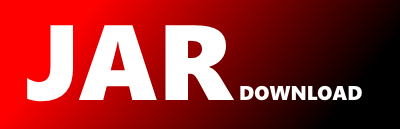
net.rgielen.com4j.office2010.word.TableOfFigures Maven / Gradle / Ivy
package net.rgielen.com4j.office2010.word ;
import com4j.*;
@IID("{00020921-0000-0000-C000-000000000046}")
public interface TableOfFigures extends Com4jObject {
// Methods:
/**
*
* Getter method for the COM property "Application"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word._Application
*/
@DISPID(1000) //= 0x3e8. The runtime will prefer the VTID if present
@VTID(7)
net.rgielen.com4j.office2010.word._Application application();
/**
*
* Getter method for the COM property "Creator"
*
* @return Returns a value of type int
*/
@DISPID(1001) //= 0x3e9. The runtime will prefer the VTID if present
@VTID(8)
int creator();
/**
*
* Getter method for the COM property "Parent"
*
* @return Returns a value of type com4j.Com4jObject
*/
@DISPID(1002) //= 0x3ea. The runtime will prefer the VTID if present
@VTID(9)
@ReturnValue(type=NativeType.Dispatch)
com4j.Com4jObject parent();
/**
*
* Getter method for the COM property "Caption"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(1) //= 0x1. The runtime will prefer the VTID if present
@VTID(10)
java.lang.String caption();
/**
*
* Setter method for the COM property "Caption"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(1) //= 0x1. The runtime will prefer the VTID if present
@VTID(11)
void caption(
java.lang.String prop);
/**
*
* Getter method for the COM property "IncludeLabel"
*
* @return Returns a value of type boolean
*/
@DISPID(2) //= 0x2. The runtime will prefer the VTID if present
@VTID(12)
boolean includeLabel();
/**
*
* Setter method for the COM property "IncludeLabel"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(2) //= 0x2. The runtime will prefer the VTID if present
@VTID(13)
void includeLabel(
boolean prop);
/**
*
* Getter method for the COM property "RightAlignPageNumbers"
*
* @return Returns a value of type boolean
*/
@DISPID(3) //= 0x3. The runtime will prefer the VTID if present
@VTID(14)
boolean rightAlignPageNumbers();
/**
*
* Setter method for the COM property "RightAlignPageNumbers"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(3) //= 0x3. The runtime will prefer the VTID if present
@VTID(15)
void rightAlignPageNumbers(
boolean prop);
/**
*
* Getter method for the COM property "UseHeadingStyles"
*
* @return Returns a value of type boolean
*/
@DISPID(4) //= 0x4. The runtime will prefer the VTID if present
@VTID(16)
boolean useHeadingStyles();
/**
*
* Setter method for the COM property "UseHeadingStyles"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(4) //= 0x4. The runtime will prefer the VTID if present
@VTID(17)
void useHeadingStyles(
boolean prop);
/**
*
* Getter method for the COM property "LowerHeadingLevel"
*
* @return Returns a value of type int
*/
@DISPID(5) //= 0x5. The runtime will prefer the VTID if present
@VTID(18)
int lowerHeadingLevel();
/**
*
* Setter method for the COM property "LowerHeadingLevel"
*
* @param prop Mandatory int parameter.
*/
@DISPID(5) //= 0x5. The runtime will prefer the VTID if present
@VTID(19)
void lowerHeadingLevel(
int prop);
/**
*
* Getter method for the COM property "UpperHeadingLevel"
*
* @return Returns a value of type int
*/
@DISPID(6) //= 0x6. The runtime will prefer the VTID if present
@VTID(20)
int upperHeadingLevel();
/**
*
* Setter method for the COM property "UpperHeadingLevel"
*
* @param prop Mandatory int parameter.
*/
@DISPID(6) //= 0x6. The runtime will prefer the VTID if present
@VTID(21)
void upperHeadingLevel(
int prop);
/**
*
* Getter method for the COM property "IncludePageNumbers"
*
* @return Returns a value of type boolean
*/
@DISPID(7) //= 0x7. The runtime will prefer the VTID if present
@VTID(22)
boolean includePageNumbers();
/**
*
* Setter method for the COM property "IncludePageNumbers"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(7) //= 0x7. The runtime will prefer the VTID if present
@VTID(23)
void includePageNumbers(
boolean prop);
/**
*
* Getter method for the COM property "Range"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Range
*/
@DISPID(8) //= 0x8. The runtime will prefer the VTID if present
@VTID(24)
net.rgielen.com4j.office2010.word.Range range();
/**
*
* Getter method for the COM property "UseFields"
*
* @return Returns a value of type boolean
*/
@DISPID(9) //= 0x9. The runtime will prefer the VTID if present
@VTID(25)
boolean useFields();
/**
*
* Setter method for the COM property "UseFields"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(9) //= 0x9. The runtime will prefer the VTID if present
@VTID(26)
void useFields(
boolean prop);
/**
*
* Getter method for the COM property "TableID"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(10) //= 0xa. The runtime will prefer the VTID if present
@VTID(27)
java.lang.String tableID();
/**
*
* Setter method for the COM property "TableID"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(10) //= 0xa. The runtime will prefer the VTID if present
@VTID(28)
void tableID(
java.lang.String prop);
/**
*
* Getter method for the COM property "HeadingStyles"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.HeadingStyles
*/
@DISPID(11) //= 0xb. The runtime will prefer the VTID if present
@VTID(29)
net.rgielen.com4j.office2010.word.HeadingStyles headingStyles();
@VTID(29)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.HeadingStyles.class})
net.rgielen.com4j.office2010.word.HeadingStyle headingStyles(
int index);
/**
*
* Getter method for the COM property "TabLeader"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdTabLeader
*/
@DISPID(12) //= 0xc. The runtime will prefer the VTID if present
@VTID(30)
net.rgielen.com4j.office2010.word.WdTabLeader tabLeader();
/**
*
* Setter method for the COM property "TabLeader"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdTabLeader parameter.
*/
@DISPID(12) //= 0xc. The runtime will prefer the VTID if present
@VTID(31)
void tabLeader(
net.rgielen.com4j.office2010.word.WdTabLeader prop);
/**
*/
@DISPID(100) //= 0x64. The runtime will prefer the VTID if present
@VTID(32)
void delete();
/**
*/
@DISPID(101) //= 0x65. The runtime will prefer the VTID if present
@VTID(33)
void updatePageNumbers();
/**
*/
@DISPID(102) //= 0x66. The runtime will prefer the VTID if present
@VTID(34)
void update();
/**
*
* Getter method for the COM property "UseHyperlinks"
*
* @return Returns a value of type boolean
*/
@DISPID(13) //= 0xd. The runtime will prefer the VTID if present
@VTID(35)
boolean useHyperlinks();
/**
*
* Setter method for the COM property "UseHyperlinks"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(13) //= 0xd. The runtime will prefer the VTID if present
@VTID(36)
void useHyperlinks(
boolean prop);
/**
*
* Getter method for the COM property "HidePageNumbersInWeb"
*
* @return Returns a value of type boolean
*/
@DISPID(14) //= 0xe. The runtime will prefer the VTID if present
@VTID(37)
boolean hidePageNumbersInWeb();
/**
*
* Setter method for the COM property "HidePageNumbersInWeb"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(14) //= 0xe. The runtime will prefer the VTID if present
@VTID(38)
void hidePageNumbersInWeb(
boolean prop);
// Properties:
}