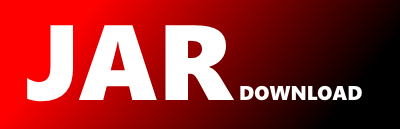
net.relaysoft.testing.azure.blob.mock.routes.GetContainerProperties.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-blob-mock Show documentation
Show all versions of azure-blob-mock Show documentation
Azure blob storage service mock
The newest version!
package net.relaysoft.testing.azure.blob.mock.routes
import akka.http.scaladsl.model.headers.RawHeader
import akka.http.scaladsl.model.{ContentType, DateTime, HttpCharsets, HttpEntity, HttpResponse, MediaTypes, StatusCodes}
import akka.http.scaladsl.server.Directives._
import akka.http.scaladsl.server.Route
import com.typesafe.scalalogging.LazyLogging
import net.relaysoft.testing.azure.blob.mock.exceptions.{ContainerNotFoundException, InternalErrorException}
import net.relaysoft.testing.azure.blob.mock.models.Container
import net.relaysoft.testing.azure.blob.mock.providers.Provider
import net.relaysoft.testing.azure.blob.mock.utils.HeaderNames
import java.util.UUID
import scala.util.{Failure, Success, Try}
case class GetContainerProperties()(implicit provider:Provider) extends LazyLogging {
def route(account: String, container:String, headers: Map[String, String]): Route = get {
parameters(Symbol("restype") ! "container") {
complete {
val headerString = headers.map(_.productIterator.mkString(":")).mkString(",")
logger.debug(s"account=$account, container=$container, message=Getting container properties, " +
s"headers=[$headerString]")
Try(provider.getContainerProperties(container)) match {
case Success(container) => HttpResponse(StatusCodes.OK).withHeaders(getResponseHeaders(container, headers))
case Failure(e: ContainerNotFoundException) => HttpResponse(
StatusCodes.NotFound,
entity = HttpEntity(
ContentType(MediaTypes.`application/xml`, HttpCharsets.`UTF-8`),
e.toXML.toString
)
)
case Failure(e) => HttpResponse(
StatusCodes.InternalServerError,
entity = HttpEntity(
ContentType(MediaTypes.`application/xml`, HttpCharsets.`UTF-8`),
InternalErrorException(e).toXML.toString
)
)
}
}
}
}
def getResponseHeaders(container: Container, requestHeaders: Map[String, String]): Seq[RawHeader] = {
val metadataHeaders = container.metaData.keySet.map(key => RawHeader(HeaderNames.PREFIX_X_MS_META + key,
container.metaData.getOrElse(key, ""))).toSeq
val headers = Seq(
RawHeader(HeaderNames.LAST_MODIFIED, container.lastModifiedDate.toRfc1123DateTimeString()),
RawHeader(HeaderNames.ETAG, container.etag),
RawHeader(HeaderNames.X_MS_REQUEST_ID, UUID.randomUUID().toString),
RawHeader(HeaderNames.X_MS_VERSION, requestHeaders.getOrElse(HeaderNames.X_MS_VERSION, "")),
RawHeader(HeaderNames.DATE, DateTime.now.toRfc1123DateTimeString()))
if(requestHeaders.contains(HeaderNames.X_MS_CLIENT_REQUEST_ID)) {
val clientHeaders = Seq(RawHeader(HeaderNames.X_MS_CLIENT_REQUEST_ID,
requestHeaders.getOrElse(HeaderNames.X_MS_CLIENT_REQUEST_ID, "")))
return headers ++ metadataHeaders ++ clientHeaders
}
headers ++ metadataHeaders
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy