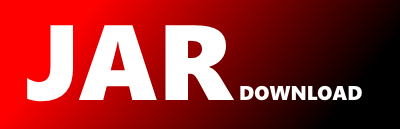
net.rgielen.com4j.office2010.word.OMathMat Maven / Gradle / Ivy
package net.rgielen.com4j.office2010.word ;
import com4j.*;
@IID("{3E061A7E-67AD-4EAA-BC1E-55057D5E596F}")
public interface OMathMat extends Com4jObject {
// Methods:
/**
*
* Getter method for the COM property "Application"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word._Application
*/
@DISPID(100) //= 0x64. The runtime will prefer the VTID if present
@VTID(7)
net.rgielen.com4j.office2010.word._Application application();
/**
*
* Getter method for the COM property "Creator"
*
* @return Returns a value of type int
*/
@DISPID(101) //= 0x65. The runtime will prefer the VTID if present
@VTID(8)
int creator();
/**
*
* Getter method for the COM property "Parent"
*
* @return Returns a value of type com4j.Com4jObject
*/
@DISPID(102) //= 0x66. The runtime will prefer the VTID if present
@VTID(9)
@ReturnValue(type=NativeType.Dispatch)
com4j.Com4jObject parent();
/**
*
* Getter method for the COM property "Rows"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.OMathMatRows
*/
@DISPID(103) //= 0x67. The runtime will prefer the VTID if present
@VTID(10)
net.rgielen.com4j.office2010.word.OMathMatRows rows();
@VTID(10)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.OMathMatRows.class})
net.rgielen.com4j.office2010.word.OMathMatRow rows(
int index);
/**
*
* Getter method for the COM property "Cols"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.OMathMatCols
*/
@DISPID(104) //= 0x68. The runtime will prefer the VTID if present
@VTID(11)
net.rgielen.com4j.office2010.word.OMathMatCols cols();
@VTID(11)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.OMathMatCols.class})
net.rgielen.com4j.office2010.word.OMathMatCol cols(
int index);
/**
*
* Getter method for the COM property "Cell"
*
* @param row Mandatory int parameter.
* @param col Mandatory int parameter.
* @return Returns a value of type net.rgielen.com4j.office2010.word.OMath
*/
@DISPID(105) //= 0x69. The runtime will prefer the VTID if present
@VTID(12)
net.rgielen.com4j.office2010.word.OMath cell(
int row,
int col);
/**
*
* Getter method for the COM property "Align"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdOMathVertAlignType
*/
@DISPID(106) //= 0x6a. The runtime will prefer the VTID if present
@VTID(13)
net.rgielen.com4j.office2010.word.WdOMathVertAlignType align();
/**
*
* Setter method for the COM property "Align"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdOMathVertAlignType parameter.
*/
@DISPID(106) //= 0x6a. The runtime will prefer the VTID if present
@VTID(14)
void align(
net.rgielen.com4j.office2010.word.WdOMathVertAlignType prop);
/**
*
* Getter method for the COM property "PlcHoldHidden"
*
* @return Returns a value of type boolean
*/
@DISPID(107) //= 0x6b. The runtime will prefer the VTID if present
@VTID(15)
boolean plcHoldHidden();
/**
*
* Setter method for the COM property "PlcHoldHidden"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(107) //= 0x6b. The runtime will prefer the VTID if present
@VTID(16)
void plcHoldHidden(
boolean prop);
/**
*
* Getter method for the COM property "RowSpacingRule"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdOMathSpacingRule
*/
@DISPID(108) //= 0x6c. The runtime will prefer the VTID if present
@VTID(17)
net.rgielen.com4j.office2010.word.WdOMathSpacingRule rowSpacingRule();
/**
*
* Setter method for the COM property "RowSpacingRule"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdOMathSpacingRule parameter.
*/
@DISPID(108) //= 0x6c. The runtime will prefer the VTID if present
@VTID(18)
void rowSpacingRule(
net.rgielen.com4j.office2010.word.WdOMathSpacingRule prop);
/**
*
* Getter method for the COM property "RowSpacing"
*
* @return Returns a value of type int
*/
@DISPID(109) //= 0x6d. The runtime will prefer the VTID if present
@VTID(19)
int rowSpacing();
/**
*
* Setter method for the COM property "RowSpacing"
*
* @param prop Mandatory int parameter.
*/
@DISPID(109) //= 0x6d. The runtime will prefer the VTID if present
@VTID(20)
void rowSpacing(
int prop);
/**
*
* Getter method for the COM property "ColSpacing"
*
* @return Returns a value of type int
*/
@DISPID(110) //= 0x6e. The runtime will prefer the VTID if present
@VTID(21)
int colSpacing();
/**
*
* Setter method for the COM property "ColSpacing"
*
* @param prop Mandatory int parameter.
*/
@DISPID(110) //= 0x6e. The runtime will prefer the VTID if present
@VTID(22)
void colSpacing(
int prop);
/**
*
* Getter method for the COM property "ColGapRule"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdOMathSpacingRule
*/
@DISPID(111) //= 0x6f. The runtime will prefer the VTID if present
@VTID(23)
net.rgielen.com4j.office2010.word.WdOMathSpacingRule colGapRule();
/**
*
* Setter method for the COM property "ColGapRule"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdOMathSpacingRule parameter.
*/
@DISPID(111) //= 0x6f. The runtime will prefer the VTID if present
@VTID(24)
void colGapRule(
net.rgielen.com4j.office2010.word.WdOMathSpacingRule prop);
/**
*
* Getter method for the COM property "ColGap"
*
* @return Returns a value of type int
*/
@DISPID(112) //= 0x70. The runtime will prefer the VTID if present
@VTID(25)
int colGap();
/**
*
* Setter method for the COM property "ColGap"
*
* @param prop Mandatory int parameter.
*/
@DISPID(112) //= 0x70. The runtime will prefer the VTID if present
@VTID(26)
void colGap(
int prop);
// Properties:
}