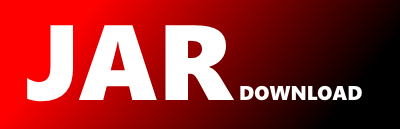
net.rgielen.com4j.office2010.word.Options Maven / Gradle / Ivy
package net.rgielen.com4j.office2010.word ;
import com4j.*;
@IID("{000209B7-0000-0000-C000-000000000046}")
public interface Options extends Com4jObject {
// Methods:
/**
*
* Getter method for the COM property "Application"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word._Application
*/
@DISPID(1000) //= 0x3e8. The runtime will prefer the VTID if present
@VTID(7)
net.rgielen.com4j.office2010.word._Application application();
/**
*
* Getter method for the COM property "Creator"
*
* @return Returns a value of type int
*/
@DISPID(1001) //= 0x3e9. The runtime will prefer the VTID if present
@VTID(8)
int creator();
/**
*
* Getter method for the COM property "Parent"
*
* @return Returns a value of type com4j.Com4jObject
*/
@DISPID(1002) //= 0x3ea. The runtime will prefer the VTID if present
@VTID(9)
@ReturnValue(type=NativeType.Dispatch)
com4j.Com4jObject parent();
/**
*
* Getter method for the COM property "AllowAccentedUppercase"
*
* @return Returns a value of type boolean
*/
@DISPID(1) //= 0x1. The runtime will prefer the VTID if present
@VTID(10)
boolean allowAccentedUppercase();
/**
*
* Setter method for the COM property "AllowAccentedUppercase"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(1) //= 0x1. The runtime will prefer the VTID if present
@VTID(11)
void allowAccentedUppercase(
boolean prop);
/**
*
* Getter method for the COM property "WPHelp"
*
* @return Returns a value of type boolean
*/
@DISPID(17) //= 0x11. The runtime will prefer the VTID if present
@VTID(12)
boolean wpHelp();
/**
*
* Setter method for the COM property "WPHelp"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(17) //= 0x11. The runtime will prefer the VTID if present
@VTID(13)
void wpHelp(
boolean prop);
/**
*
* Getter method for the COM property "WPDocNavKeys"
*
* @return Returns a value of type boolean
*/
@DISPID(18) //= 0x12. The runtime will prefer the VTID if present
@VTID(14)
boolean wpDocNavKeys();
/**
*
* Setter method for the COM property "WPDocNavKeys"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(18) //= 0x12. The runtime will prefer the VTID if present
@VTID(15)
void wpDocNavKeys(
boolean prop);
/**
*
* Getter method for the COM property "Pagination"
*
* @return Returns a value of type boolean
*/
@DISPID(19) //= 0x13. The runtime will prefer the VTID if present
@VTID(16)
boolean pagination();
/**
*
* Setter method for the COM property "Pagination"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(19) //= 0x13. The runtime will prefer the VTID if present
@VTID(17)
void pagination(
boolean prop);
/**
*
* Getter method for the COM property "BlueScreen"
*
* @return Returns a value of type boolean
*/
@DISPID(20) //= 0x14. The runtime will prefer the VTID if present
@VTID(18)
boolean blueScreen();
/**
*
* Setter method for the COM property "BlueScreen"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(20) //= 0x14. The runtime will prefer the VTID if present
@VTID(19)
void blueScreen(
boolean prop);
/**
*
* Getter method for the COM property "EnableSound"
*
* @return Returns a value of type boolean
*/
@DISPID(21) //= 0x15. The runtime will prefer the VTID if present
@VTID(20)
boolean enableSound();
/**
*
* Setter method for the COM property "EnableSound"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(21) //= 0x15. The runtime will prefer the VTID if present
@VTID(21)
void enableSound(
boolean prop);
/**
*
* Getter method for the COM property "ConfirmConversions"
*
* @return Returns a value of type boolean
*/
@DISPID(22) //= 0x16. The runtime will prefer the VTID if present
@VTID(22)
boolean confirmConversions();
/**
*
* Setter method for the COM property "ConfirmConversions"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(22) //= 0x16. The runtime will prefer the VTID if present
@VTID(23)
void confirmConversions(
boolean prop);
/**
*
* Getter method for the COM property "UpdateLinksAtOpen"
*
* @return Returns a value of type boolean
*/
@DISPID(23) //= 0x17. The runtime will prefer the VTID if present
@VTID(24)
boolean updateLinksAtOpen();
/**
*
* Setter method for the COM property "UpdateLinksAtOpen"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(23) //= 0x17. The runtime will prefer the VTID if present
@VTID(25)
void updateLinksAtOpen(
boolean prop);
/**
*
* Getter method for the COM property "SendMailAttach"
*
* @return Returns a value of type boolean
*/
@DISPID(24) //= 0x18. The runtime will prefer the VTID if present
@VTID(26)
boolean sendMailAttach();
/**
*
* Setter method for the COM property "SendMailAttach"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(24) //= 0x18. The runtime will prefer the VTID if present
@VTID(27)
void sendMailAttach(
boolean prop);
/**
*
* Getter method for the COM property "MeasurementUnit"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdMeasurementUnits
*/
@DISPID(26) //= 0x1a. The runtime will prefer the VTID if present
@VTID(28)
net.rgielen.com4j.office2010.word.WdMeasurementUnits measurementUnit();
/**
*
* Setter method for the COM property "MeasurementUnit"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdMeasurementUnits parameter.
*/
@DISPID(26) //= 0x1a. The runtime will prefer the VTID if present
@VTID(29)
void measurementUnit(
net.rgielen.com4j.office2010.word.WdMeasurementUnits prop);
/**
*
* Getter method for the COM property "ButtonFieldClicks"
*
* @return Returns a value of type int
*/
@DISPID(27) //= 0x1b. The runtime will prefer the VTID if present
@VTID(30)
int buttonFieldClicks();
/**
*
* Setter method for the COM property "ButtonFieldClicks"
*
* @param prop Mandatory int parameter.
*/
@DISPID(27) //= 0x1b. The runtime will prefer the VTID if present
@VTID(31)
void buttonFieldClicks(
int prop);
/**
*
* Getter method for the COM property "ShortMenuNames"
*
* @return Returns a value of type boolean
*/
@DISPID(28) //= 0x1c. The runtime will prefer the VTID if present
@VTID(32)
boolean shortMenuNames();
/**
*
* Setter method for the COM property "ShortMenuNames"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(28) //= 0x1c. The runtime will prefer the VTID if present
@VTID(33)
void shortMenuNames(
boolean prop);
/**
*
* Getter method for the COM property "RTFInClipboard"
*
* @return Returns a value of type boolean
*/
@DISPID(29) //= 0x1d. The runtime will prefer the VTID if present
@VTID(34)
boolean rtfInClipboard();
/**
*
* Setter method for the COM property "RTFInClipboard"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(29) //= 0x1d. The runtime will prefer the VTID if present
@VTID(35)
void rtfInClipboard(
boolean prop);
/**
*
* Getter method for the COM property "UpdateFieldsAtPrint"
*
* @return Returns a value of type boolean
*/
@DISPID(30) //= 0x1e. The runtime will prefer the VTID if present
@VTID(36)
boolean updateFieldsAtPrint();
/**
*
* Setter method for the COM property "UpdateFieldsAtPrint"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(30) //= 0x1e. The runtime will prefer the VTID if present
@VTID(37)
void updateFieldsAtPrint(
boolean prop);
/**
*
* Getter method for the COM property "PrintProperties"
*
* @return Returns a value of type boolean
*/
@DISPID(31) //= 0x1f. The runtime will prefer the VTID if present
@VTID(38)
boolean printProperties();
/**
*
* Setter method for the COM property "PrintProperties"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(31) //= 0x1f. The runtime will prefer the VTID if present
@VTID(39)
void printProperties(
boolean prop);
/**
*
* Getter method for the COM property "PrintFieldCodes"
*
* @return Returns a value of type boolean
*/
@DISPID(32) //= 0x20. The runtime will prefer the VTID if present
@VTID(40)
boolean printFieldCodes();
/**
*
* Setter method for the COM property "PrintFieldCodes"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(32) //= 0x20. The runtime will prefer the VTID if present
@VTID(41)
void printFieldCodes(
boolean prop);
/**
*
* Getter method for the COM property "PrintComments"
*
* @return Returns a value of type boolean
*/
@DISPID(33) //= 0x21. The runtime will prefer the VTID if present
@VTID(42)
boolean printComments();
/**
*
* Setter method for the COM property "PrintComments"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(33) //= 0x21. The runtime will prefer the VTID if present
@VTID(43)
void printComments(
boolean prop);
/**
*
* Getter method for the COM property "PrintHiddenText"
*
* @return Returns a value of type boolean
*/
@DISPID(34) //= 0x22. The runtime will prefer the VTID if present
@VTID(44)
boolean printHiddenText();
/**
*
* Setter method for the COM property "PrintHiddenText"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(34) //= 0x22. The runtime will prefer the VTID if present
@VTID(45)
void printHiddenText(
boolean prop);
/**
*
* Getter method for the COM property "EnvelopeFeederInstalled"
*
* @return Returns a value of type boolean
*/
@DISPID(35) //= 0x23. The runtime will prefer the VTID if present
@VTID(46)
boolean envelopeFeederInstalled();
/**
*
* Getter method for the COM property "UpdateLinksAtPrint"
*
* @return Returns a value of type boolean
*/
@DISPID(36) //= 0x24. The runtime will prefer the VTID if present
@VTID(47)
boolean updateLinksAtPrint();
/**
*
* Setter method for the COM property "UpdateLinksAtPrint"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(36) //= 0x24. The runtime will prefer the VTID if present
@VTID(48)
void updateLinksAtPrint(
boolean prop);
/**
*
* Getter method for the COM property "PrintBackground"
*
* @return Returns a value of type boolean
*/
@DISPID(37) //= 0x25. The runtime will prefer the VTID if present
@VTID(49)
boolean printBackground();
/**
*
* Setter method for the COM property "PrintBackground"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(37) //= 0x25. The runtime will prefer the VTID if present
@VTID(50)
void printBackground(
boolean prop);
/**
*
* Getter method for the COM property "PrintDrawingObjects"
*
* @return Returns a value of type boolean
*/
@DISPID(38) //= 0x26. The runtime will prefer the VTID if present
@VTID(51)
boolean printDrawingObjects();
/**
*
* Setter method for the COM property "PrintDrawingObjects"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(38) //= 0x26. The runtime will prefer the VTID if present
@VTID(52)
void printDrawingObjects(
boolean prop);
/**
*
* Getter method for the COM property "DefaultTray"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(39) //= 0x27. The runtime will prefer the VTID if present
@VTID(53)
java.lang.String defaultTray();
/**
*
* Setter method for the COM property "DefaultTray"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(39) //= 0x27. The runtime will prefer the VTID if present
@VTID(54)
void defaultTray(
java.lang.String prop);
/**
*
* Getter method for the COM property "DefaultTrayID"
*
* @return Returns a value of type int
*/
@DISPID(40) //= 0x28. The runtime will prefer the VTID if present
@VTID(55)
int defaultTrayID();
/**
*
* Setter method for the COM property "DefaultTrayID"
*
* @param prop Mandatory int parameter.
*/
@DISPID(40) //= 0x28. The runtime will prefer the VTID if present
@VTID(56)
void defaultTrayID(
int prop);
/**
*
* Getter method for the COM property "CreateBackup"
*
* @return Returns a value of type boolean
*/
@DISPID(41) //= 0x29. The runtime will prefer the VTID if present
@VTID(57)
boolean createBackup();
/**
*
* Setter method for the COM property "CreateBackup"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(41) //= 0x29. The runtime will prefer the VTID if present
@VTID(58)
void createBackup(
boolean prop);
/**
*
* Getter method for the COM property "AllowFastSave"
*
* @return Returns a value of type boolean
*/
@DISPID(42) //= 0x2a. The runtime will prefer the VTID if present
@VTID(59)
boolean allowFastSave();
/**
*
* Setter method for the COM property "AllowFastSave"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(42) //= 0x2a. The runtime will prefer the VTID if present
@VTID(60)
void allowFastSave(
boolean prop);
/**
*
* Getter method for the COM property "SavePropertiesPrompt"
*
* @return Returns a value of type boolean
*/
@DISPID(43) //= 0x2b. The runtime will prefer the VTID if present
@VTID(61)
boolean savePropertiesPrompt();
/**
*
* Setter method for the COM property "SavePropertiesPrompt"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(43) //= 0x2b. The runtime will prefer the VTID if present
@VTID(62)
void savePropertiesPrompt(
boolean prop);
/**
*
* Getter method for the COM property "SaveNormalPrompt"
*
* @return Returns a value of type boolean
*/
@DISPID(44) //= 0x2c. The runtime will prefer the VTID if present
@VTID(63)
boolean saveNormalPrompt();
/**
*
* Setter method for the COM property "SaveNormalPrompt"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(44) //= 0x2c. The runtime will prefer the VTID if present
@VTID(64)
void saveNormalPrompt(
boolean prop);
/**
*
* Getter method for the COM property "SaveInterval"
*
* @return Returns a value of type int
*/
@DISPID(45) //= 0x2d. The runtime will prefer the VTID if present
@VTID(65)
int saveInterval();
/**
*
* Setter method for the COM property "SaveInterval"
*
* @param prop Mandatory int parameter.
*/
@DISPID(45) //= 0x2d. The runtime will prefer the VTID if present
@VTID(66)
void saveInterval(
int prop);
/**
*
* Getter method for the COM property "BackgroundSave"
*
* @return Returns a value of type boolean
*/
@DISPID(46) //= 0x2e. The runtime will prefer the VTID if present
@VTID(67)
boolean backgroundSave();
/**
*
* Setter method for the COM property "BackgroundSave"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(46) //= 0x2e. The runtime will prefer the VTID if present
@VTID(68)
void backgroundSave(
boolean prop);
/**
*
* Getter method for the COM property "InsertedTextMark"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdInsertedTextMark
*/
@DISPID(57) //= 0x39. The runtime will prefer the VTID if present
@VTID(69)
net.rgielen.com4j.office2010.word.WdInsertedTextMark insertedTextMark();
/**
*
* Setter method for the COM property "InsertedTextMark"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdInsertedTextMark parameter.
*/
@DISPID(57) //= 0x39. The runtime will prefer the VTID if present
@VTID(70)
void insertedTextMark(
net.rgielen.com4j.office2010.word.WdInsertedTextMark prop);
/**
*
* Getter method for the COM property "DeletedTextMark"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdDeletedTextMark
*/
@DISPID(58) //= 0x3a. The runtime will prefer the VTID if present
@VTID(71)
net.rgielen.com4j.office2010.word.WdDeletedTextMark deletedTextMark();
/**
*
* Setter method for the COM property "DeletedTextMark"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdDeletedTextMark parameter.
*/
@DISPID(58) //= 0x3a. The runtime will prefer the VTID if present
@VTID(72)
void deletedTextMark(
net.rgielen.com4j.office2010.word.WdDeletedTextMark prop);
/**
*
* Getter method for the COM property "RevisedLinesMark"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdRevisedLinesMark
*/
@DISPID(59) //= 0x3b. The runtime will prefer the VTID if present
@VTID(73)
net.rgielen.com4j.office2010.word.WdRevisedLinesMark revisedLinesMark();
/**
*
* Setter method for the COM property "RevisedLinesMark"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdRevisedLinesMark parameter.
*/
@DISPID(59) //= 0x3b. The runtime will prefer the VTID if present
@VTID(74)
void revisedLinesMark(
net.rgielen.com4j.office2010.word.WdRevisedLinesMark prop);
/**
*
* Getter method for the COM property "InsertedTextColor"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdColorIndex
*/
@DISPID(60) //= 0x3c. The runtime will prefer the VTID if present
@VTID(75)
net.rgielen.com4j.office2010.word.WdColorIndex insertedTextColor();
/**
*
* Setter method for the COM property "InsertedTextColor"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdColorIndex parameter.
*/
@DISPID(60) //= 0x3c. The runtime will prefer the VTID if present
@VTID(76)
void insertedTextColor(
net.rgielen.com4j.office2010.word.WdColorIndex prop);
/**
*
* Getter method for the COM property "DeletedTextColor"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdColorIndex
*/
@DISPID(61) //= 0x3d. The runtime will prefer the VTID if present
@VTID(77)
net.rgielen.com4j.office2010.word.WdColorIndex deletedTextColor();
/**
*
* Setter method for the COM property "DeletedTextColor"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdColorIndex parameter.
*/
@DISPID(61) //= 0x3d. The runtime will prefer the VTID if present
@VTID(78)
void deletedTextColor(
net.rgielen.com4j.office2010.word.WdColorIndex prop);
/**
*
* Getter method for the COM property "RevisedLinesColor"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdColorIndex
*/
@DISPID(62) //= 0x3e. The runtime will prefer the VTID if present
@VTID(79)
net.rgielen.com4j.office2010.word.WdColorIndex revisedLinesColor();
/**
*
* Setter method for the COM property "RevisedLinesColor"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdColorIndex parameter.
*/
@DISPID(62) //= 0x3e. The runtime will prefer the VTID if present
@VTID(80)
void revisedLinesColor(
net.rgielen.com4j.office2010.word.WdColorIndex prop);
/**
*
* Getter method for the COM property "DefaultFilePath"
*
* @param path Mandatory net.rgielen.com4j.office2010.word.WdDefaultFilePath parameter.
* @return Returns a value of type java.lang.String
*/
@DISPID(65) //= 0x41. The runtime will prefer the VTID if present
@VTID(81)
java.lang.String defaultFilePath(
net.rgielen.com4j.office2010.word.WdDefaultFilePath path);
/**
*
* Setter method for the COM property "DefaultFilePath"
*
* @param path Mandatory net.rgielen.com4j.office2010.word.WdDefaultFilePath parameter.
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(65) //= 0x41. The runtime will prefer the VTID if present
@VTID(82)
void defaultFilePath(
net.rgielen.com4j.office2010.word.WdDefaultFilePath path,
java.lang.String prop);
/**
*
* Getter method for the COM property "Overtype"
*
* @return Returns a value of type boolean
*/
@DISPID(66) //= 0x42. The runtime will prefer the VTID if present
@VTID(83)
boolean overtype();
/**
*
* Setter method for the COM property "Overtype"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(66) //= 0x42. The runtime will prefer the VTID if present
@VTID(84)
void overtype(
boolean prop);
/**
*
* Getter method for the COM property "ReplaceSelection"
*
* @return Returns a value of type boolean
*/
@DISPID(67) //= 0x43. The runtime will prefer the VTID if present
@VTID(85)
boolean replaceSelection();
/**
*
* Setter method for the COM property "ReplaceSelection"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(67) //= 0x43. The runtime will prefer the VTID if present
@VTID(86)
void replaceSelection(
boolean prop);
/**
*
* Getter method for the COM property "AllowDragAndDrop"
*
* @return Returns a value of type boolean
*/
@DISPID(68) //= 0x44. The runtime will prefer the VTID if present
@VTID(87)
boolean allowDragAndDrop();
/**
*
* Setter method for the COM property "AllowDragAndDrop"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(68) //= 0x44. The runtime will prefer the VTID if present
@VTID(88)
void allowDragAndDrop(
boolean prop);
/**
*
* Getter method for the COM property "AutoWordSelection"
*
* @return Returns a value of type boolean
*/
@DISPID(69) //= 0x45. The runtime will prefer the VTID if present
@VTID(89)
boolean autoWordSelection();
/**
*
* Setter method for the COM property "AutoWordSelection"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(69) //= 0x45. The runtime will prefer the VTID if present
@VTID(90)
void autoWordSelection(
boolean prop);
/**
*
* Getter method for the COM property "INSKeyForPaste"
*
* @return Returns a value of type boolean
*/
@DISPID(70) //= 0x46. The runtime will prefer the VTID if present
@VTID(91)
boolean insKeyForPaste();
/**
*
* Setter method for the COM property "INSKeyForPaste"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(70) //= 0x46. The runtime will prefer the VTID if present
@VTID(92)
void insKeyForPaste(
boolean prop);
/**
*
* Getter method for the COM property "SmartCutPaste"
*
* @return Returns a value of type boolean
*/
@DISPID(71) //= 0x47. The runtime will prefer the VTID if present
@VTID(93)
boolean smartCutPaste();
/**
*
* Setter method for the COM property "SmartCutPaste"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(71) //= 0x47. The runtime will prefer the VTID if present
@VTID(94)
void smartCutPaste(
boolean prop);
/**
*
* Getter method for the COM property "TabIndentKey"
*
* @return Returns a value of type boolean
*/
@DISPID(72) //= 0x48. The runtime will prefer the VTID if present
@VTID(95)
boolean tabIndentKey();
/**
*
* Setter method for the COM property "TabIndentKey"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(72) //= 0x48. The runtime will prefer the VTID if present
@VTID(96)
void tabIndentKey(
boolean prop);
/**
*
* Getter method for the COM property "PictureEditor"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(73) //= 0x49. The runtime will prefer the VTID if present
@VTID(97)
java.lang.String pictureEditor();
/**
*
* Setter method for the COM property "PictureEditor"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(73) //= 0x49. The runtime will prefer the VTID if present
@VTID(98)
void pictureEditor(
java.lang.String prop);
/**
*
* Getter method for the COM property "AnimateScreenMovements"
*
* @return Returns a value of type boolean
*/
@DISPID(74) //= 0x4a. The runtime will prefer the VTID if present
@VTID(99)
boolean animateScreenMovements();
/**
*
* Setter method for the COM property "AnimateScreenMovements"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(74) //= 0x4a. The runtime will prefer the VTID if present
@VTID(100)
void animateScreenMovements(
boolean prop);
/**
*
* Getter method for the COM property "VirusProtection"
*
* @return Returns a value of type boolean
*/
@DISPID(75) //= 0x4b. The runtime will prefer the VTID if present
@VTID(101)
boolean virusProtection();
/**
*
* Setter method for the COM property "VirusProtection"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(75) //= 0x4b. The runtime will prefer the VTID if present
@VTID(102)
void virusProtection(
boolean prop);
/**
*
* Getter method for the COM property "RevisedPropertiesMark"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdRevisedPropertiesMark
*/
@DISPID(76) //= 0x4c. The runtime will prefer the VTID if present
@VTID(103)
net.rgielen.com4j.office2010.word.WdRevisedPropertiesMark revisedPropertiesMark();
/**
*
* Setter method for the COM property "RevisedPropertiesMark"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdRevisedPropertiesMark parameter.
*/
@DISPID(76) //= 0x4c. The runtime will prefer the VTID if present
@VTID(104)
void revisedPropertiesMark(
net.rgielen.com4j.office2010.word.WdRevisedPropertiesMark prop);
/**
*
* Getter method for the COM property "RevisedPropertiesColor"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdColorIndex
*/
@DISPID(77) //= 0x4d. The runtime will prefer the VTID if present
@VTID(105)
net.rgielen.com4j.office2010.word.WdColorIndex revisedPropertiesColor();
/**
*
* Setter method for the COM property "RevisedPropertiesColor"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdColorIndex parameter.
*/
@DISPID(77) //= 0x4d. The runtime will prefer the VTID if present
@VTID(106)
void revisedPropertiesColor(
net.rgielen.com4j.office2010.word.WdColorIndex prop);
/**
*
* Getter method for the COM property "SnapToGrid"
*
* @return Returns a value of type boolean
*/
@DISPID(79) //= 0x4f. The runtime will prefer the VTID if present
@VTID(107)
boolean snapToGrid();
/**
*
* Setter method for the COM property "SnapToGrid"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(79) //= 0x4f. The runtime will prefer the VTID if present
@VTID(108)
void snapToGrid(
boolean prop);
/**
*
* Getter method for the COM property "SnapToShapes"
*
* @return Returns a value of type boolean
*/
@DISPID(80) //= 0x50. The runtime will prefer the VTID if present
@VTID(109)
boolean snapToShapes();
/**
*
* Setter method for the COM property "SnapToShapes"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(80) //= 0x50. The runtime will prefer the VTID if present
@VTID(110)
void snapToShapes(
boolean prop);
/**
*
* Getter method for the COM property "GridDistanceHorizontal"
*
* @return Returns a value of type float
*/
@DISPID(81) //= 0x51. The runtime will prefer the VTID if present
@VTID(111)
float gridDistanceHorizontal();
/**
*
* Setter method for the COM property "GridDistanceHorizontal"
*
* @param prop Mandatory float parameter.
*/
@DISPID(81) //= 0x51. The runtime will prefer the VTID if present
@VTID(112)
void gridDistanceHorizontal(
float prop);
/**
*
* Getter method for the COM property "GridDistanceVertical"
*
* @return Returns a value of type float
*/
@DISPID(82) //= 0x52. The runtime will prefer the VTID if present
@VTID(113)
float gridDistanceVertical();
/**
*
* Setter method for the COM property "GridDistanceVertical"
*
* @param prop Mandatory float parameter.
*/
@DISPID(82) //= 0x52. The runtime will prefer the VTID if present
@VTID(114)
void gridDistanceVertical(
float prop);
/**
*
* Getter method for the COM property "GridOriginHorizontal"
*
* @return Returns a value of type float
*/
@DISPID(83) //= 0x53. The runtime will prefer the VTID if present
@VTID(115)
float gridOriginHorizontal();
/**
*
* Setter method for the COM property "GridOriginHorizontal"
*
* @param prop Mandatory float parameter.
*/
@DISPID(83) //= 0x53. The runtime will prefer the VTID if present
@VTID(116)
void gridOriginHorizontal(
float prop);
/**
*
* Getter method for the COM property "GridOriginVertical"
*
* @return Returns a value of type float
*/
@DISPID(84) //= 0x54. The runtime will prefer the VTID if present
@VTID(117)
float gridOriginVertical();
/**
*
* Setter method for the COM property "GridOriginVertical"
*
* @param prop Mandatory float parameter.
*/
@DISPID(84) //= 0x54. The runtime will prefer the VTID if present
@VTID(118)
void gridOriginVertical(
float prop);
/**
*
* Getter method for the COM property "InlineConversion"
*
* @return Returns a value of type boolean
*/
@DISPID(86) //= 0x56. The runtime will prefer the VTID if present
@VTID(119)
boolean inlineConversion();
/**
*
* Setter method for the COM property "InlineConversion"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(86) //= 0x56. The runtime will prefer the VTID if present
@VTID(120)
void inlineConversion(
boolean prop);
/**
*
* Getter method for the COM property "IMEAutomaticControl"
*
* @return Returns a value of type boolean
*/
@DISPID(87) //= 0x57. The runtime will prefer the VTID if present
@VTID(121)
boolean imeAutomaticControl();
/**
*
* Setter method for the COM property "IMEAutomaticControl"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(87) //= 0x57. The runtime will prefer the VTID if present
@VTID(122)
void imeAutomaticControl(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatApplyHeadings"
*
* @return Returns a value of type boolean
*/
@DISPID(250) //= 0xfa. The runtime will prefer the VTID if present
@VTID(123)
boolean autoFormatApplyHeadings();
/**
*
* Setter method for the COM property "AutoFormatApplyHeadings"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(250) //= 0xfa. The runtime will prefer the VTID if present
@VTID(124)
void autoFormatApplyHeadings(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatApplyLists"
*
* @return Returns a value of type boolean
*/
@DISPID(251) //= 0xfb. The runtime will prefer the VTID if present
@VTID(125)
boolean autoFormatApplyLists();
/**
*
* Setter method for the COM property "AutoFormatApplyLists"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(251) //= 0xfb. The runtime will prefer the VTID if present
@VTID(126)
void autoFormatApplyLists(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatApplyBulletedLists"
*
* @return Returns a value of type boolean
*/
@DISPID(252) //= 0xfc. The runtime will prefer the VTID if present
@VTID(127)
boolean autoFormatApplyBulletedLists();
/**
*
* Setter method for the COM property "AutoFormatApplyBulletedLists"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(252) //= 0xfc. The runtime will prefer the VTID if present
@VTID(128)
void autoFormatApplyBulletedLists(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatApplyOtherParas"
*
* @return Returns a value of type boolean
*/
@DISPID(253) //= 0xfd. The runtime will prefer the VTID if present
@VTID(129)
boolean autoFormatApplyOtherParas();
/**
*
* Setter method for the COM property "AutoFormatApplyOtherParas"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(253) //= 0xfd. The runtime will prefer the VTID if present
@VTID(130)
void autoFormatApplyOtherParas(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatReplaceQuotes"
*
* @return Returns a value of type boolean
*/
@DISPID(254) //= 0xfe. The runtime will prefer the VTID if present
@VTID(131)
boolean autoFormatReplaceQuotes();
/**
*
* Setter method for the COM property "AutoFormatReplaceQuotes"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(254) //= 0xfe. The runtime will prefer the VTID if present
@VTID(132)
void autoFormatReplaceQuotes(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatReplaceSymbols"
*
* @return Returns a value of type boolean
*/
@DISPID(255) //= 0xff. The runtime will prefer the VTID if present
@VTID(133)
boolean autoFormatReplaceSymbols();
/**
*
* Setter method for the COM property "AutoFormatReplaceSymbols"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(255) //= 0xff. The runtime will prefer the VTID if present
@VTID(134)
void autoFormatReplaceSymbols(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatReplaceOrdinals"
*
* @return Returns a value of type boolean
*/
@DISPID(256) //= 0x100. The runtime will prefer the VTID if present
@VTID(135)
boolean autoFormatReplaceOrdinals();
/**
*
* Setter method for the COM property "AutoFormatReplaceOrdinals"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(256) //= 0x100. The runtime will prefer the VTID if present
@VTID(136)
void autoFormatReplaceOrdinals(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatReplaceFractions"
*
* @return Returns a value of type boolean
*/
@DISPID(257) //= 0x101. The runtime will prefer the VTID if present
@VTID(137)
boolean autoFormatReplaceFractions();
/**
*
* Setter method for the COM property "AutoFormatReplaceFractions"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(257) //= 0x101. The runtime will prefer the VTID if present
@VTID(138)
void autoFormatReplaceFractions(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatReplacePlainTextEmphasis"
*
* @return Returns a value of type boolean
*/
@DISPID(258) //= 0x102. The runtime will prefer the VTID if present
@VTID(139)
boolean autoFormatReplacePlainTextEmphasis();
/**
*
* Setter method for the COM property "AutoFormatReplacePlainTextEmphasis"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(258) //= 0x102. The runtime will prefer the VTID if present
@VTID(140)
void autoFormatReplacePlainTextEmphasis(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatPreserveStyles"
*
* @return Returns a value of type boolean
*/
@DISPID(259) //= 0x103. The runtime will prefer the VTID if present
@VTID(141)
boolean autoFormatPreserveStyles();
/**
*
* Setter method for the COM property "AutoFormatPreserveStyles"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(259) //= 0x103. The runtime will prefer the VTID if present
@VTID(142)
void autoFormatPreserveStyles(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeApplyHeadings"
*
* @return Returns a value of type boolean
*/
@DISPID(260) //= 0x104. The runtime will prefer the VTID if present
@VTID(143)
boolean autoFormatAsYouTypeApplyHeadings();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeApplyHeadings"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(260) //= 0x104. The runtime will prefer the VTID if present
@VTID(144)
void autoFormatAsYouTypeApplyHeadings(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeApplyBorders"
*
* @return Returns a value of type boolean
*/
@DISPID(261) //= 0x105. The runtime will prefer the VTID if present
@VTID(145)
boolean autoFormatAsYouTypeApplyBorders();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeApplyBorders"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(261) //= 0x105. The runtime will prefer the VTID if present
@VTID(146)
void autoFormatAsYouTypeApplyBorders(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeApplyBulletedLists"
*
* @return Returns a value of type boolean
*/
@DISPID(262) //= 0x106. The runtime will prefer the VTID if present
@VTID(147)
boolean autoFormatAsYouTypeApplyBulletedLists();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeApplyBulletedLists"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(262) //= 0x106. The runtime will prefer the VTID if present
@VTID(148)
void autoFormatAsYouTypeApplyBulletedLists(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeApplyNumberedLists"
*
* @return Returns a value of type boolean
*/
@DISPID(263) //= 0x107. The runtime will prefer the VTID if present
@VTID(149)
boolean autoFormatAsYouTypeApplyNumberedLists();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeApplyNumberedLists"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(263) //= 0x107. The runtime will prefer the VTID if present
@VTID(150)
void autoFormatAsYouTypeApplyNumberedLists(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeReplaceQuotes"
*
* @return Returns a value of type boolean
*/
@DISPID(264) //= 0x108. The runtime will prefer the VTID if present
@VTID(151)
boolean autoFormatAsYouTypeReplaceQuotes();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeReplaceQuotes"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(264) //= 0x108. The runtime will prefer the VTID if present
@VTID(152)
void autoFormatAsYouTypeReplaceQuotes(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeReplaceSymbols"
*
* @return Returns a value of type boolean
*/
@DISPID(265) //= 0x109. The runtime will prefer the VTID if present
@VTID(153)
boolean autoFormatAsYouTypeReplaceSymbols();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeReplaceSymbols"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(265) //= 0x109. The runtime will prefer the VTID if present
@VTID(154)
void autoFormatAsYouTypeReplaceSymbols(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeReplaceOrdinals"
*
* @return Returns a value of type boolean
*/
@DISPID(266) //= 0x10a. The runtime will prefer the VTID if present
@VTID(155)
boolean autoFormatAsYouTypeReplaceOrdinals();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeReplaceOrdinals"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(266) //= 0x10a. The runtime will prefer the VTID if present
@VTID(156)
void autoFormatAsYouTypeReplaceOrdinals(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeReplaceFractions"
*
* @return Returns a value of type boolean
*/
@DISPID(267) //= 0x10b. The runtime will prefer the VTID if present
@VTID(157)
boolean autoFormatAsYouTypeReplaceFractions();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeReplaceFractions"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(267) //= 0x10b. The runtime will prefer the VTID if present
@VTID(158)
void autoFormatAsYouTypeReplaceFractions(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeReplacePlainTextEmphasis"
*
* @return Returns a value of type boolean
*/
@DISPID(268) //= 0x10c. The runtime will prefer the VTID if present
@VTID(159)
boolean autoFormatAsYouTypeReplacePlainTextEmphasis();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeReplacePlainTextEmphasis"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(268) //= 0x10c. The runtime will prefer the VTID if present
@VTID(160)
void autoFormatAsYouTypeReplacePlainTextEmphasis(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeFormatListItemBeginning"
*
* @return Returns a value of type boolean
*/
@DISPID(269) //= 0x10d. The runtime will prefer the VTID if present
@VTID(161)
boolean autoFormatAsYouTypeFormatListItemBeginning();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeFormatListItemBeginning"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(269) //= 0x10d. The runtime will prefer the VTID if present
@VTID(162)
void autoFormatAsYouTypeFormatListItemBeginning(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeDefineStyles"
*
* @return Returns a value of type boolean
*/
@DISPID(270) //= 0x10e. The runtime will prefer the VTID if present
@VTID(163)
boolean autoFormatAsYouTypeDefineStyles();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeDefineStyles"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(270) //= 0x10e. The runtime will prefer the VTID if present
@VTID(164)
void autoFormatAsYouTypeDefineStyles(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatPlainTextWordMail"
*
* @return Returns a value of type boolean
*/
@DISPID(271) //= 0x10f. The runtime will prefer the VTID if present
@VTID(165)
boolean autoFormatPlainTextWordMail();
/**
*
* Setter method for the COM property "AutoFormatPlainTextWordMail"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(271) //= 0x10f. The runtime will prefer the VTID if present
@VTID(166)
void autoFormatPlainTextWordMail(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeReplaceHyperlinks"
*
* @return Returns a value of type boolean
*/
@DISPID(272) //= 0x110. The runtime will prefer the VTID if present
@VTID(167)
boolean autoFormatAsYouTypeReplaceHyperlinks();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeReplaceHyperlinks"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(272) //= 0x110. The runtime will prefer the VTID if present
@VTID(168)
void autoFormatAsYouTypeReplaceHyperlinks(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatReplaceHyperlinks"
*
* @return Returns a value of type boolean
*/
@DISPID(273) //= 0x111. The runtime will prefer the VTID if present
@VTID(169)
boolean autoFormatReplaceHyperlinks();
/**
*
* Setter method for the COM property "AutoFormatReplaceHyperlinks"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(273) //= 0x111. The runtime will prefer the VTID if present
@VTID(170)
void autoFormatReplaceHyperlinks(
boolean prop);
/**
*
* Getter method for the COM property "DefaultHighlightColorIndex"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdColorIndex
*/
@DISPID(274) //= 0x112. The runtime will prefer the VTID if present
@VTID(171)
net.rgielen.com4j.office2010.word.WdColorIndex defaultHighlightColorIndex();
/**
*
* Setter method for the COM property "DefaultHighlightColorIndex"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdColorIndex parameter.
*/
@DISPID(274) //= 0x112. The runtime will prefer the VTID if present
@VTID(172)
void defaultHighlightColorIndex(
net.rgielen.com4j.office2010.word.WdColorIndex prop);
/**
*
* Getter method for the COM property "DefaultBorderLineStyle"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdLineStyle
*/
@DISPID(275) //= 0x113. The runtime will prefer the VTID if present
@VTID(173)
net.rgielen.com4j.office2010.word.WdLineStyle defaultBorderLineStyle();
/**
*
* Setter method for the COM property "DefaultBorderLineStyle"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdLineStyle parameter.
*/
@DISPID(275) //= 0x113. The runtime will prefer the VTID if present
@VTID(174)
void defaultBorderLineStyle(
net.rgielen.com4j.office2010.word.WdLineStyle prop);
/**
*
* Getter method for the COM property "CheckSpellingAsYouType"
*
* @return Returns a value of type boolean
*/
@DISPID(276) //= 0x114. The runtime will prefer the VTID if present
@VTID(175)
boolean checkSpellingAsYouType();
/**
*
* Setter method for the COM property "CheckSpellingAsYouType"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(276) //= 0x114. The runtime will prefer the VTID if present
@VTID(176)
void checkSpellingAsYouType(
boolean prop);
/**
*
* Getter method for the COM property "CheckGrammarAsYouType"
*
* @return Returns a value of type boolean
*/
@DISPID(277) //= 0x115. The runtime will prefer the VTID if present
@VTID(177)
boolean checkGrammarAsYouType();
/**
*
* Setter method for the COM property "CheckGrammarAsYouType"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(277) //= 0x115. The runtime will prefer the VTID if present
@VTID(178)
void checkGrammarAsYouType(
boolean prop);
/**
*
* Getter method for the COM property "IgnoreInternetAndFileAddresses"
*
* @return Returns a value of type boolean
*/
@DISPID(278) //= 0x116. The runtime will prefer the VTID if present
@VTID(179)
boolean ignoreInternetAndFileAddresses();
/**
*
* Setter method for the COM property "IgnoreInternetAndFileAddresses"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(278) //= 0x116. The runtime will prefer the VTID if present
@VTID(180)
void ignoreInternetAndFileAddresses(
boolean prop);
/**
*
* Getter method for the COM property "ShowReadabilityStatistics"
*
* @return Returns a value of type boolean
*/
@DISPID(279) //= 0x117. The runtime will prefer the VTID if present
@VTID(181)
boolean showReadabilityStatistics();
/**
*
* Setter method for the COM property "ShowReadabilityStatistics"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(279) //= 0x117. The runtime will prefer the VTID if present
@VTID(182)
void showReadabilityStatistics(
boolean prop);
/**
*
* Getter method for the COM property "IgnoreUppercase"
*
* @return Returns a value of type boolean
*/
@DISPID(280) //= 0x118. The runtime will prefer the VTID if present
@VTID(183)
boolean ignoreUppercase();
/**
*
* Setter method for the COM property "IgnoreUppercase"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(280) //= 0x118. The runtime will prefer the VTID if present
@VTID(184)
void ignoreUppercase(
boolean prop);
/**
*
* Getter method for the COM property "IgnoreMixedDigits"
*
* @return Returns a value of type boolean
*/
@DISPID(281) //= 0x119. The runtime will prefer the VTID if present
@VTID(185)
boolean ignoreMixedDigits();
/**
*
* Setter method for the COM property "IgnoreMixedDigits"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(281) //= 0x119. The runtime will prefer the VTID if present
@VTID(186)
void ignoreMixedDigits(
boolean prop);
/**
*
* Getter method for the COM property "SuggestFromMainDictionaryOnly"
*
* @return Returns a value of type boolean
*/
@DISPID(282) //= 0x11a. The runtime will prefer the VTID if present
@VTID(187)
boolean suggestFromMainDictionaryOnly();
/**
*
* Setter method for the COM property "SuggestFromMainDictionaryOnly"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(282) //= 0x11a. The runtime will prefer the VTID if present
@VTID(188)
void suggestFromMainDictionaryOnly(
boolean prop);
/**
*
* Getter method for the COM property "SuggestSpellingCorrections"
*
* @return Returns a value of type boolean
*/
@DISPID(283) //= 0x11b. The runtime will prefer the VTID if present
@VTID(189)
boolean suggestSpellingCorrections();
/**
*
* Setter method for the COM property "SuggestSpellingCorrections"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(283) //= 0x11b. The runtime will prefer the VTID if present
@VTID(190)
void suggestSpellingCorrections(
boolean prop);
/**
*
* Getter method for the COM property "DefaultBorderLineWidth"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdLineWidth
*/
@DISPID(284) //= 0x11c. The runtime will prefer the VTID if present
@VTID(191)
net.rgielen.com4j.office2010.word.WdLineWidth defaultBorderLineWidth();
/**
*
* Setter method for the COM property "DefaultBorderLineWidth"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdLineWidth parameter.
*/
@DISPID(284) //= 0x11c. The runtime will prefer the VTID if present
@VTID(192)
void defaultBorderLineWidth(
net.rgielen.com4j.office2010.word.WdLineWidth prop);
/**
*
* Getter method for the COM property "CheckGrammarWithSpelling"
*
* @return Returns a value of type boolean
*/
@DISPID(285) //= 0x11d. The runtime will prefer the VTID if present
@VTID(193)
boolean checkGrammarWithSpelling();
/**
*
* Setter method for the COM property "CheckGrammarWithSpelling"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(285) //= 0x11d. The runtime will prefer the VTID if present
@VTID(194)
void checkGrammarWithSpelling(
boolean prop);
/**
*
* Getter method for the COM property "DefaultOpenFormat"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdOpenFormat
*/
@DISPID(286) //= 0x11e. The runtime will prefer the VTID if present
@VTID(195)
net.rgielen.com4j.office2010.word.WdOpenFormat defaultOpenFormat();
/**
*
* Setter method for the COM property "DefaultOpenFormat"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdOpenFormat parameter.
*/
@DISPID(286) //= 0x11e. The runtime will prefer the VTID if present
@VTID(196)
void defaultOpenFormat(
net.rgielen.com4j.office2010.word.WdOpenFormat prop);
/**
*
* Getter method for the COM property "PrintDraft"
*
* @return Returns a value of type boolean
*/
@DISPID(287) //= 0x11f. The runtime will prefer the VTID if present
@VTID(197)
boolean printDraft();
/**
*
* Setter method for the COM property "PrintDraft"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(287) //= 0x11f. The runtime will prefer the VTID if present
@VTID(198)
void printDraft(
boolean prop);
/**
*
* Getter method for the COM property "PrintReverse"
*
* @return Returns a value of type boolean
*/
@DISPID(288) //= 0x120. The runtime will prefer the VTID if present
@VTID(199)
boolean printReverse();
/**
*
* Setter method for the COM property "PrintReverse"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(288) //= 0x120. The runtime will prefer the VTID if present
@VTID(200)
void printReverse(
boolean prop);
/**
*
* Getter method for the COM property "MapPaperSize"
*
* @return Returns a value of type boolean
*/
@DISPID(289) //= 0x121. The runtime will prefer the VTID if present
@VTID(201)
boolean mapPaperSize();
/**
*
* Setter method for the COM property "MapPaperSize"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(289) //= 0x121. The runtime will prefer the VTID if present
@VTID(202)
void mapPaperSize(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeApplyTables"
*
* @return Returns a value of type boolean
*/
@DISPID(290) //= 0x122. The runtime will prefer the VTID if present
@VTID(203)
boolean autoFormatAsYouTypeApplyTables();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeApplyTables"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(290) //= 0x122. The runtime will prefer the VTID if present
@VTID(204)
void autoFormatAsYouTypeApplyTables(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatApplyFirstIndents"
*
* @return Returns a value of type boolean
*/
@DISPID(291) //= 0x123. The runtime will prefer the VTID if present
@VTID(205)
boolean autoFormatApplyFirstIndents();
/**
*
* Setter method for the COM property "AutoFormatApplyFirstIndents"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(291) //= 0x123. The runtime will prefer the VTID if present
@VTID(206)
void autoFormatApplyFirstIndents(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatMatchParentheses"
*
* @return Returns a value of type boolean
*/
@DISPID(294) //= 0x126. The runtime will prefer the VTID if present
@VTID(207)
boolean autoFormatMatchParentheses();
/**
*
* Setter method for the COM property "AutoFormatMatchParentheses"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(294) //= 0x126. The runtime will prefer the VTID if present
@VTID(208)
void autoFormatMatchParentheses(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatReplaceFarEastDashes"
*
* @return Returns a value of type boolean
*/
@DISPID(295) //= 0x127. The runtime will prefer the VTID if present
@VTID(209)
boolean autoFormatReplaceFarEastDashes();
/**
*
* Setter method for the COM property "AutoFormatReplaceFarEastDashes"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(295) //= 0x127. The runtime will prefer the VTID if present
@VTID(210)
void autoFormatReplaceFarEastDashes(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatDeleteAutoSpaces"
*
* @return Returns a value of type boolean
*/
@DISPID(296) //= 0x128. The runtime will prefer the VTID if present
@VTID(211)
boolean autoFormatDeleteAutoSpaces();
/**
*
* Setter method for the COM property "AutoFormatDeleteAutoSpaces"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(296) //= 0x128. The runtime will prefer the VTID if present
@VTID(212)
void autoFormatDeleteAutoSpaces(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeApplyFirstIndents"
*
* @return Returns a value of type boolean
*/
@DISPID(297) //= 0x129. The runtime will prefer the VTID if present
@VTID(213)
boolean autoFormatAsYouTypeApplyFirstIndents();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeApplyFirstIndents"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(297) //= 0x129. The runtime will prefer the VTID if present
@VTID(214)
void autoFormatAsYouTypeApplyFirstIndents(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeApplyDates"
*
* @return Returns a value of type boolean
*/
@DISPID(298) //= 0x12a. The runtime will prefer the VTID if present
@VTID(215)
boolean autoFormatAsYouTypeApplyDates();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeApplyDates"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(298) //= 0x12a. The runtime will prefer the VTID if present
@VTID(216)
void autoFormatAsYouTypeApplyDates(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeApplyClosings"
*
* @return Returns a value of type boolean
*/
@DISPID(299) //= 0x12b. The runtime will prefer the VTID if present
@VTID(217)
boolean autoFormatAsYouTypeApplyClosings();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeApplyClosings"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(299) //= 0x12b. The runtime will prefer the VTID if present
@VTID(218)
void autoFormatAsYouTypeApplyClosings(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeMatchParentheses"
*
* @return Returns a value of type boolean
*/
@DISPID(300) //= 0x12c. The runtime will prefer the VTID if present
@VTID(219)
boolean autoFormatAsYouTypeMatchParentheses();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeMatchParentheses"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(300) //= 0x12c. The runtime will prefer the VTID if present
@VTID(220)
void autoFormatAsYouTypeMatchParentheses(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeReplaceFarEastDashes"
*
* @return Returns a value of type boolean
*/
@DISPID(301) //= 0x12d. The runtime will prefer the VTID if present
@VTID(221)
boolean autoFormatAsYouTypeReplaceFarEastDashes();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeReplaceFarEastDashes"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(301) //= 0x12d. The runtime will prefer the VTID if present
@VTID(222)
void autoFormatAsYouTypeReplaceFarEastDashes(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeDeleteAutoSpaces"
*
* @return Returns a value of type boolean
*/
@DISPID(302) //= 0x12e. The runtime will prefer the VTID if present
@VTID(223)
boolean autoFormatAsYouTypeDeleteAutoSpaces();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeDeleteAutoSpaces"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(302) //= 0x12e. The runtime will prefer the VTID if present
@VTID(224)
void autoFormatAsYouTypeDeleteAutoSpaces(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeInsertClosings"
*
* @return Returns a value of type boolean
*/
@DISPID(303) //= 0x12f. The runtime will prefer the VTID if present
@VTID(225)
boolean autoFormatAsYouTypeInsertClosings();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeInsertClosings"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(303) //= 0x12f. The runtime will prefer the VTID if present
@VTID(226)
void autoFormatAsYouTypeInsertClosings(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeAutoLetterWizard"
*
* @return Returns a value of type boolean
*/
@DISPID(304) //= 0x130. The runtime will prefer the VTID if present
@VTID(227)
boolean autoFormatAsYouTypeAutoLetterWizard();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeAutoLetterWizard"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(304) //= 0x130. The runtime will prefer the VTID if present
@VTID(228)
void autoFormatAsYouTypeAutoLetterWizard(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatAsYouTypeInsertOvers"
*
* @return Returns a value of type boolean
*/
@DISPID(305) //= 0x131. The runtime will prefer the VTID if present
@VTID(229)
boolean autoFormatAsYouTypeInsertOvers();
/**
*
* Setter method for the COM property "AutoFormatAsYouTypeInsertOvers"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(305) //= 0x131. The runtime will prefer the VTID if present
@VTID(230)
void autoFormatAsYouTypeInsertOvers(
boolean prop);
/**
*
* Getter method for the COM property "DisplayGridLines"
*
* @return Returns a value of type boolean
*/
@DISPID(306) //= 0x132. The runtime will prefer the VTID if present
@VTID(231)
boolean displayGridLines();
/**
*
* Setter method for the COM property "DisplayGridLines"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(306) //= 0x132. The runtime will prefer the VTID if present
@VTID(232)
void displayGridLines(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyCase"
*
* @return Returns a value of type boolean
*/
@DISPID(309) //= 0x135. The runtime will prefer the VTID if present
@VTID(233)
boolean matchFuzzyCase();
/**
*
* Setter method for the COM property "MatchFuzzyCase"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(309) //= 0x135. The runtime will prefer the VTID if present
@VTID(234)
void matchFuzzyCase(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyByte"
*
* @return Returns a value of type boolean
*/
@DISPID(310) //= 0x136. The runtime will prefer the VTID if present
@VTID(235)
boolean matchFuzzyByte();
/**
*
* Setter method for the COM property "MatchFuzzyByte"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(310) //= 0x136. The runtime will prefer the VTID if present
@VTID(236)
void matchFuzzyByte(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyHiragana"
*
* @return Returns a value of type boolean
*/
@DISPID(311) //= 0x137. The runtime will prefer the VTID if present
@VTID(237)
boolean matchFuzzyHiragana();
/**
*
* Setter method for the COM property "MatchFuzzyHiragana"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(311) //= 0x137. The runtime will prefer the VTID if present
@VTID(238)
void matchFuzzyHiragana(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzySmallKana"
*
* @return Returns a value of type boolean
*/
@DISPID(312) //= 0x138. The runtime will prefer the VTID if present
@VTID(239)
boolean matchFuzzySmallKana();
/**
*
* Setter method for the COM property "MatchFuzzySmallKana"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(312) //= 0x138. The runtime will prefer the VTID if present
@VTID(240)
void matchFuzzySmallKana(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyDash"
*
* @return Returns a value of type boolean
*/
@DISPID(313) //= 0x139. The runtime will prefer the VTID if present
@VTID(241)
boolean matchFuzzyDash();
/**
*
* Setter method for the COM property "MatchFuzzyDash"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(313) //= 0x139. The runtime will prefer the VTID if present
@VTID(242)
void matchFuzzyDash(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyIterationMark"
*
* @return Returns a value of type boolean
*/
@DISPID(314) //= 0x13a. The runtime will prefer the VTID if present
@VTID(243)
boolean matchFuzzyIterationMark();
/**
*
* Setter method for the COM property "MatchFuzzyIterationMark"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(314) //= 0x13a. The runtime will prefer the VTID if present
@VTID(244)
void matchFuzzyIterationMark(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyKanji"
*
* @return Returns a value of type boolean
*/
@DISPID(315) //= 0x13b. The runtime will prefer the VTID if present
@VTID(245)
boolean matchFuzzyKanji();
/**
*
* Setter method for the COM property "MatchFuzzyKanji"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(315) //= 0x13b. The runtime will prefer the VTID if present
@VTID(246)
void matchFuzzyKanji(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyOldKana"
*
* @return Returns a value of type boolean
*/
@DISPID(316) //= 0x13c. The runtime will prefer the VTID if present
@VTID(247)
boolean matchFuzzyOldKana();
/**
*
* Setter method for the COM property "MatchFuzzyOldKana"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(316) //= 0x13c. The runtime will prefer the VTID if present
@VTID(248)
void matchFuzzyOldKana(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyProlongedSoundMark"
*
* @return Returns a value of type boolean
*/
@DISPID(317) //= 0x13d. The runtime will prefer the VTID if present
@VTID(249)
boolean matchFuzzyProlongedSoundMark();
/**
*
* Setter method for the COM property "MatchFuzzyProlongedSoundMark"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(317) //= 0x13d. The runtime will prefer the VTID if present
@VTID(250)
void matchFuzzyProlongedSoundMark(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyDZ"
*
* @return Returns a value of type boolean
*/
@DISPID(318) //= 0x13e. The runtime will prefer the VTID if present
@VTID(251)
boolean matchFuzzyDZ();
/**
*
* Setter method for the COM property "MatchFuzzyDZ"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(318) //= 0x13e. The runtime will prefer the VTID if present
@VTID(252)
void matchFuzzyDZ(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyBV"
*
* @return Returns a value of type boolean
*/
@DISPID(319) //= 0x13f. The runtime will prefer the VTID if present
@VTID(253)
boolean matchFuzzyBV();
/**
*
* Setter method for the COM property "MatchFuzzyBV"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(319) //= 0x13f. The runtime will prefer the VTID if present
@VTID(254)
void matchFuzzyBV(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyTC"
*
* @return Returns a value of type boolean
*/
@DISPID(320) //= 0x140. The runtime will prefer the VTID if present
@VTID(255)
boolean matchFuzzyTC();
/**
*
* Setter method for the COM property "MatchFuzzyTC"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(320) //= 0x140. The runtime will prefer the VTID if present
@VTID(256)
void matchFuzzyTC(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyHF"
*
* @return Returns a value of type boolean
*/
@DISPID(321) //= 0x141. The runtime will prefer the VTID if present
@VTID(257)
boolean matchFuzzyHF();
/**
*
* Setter method for the COM property "MatchFuzzyHF"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(321) //= 0x141. The runtime will prefer the VTID if present
@VTID(258)
void matchFuzzyHF(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyZJ"
*
* @return Returns a value of type boolean
*/
@DISPID(322) //= 0x142. The runtime will prefer the VTID if present
@VTID(259)
boolean matchFuzzyZJ();
/**
*
* Setter method for the COM property "MatchFuzzyZJ"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(322) //= 0x142. The runtime will prefer the VTID if present
@VTID(260)
void matchFuzzyZJ(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyAY"
*
* @return Returns a value of type boolean
*/
@DISPID(323) //= 0x143. The runtime will prefer the VTID if present
@VTID(261)
boolean matchFuzzyAY();
/**
*
* Setter method for the COM property "MatchFuzzyAY"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(323) //= 0x143. The runtime will prefer the VTID if present
@VTID(262)
void matchFuzzyAY(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyKiKu"
*
* @return Returns a value of type boolean
*/
@DISPID(324) //= 0x144. The runtime will prefer the VTID if present
@VTID(263)
boolean matchFuzzyKiKu();
/**
*
* Setter method for the COM property "MatchFuzzyKiKu"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(324) //= 0x144. The runtime will prefer the VTID if present
@VTID(264)
void matchFuzzyKiKu(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzyPunctuation"
*
* @return Returns a value of type boolean
*/
@DISPID(325) //= 0x145. The runtime will prefer the VTID if present
@VTID(265)
boolean matchFuzzyPunctuation();
/**
*
* Setter method for the COM property "MatchFuzzyPunctuation"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(325) //= 0x145. The runtime will prefer the VTID if present
@VTID(266)
void matchFuzzyPunctuation(
boolean prop);
/**
*
* Getter method for the COM property "MatchFuzzySpace"
*
* @return Returns a value of type boolean
*/
@DISPID(326) //= 0x146. The runtime will prefer the VTID if present
@VTID(267)
boolean matchFuzzySpace();
/**
*
* Setter method for the COM property "MatchFuzzySpace"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(326) //= 0x146. The runtime will prefer the VTID if present
@VTID(268)
void matchFuzzySpace(
boolean prop);
/**
*
* Getter method for the COM property "ApplyFarEastFontsToAscii"
*
* @return Returns a value of type boolean
*/
@DISPID(327) //= 0x147. The runtime will prefer the VTID if present
@VTID(269)
boolean applyFarEastFontsToAscii();
/**
*
* Setter method for the COM property "ApplyFarEastFontsToAscii"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(327) //= 0x147. The runtime will prefer the VTID if present
@VTID(270)
void applyFarEastFontsToAscii(
boolean prop);
/**
*
* Getter method for the COM property "ConvertHighAnsiToFarEast"
*
* @return Returns a value of type boolean
*/
@DISPID(328) //= 0x148. The runtime will prefer the VTID if present
@VTID(271)
boolean convertHighAnsiToFarEast();
/**
*
* Setter method for the COM property "ConvertHighAnsiToFarEast"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(328) //= 0x148. The runtime will prefer the VTID if present
@VTID(272)
void convertHighAnsiToFarEast(
boolean prop);
/**
*
* Getter method for the COM property "PrintOddPagesInAscendingOrder"
*
* @return Returns a value of type boolean
*/
@DISPID(330) //= 0x14a. The runtime will prefer the VTID if present
@VTID(273)
boolean printOddPagesInAscendingOrder();
/**
*
* Setter method for the COM property "PrintOddPagesInAscendingOrder"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(330) //= 0x14a. The runtime will prefer the VTID if present
@VTID(274)
void printOddPagesInAscendingOrder(
boolean prop);
/**
*
* Getter method for the COM property "PrintEvenPagesInAscendingOrder"
*
* @return Returns a value of type boolean
*/
@DISPID(331) //= 0x14b. The runtime will prefer the VTID if present
@VTID(275)
boolean printEvenPagesInAscendingOrder();
/**
*
* Setter method for the COM property "PrintEvenPagesInAscendingOrder"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(331) //= 0x14b. The runtime will prefer the VTID if present
@VTID(276)
void printEvenPagesInAscendingOrder(
boolean prop);
/**
*
* Getter method for the COM property "DefaultBorderColorIndex"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdColorIndex
*/
@DISPID(337) //= 0x151. The runtime will prefer the VTID if present
@VTID(277)
net.rgielen.com4j.office2010.word.WdColorIndex defaultBorderColorIndex();
/**
*
* Setter method for the COM property "DefaultBorderColorIndex"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdColorIndex parameter.
*/
@DISPID(337) //= 0x151. The runtime will prefer the VTID if present
@VTID(278)
void defaultBorderColorIndex(
net.rgielen.com4j.office2010.word.WdColorIndex prop);
/**
*
* Getter method for the COM property "EnableMisusedWordsDictionary"
*
* @return Returns a value of type boolean
*/
@DISPID(338) //= 0x152. The runtime will prefer the VTID if present
@VTID(279)
boolean enableMisusedWordsDictionary();
/**
*
* Setter method for the COM property "EnableMisusedWordsDictionary"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(338) //= 0x152. The runtime will prefer the VTID if present
@VTID(280)
void enableMisusedWordsDictionary(
boolean prop);
/**
*
* Getter method for the COM property "AllowCombinedAuxiliaryForms"
*
* @return Returns a value of type boolean
*/
@DISPID(339) //= 0x153. The runtime will prefer the VTID if present
@VTID(281)
boolean allowCombinedAuxiliaryForms();
/**
*
* Setter method for the COM property "AllowCombinedAuxiliaryForms"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(339) //= 0x153. The runtime will prefer the VTID if present
@VTID(282)
void allowCombinedAuxiliaryForms(
boolean prop);
/**
*
* Getter method for the COM property "HangulHanjaFastConversion"
*
* @return Returns a value of type boolean
*/
@DISPID(340) //= 0x154. The runtime will prefer the VTID if present
@VTID(283)
boolean hangulHanjaFastConversion();
/**
*
* Setter method for the COM property "HangulHanjaFastConversion"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(340) //= 0x154. The runtime will prefer the VTID if present
@VTID(284)
void hangulHanjaFastConversion(
boolean prop);
/**
*
* Getter method for the COM property "CheckHangulEndings"
*
* @return Returns a value of type boolean
*/
@DISPID(341) //= 0x155. The runtime will prefer the VTID if present
@VTID(285)
boolean checkHangulEndings();
/**
*
* Setter method for the COM property "CheckHangulEndings"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(341) //= 0x155. The runtime will prefer the VTID if present
@VTID(286)
void checkHangulEndings(
boolean prop);
/**
*
* Getter method for the COM property "EnableHangulHanjaRecentOrdering"
*
* @return Returns a value of type boolean
*/
@DISPID(342) //= 0x156. The runtime will prefer the VTID if present
@VTID(287)
boolean enableHangulHanjaRecentOrdering();
/**
*
* Setter method for the COM property "EnableHangulHanjaRecentOrdering"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(342) //= 0x156. The runtime will prefer the VTID if present
@VTID(288)
void enableHangulHanjaRecentOrdering(
boolean prop);
/**
*
* Getter method for the COM property "MultipleWordConversionsMode"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdMultipleWordConversionsMode
*/
@DISPID(343) //= 0x157. The runtime will prefer the VTID if present
@VTID(289)
net.rgielen.com4j.office2010.word.WdMultipleWordConversionsMode multipleWordConversionsMode();
/**
*
* Setter method for the COM property "MultipleWordConversionsMode"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdMultipleWordConversionsMode parameter.
*/
@DISPID(343) //= 0x157. The runtime will prefer the VTID if present
@VTID(290)
void multipleWordConversionsMode(
net.rgielen.com4j.office2010.word.WdMultipleWordConversionsMode prop);
/**
* @param commandKeyHelp Optional parameter. Default value is com4j.Variant.getMissing()
* @param docNavigationKeys Optional parameter. Default value is com4j.Variant.getMissing()
* @param mouseSimulation Optional parameter. Default value is com4j.Variant.getMissing()
* @param demoGuidance Optional parameter. Default value is com4j.Variant.getMissing()
* @param demoSpeed Optional parameter. Default value is com4j.Variant.getMissing()
* @param helpType Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(333) //= 0x14d. The runtime will prefer the VTID if present
@VTID(291)
void setWPHelpOptions(
@Optional java.lang.Object commandKeyHelp,
@Optional java.lang.Object docNavigationKeys,
@Optional java.lang.Object mouseSimulation,
@Optional java.lang.Object demoGuidance,
@Optional java.lang.Object demoSpeed,
@Optional java.lang.Object helpType);
/**
*
* Getter method for the COM property "DefaultBorderColor"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdColor
*/
@DISPID(344) //= 0x158. The runtime will prefer the VTID if present
@VTID(292)
net.rgielen.com4j.office2010.word.WdColor defaultBorderColor();
/**
*
* Setter method for the COM property "DefaultBorderColor"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdColor parameter.
*/
@DISPID(344) //= 0x158. The runtime will prefer the VTID if present
@VTID(293)
void defaultBorderColor(
net.rgielen.com4j.office2010.word.WdColor prop);
/**
*
* Getter method for the COM property "AllowPixelUnits"
*
* @return Returns a value of type boolean
*/
@DISPID(345) //= 0x159. The runtime will prefer the VTID if present
@VTID(294)
boolean allowPixelUnits();
/**
*
* Setter method for the COM property "AllowPixelUnits"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(345) //= 0x159. The runtime will prefer the VTID if present
@VTID(295)
void allowPixelUnits(
boolean prop);
/**
*
* Getter method for the COM property "UseCharacterUnit"
*
* @return Returns a value of type boolean
*/
@DISPID(346) //= 0x15a. The runtime will prefer the VTID if present
@VTID(296)
boolean useCharacterUnit();
/**
*
* Setter method for the COM property "UseCharacterUnit"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(346) //= 0x15a. The runtime will prefer the VTID if present
@VTID(297)
void useCharacterUnit(
boolean prop);
/**
*
* Getter method for the COM property "AllowCompoundNounProcessing"
*
* @return Returns a value of type boolean
*/
@DISPID(347) //= 0x15b. The runtime will prefer the VTID if present
@VTID(298)
boolean allowCompoundNounProcessing();
/**
*
* Setter method for the COM property "AllowCompoundNounProcessing"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(347) //= 0x15b. The runtime will prefer the VTID if present
@VTID(299)
void allowCompoundNounProcessing(
boolean prop);
/**
*
* Getter method for the COM property "AutoKeyboardSwitching"
*
* @return Returns a value of type boolean
*/
@DISPID(399) //= 0x18f. The runtime will prefer the VTID if present
@VTID(300)
boolean autoKeyboardSwitching();
/**
*
* Setter method for the COM property "AutoKeyboardSwitching"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(399) //= 0x18f. The runtime will prefer the VTID if present
@VTID(301)
void autoKeyboardSwitching(
boolean prop);
/**
*
* Getter method for the COM property "DocumentViewDirection"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdDocumentViewDirection
*/
@DISPID(400) //= 0x190. The runtime will prefer the VTID if present
@VTID(302)
net.rgielen.com4j.office2010.word.WdDocumentViewDirection documentViewDirection();
/**
*
* Setter method for the COM property "DocumentViewDirection"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdDocumentViewDirection parameter.
*/
@DISPID(400) //= 0x190. The runtime will prefer the VTID if present
@VTID(303)
void documentViewDirection(
net.rgielen.com4j.office2010.word.WdDocumentViewDirection prop);
/**
*
* Getter method for the COM property "ArabicNumeral"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdArabicNumeral
*/
@DISPID(401) //= 0x191. The runtime will prefer the VTID if present
@VTID(304)
net.rgielen.com4j.office2010.word.WdArabicNumeral arabicNumeral();
/**
*
* Setter method for the COM property "ArabicNumeral"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdArabicNumeral parameter.
*/
@DISPID(401) //= 0x191. The runtime will prefer the VTID if present
@VTID(305)
void arabicNumeral(
net.rgielen.com4j.office2010.word.WdArabicNumeral prop);
/**
*
* Getter method for the COM property "MonthNames"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdMonthNames
*/
@DISPID(402) //= 0x192. The runtime will prefer the VTID if present
@VTID(306)
net.rgielen.com4j.office2010.word.WdMonthNames monthNames();
/**
*
* Setter method for the COM property "MonthNames"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdMonthNames parameter.
*/
@DISPID(402) //= 0x192. The runtime will prefer the VTID if present
@VTID(307)
void monthNames(
net.rgielen.com4j.office2010.word.WdMonthNames prop);
/**
*
* Getter method for the COM property "CursorMovement"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdCursorMovement
*/
@DISPID(403) //= 0x193. The runtime will prefer the VTID if present
@VTID(308)
net.rgielen.com4j.office2010.word.WdCursorMovement cursorMovement();
/**
*
* Setter method for the COM property "CursorMovement"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdCursorMovement parameter.
*/
@DISPID(403) //= 0x193. The runtime will prefer the VTID if present
@VTID(309)
void cursorMovement(
net.rgielen.com4j.office2010.word.WdCursorMovement prop);
/**
*
* Getter method for the COM property "VisualSelection"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdVisualSelection
*/
@DISPID(404) //= 0x194. The runtime will prefer the VTID if present
@VTID(310)
net.rgielen.com4j.office2010.word.WdVisualSelection visualSelection();
/**
*
* Setter method for the COM property "VisualSelection"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdVisualSelection parameter.
*/
@DISPID(404) //= 0x194. The runtime will prefer the VTID if present
@VTID(311)
void visualSelection(
net.rgielen.com4j.office2010.word.WdVisualSelection prop);
/**
*
* Getter method for the COM property "ShowDiacritics"
*
* @return Returns a value of type boolean
*/
@DISPID(405) //= 0x195. The runtime will prefer the VTID if present
@VTID(312)
boolean showDiacritics();
/**
*
* Setter method for the COM property "ShowDiacritics"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(405) //= 0x195. The runtime will prefer the VTID if present
@VTID(313)
void showDiacritics(
boolean prop);
/**
*
* Getter method for the COM property "ShowControlCharacters"
*
* @return Returns a value of type boolean
*/
@DISPID(406) //= 0x196. The runtime will prefer the VTID if present
@VTID(314)
boolean showControlCharacters();
/**
*
* Setter method for the COM property "ShowControlCharacters"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(406) //= 0x196. The runtime will prefer the VTID if present
@VTID(315)
void showControlCharacters(
boolean prop);
/**
*
* Getter method for the COM property "AddControlCharacters"
*
* @return Returns a value of type boolean
*/
@DISPID(407) //= 0x197. The runtime will prefer the VTID if present
@VTID(316)
boolean addControlCharacters();
/**
*
* Setter method for the COM property "AddControlCharacters"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(407) //= 0x197. The runtime will prefer the VTID if present
@VTID(317)
void addControlCharacters(
boolean prop);
/**
*
* Getter method for the COM property "AddBiDirectionalMarksWhenSavingTextFile"
*
* @return Returns a value of type boolean
*/
@DISPID(408) //= 0x198. The runtime will prefer the VTID if present
@VTID(318)
boolean addBiDirectionalMarksWhenSavingTextFile();
/**
*
* Setter method for the COM property "AddBiDirectionalMarksWhenSavingTextFile"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(408) //= 0x198. The runtime will prefer the VTID if present
@VTID(319)
void addBiDirectionalMarksWhenSavingTextFile(
boolean prop);
/**
*
* Getter method for the COM property "StrictInitialAlefHamza"
*
* @return Returns a value of type boolean
*/
@DISPID(409) //= 0x199. The runtime will prefer the VTID if present
@VTID(320)
boolean strictInitialAlefHamza();
/**
*
* Setter method for the COM property "StrictInitialAlefHamza"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(409) //= 0x199. The runtime will prefer the VTID if present
@VTID(321)
void strictInitialAlefHamza(
boolean prop);
/**
*
* Getter method for the COM property "StrictFinalYaa"
*
* @return Returns a value of type boolean
*/
@DISPID(410) //= 0x19a. The runtime will prefer the VTID if present
@VTID(322)
boolean strictFinalYaa();
/**
*
* Setter method for the COM property "StrictFinalYaa"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(410) //= 0x19a. The runtime will prefer the VTID if present
@VTID(323)
void strictFinalYaa(
boolean prop);
/**
*
* Getter method for the COM property "HebrewMode"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdHebSpellStart
*/
@DISPID(411) //= 0x19b. The runtime will prefer the VTID if present
@VTID(324)
net.rgielen.com4j.office2010.word.WdHebSpellStart hebrewMode();
/**
*
* Setter method for the COM property "HebrewMode"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdHebSpellStart parameter.
*/
@DISPID(411) //= 0x19b. The runtime will prefer the VTID if present
@VTID(325)
void hebrewMode(
net.rgielen.com4j.office2010.word.WdHebSpellStart prop);
/**
*
* Getter method for the COM property "ArabicMode"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdAraSpeller
*/
@DISPID(412) //= 0x19c. The runtime will prefer the VTID if present
@VTID(326)
net.rgielen.com4j.office2010.word.WdAraSpeller arabicMode();
/**
*
* Setter method for the COM property "ArabicMode"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdAraSpeller parameter.
*/
@DISPID(412) //= 0x19c. The runtime will prefer the VTID if present
@VTID(327)
void arabicMode(
net.rgielen.com4j.office2010.word.WdAraSpeller prop);
/**
*
* Getter method for the COM property "AllowClickAndTypeMouse"
*
* @return Returns a value of type boolean
*/
@DISPID(413) //= 0x19d. The runtime will prefer the VTID if present
@VTID(328)
boolean allowClickAndTypeMouse();
/**
*
* Setter method for the COM property "AllowClickAndTypeMouse"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(413) //= 0x19d. The runtime will prefer the VTID if present
@VTID(329)
void allowClickAndTypeMouse(
boolean prop);
/**
*
* Getter method for the COM property "UseGermanSpellingReform"
*
* @return Returns a value of type boolean
*/
@DISPID(415) //= 0x19f. The runtime will prefer the VTID if present
@VTID(330)
boolean useGermanSpellingReform();
/**
*
* Setter method for the COM property "UseGermanSpellingReform"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(415) //= 0x19f. The runtime will prefer the VTID if present
@VTID(331)
void useGermanSpellingReform(
boolean prop);
/**
*
* Getter method for the COM property "InterpretHighAnsi"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdHighAnsiText
*/
@DISPID(418) //= 0x1a2. The runtime will prefer the VTID if present
@VTID(332)
net.rgielen.com4j.office2010.word.WdHighAnsiText interpretHighAnsi();
/**
*
* Setter method for the COM property "InterpretHighAnsi"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdHighAnsiText parameter.
*/
@DISPID(418) //= 0x1a2. The runtime will prefer the VTID if present
@VTID(333)
void interpretHighAnsi(
net.rgielen.com4j.office2010.word.WdHighAnsiText prop);
/**
*
* Getter method for the COM property "AddHebDoubleQuote"
*
* @return Returns a value of type boolean
*/
@DISPID(419) //= 0x1a3. The runtime will prefer the VTID if present
@VTID(334)
boolean addHebDoubleQuote();
/**
*
* Setter method for the COM property "AddHebDoubleQuote"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(419) //= 0x1a3. The runtime will prefer the VTID if present
@VTID(335)
void addHebDoubleQuote(
boolean prop);
/**
*
* Getter method for the COM property "UseDiffDiacColor"
*
* @return Returns a value of type boolean
*/
@DISPID(420) //= 0x1a4. The runtime will prefer the VTID if present
@VTID(336)
boolean useDiffDiacColor();
/**
*
* Setter method for the COM property "UseDiffDiacColor"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(420) //= 0x1a4. The runtime will prefer the VTID if present
@VTID(337)
void useDiffDiacColor(
boolean prop);
/**
*
* Getter method for the COM property "DiacriticColorVal"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdColor
*/
@DISPID(421) //= 0x1a5. The runtime will prefer the VTID if present
@VTID(338)
net.rgielen.com4j.office2010.word.WdColor diacriticColorVal();
/**
*
* Setter method for the COM property "DiacriticColorVal"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdColor parameter.
*/
@DISPID(421) //= 0x1a5. The runtime will prefer the VTID if present
@VTID(339)
void diacriticColorVal(
net.rgielen.com4j.office2010.word.WdColor prop);
/**
*
* Getter method for the COM property "OptimizeForWord97byDefault"
*
* @return Returns a value of type boolean
*/
@DISPID(423) //= 0x1a7. The runtime will prefer the VTID if present
@VTID(340)
boolean optimizeForWord97byDefault();
/**
*
* Setter method for the COM property "OptimizeForWord97byDefault"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(423) //= 0x1a7. The runtime will prefer the VTID if present
@VTID(341)
void optimizeForWord97byDefault(
boolean prop);
/**
*
* Getter method for the COM property "LocalNetworkFile"
*
* @return Returns a value of type boolean
*/
@DISPID(424) //= 0x1a8. The runtime will prefer the VTID if present
@VTID(342)
boolean localNetworkFile();
/**
*
* Setter method for the COM property "LocalNetworkFile"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(424) //= 0x1a8. The runtime will prefer the VTID if present
@VTID(343)
void localNetworkFile(
boolean prop);
/**
*
* Getter method for the COM property "TypeNReplace"
*
* @return Returns a value of type boolean
*/
@DISPID(425) //= 0x1a9. The runtime will prefer the VTID if present
@VTID(344)
boolean typeNReplace();
/**
*
* Setter method for the COM property "TypeNReplace"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(425) //= 0x1a9. The runtime will prefer the VTID if present
@VTID(345)
void typeNReplace(
boolean prop);
/**
*
* Getter method for the COM property "SequenceCheck"
*
* @return Returns a value of type boolean
*/
@DISPID(426) //= 0x1aa. The runtime will prefer the VTID if present
@VTID(346)
boolean sequenceCheck();
/**
*
* Setter method for the COM property "SequenceCheck"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(426) //= 0x1aa. The runtime will prefer the VTID if present
@VTID(347)
void sequenceCheck(
boolean prop);
/**
*
* Getter method for the COM property "BackgroundOpen"
*
* @return Returns a value of type boolean
*/
@DISPID(427) //= 0x1ab. The runtime will prefer the VTID if present
@VTID(348)
boolean backgroundOpen();
/**
*
* Setter method for the COM property "BackgroundOpen"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(427) //= 0x1ab. The runtime will prefer the VTID if present
@VTID(349)
void backgroundOpen(
boolean prop);
/**
*
* Getter method for the COM property "DisableFeaturesbyDefault"
*
* @return Returns a value of type boolean
*/
@DISPID(428) //= 0x1ac. The runtime will prefer the VTID if present
@VTID(350)
boolean disableFeaturesbyDefault();
/**
*
* Setter method for the COM property "DisableFeaturesbyDefault"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(428) //= 0x1ac. The runtime will prefer the VTID if present
@VTID(351)
void disableFeaturesbyDefault(
boolean prop);
/**
*
* Getter method for the COM property "PasteAdjustWordSpacing"
*
* @return Returns a value of type boolean
*/
@DISPID(429) //= 0x1ad. The runtime will prefer the VTID if present
@VTID(352)
boolean pasteAdjustWordSpacing();
/**
*
* Setter method for the COM property "PasteAdjustWordSpacing"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(429) //= 0x1ad. The runtime will prefer the VTID if present
@VTID(353)
void pasteAdjustWordSpacing(
boolean prop);
/**
*
* Getter method for the COM property "PasteAdjustParagraphSpacing"
*
* @return Returns a value of type boolean
*/
@DISPID(430) //= 0x1ae. The runtime will prefer the VTID if present
@VTID(354)
boolean pasteAdjustParagraphSpacing();
/**
*
* Setter method for the COM property "PasteAdjustParagraphSpacing"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(430) //= 0x1ae. The runtime will prefer the VTID if present
@VTID(355)
void pasteAdjustParagraphSpacing(
boolean prop);
/**
*
* Getter method for the COM property "PasteAdjustTableFormatting"
*
* @return Returns a value of type boolean
*/
@DISPID(431) //= 0x1af. The runtime will prefer the VTID if present
@VTID(356)
boolean pasteAdjustTableFormatting();
/**
*
* Setter method for the COM property "PasteAdjustTableFormatting"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(431) //= 0x1af. The runtime will prefer the VTID if present
@VTID(357)
void pasteAdjustTableFormatting(
boolean prop);
/**
*
* Getter method for the COM property "PasteSmartStyleBehavior"
*
* @return Returns a value of type boolean
*/
@DISPID(432) //= 0x1b0. The runtime will prefer the VTID if present
@VTID(358)
boolean pasteSmartStyleBehavior();
/**
*
* Setter method for the COM property "PasteSmartStyleBehavior"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(432) //= 0x1b0. The runtime will prefer the VTID if present
@VTID(359)
void pasteSmartStyleBehavior(
boolean prop);
/**
*
* Getter method for the COM property "PasteMergeFromPPT"
*
* @return Returns a value of type boolean
*/
@DISPID(433) //= 0x1b1. The runtime will prefer the VTID if present
@VTID(360)
boolean pasteMergeFromPPT();
/**
*
* Setter method for the COM property "PasteMergeFromPPT"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(433) //= 0x1b1. The runtime will prefer the VTID if present
@VTID(361)
void pasteMergeFromPPT(
boolean prop);
/**
*
* Getter method for the COM property "PasteMergeFromXL"
*
* @return Returns a value of type boolean
*/
@DISPID(434) //= 0x1b2. The runtime will prefer the VTID if present
@VTID(362)
boolean pasteMergeFromXL();
/**
*
* Setter method for the COM property "PasteMergeFromXL"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(434) //= 0x1b2. The runtime will prefer the VTID if present
@VTID(363)
void pasteMergeFromXL(
boolean prop);
/**
*
* Getter method for the COM property "CtrlClickHyperlinkToOpen"
*
* @return Returns a value of type boolean
*/
@DISPID(435) //= 0x1b3. The runtime will prefer the VTID if present
@VTID(364)
boolean ctrlClickHyperlinkToOpen();
/**
*
* Setter method for the COM property "CtrlClickHyperlinkToOpen"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(435) //= 0x1b3. The runtime will prefer the VTID if present
@VTID(365)
void ctrlClickHyperlinkToOpen(
boolean prop);
/**
*
* Getter method for the COM property "PictureWrapType"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdWrapTypeMerged
*/
@DISPID(436) //= 0x1b4. The runtime will prefer the VTID if present
@VTID(366)
net.rgielen.com4j.office2010.word.WdWrapTypeMerged pictureWrapType();
/**
*
* Setter method for the COM property "PictureWrapType"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdWrapTypeMerged parameter.
*/
@DISPID(436) //= 0x1b4. The runtime will prefer the VTID if present
@VTID(367)
void pictureWrapType(
net.rgielen.com4j.office2010.word.WdWrapTypeMerged prop);
/**
*
* Getter method for the COM property "DisableFeaturesIntroducedAfterbyDefault"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdDisableFeaturesIntroducedAfter
*/
@DISPID(437) //= 0x1b5. The runtime will prefer the VTID if present
@VTID(368)
net.rgielen.com4j.office2010.word.WdDisableFeaturesIntroducedAfter disableFeaturesIntroducedAfterbyDefault();
/**
*
* Setter method for the COM property "DisableFeaturesIntroducedAfterbyDefault"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdDisableFeaturesIntroducedAfter parameter.
*/
@DISPID(437) //= 0x1b5. The runtime will prefer the VTID if present
@VTID(369)
void disableFeaturesIntroducedAfterbyDefault(
net.rgielen.com4j.office2010.word.WdDisableFeaturesIntroducedAfter prop);
/**
*
* Getter method for the COM property "PasteSmartCutPaste"
*
* @return Returns a value of type boolean
*/
@DISPID(438) //= 0x1b6. The runtime will prefer the VTID if present
@VTID(370)
boolean pasteSmartCutPaste();
/**
*
* Setter method for the COM property "PasteSmartCutPaste"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(438) //= 0x1b6. The runtime will prefer the VTID if present
@VTID(371)
void pasteSmartCutPaste(
boolean prop);
/**
*
* Getter method for the COM property "DisplayPasteOptions"
*
* @return Returns a value of type boolean
*/
@DISPID(439) //= 0x1b7. The runtime will prefer the VTID if present
@VTID(372)
boolean displayPasteOptions();
/**
*
* Setter method for the COM property "DisplayPasteOptions"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(439) //= 0x1b7. The runtime will prefer the VTID if present
@VTID(373)
void displayPasteOptions(
boolean prop);
/**
*
* Getter method for the COM property "PromptUpdateStyle"
*
* @return Returns a value of type boolean
*/
@DISPID(441) //= 0x1b9. The runtime will prefer the VTID if present
@VTID(374)
boolean promptUpdateStyle();
/**
*
* Setter method for the COM property "PromptUpdateStyle"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(441) //= 0x1b9. The runtime will prefer the VTID if present
@VTID(375)
void promptUpdateStyle(
boolean prop);
/**
*
* Getter method for the COM property "DefaultEPostageApp"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(442) //= 0x1ba. The runtime will prefer the VTID if present
@VTID(376)
java.lang.String defaultEPostageApp();
/**
*
* Setter method for the COM property "DefaultEPostageApp"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(442) //= 0x1ba. The runtime will prefer the VTID if present
@VTID(377)
void defaultEPostageApp(
java.lang.String prop);
/**
*
* Getter method for the COM property "DefaultTextEncoding"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.MsoEncoding
*/
@DISPID(443) //= 0x1bb. The runtime will prefer the VTID if present
@VTID(378)
net.rgielen.com4j.office2010.office.MsoEncoding defaultTextEncoding();
/**
*
* Setter method for the COM property "DefaultTextEncoding"
*
* @param prop Mandatory net.rgielen.com4j.office2010.office.MsoEncoding parameter.
*/
@DISPID(443) //= 0x1bb. The runtime will prefer the VTID if present
@VTID(379)
void defaultTextEncoding(
net.rgielen.com4j.office2010.office.MsoEncoding prop);
/**
*
* Getter method for the COM property "LabelSmartTags"
*
* @return Returns a value of type boolean
*/
@DISPID(444) //= 0x1bc. The runtime will prefer the VTID if present
@VTID(380)
boolean labelSmartTags();
/**
*
* Setter method for the COM property "LabelSmartTags"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(444) //= 0x1bc. The runtime will prefer the VTID if present
@VTID(381)
void labelSmartTags(
boolean prop);
/**
*
* Getter method for the COM property "DisplaySmartTagButtons"
*
* @return Returns a value of type boolean
*/
@DISPID(445) //= 0x1bd. The runtime will prefer the VTID if present
@VTID(382)
boolean displaySmartTagButtons();
/**
*
* Setter method for the COM property "DisplaySmartTagButtons"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(445) //= 0x1bd. The runtime will prefer the VTID if present
@VTID(383)
void displaySmartTagButtons(
boolean prop);
/**
*
* Getter method for the COM property "WarnBeforeSavingPrintingSendingMarkup"
*
* @return Returns a value of type boolean
*/
@DISPID(446) //= 0x1be. The runtime will prefer the VTID if present
@VTID(384)
boolean warnBeforeSavingPrintingSendingMarkup();
/**
*
* Setter method for the COM property "WarnBeforeSavingPrintingSendingMarkup"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(446) //= 0x1be. The runtime will prefer the VTID if present
@VTID(385)
void warnBeforeSavingPrintingSendingMarkup(
boolean prop);
/**
*
* Getter method for the COM property "StoreRSIDOnSave"
*
* @return Returns a value of type boolean
*/
@DISPID(447) //= 0x1bf. The runtime will prefer the VTID if present
@VTID(386)
boolean storeRSIDOnSave();
/**
*
* Setter method for the COM property "StoreRSIDOnSave"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(447) //= 0x1bf. The runtime will prefer the VTID if present
@VTID(387)
void storeRSIDOnSave(
boolean prop);
/**
*
* Getter method for the COM property "ShowFormatError"
*
* @return Returns a value of type boolean
*/
@DISPID(448) //= 0x1c0. The runtime will prefer the VTID if present
@VTID(388)
boolean showFormatError();
/**
*
* Setter method for the COM property "ShowFormatError"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(448) //= 0x1c0. The runtime will prefer the VTID if present
@VTID(389)
void showFormatError(
boolean prop);
/**
*
* Getter method for the COM property "FormatScanning"
*
* @return Returns a value of type boolean
*/
@DISPID(449) //= 0x1c1. The runtime will prefer the VTID if present
@VTID(390)
boolean formatScanning();
/**
*
* Setter method for the COM property "FormatScanning"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(449) //= 0x1c1. The runtime will prefer the VTID if present
@VTID(391)
void formatScanning(
boolean prop);
/**
*
* Getter method for the COM property "PasteMergeLists"
*
* @return Returns a value of type boolean
*/
@DISPID(450) //= 0x1c2. The runtime will prefer the VTID if present
@VTID(392)
boolean pasteMergeLists();
/**
*
* Setter method for the COM property "PasteMergeLists"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(450) //= 0x1c2. The runtime will prefer the VTID if present
@VTID(393)
void pasteMergeLists(
boolean prop);
/**
*
* Getter method for the COM property "AutoCreateNewDrawings"
*
* @return Returns a value of type boolean
*/
@DISPID(451) //= 0x1c3. The runtime will prefer the VTID if present
@VTID(394)
boolean autoCreateNewDrawings();
/**
*
* Setter method for the COM property "AutoCreateNewDrawings"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(451) //= 0x1c3. The runtime will prefer the VTID if present
@VTID(395)
void autoCreateNewDrawings(
boolean prop);
/**
*
* Getter method for the COM property "SmartParaSelection"
*
* @return Returns a value of type boolean
*/
@DISPID(452) //= 0x1c4. The runtime will prefer the VTID if present
@VTID(396)
boolean smartParaSelection();
/**
*
* Setter method for the COM property "SmartParaSelection"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(452) //= 0x1c4. The runtime will prefer the VTID if present
@VTID(397)
void smartParaSelection(
boolean prop);
/**
*
* Getter method for the COM property "RevisionsBalloonPrintOrientation"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdRevisionsBalloonPrintOrientation
*/
@DISPID(453) //= 0x1c5. The runtime will prefer the VTID if present
@VTID(398)
net.rgielen.com4j.office2010.word.WdRevisionsBalloonPrintOrientation revisionsBalloonPrintOrientation();
/**
*
* Setter method for the COM property "RevisionsBalloonPrintOrientation"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdRevisionsBalloonPrintOrientation parameter.
*/
@DISPID(453) //= 0x1c5. The runtime will prefer the VTID if present
@VTID(399)
void revisionsBalloonPrintOrientation(
net.rgielen.com4j.office2010.word.WdRevisionsBalloonPrintOrientation prop);
/**
*
* Getter method for the COM property "CommentsColor"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdColorIndex
*/
@DISPID(454) //= 0x1c6. The runtime will prefer the VTID if present
@VTID(400)
net.rgielen.com4j.office2010.word.WdColorIndex commentsColor();
/**
*
* Setter method for the COM property "CommentsColor"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdColorIndex parameter.
*/
@DISPID(454) //= 0x1c6. The runtime will prefer the VTID if present
@VTID(401)
void commentsColor(
net.rgielen.com4j.office2010.word.WdColorIndex prop);
/**
*
* Getter method for the COM property "PrintXMLTag"
*
* @return Returns a value of type boolean
*/
@DISPID(455) //= 0x1c7. The runtime will prefer the VTID if present
@VTID(402)
boolean printXMLTag();
/**
*
* Setter method for the COM property "PrintXMLTag"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(455) //= 0x1c7. The runtime will prefer the VTID if present
@VTID(403)
void printXMLTag(
boolean prop);
/**
*
* Getter method for the COM property "PrintBackgrounds"
*
* @return Returns a value of type boolean
*/
@DISPID(456) //= 0x1c8. The runtime will prefer the VTID if present
@VTID(404)
boolean printBackgrounds();
/**
*
* Setter method for the COM property "PrintBackgrounds"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(456) //= 0x1c8. The runtime will prefer the VTID if present
@VTID(405)
void printBackgrounds(
boolean prop);
/**
*
* Getter method for the COM property "AllowReadingMode"
*
* @return Returns a value of type boolean
*/
@DISPID(457) //= 0x1c9. The runtime will prefer the VTID if present
@VTID(406)
boolean allowReadingMode();
/**
*
* Setter method for the COM property "AllowReadingMode"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(457) //= 0x1c9. The runtime will prefer the VTID if present
@VTID(407)
void allowReadingMode(
boolean prop);
/**
*
* Getter method for the COM property "ShowMarkupOpenSave"
*
* @return Returns a value of type boolean
*/
@DISPID(458) //= 0x1ca. The runtime will prefer the VTID if present
@VTID(408)
boolean showMarkupOpenSave();
/**
*
* Setter method for the COM property "ShowMarkupOpenSave"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(458) //= 0x1ca. The runtime will prefer the VTID if present
@VTID(409)
void showMarkupOpenSave(
boolean prop);
/**
*
* Getter method for the COM property "SmartCursoring"
*
* @return Returns a value of type boolean
*/
@DISPID(459) //= 0x1cb. The runtime will prefer the VTID if present
@VTID(410)
boolean smartCursoring();
/**
*
* Setter method for the COM property "SmartCursoring"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(459) //= 0x1cb. The runtime will prefer the VTID if present
@VTID(411)
void smartCursoring(
boolean prop);
/**
*
* Getter method for the COM property "MoveToTextMark"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdMoveToTextMark
*/
@DISPID(460) //= 0x1cc. The runtime will prefer the VTID if present
@VTID(412)
net.rgielen.com4j.office2010.word.WdMoveToTextMark moveToTextMark();
/**
*
* Setter method for the COM property "MoveToTextMark"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdMoveToTextMark parameter.
*/
@DISPID(460) //= 0x1cc. The runtime will prefer the VTID if present
@VTID(413)
void moveToTextMark(
net.rgielen.com4j.office2010.word.WdMoveToTextMark prop);
/**
*
* Getter method for the COM property "MoveFromTextMark"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdMoveFromTextMark
*/
@DISPID(461) //= 0x1cd. The runtime will prefer the VTID if present
@VTID(414)
net.rgielen.com4j.office2010.word.WdMoveFromTextMark moveFromTextMark();
/**
*
* Setter method for the COM property "MoveFromTextMark"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdMoveFromTextMark parameter.
*/
@DISPID(461) //= 0x1cd. The runtime will prefer the VTID if present
@VTID(415)
void moveFromTextMark(
net.rgielen.com4j.office2010.word.WdMoveFromTextMark prop);
/**
*
* Getter method for the COM property "BibliographyStyle"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(462) //= 0x1ce. The runtime will prefer the VTID if present
@VTID(416)
java.lang.String bibliographyStyle();
/**
*
* Setter method for the COM property "BibliographyStyle"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(462) //= 0x1ce. The runtime will prefer the VTID if present
@VTID(417)
void bibliographyStyle(
java.lang.String prop);
/**
*
* Getter method for the COM property "BibliographySort"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(463) //= 0x1cf. The runtime will prefer the VTID if present
@VTID(418)
java.lang.String bibliographySort();
/**
*
* Setter method for the COM property "BibliographySort"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(463) //= 0x1cf. The runtime will prefer the VTID if present
@VTID(419)
void bibliographySort(
java.lang.String prop);
/**
*
* Getter method for the COM property "InsertedCellColor"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdCellColor
*/
@DISPID(464) //= 0x1d0. The runtime will prefer the VTID if present
@VTID(420)
net.rgielen.com4j.office2010.word.WdCellColor insertedCellColor();
/**
*
* Setter method for the COM property "InsertedCellColor"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdCellColor parameter.
*/
@DISPID(464) //= 0x1d0. The runtime will prefer the VTID if present
@VTID(421)
void insertedCellColor(
net.rgielen.com4j.office2010.word.WdCellColor prop);
/**
*
* Getter method for the COM property "DeletedCellColor"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdCellColor
*/
@DISPID(465) //= 0x1d1. The runtime will prefer the VTID if present
@VTID(422)
net.rgielen.com4j.office2010.word.WdCellColor deletedCellColor();
/**
*
* Setter method for the COM property "DeletedCellColor"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdCellColor parameter.
*/
@DISPID(465) //= 0x1d1. The runtime will prefer the VTID if present
@VTID(423)
void deletedCellColor(
net.rgielen.com4j.office2010.word.WdCellColor prop);
/**
*
* Getter method for the COM property "MergedCellColor"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdCellColor
*/
@DISPID(466) //= 0x1d2. The runtime will prefer the VTID if present
@VTID(424)
net.rgielen.com4j.office2010.word.WdCellColor mergedCellColor();
/**
*
* Setter method for the COM property "MergedCellColor"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdCellColor parameter.
*/
@DISPID(466) //= 0x1d2. The runtime will prefer the VTID if present
@VTID(425)
void mergedCellColor(
net.rgielen.com4j.office2010.word.WdCellColor prop);
/**
*
* Getter method for the COM property "SplitCellColor"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdCellColor
*/
@DISPID(467) //= 0x1d3. The runtime will prefer the VTID if present
@VTID(426)
net.rgielen.com4j.office2010.word.WdCellColor splitCellColor();
/**
*
* Setter method for the COM property "SplitCellColor"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdCellColor parameter.
*/
@DISPID(467) //= 0x1d3. The runtime will prefer the VTID if present
@VTID(427)
void splitCellColor(
net.rgielen.com4j.office2010.word.WdCellColor prop);
/**
*
* Getter method for the COM property "ShowSelectionFloaties"
*
* @return Returns a value of type boolean
*/
@DISPID(468) //= 0x1d4. The runtime will prefer the VTID if present
@VTID(428)
boolean showSelectionFloaties();
/**
*
* Setter method for the COM property "ShowSelectionFloaties"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(468) //= 0x1d4. The runtime will prefer the VTID if present
@VTID(429)
void showSelectionFloaties(
boolean prop);
/**
*
* Getter method for the COM property "ShowMenuFloaties"
*
* @return Returns a value of type boolean
*/
@DISPID(469) //= 0x1d5. The runtime will prefer the VTID if present
@VTID(430)
boolean showMenuFloaties();
/**
*
* Setter method for the COM property "ShowMenuFloaties"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(469) //= 0x1d5. The runtime will prefer the VTID if present
@VTID(431)
void showMenuFloaties(
boolean prop);
/**
*
* Getter method for the COM property "ShowDevTools"
*
* @return Returns a value of type boolean
*/
@DISPID(470) //= 0x1d6. The runtime will prefer the VTID if present
@VTID(432)
boolean showDevTools();
/**
*
* Setter method for the COM property "ShowDevTools"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(470) //= 0x1d6. The runtime will prefer the VTID if present
@VTID(433)
void showDevTools(
boolean prop);
/**
*
* Getter method for the COM property "EnableLivePreview"
*
* @return Returns a value of type boolean
*/
@DISPID(472) //= 0x1d8. The runtime will prefer the VTID if present
@VTID(434)
boolean enableLivePreview();
/**
*
* Setter method for the COM property "EnableLivePreview"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(472) //= 0x1d8. The runtime will prefer the VTID if present
@VTID(435)
void enableLivePreview(
boolean prop);
/**
*
* Getter method for the COM property "OMathAutoBuildUp"
*
* @return Returns a value of type boolean
*/
@DISPID(474) //= 0x1da. The runtime will prefer the VTID if present
@VTID(436)
boolean oMathAutoBuildUp();
/**
*
* Setter method for the COM property "OMathAutoBuildUp"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(474) //= 0x1da. The runtime will prefer the VTID if present
@VTID(437)
void oMathAutoBuildUp(
boolean prop);
/**
*
* Getter method for the COM property "AlwaysUseClearType"
*
* @return Returns a value of type boolean
*/
@DISPID(476) //= 0x1dc. The runtime will prefer the VTID if present
@VTID(438)
boolean alwaysUseClearType();
/**
*
* Setter method for the COM property "AlwaysUseClearType"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(476) //= 0x1dc. The runtime will prefer the VTID if present
@VTID(439)
void alwaysUseClearType(
boolean prop);
/**
*
* Getter method for the COM property "PasteFormatWithinDocument"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdPasteOptions
*/
@DISPID(477) //= 0x1dd. The runtime will prefer the VTID if present
@VTID(440)
net.rgielen.com4j.office2010.word.WdPasteOptions pasteFormatWithinDocument();
/**
*
* Setter method for the COM property "PasteFormatWithinDocument"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdPasteOptions parameter.
*/
@DISPID(477) //= 0x1dd. The runtime will prefer the VTID if present
@VTID(441)
void pasteFormatWithinDocument(
net.rgielen.com4j.office2010.word.WdPasteOptions prop);
/**
*
* Getter method for the COM property "PasteFormatBetweenDocuments"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdPasteOptions
*/
@DISPID(478) //= 0x1de. The runtime will prefer the VTID if present
@VTID(442)
net.rgielen.com4j.office2010.word.WdPasteOptions pasteFormatBetweenDocuments();
/**
*
* Setter method for the COM property "PasteFormatBetweenDocuments"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdPasteOptions parameter.
*/
@DISPID(478) //= 0x1de. The runtime will prefer the VTID if present
@VTID(443)
void pasteFormatBetweenDocuments(
net.rgielen.com4j.office2010.word.WdPasteOptions prop);
/**
*
* Getter method for the COM property "PasteFormatBetweenStyledDocuments"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdPasteOptions
*/
@DISPID(479) //= 0x1df. The runtime will prefer the VTID if present
@VTID(444)
net.rgielen.com4j.office2010.word.WdPasteOptions pasteFormatBetweenStyledDocuments();
/**
*
* Setter method for the COM property "PasteFormatBetweenStyledDocuments"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdPasteOptions parameter.
*/
@DISPID(479) //= 0x1df. The runtime will prefer the VTID if present
@VTID(445)
void pasteFormatBetweenStyledDocuments(
net.rgielen.com4j.office2010.word.WdPasteOptions prop);
/**
*
* Getter method for the COM property "PasteFormatFromExternalSource"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdPasteOptions
*/
@DISPID(480) //= 0x1e0. The runtime will prefer the VTID if present
@VTID(446)
net.rgielen.com4j.office2010.word.WdPasteOptions pasteFormatFromExternalSource();
/**
*
* Setter method for the COM property "PasteFormatFromExternalSource"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdPasteOptions parameter.
*/
@DISPID(480) //= 0x1e0. The runtime will prefer the VTID if present
@VTID(447)
void pasteFormatFromExternalSource(
net.rgielen.com4j.office2010.word.WdPasteOptions prop);
/**
*
* Getter method for the COM property "PasteOptionKeepBulletsAndNumbers"
*
* @return Returns a value of type boolean
*/
@DISPID(481) //= 0x1e1. The runtime will prefer the VTID if present
@VTID(448)
boolean pasteOptionKeepBulletsAndNumbers();
/**
*
* Setter method for the COM property "PasteOptionKeepBulletsAndNumbers"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(481) //= 0x1e1. The runtime will prefer the VTID if present
@VTID(449)
void pasteOptionKeepBulletsAndNumbers(
boolean prop);
/**
*
* Getter method for the COM property "INSKeyForOvertype"
*
* @return Returns a value of type boolean
*/
@DISPID(482) //= 0x1e2. The runtime will prefer the VTID if present
@VTID(450)
boolean insKeyForOvertype();
/**
*
* Setter method for the COM property "INSKeyForOvertype"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(482) //= 0x1e2. The runtime will prefer the VTID if present
@VTID(451)
void insKeyForOvertype(
boolean prop);
/**
*
* Getter method for the COM property "RepeatWord"
*
* @return Returns a value of type boolean
*/
@DISPID(483) //= 0x1e3. The runtime will prefer the VTID if present
@VTID(452)
boolean repeatWord();
/**
*
* Setter method for the COM property "RepeatWord"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(483) //= 0x1e3. The runtime will prefer the VTID if present
@VTID(453)
void repeatWord(
boolean prop);
/**
*
* Getter method for the COM property "FrenchReform"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdFrenchSpeller
*/
@DISPID(484) //= 0x1e4. The runtime will prefer the VTID if present
@VTID(454)
net.rgielen.com4j.office2010.word.WdFrenchSpeller frenchReform();
/**
*
* Setter method for the COM property "FrenchReform"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdFrenchSpeller parameter.
*/
@DISPID(484) //= 0x1e4. The runtime will prefer the VTID if present
@VTID(455)
void frenchReform(
net.rgielen.com4j.office2010.word.WdFrenchSpeller prop);
/**
*
* Getter method for the COM property "ContextualSpeller"
*
* @return Returns a value of type boolean
*/
@DISPID(485) //= 0x1e5. The runtime will prefer the VTID if present
@VTID(456)
boolean contextualSpeller();
/**
*
* Setter method for the COM property "ContextualSpeller"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(485) //= 0x1e5. The runtime will prefer the VTID if present
@VTID(457)
void contextualSpeller(
boolean prop);
/**
*
* Getter method for the COM property "MoveToTextColor"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdColorIndex
*/
@DISPID(486) //= 0x1e6. The runtime will prefer the VTID if present
@VTID(458)
net.rgielen.com4j.office2010.word.WdColorIndex moveToTextColor();
/**
*
* Setter method for the COM property "MoveToTextColor"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdColorIndex parameter.
*/
@DISPID(486) //= 0x1e6. The runtime will prefer the VTID if present
@VTID(459)
void moveToTextColor(
net.rgielen.com4j.office2010.word.WdColorIndex prop);
/**
*
* Getter method for the COM property "MoveFromTextColor"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdColorIndex
*/
@DISPID(487) //= 0x1e7. The runtime will prefer the VTID if present
@VTID(460)
net.rgielen.com4j.office2010.word.WdColorIndex moveFromTextColor();
/**
*
* Setter method for the COM property "MoveFromTextColor"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdColorIndex parameter.
*/
@DISPID(487) //= 0x1e7. The runtime will prefer the VTID if present
@VTID(461)
void moveFromTextColor(
net.rgielen.com4j.office2010.word.WdColorIndex prop);
/**
*
* Getter method for the COM property "OMathCopyLF"
*
* @return Returns a value of type boolean
*/
@DISPID(488) //= 0x1e8. The runtime will prefer the VTID if present
@VTID(462)
boolean oMathCopyLF();
/**
*
* Setter method for the COM property "OMathCopyLF"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(488) //= 0x1e8. The runtime will prefer the VTID if present
@VTID(463)
void oMathCopyLF(
boolean prop);
/**
*
* Getter method for the COM property "UseNormalStyleForList"
*
* @return Returns a value of type boolean
*/
@DISPID(489) //= 0x1e9. The runtime will prefer the VTID if present
@VTID(464)
boolean useNormalStyleForList();
/**
*
* Setter method for the COM property "UseNormalStyleForList"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(489) //= 0x1e9. The runtime will prefer the VTID if present
@VTID(465)
void useNormalStyleForList(
boolean prop);
/**
*
* Getter method for the COM property "AllowOpenInDraftView"
*
* @return Returns a value of type boolean
*/
@DISPID(490) //= 0x1ea. The runtime will prefer the VTID if present
@VTID(466)
boolean allowOpenInDraftView();
/**
*
* Setter method for the COM property "AllowOpenInDraftView"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(490) //= 0x1ea. The runtime will prefer the VTID if present
@VTID(467)
void allowOpenInDraftView(
boolean prop);
/**
*
* Getter method for the COM property "EnableLegacyIMEMode"
*
* @return Returns a value of type boolean
*/
@DISPID(492) //= 0x1ec. The runtime will prefer the VTID if present
@VTID(468)
boolean enableLegacyIMEMode();
/**
*
* Setter method for the COM property "EnableLegacyIMEMode"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(492) //= 0x1ec. The runtime will prefer the VTID if present
@VTID(469)
void enableLegacyIMEMode(
boolean prop);
/**
*
* Getter method for the COM property "DoNotPromptForConvert"
*
* @return Returns a value of type boolean
*/
@DISPID(493) //= 0x1ed. The runtime will prefer the VTID if present
@VTID(470)
boolean doNotPromptForConvert();
/**
*
* Setter method for the COM property "DoNotPromptForConvert"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(493) //= 0x1ed. The runtime will prefer the VTID if present
@VTID(471)
void doNotPromptForConvert(
boolean prop);
/**
*
* Getter method for the COM property "PrecisePositioning"
*
* @return Returns a value of type boolean
*/
@DISPID(494) //= 0x1ee. The runtime will prefer the VTID if present
@VTID(472)
boolean precisePositioning();
/**
*
* Setter method for the COM property "PrecisePositioning"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(494) //= 0x1ee. The runtime will prefer the VTID if present
@VTID(473)
void precisePositioning(
boolean prop);
/**
*
* Getter method for the COM property "UpdateStyleListBehavior"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdUpdateStyleListBehavior
*/
@DISPID(495) //= 0x1ef. The runtime will prefer the VTID if present
@VTID(474)
net.rgielen.com4j.office2010.word.WdUpdateStyleListBehavior updateStyleListBehavior();
/**
*
* Setter method for the COM property "UpdateStyleListBehavior"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdUpdateStyleListBehavior parameter.
*/
@DISPID(495) //= 0x1ef. The runtime will prefer the VTID if present
@VTID(475)
void updateStyleListBehavior(
net.rgielen.com4j.office2010.word.WdUpdateStyleListBehavior prop);
/**
*
* Getter method for the COM property "StrictTaaMarboota"
*
* @return Returns a value of type boolean
*/
@DISPID(496) //= 0x1f0. The runtime will prefer the VTID if present
@VTID(476)
boolean strictTaaMarboota();
/**
*
* Setter method for the COM property "StrictTaaMarboota"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(496) //= 0x1f0. The runtime will prefer the VTID if present
@VTID(477)
void strictTaaMarboota(
boolean prop);
/**
*
* Getter method for the COM property "StrictRussianE"
*
* @return Returns a value of type boolean
*/
@DISPID(497) //= 0x1f1. The runtime will prefer the VTID if present
@VTID(478)
boolean strictRussianE();
/**
*
* Setter method for the COM property "StrictRussianE"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(497) //= 0x1f1. The runtime will prefer the VTID if present
@VTID(479)
void strictRussianE(
boolean prop);
/**
*
* Getter method for the COM property "SpanishMode"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdSpanishSpeller
*/
@DISPID(498) //= 0x1f2. The runtime will prefer the VTID if present
@VTID(480)
net.rgielen.com4j.office2010.word.WdSpanishSpeller spanishMode();
/**
*
* Setter method for the COM property "SpanishMode"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdSpanishSpeller parameter.
*/
@DISPID(498) //= 0x1f2. The runtime will prefer the VTID if present
@VTID(481)
void spanishMode(
net.rgielen.com4j.office2010.word.WdSpanishSpeller prop);
/**
*
* Getter method for the COM property "PortugalReform"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdPortugueseReform
*/
@DISPID(501) //= 0x1f5. The runtime will prefer the VTID if present
@VTID(482)
net.rgielen.com4j.office2010.word.WdPortugueseReform portugalReform();
/**
*
* Setter method for the COM property "PortugalReform"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdPortugueseReform parameter.
*/
@DISPID(501) //= 0x1f5. The runtime will prefer the VTID if present
@VTID(483)
void portugalReform(
net.rgielen.com4j.office2010.word.WdPortugueseReform prop);
/**
*
* Getter method for the COM property "BrazilReform"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdPortugueseReform
*/
@DISPID(502) //= 0x1f6. The runtime will prefer the VTID if present
@VTID(484)
net.rgielen.com4j.office2010.word.WdPortugueseReform brazilReform();
/**
*
* Setter method for the COM property "BrazilReform"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdPortugueseReform parameter.
*/
@DISPID(502) //= 0x1f6. The runtime will prefer the VTID if present
@VTID(485)
void brazilReform(
net.rgielen.com4j.office2010.word.WdPortugueseReform prop);
/**
*
* Getter method for the COM property "UpdateFieldsWithTrackedChangesAtPrint"
*
* @return Returns a value of type boolean
*/
@DISPID(503) //= 0x1f7. The runtime will prefer the VTID if present
@VTID(486)
boolean updateFieldsWithTrackedChangesAtPrint();
/**
*
* Setter method for the COM property "UpdateFieldsWithTrackedChangesAtPrint"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(503) //= 0x1f7. The runtime will prefer the VTID if present
@VTID(487)
void updateFieldsWithTrackedChangesAtPrint(
boolean prop);
// Properties:
}