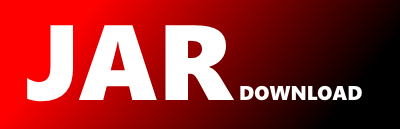
redora.configuration.rdo.sql.base.RedoraConfigurationSQLBase Maven / Gradle / Ivy
The newest version!
/*
* DO NOT CHANGE THIS FILE. Changes will be overwritten.
* -----------------------------------------------------
* This file is generated by Redora (www.redora.net) a source code generator.
* Copyright to this file belongs to You: the person or organization who has
* used Redora to generate this file.
* Redora is released under the open source Apache License, but this generated code
* can be released under any license or stay unreleased, as wished by the copyright
* owner.
*
* Unless required by applicable law or agreed to in writing, this software
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND
* , either express or implied.
*/
package redora.configuration.rdo.sql.base;
/**
* All the generated SQL statements for RedoraConfiguration go in here.
* If you have custom queries, or you want to override existing ones,
* best put them in here redora.configuration.rdo.service.RedoraConfiguration.
* @author Redora (www.redora.net)
* @see redora.configuration.rdo.sql.RedoraConfigurationSQL
*/
public class RedoraConfigurationSQLBase {
/**
* Sequence of this model in the list of all models. This number is used
* to create an unique alias for select queries and is can also be used to
* get the table name from redora.configuration.rdo.service.RedoraConfigurationService.OBJECTS[].
*
* Do not use the number hard coded, it can change when the model changes. Reference this
* or ALIAS instead.
*/
public final static int SEQUENCE = 1;
/** Table alias used for select queries.*/
public final static String ALIAS = "o" + SEQUENCE;
/**
* The generated finder methods are added to this enum. Also the GWT client has
* the same DefaultFinder, ordered in exactly the same manner. Therefor it is
* possible to use the enum's ordinal position:
*
* int ord = GwtClientObject.DefaultFinder.FindAll.ordinal();
* if (ClientObjectSQL.DefaultFinder.values()[ord].name().equals("FindAll") {
* System.out.print("Hello World");
* }
*
*/
public enum DefaultFinder {
FindAll(FIND_ALL_LIST, FIND_ALL_TABLE, false)
, FindByScriptName(FIND_BY_SCRIPT_NAME_ID_LIST, FIND_BY_SCRIPT_NAME_ID_TABLE, false)
, FindByStatus(FIND_BY__STATUS_LIST, FIND_BY__STATUS_TABLE, false)
;
public final String sqlTable;
public final String sqlList;
public boolean allowJson;
DefaultFinder(String sqlList, String sqlTable, boolean allowJson) {
this.sqlTable = sqlTable;
this.sqlList = sqlList;
this.allowJson = allowJson;
}
}
/** All the fields that are usually needed in the drop list as defined in the model.*/
public final static String LIST_FIELDS = "o1.id"
+ ",o1.status"
;
/** The most important fields used for a tabular layout.*/
public final static String TABLE_FIELDS = "o1.id"
+ ",o1.scriptName"
+ ",o1.status"
+ ",o1.creationDate,o1.updateDate"
;
/** All the fields except the related children.*/
public final static String FORM_FIELDS = ALIAS + ".id," +
ALIAS + ".scriptName," +
ALIAS + ".status," +
ALIAS + ".output," +
ALIAS + ".creationDate," + ALIAS + ".updateDate";
/** All the fields that are lazy loading as defined in the model.*/
public final static String LAZY_FIELDS = ALIAS + ".id," +
ALIAS + ".output," +
ALIAS + ".creationDate," + ALIAS + ".updateDate";
/** delete from RedoraConfiguration where id = ? */
public final static String DELETE = "delete from `RedoraConfiguration` where id = ?";
/** default order for query */
public final static String DEFAULT_ORDER = " order by "
+ ALIAS + ".status"
;
public final static String FIND_BY_ID_FORM =
"select " + FORM_FIELDS + " from `RedoraConfiguration` as " + ALIAS + " where " + ALIAS + ".id = ? ";
public final static String FIND_BY_ID_TABLE =
"select " + TABLE_FIELDS + " from `RedoraConfiguration` as " + ALIAS + " where " + ALIAS + ".id = ? ";
public final static String FIND_BY_ID_LIST =
"select " + LIST_FIELDS + " from `RedoraConfiguration` as " + ALIAS + " where " + ALIAS + ".id = ? ";
public final static String FIND_IN_ID_FORM =
"select " + FORM_FIELDS + " from `RedoraConfiguration` as " + ALIAS + " where " + ALIAS + ".id in (?) ";
public final static String FIND_IN_ID_TABLE =
"select " + TABLE_FIELDS + " from `RedoraConfiguration` as " + ALIAS + " where " + ALIAS + ".id in (?) ";
public final static String FIND_IN_ID_LIST =
"select " + LIST_FIELDS + " from `RedoraConfiguration` as " + ALIAS + " where " + ALIAS + ".id in (?) ";
public final static String FIND_ALL_TABLE =
"select " + TABLE_FIELDS + " from `RedoraConfiguration` as " + ALIAS + " " + DEFAULT_ORDER;
public final static String FIND_ALL_LIST =
"select " + LIST_FIELDS + " from `RedoraConfiguration` as " + ALIAS + " " + DEFAULT_ORDER;
public static final String FIND_BY_SCRIPT_NAME_ID_LIST =
"select " + LIST_FIELDS + " from `RedoraConfiguration` as " + ALIAS + " where " + ALIAS + ".scriptName = ?" + DEFAULT_ORDER;
public static final String FIND_BY_SCRIPT_NAME_ID_TABLE =
"select " + TABLE_FIELDS + " from `RedoraConfiguration` as " + ALIAS + " where " + ALIAS + ".scriptName = ?" + DEFAULT_ORDER;
public static final String FIND_BY__STATUS_LIST =
"select " + LIST_FIELDS + " from `RedoraConfiguration` as " + ALIAS + " where " + ALIAS + ".status = ?" + DEFAULT_ORDER;
public static final String FIND_BY__STATUS_TABLE =
"select " + TABLE_FIELDS + " from `RedoraConfiguration` as " + ALIAS + " where " + ALIAS + ".status = ?" + DEFAULT_ORDER;
public static final String FETCH_LAZY_BY_ID =
"select " + LAZY_FIELDS + " from `RedoraConfiguration` as " + ALIAS + " where " + ALIAS + ".id = ?";
/** Create table statement for MySQL */
public final static String CREATE_TABLE = "create table `RedoraConfiguration` ("
+ "id bigint unsigned not null auto_increment, "
+ "scriptName varchar(255) ,"
+ "status enum('New','Ready','Error') default 'New' not null ,"
+ "output text ,"
+ "creationDate timestamp default CURRENT_TIMESTAMP, updateDate timestamp null"
+ ", primary key (id)"
+ ", index RedoraConfiguration_scriptName (scriptName)"
+ ", index RedoraConfiguration_status (status)"
+ ") default CHARSET=utf8 ENGINE INNODB";
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy