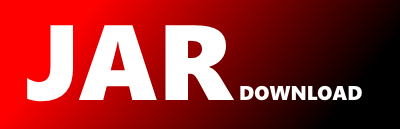
net.razorvine.pyro.serializer.PyroProxySerpent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pyrolite Show documentation
Show all versions of pyrolite Show documentation
This library allows your Java program to interface very easily with the Python world. It uses the Pyro protocol to call methods on remote objects. (See https://pyro5.readthedocs.io/).
Pyrolite only implements part of the client side Pyro library, hence its name 'lite'... So if you don't need Pyro's full feature set, Pyrolite may be a good choice to connect java or .NET and python.
Version 5.0 changes: support Pyro5 wire protocol. Dropped support of Pyro4 (stick to version 4.xx for that).
package net.razorvine.pyro.serializer;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import javax.xml.bind.DatatypeConverter;
import net.razorvine.pyro.PyroException;
import net.razorvine.pyro.PyroProxy;
import net.razorvine.pyro.PyroURI;
import net.razorvine.serpent.IClassSerializer;
/**
* Serpent extension to be able to serialize PyroProxy objects with Serpent.
*
* @author Irmen de Jong ([email protected])
*/
public class PyroProxySerpent implements IClassSerializer {
public static Object FromSerpentDict(Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy