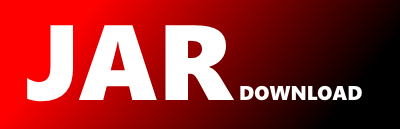
net.rgielen.com4j.office2010.word.InlineShape Maven / Gradle / Ivy
package net.rgielen.com4j.office2010.word ;
import com4j.*;
@IID("{000209A8-0000-0000-C000-000000000046}")
public interface InlineShape extends Com4jObject {
// Methods:
/**
*
* Getter method for the COM property "Application"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word._Application
*/
@DISPID(1000) //= 0x3e8. The runtime will prefer the VTID if present
@VTID(7)
net.rgielen.com4j.office2010.word._Application application();
/**
*
* Getter method for the COM property "Creator"
*
* @return Returns a value of type int
*/
@DISPID(1001) //= 0x3e9. The runtime will prefer the VTID if present
@VTID(8)
int creator();
/**
*
* Getter method for the COM property "Parent"
*
* @return Returns a value of type com4j.Com4jObject
*/
@DISPID(1002) //= 0x3ea. The runtime will prefer the VTID if present
@VTID(9)
@ReturnValue(type=NativeType.Dispatch)
com4j.Com4jObject parent();
/**
*
* Getter method for the COM property "Borders"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Borders
*/
@DISPID(1100) //= 0x44c. The runtime will prefer the VTID if present
@VTID(10)
net.rgielen.com4j.office2010.word.Borders borders();
@VTID(10)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Borders.class})
net.rgielen.com4j.office2010.word.Border borders(
net.rgielen.com4j.office2010.word.WdBorderType index);
/**
*
* Setter method for the COM property "Borders"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.Borders parameter.
*/
@DISPID(1100) //= 0x44c. The runtime will prefer the VTID if present
@VTID(11)
void borders(
net.rgielen.com4j.office2010.word.Borders prop);
/**
*
* Getter method for the COM property "Range"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Range
*/
@DISPID(2) //= 0x2. The runtime will prefer the VTID if present
@VTID(12)
net.rgielen.com4j.office2010.word.Range range();
/**
*
* Getter method for the COM property "LinkFormat"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.LinkFormat
*/
@DISPID(3) //= 0x3. The runtime will prefer the VTID if present
@VTID(13)
net.rgielen.com4j.office2010.word.LinkFormat linkFormat();
/**
*
* Getter method for the COM property "Field"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Field
*/
@DISPID(4) //= 0x4. The runtime will prefer the VTID if present
@VTID(14)
net.rgielen.com4j.office2010.word.Field field();
/**
*
* Getter method for the COM property "OLEFormat"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.OLEFormat
*/
@DISPID(5) //= 0x5. The runtime will prefer the VTID if present
@VTID(15)
net.rgielen.com4j.office2010.word.OLEFormat oleFormat();
/**
*
* Getter method for the COM property "Type"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdInlineShapeType
*/
@DISPID(6) //= 0x6. The runtime will prefer the VTID if present
@VTID(16)
net.rgielen.com4j.office2010.word.WdInlineShapeType type();
/**
*
* Getter method for the COM property "Hyperlink"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Hyperlink
*/
@DISPID(7) //= 0x7. The runtime will prefer the VTID if present
@VTID(17)
net.rgielen.com4j.office2010.word.Hyperlink hyperlink();
/**
*
* Getter method for the COM property "Height"
*
* @return Returns a value of type float
*/
@DISPID(8) //= 0x8. The runtime will prefer the VTID if present
@VTID(18)
float height();
/**
*
* Setter method for the COM property "Height"
*
* @param prop Mandatory float parameter.
*/
@DISPID(8) //= 0x8. The runtime will prefer the VTID if present
@VTID(19)
void height(
float prop);
/**
*
* Getter method for the COM property "Width"
*
* @return Returns a value of type float
*/
@DISPID(9) //= 0x9. The runtime will prefer the VTID if present
@VTID(20)
float width();
/**
*
* Setter method for the COM property "Width"
*
* @param prop Mandatory float parameter.
*/
@DISPID(9) //= 0x9. The runtime will prefer the VTID if present
@VTID(21)
void width(
float prop);
/**
*
* Getter method for the COM property "ScaleHeight"
*
* @return Returns a value of type float
*/
@DISPID(10) //= 0xa. The runtime will prefer the VTID if present
@VTID(22)
float scaleHeight();
/**
*
* Setter method for the COM property "ScaleHeight"
*
* @param prop Mandatory float parameter.
*/
@DISPID(10) //= 0xa. The runtime will prefer the VTID if present
@VTID(23)
void scaleHeight(
float prop);
/**
*
* Getter method for the COM property "ScaleWidth"
*
* @return Returns a value of type float
*/
@DISPID(11) //= 0xb. The runtime will prefer the VTID if present
@VTID(24)
float scaleWidth();
/**
*
* Setter method for the COM property "ScaleWidth"
*
* @param prop Mandatory float parameter.
*/
@DISPID(11) //= 0xb. The runtime will prefer the VTID if present
@VTID(25)
void scaleWidth(
float prop);
/**
*
* Getter method for the COM property "LockAspectRatio"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.MsoTriState
*/
@DISPID(12) //= 0xc. The runtime will prefer the VTID if present
@VTID(26)
net.rgielen.com4j.office2010.office.MsoTriState lockAspectRatio();
/**
*
* Setter method for the COM property "LockAspectRatio"
*
* @param prop Mandatory net.rgielen.com4j.office2010.office.MsoTriState parameter.
*/
@DISPID(12) //= 0xc. The runtime will prefer the VTID if present
@VTID(27)
void lockAspectRatio(
net.rgielen.com4j.office2010.office.MsoTriState prop);
/**
*
* Getter method for the COM property "Line"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.LineFormat
*/
@DISPID(112) //= 0x70. The runtime will prefer the VTID if present
@VTID(28)
net.rgielen.com4j.office2010.word.LineFormat line();
/**
*
* Getter method for the COM property "Fill"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.FillFormat
*/
@DISPID(107) //= 0x6b. The runtime will prefer the VTID if present
@VTID(29)
net.rgielen.com4j.office2010.word.FillFormat fill();
/**
*
* Getter method for the COM property "PictureFormat"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.PictureFormat
*/
@DISPID(118) //= 0x76. The runtime will prefer the VTID if present
@VTID(30)
net.rgielen.com4j.office2010.word.PictureFormat pictureFormat();
/**
*
* Setter method for the COM property "PictureFormat"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.PictureFormat parameter.
*/
@DISPID(118) //= 0x76. The runtime will prefer the VTID if present
@VTID(31)
void pictureFormat(
net.rgielen.com4j.office2010.word.PictureFormat prop);
/**
*/
@DISPID(100) //= 0x64. The runtime will prefer the VTID if present
@VTID(32)
void activate();
/**
*/
@DISPID(101) //= 0x65. The runtime will prefer the VTID if present
@VTID(33)
void reset();
/**
*/
@DISPID(102) //= 0x66. The runtime will prefer the VTID if present
@VTID(34)
void delete();
/**
*/
@DISPID(65535) //= 0xffff. The runtime will prefer the VTID if present
@VTID(35)
void select();
/**
* @return Returns a value of type net.rgielen.com4j.office2010.word.Shape
*/
@DISPID(104) //= 0x68. The runtime will prefer the VTID if present
@VTID(36)
net.rgielen.com4j.office2010.word.Shape convertToShape();
/**
*
* Getter method for the COM property "HorizontalLineFormat"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.HorizontalLineFormat
*/
@DISPID(119) //= 0x77. The runtime will prefer the VTID if present
@VTID(37)
net.rgielen.com4j.office2010.word.HorizontalLineFormat horizontalLineFormat();
/**
*
* Getter method for the COM property "Script"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.Script
*/
@DISPID(122) //= 0x7a. The runtime will prefer the VTID if present
@VTID(38)
net.rgielen.com4j.office2010.office.Script script();
/**
*
* Getter method for the COM property "OWSAnchor"
*
* @return Returns a value of type int
*/
@DISPID(130) //= 0x82. The runtime will prefer the VTID if present
@VTID(39)
int owsAnchor();
/**
*
* Getter method for the COM property "TextEffect"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.TextEffectFormat
*/
@DISPID(120) //= 0x78. The runtime will prefer the VTID if present
@VTID(40)
net.rgielen.com4j.office2010.word.TextEffectFormat textEffect();
/**
*
* Setter method for the COM property "TextEffect"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.TextEffectFormat parameter.
*/
@DISPID(120) //= 0x78. The runtime will prefer the VTID if present
@VTID(41)
void textEffect(
net.rgielen.com4j.office2010.word.TextEffectFormat prop);
/**
*
* Getter method for the COM property "AlternativeText"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(131) //= 0x83. The runtime will prefer the VTID if present
@VTID(42)
java.lang.String alternativeText();
/**
*
* Setter method for the COM property "AlternativeText"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(131) //= 0x83. The runtime will prefer the VTID if present
@VTID(43)
void alternativeText(
java.lang.String prop);
/**
*
* Getter method for the COM property "IsPictureBullet"
*
* @return Returns a value of type boolean
*/
@DISPID(132) //= 0x84. The runtime will prefer the VTID if present
@VTID(44)
boolean isPictureBullet();
/**
*
* Getter method for the COM property "GroupItems"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.GroupShapes
*/
@DISPID(133) //= 0x85. The runtime will prefer the VTID if present
@VTID(45)
net.rgielen.com4j.office2010.word.GroupShapes groupItems();
@VTID(45)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.GroupShapes.class})
net.rgielen.com4j.office2010.word.Shape groupItems(
java.lang.Object index);
/**
*
* Getter method for the COM property "HasChart"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.MsoTriState
*/
@DISPID(148) //= 0x94. The runtime will prefer the VTID if present
@VTID(46)
net.rgielen.com4j.office2010.office.MsoTriState hasChart();
/**
*
* Getter method for the COM property "Chart"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Chart
*/
@DISPID(149) //= 0x95. The runtime will prefer the VTID if present
@VTID(47)
net.rgielen.com4j.office2010.word.Chart chart();
/**
*
* Getter method for the COM property "SoftEdge"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.SoftEdgeFormat
*/
@DISPID(152) //= 0x98. The runtime will prefer the VTID if present
@VTID(48)
net.rgielen.com4j.office2010.word.SoftEdgeFormat softEdge();
/**
*
* Getter method for the COM property "Glow"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.GlowFormat
*/
@DISPID(153) //= 0x99. The runtime will prefer the VTID if present
@VTID(49)
net.rgielen.com4j.office2010.word.GlowFormat glow();
/**
*
* Getter method for the COM property "Reflection"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.ReflectionFormat
*/
@DISPID(154) //= 0x9a. The runtime will prefer the VTID if present
@VTID(50)
net.rgielen.com4j.office2010.word.ReflectionFormat reflection();
/**
*
* Getter method for the COM property "Shadow"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.ShadowFormat
*/
@DISPID(1101) //= 0x44d. The runtime will prefer the VTID if present
@VTID(51)
net.rgielen.com4j.office2010.word.ShadowFormat shadow();
/**
*
* Getter method for the COM property "HasSmartArt"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.MsoTriState
*/
@DISPID(155) //= 0x9b. The runtime will prefer the VTID if present
@VTID(52)
net.rgielen.com4j.office2010.office.MsoTriState hasSmartArt();
/**
*
* Getter method for the COM property "SmartArt"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.SmartArt
*/
@DISPID(156) //= 0x9c. The runtime will prefer the VTID if present
@VTID(53)
net.rgielen.com4j.office2010.office.SmartArt smartArt();
/**
*
* Getter method for the COM property "Title"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(158) //= 0x9e. The runtime will prefer the VTID if present
@VTID(54)
java.lang.String title();
/**
*
* Setter method for the COM property "Title"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(158) //= 0x9e. The runtime will prefer the VTID if present
@VTID(55)
void title(
java.lang.String prop);
/**
*
* Getter method for the COM property "AnchorID"
*
* @return Returns a value of type int
*/
@DISPID(207) //= 0xcf. The runtime will prefer the VTID if present
@VTID(56)
int anchorID();
/**
*
* Getter method for the COM property "EditID"
*
* @return Returns a value of type int
*/
@DISPID(208) //= 0xd0. The runtime will prefer the VTID if present
@VTID(57)
int editID();
// Properties:
}