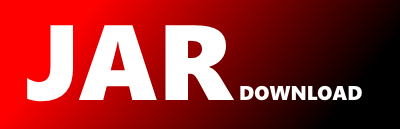
redora.configuration.rdo.service.check.RedoraTrashTableCheck Maven / Gradle / Ivy
/*
* DO NOT CHANGE THIS FILE. Changes will be overwritten.
* -----------------------------------------------------
* This file is generated by Redora (www.redora.net) a source code generator.
* Copyright to this file belongs to You: the person or organization who has
* used Redora to generate this file.
* Redora is released under the open source Apache License, but this generated code
* can be released under any license or stay unreleased, as wished by the copyright
* owner.
*
* Unless required by applicable law or agreed to in writing, this software
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND
* , either express or implied.
*/
package redora.configuration.rdo.service.check;
import org.jetbrains.annotations.NotNull;
import java.sql.ResultSet;
import java.util.HashMap;
import java.util.Map;
import java.sql.SQLException;
import java.util.logging.Logger;
import java.io.PrintWriter;
import redora.db.Statement;
import redora.configuration.rdo.model.fields.RedoraTrashFields;
import redora.exceptions.ConnectException;
import redora.exceptions.QueryException;
import static java.util.logging.Level.SEVERE;
import static redora.service.ServiceBase.close;
/**
* Handler for the model vs database synchronization. It can create the RedoraTrash table if it does exists
* and it can check if the database definition is still in sync with your model with the check() function.
* For your convenience is can also drop the RedoraTrash table.
* @author Redora (www.redora.net)
*/
public class RedoraTrashTableCheck {
private static final transient Logger l = Logger.getLogger("redora.configuration.rdo.service.check.RedoraTrashTableCheck");
private final Statement st;
public RedoraTrashTableCheck(Statement st) {
this.st = st;
}
/**
* Checks if the model is the same with the database table, logs warning level message
* if this is not the case.
* @param out HTML stream with check results. If the check results OK, nothing is streamed
*/
public void check(@NotNull PrintWriter out) throws ConnectException, QueryException {
ResultSet rs = null;
try {
rs = st.con.con.getMetaData().getColumns("", "", "RedoraTrash", "");
Map> map = new HashMap>();
while (rs.next()) {
Map list = new HashMap();
list.put("COLUMN_NAME", rs.getString("COLUMN_NAME"));
list.put("DATA_TYPE", rs.getInt("DATA_TYPE"));
list.put("COLUMN_SIZE", rs.getInt("COLUMN_SIZE"));
list.put("IS_NULLABLE", rs.getString("IS_NULLABLE"));
map.put(rs.getString("COLUMN_NAME"), list);
}
for (String key : map.keySet()) {
boolean check = false;
for (RedoraTrashFields fields : RedoraTrashFields.values()) {
if (map.get(key).get("COLUMN_NAME").toString().equalsIgnoreCase(fields.name())) {
check = true;
break;
}
}
if (!check) {
out.print("RedoraTrash does not have " + key + " attribute but it exists in database.
");
}
}
for (RedoraTrashFields fields : RedoraTrashFields.values()) {
if (fields.name().equalsIgnoreCase("creationDate")) {
fields.sqlType = java.sql.Types.TIMESTAMP;
}
if (fields.name().equalsIgnoreCase("updateDate")) {
fields.sqlType = java.sql.Types.TIMESTAMP;
}
if (fields.sqlType == java.sql.Types.BOOLEAN) {
fields.sqlType = java.sql.Types.BIT;
}
if (map.containsKey(fields.name()) && Integer.parseInt(map.get(fields.name()).get("DATA_TYPE").toString()) == fields.sqlType) {
if (fields.name().equalsIgnoreCase("objectId")) {
if (!map.get(fields.name()).get("IS_NULLABLE").toString().equalsIgnoreCase("NO")) {
out.print("RedoraTrash." + fields.name()+ " can't have null values.
");
}
}
if (fields.name().equalsIgnoreCase("undoHash")) {
if (!map.get(fields.name()).get("IS_NULLABLE").toString().equalsIgnoreCase("NO")) {
out.print("RedoraTrash." + fields.name()+ " can't have null values.
");
}
if (Integer.parseInt(map.get(fields.name()).get("COLUMN_SIZE").toString()) != 40) {
out.print("RedoraTrash." + fields.name()+ "'s maxlength do not match the database's.
");
}
}
if (fields.name().equalsIgnoreCase("objectName")) {
if (!map.get(fields.name()).get("IS_NULLABLE").toString().equalsIgnoreCase("NO")) {
out.print("RedoraTrash." + fields.name()+ " can't have null values.
");
}
if (Integer.parseInt(map.get(fields.name()).get("COLUMN_SIZE").toString()) != 255) {
out.print("RedoraTrash." + fields.name()+ "'s maxlength do not match the database's.
");
}
}
if (fields.name().equalsIgnoreCase("userId")) {
if (!map.get(fields.name()).get("IS_NULLABLE").toString().equalsIgnoreCase("YES")) {
out.print("RedoraTrash." + fields.name()+ " can have null values.
");
}
}
} else {
out.print("RedoraTrash." + fields.name() + " does not exist or its sqltype does not match according to xml.
");
}
}
} catch (SQLException e) {
l.log(SEVERE, "Failed to execute check RedoraTrash", e);
throw new QueryException("failed to execute check RedoraTrash", e);
} finally {
close(rs);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy