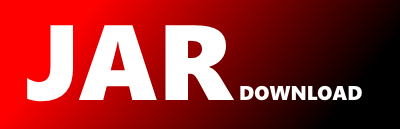
redora.client.ui.text.RichTextArea Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2009-2010 Nanjing RedOrange ltd (http://www.red-orange.cn)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package redora.client.ui.text;
import com.google.gwt.core.client.GWT;
import com.google.gwt.dom.client.Style;
import com.google.gwt.event.logical.shared.ValueChangeEvent;
import com.google.gwt.event.logical.shared.ValueChangeHandler;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.user.client.DOM;
import com.google.gwt.user.client.ui.*;
import redora.client.ui.RedoraResource;
/**
* RichTextArea in GWT.
*
* @author Nanjing RedOrange (http://www.red-orange.cn)
*/
public class RichTextArea extends Composite implements HasValue, HasName {
static RedoraResource resources = GWT.create(RedoraResource.class);
final com.google.gwt.user.client.ui.RichTextArea rta;
final RichTextToolbar toolbar;
public RichTextArea(String style, String id) {
rta = new com.google.gwt.user.client.ui.RichTextArea();
toolbar = new RichTextToolbar(rta);
rta.addStyleName(resources.css().redoraTableInput());
toolbar.addStyleName(resources.css().redoraTableInput());
final Grid grid = new Grid(2, 1);
VerticalPanel panel = new VerticalPanel();
panel.add(toolbar);
//panel.setWidgetLeftWidth(toolbar, 0, Style.Unit.PX, 100, Style.Unit.PCT);
//panel.setWidgetTopHeight(toolbar, 0, Style.Unit.PX, 20, Style.Unit.PCT);
panel.add(rta);
//panel.setWidgetLeftWidth(rta, 0, Style.Unit.PX, 100, Style.Unit.PCT);
//panel.setWidgetTopHeight(rta, 20, Style.Unit.PCT, 80, Style.Unit.PCT);
//grid.setWidget(0, 0, toolbar);
//grid.setWidget(1, 0, rta);
this.initWidget(panel);
getElement().setId(id);
addStyleName(style);
addStyleName(resources.css().redoraHtml());
}
public String getValue() {
return rta.getHTML(); //TODO getText()?
}
public void setValue(String value) {
setValue(value, false);
}
public void setHTML(String html) {
rta.setHTML(html);
}
public String getHTML() {
return rta.getHTML();
}
public RichTextToolbar getToolbar() {
return toolbar;
}
public void setValue(String value, boolean fireEvents) {
String oldValue = rta.getText();
rta.setHTML(value);
if (fireEvents) {
ValueChangeEvent.fireIfNotEqual(this, oldValue, value);
}
}
public HandlerRegistration addValueChangeHandler(
ValueChangeHandler handler) {
return addHandler(handler, ValueChangeEvent.getType());
}
public String getName() {
return DOM.getElementProperty(getElement(), "name");
}
public void setName(String name) {
DOM.setElementProperty(getElement(), "name", name);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy