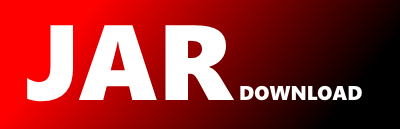
net.rgielen.com4j.office2010.word._Document Maven / Gradle / Ivy
package net.rgielen.com4j.office2010.word ;
import com4j.*;
@IID("{0002096B-0000-0000-C000-000000000046}")
public interface _Document extends Com4jObject {
// Methods:
/**
*
* Getter method for the COM property "Name"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(0) //= 0x0. The runtime will prefer the VTID if present
@VTID(7)
@DefaultMethod
java.lang.String name();
/**
*
* Getter method for the COM property "Application"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word._Application
*/
@DISPID(1) //= 0x1. The runtime will prefer the VTID if present
@VTID(8)
net.rgielen.com4j.office2010.word._Application application();
/**
*
* Getter method for the COM property "Creator"
*
* @return Returns a value of type int
*/
@DISPID(1001) //= 0x3e9. The runtime will prefer the VTID if present
@VTID(9)
int creator();
/**
*
* Getter method for the COM property "Parent"
*
* @return Returns a value of type com4j.Com4jObject
*/
@DISPID(1002) //= 0x3ea. The runtime will prefer the VTID if present
@VTID(10)
@ReturnValue(type=NativeType.Dispatch)
com4j.Com4jObject parent();
/**
*
* Getter method for the COM property "BuiltInDocumentProperties"
*
* @return Returns a value of type com4j.Com4jObject
*/
@DISPID(1000) //= 0x3e8. The runtime will prefer the VTID if present
@VTID(11)
@ReturnValue(type=NativeType.Dispatch)
com4j.Com4jObject builtInDocumentProperties();
/**
*
* Getter method for the COM property "CustomDocumentProperties"
*
* @return Returns a value of type com4j.Com4jObject
*/
@DISPID(2) //= 0x2. The runtime will prefer the VTID if present
@VTID(12)
@ReturnValue(type=NativeType.Dispatch)
com4j.Com4jObject customDocumentProperties();
/**
*
* Getter method for the COM property "Path"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(3) //= 0x3. The runtime will prefer the VTID if present
@VTID(13)
java.lang.String path();
/**
*
* Getter method for the COM property "Bookmarks"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Bookmarks
*/
@DISPID(4) //= 0x4. The runtime will prefer the VTID if present
@VTID(14)
net.rgielen.com4j.office2010.word.Bookmarks bookmarks();
@VTID(14)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Bookmarks.class})
net.rgielen.com4j.office2010.word.Bookmark bookmarks(
java.lang.Object index);
/**
*
* Getter method for the COM property "Tables"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Tables
*/
@DISPID(6) //= 0x6. The runtime will prefer the VTID if present
@VTID(15)
net.rgielen.com4j.office2010.word.Tables tables();
@VTID(15)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Tables.class})
net.rgielen.com4j.office2010.word.Table tables(
int index);
/**
*
* Getter method for the COM property "Footnotes"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Footnotes
*/
@DISPID(7) //= 0x7. The runtime will prefer the VTID if present
@VTID(16)
net.rgielen.com4j.office2010.word.Footnotes footnotes();
@VTID(16)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Footnotes.class})
net.rgielen.com4j.office2010.word.Footnote footnotes(
int index);
/**
*
* Getter method for the COM property "Endnotes"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Endnotes
*/
@DISPID(8) //= 0x8. The runtime will prefer the VTID if present
@VTID(17)
net.rgielen.com4j.office2010.word.Endnotes endnotes();
@VTID(17)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Endnotes.class})
net.rgielen.com4j.office2010.word.Endnote endnotes(
int index);
/**
*
* Getter method for the COM property "Comments"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Comments
*/
@DISPID(9) //= 0x9. The runtime will prefer the VTID if present
@VTID(18)
net.rgielen.com4j.office2010.word.Comments comments();
@VTID(18)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Comments.class})
net.rgielen.com4j.office2010.word.Comment comments(
int index);
/**
*
* Getter method for the COM property "Type"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdDocumentType
*/
@DISPID(10) //= 0xa. The runtime will prefer the VTID if present
@VTID(19)
net.rgielen.com4j.office2010.word.WdDocumentType type();
/**
*
* Getter method for the COM property "AutoHyphenation"
*
* @return Returns a value of type boolean
*/
@DISPID(11) //= 0xb. The runtime will prefer the VTID if present
@VTID(20)
boolean autoHyphenation();
/**
*
* Setter method for the COM property "AutoHyphenation"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(11) //= 0xb. The runtime will prefer the VTID if present
@VTID(21)
void autoHyphenation(
boolean prop);
/**
*
* Getter method for the COM property "HyphenateCaps"
*
* @return Returns a value of type boolean
*/
@DISPID(12) //= 0xc. The runtime will prefer the VTID if present
@VTID(22)
boolean hyphenateCaps();
/**
*
* Setter method for the COM property "HyphenateCaps"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(12) //= 0xc. The runtime will prefer the VTID if present
@VTID(23)
void hyphenateCaps(
boolean prop);
/**
*
* Getter method for the COM property "HyphenationZone"
*
* @return Returns a value of type int
*/
@DISPID(13) //= 0xd. The runtime will prefer the VTID if present
@VTID(24)
int hyphenationZone();
/**
*
* Setter method for the COM property "HyphenationZone"
*
* @param prop Mandatory int parameter.
*/
@DISPID(13) //= 0xd. The runtime will prefer the VTID if present
@VTID(25)
void hyphenationZone(
int prop);
/**
*
* Getter method for the COM property "ConsecutiveHyphensLimit"
*
* @return Returns a value of type int
*/
@DISPID(14) //= 0xe. The runtime will prefer the VTID if present
@VTID(26)
int consecutiveHyphensLimit();
/**
*
* Setter method for the COM property "ConsecutiveHyphensLimit"
*
* @param prop Mandatory int parameter.
*/
@DISPID(14) //= 0xe. The runtime will prefer the VTID if present
@VTID(27)
void consecutiveHyphensLimit(
int prop);
/**
*
* Getter method for the COM property "Sections"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Sections
*/
@DISPID(15) //= 0xf. The runtime will prefer the VTID if present
@VTID(28)
net.rgielen.com4j.office2010.word.Sections sections();
@VTID(28)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Sections.class})
net.rgielen.com4j.office2010.word.Section sections(
int index);
/**
*
* Getter method for the COM property "Paragraphs"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Paragraphs
*/
@DISPID(16) //= 0x10. The runtime will prefer the VTID if present
@VTID(29)
net.rgielen.com4j.office2010.word.Paragraphs paragraphs();
@VTID(29)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Paragraphs.class})
net.rgielen.com4j.office2010.word.Paragraph paragraphs(
int index);
/**
*
* Getter method for the COM property "Words"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Words
*/
@DISPID(17) //= 0x11. The runtime will prefer the VTID if present
@VTID(30)
net.rgielen.com4j.office2010.word.Words words();
@VTID(30)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Words.class})
net.rgielen.com4j.office2010.word.Range words(
int index);
/**
*
* Getter method for the COM property "Sentences"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Sentences
*/
@DISPID(18) //= 0x12. The runtime will prefer the VTID if present
@VTID(31)
net.rgielen.com4j.office2010.word.Sentences sentences();
@VTID(31)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Sentences.class})
net.rgielen.com4j.office2010.word.Range sentences(
int index);
/**
*
* Getter method for the COM property "Characters"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Characters
*/
@DISPID(19) //= 0x13. The runtime will prefer the VTID if present
@VTID(32)
net.rgielen.com4j.office2010.word.Characters characters();
@VTID(32)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Characters.class})
net.rgielen.com4j.office2010.word.Range characters(
int index);
/**
*
* Getter method for the COM property "Fields"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Fields
*/
@DISPID(20) //= 0x14. The runtime will prefer the VTID if present
@VTID(33)
net.rgielen.com4j.office2010.word.Fields fields();
@VTID(33)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Fields.class})
net.rgielen.com4j.office2010.word.Field fields(
int index);
/**
*
* Getter method for the COM property "FormFields"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.FormFields
*/
@DISPID(21) //= 0x15. The runtime will prefer the VTID if present
@VTID(34)
net.rgielen.com4j.office2010.word.FormFields formFields();
@VTID(34)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.FormFields.class})
net.rgielen.com4j.office2010.word.FormField formFields(
java.lang.Object index);
/**
*
* Getter method for the COM property "Styles"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Styles
*/
@DISPID(22) //= 0x16. The runtime will prefer the VTID if present
@VTID(35)
net.rgielen.com4j.office2010.word.Styles styles();
@VTID(35)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Styles.class})
net.rgielen.com4j.office2010.word.Style styles(
java.lang.Object index);
/**
*
* Getter method for the COM property "Frames"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Frames
*/
@DISPID(23) //= 0x17. The runtime will prefer the VTID if present
@VTID(36)
net.rgielen.com4j.office2010.word.Frames frames();
@VTID(36)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Frames.class})
net.rgielen.com4j.office2010.word.Frame frames(
int index);
/**
*
* Getter method for the COM property "TablesOfFigures"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.TablesOfFigures
*/
@DISPID(25) //= 0x19. The runtime will prefer the VTID if present
@VTID(37)
net.rgielen.com4j.office2010.word.TablesOfFigures tablesOfFigures();
@VTID(37)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.TablesOfFigures.class})
net.rgielen.com4j.office2010.word.TableOfFigures tablesOfFigures(
int index);
/**
*
* Getter method for the COM property "Variables"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Variables
*/
@DISPID(26) //= 0x1a. The runtime will prefer the VTID if present
@VTID(38)
net.rgielen.com4j.office2010.word.Variables variables();
@VTID(38)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Variables.class})
net.rgielen.com4j.office2010.word.Variable variables(
java.lang.Object index);
/**
*
* Getter method for the COM property "MailMerge"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.MailMerge
*/
@DISPID(27) //= 0x1b. The runtime will prefer the VTID if present
@VTID(39)
net.rgielen.com4j.office2010.word.MailMerge mailMerge();
/**
*
* Getter method for the COM property "Envelope"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Envelope
*/
@DISPID(28) //= 0x1c. The runtime will prefer the VTID if present
@VTID(40)
net.rgielen.com4j.office2010.word.Envelope envelope();
/**
*
* Getter method for the COM property "FullName"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(29) //= 0x1d. The runtime will prefer the VTID if present
@VTID(41)
java.lang.String fullName();
/**
*
* Getter method for the COM property "Revisions"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Revisions
*/
@DISPID(30) //= 0x1e. The runtime will prefer the VTID if present
@VTID(42)
net.rgielen.com4j.office2010.word.Revisions revisions();
@VTID(42)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Revisions.class})
net.rgielen.com4j.office2010.word.Revision revisions(
int index);
/**
*
* Getter method for the COM property "TablesOfContents"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.TablesOfContents
*/
@DISPID(31) //= 0x1f. The runtime will prefer the VTID if present
@VTID(43)
net.rgielen.com4j.office2010.word.TablesOfContents tablesOfContents();
@VTID(43)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.TablesOfContents.class})
net.rgielen.com4j.office2010.word.TableOfContents tablesOfContents(
int index);
/**
*
* Getter method for the COM property "TablesOfAuthorities"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.TablesOfAuthorities
*/
@DISPID(32) //= 0x20. The runtime will prefer the VTID if present
@VTID(44)
net.rgielen.com4j.office2010.word.TablesOfAuthorities tablesOfAuthorities();
@VTID(44)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.TablesOfAuthorities.class})
net.rgielen.com4j.office2010.word.TableOfAuthorities tablesOfAuthorities(
int index);
/**
*
* Getter method for the COM property "PageSetup"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.PageSetup
*/
@DISPID(1101) //= 0x44d. The runtime will prefer the VTID if present
@VTID(45)
net.rgielen.com4j.office2010.word.PageSetup pageSetup();
/**
*
* Setter method for the COM property "PageSetup"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.PageSetup parameter.
*/
@DISPID(1101) //= 0x44d. The runtime will prefer the VTID if present
@VTID(46)
void pageSetup(
net.rgielen.com4j.office2010.word.PageSetup prop);
/**
*
* Getter method for the COM property "Windows"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Windows
*/
@DISPID(34) //= 0x22. The runtime will prefer the VTID if present
@VTID(47)
net.rgielen.com4j.office2010.word.Windows windows();
@VTID(47)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Windows.class})
net.rgielen.com4j.office2010.word.Window windows(
java.lang.Object index);
/**
*
* Getter method for the COM property "HasRoutingSlip"
*
* @return Returns a value of type boolean
*/
@DISPID(35) //= 0x23. The runtime will prefer the VTID if present
@VTID(48)
boolean hasRoutingSlip();
/**
*
* Setter method for the COM property "HasRoutingSlip"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(35) //= 0x23. The runtime will prefer the VTID if present
@VTID(49)
void hasRoutingSlip(
boolean prop);
/**
*
* Getter method for the COM property "RoutingSlip"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.RoutingSlip
*/
@DISPID(36) //= 0x24. The runtime will prefer the VTID if present
@VTID(50)
net.rgielen.com4j.office2010.word.RoutingSlip routingSlip();
/**
*
* Getter method for the COM property "Routed"
*
* @return Returns a value of type boolean
*/
@DISPID(37) //= 0x25. The runtime will prefer the VTID if present
@VTID(51)
boolean routed();
/**
*
* Getter method for the COM property "TablesOfAuthoritiesCategories"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.TablesOfAuthoritiesCategories
*/
@DISPID(38) //= 0x26. The runtime will prefer the VTID if present
@VTID(52)
net.rgielen.com4j.office2010.word.TablesOfAuthoritiesCategories tablesOfAuthoritiesCategories();
@VTID(52)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.TablesOfAuthoritiesCategories.class})
net.rgielen.com4j.office2010.word.TableOfAuthoritiesCategory tablesOfAuthoritiesCategories(
java.lang.Object index);
/**
*
* Getter method for the COM property "Indexes"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Indexes
*/
@DISPID(39) //= 0x27. The runtime will prefer the VTID if present
@VTID(53)
net.rgielen.com4j.office2010.word.Indexes indexes();
@VTID(53)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Indexes.class})
net.rgielen.com4j.office2010.word.Index indexes(
int index);
/**
*
* Getter method for the COM property "Saved"
*
* @return Returns a value of type boolean
*/
@DISPID(40) //= 0x28. The runtime will prefer the VTID if present
@VTID(54)
boolean saved();
/**
*
* Setter method for the COM property "Saved"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(40) //= 0x28. The runtime will prefer the VTID if present
@VTID(55)
void saved(
boolean prop);
/**
*
* Getter method for the COM property "Content"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Range
*/
@DISPID(41) //= 0x29. The runtime will prefer the VTID if present
@VTID(56)
net.rgielen.com4j.office2010.word.Range content();
/**
*
* Getter method for the COM property "ActiveWindow"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Window
*/
@DISPID(42) //= 0x2a. The runtime will prefer the VTID if present
@VTID(57)
net.rgielen.com4j.office2010.word.Window activeWindow();
/**
*
* Getter method for the COM property "Kind"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdDocumentKind
*/
@DISPID(43) //= 0x2b. The runtime will prefer the VTID if present
@VTID(58)
net.rgielen.com4j.office2010.word.WdDocumentKind kind();
/**
*
* Setter method for the COM property "Kind"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdDocumentKind parameter.
*/
@DISPID(43) //= 0x2b. The runtime will prefer the VTID if present
@VTID(59)
void kind(
net.rgielen.com4j.office2010.word.WdDocumentKind prop);
/**
*
* Getter method for the COM property "ReadOnly"
*
* @return Returns a value of type boolean
*/
@DISPID(44) //= 0x2c. The runtime will prefer the VTID if present
@VTID(60)
boolean readOnly();
/**
*
* Getter method for the COM property "Subdocuments"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Subdocuments
*/
@DISPID(45) //= 0x2d. The runtime will prefer the VTID if present
@VTID(61)
net.rgielen.com4j.office2010.word.Subdocuments subdocuments();
@VTID(61)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Subdocuments.class})
net.rgielen.com4j.office2010.word.Subdocument subdocuments(
int index);
/**
*
* Getter method for the COM property "IsMasterDocument"
*
* @return Returns a value of type boolean
*/
@DISPID(46) //= 0x2e. The runtime will prefer the VTID if present
@VTID(62)
boolean isMasterDocument();
/**
*
* Getter method for the COM property "DefaultTabStop"
*
* @return Returns a value of type float
*/
@DISPID(48) //= 0x30. The runtime will prefer the VTID if present
@VTID(63)
float defaultTabStop();
/**
*
* Setter method for the COM property "DefaultTabStop"
*
* @param prop Mandatory float parameter.
*/
@DISPID(48) //= 0x30. The runtime will prefer the VTID if present
@VTID(64)
void defaultTabStop(
float prop);
/**
*
* Getter method for the COM property "EmbedTrueTypeFonts"
*
* @return Returns a value of type boolean
*/
@DISPID(50) //= 0x32. The runtime will prefer the VTID if present
@VTID(65)
boolean embedTrueTypeFonts();
/**
*
* Setter method for the COM property "EmbedTrueTypeFonts"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(50) //= 0x32. The runtime will prefer the VTID if present
@VTID(66)
void embedTrueTypeFonts(
boolean prop);
/**
*
* Getter method for the COM property "SaveFormsData"
*
* @return Returns a value of type boolean
*/
@DISPID(51) //= 0x33. The runtime will prefer the VTID if present
@VTID(67)
boolean saveFormsData();
/**
*
* Setter method for the COM property "SaveFormsData"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(51) //= 0x33. The runtime will prefer the VTID if present
@VTID(68)
void saveFormsData(
boolean prop);
/**
*
* Getter method for the COM property "ReadOnlyRecommended"
*
* @return Returns a value of type boolean
*/
@DISPID(52) //= 0x34. The runtime will prefer the VTID if present
@VTID(69)
boolean readOnlyRecommended();
/**
*
* Setter method for the COM property "ReadOnlyRecommended"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(52) //= 0x34. The runtime will prefer the VTID if present
@VTID(70)
void readOnlyRecommended(
boolean prop);
/**
*
* Getter method for the COM property "SaveSubsetFonts"
*
* @return Returns a value of type boolean
*/
@DISPID(53) //= 0x35. The runtime will prefer the VTID if present
@VTID(71)
boolean saveSubsetFonts();
/**
*
* Setter method for the COM property "SaveSubsetFonts"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(53) //= 0x35. The runtime will prefer the VTID if present
@VTID(72)
void saveSubsetFonts(
boolean prop);
/**
*
* Getter method for the COM property "Compatibility"
*
* @param type Mandatory net.rgielen.com4j.office2010.word.WdCompatibility parameter.
* @return Returns a value of type boolean
*/
@DISPID(55) //= 0x37. The runtime will prefer the VTID if present
@VTID(73)
boolean compatibility(
net.rgielen.com4j.office2010.word.WdCompatibility type);
/**
*
* Setter method for the COM property "Compatibility"
*
* @param type Mandatory net.rgielen.com4j.office2010.word.WdCompatibility parameter.
* @param prop Mandatory boolean parameter.
*/
@DISPID(55) //= 0x37. The runtime will prefer the VTID if present
@VTID(74)
void compatibility(
net.rgielen.com4j.office2010.word.WdCompatibility type,
boolean prop);
/**
*
* Getter method for the COM property "StoryRanges"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.StoryRanges
*/
@DISPID(56) //= 0x38. The runtime will prefer the VTID if present
@VTID(75)
net.rgielen.com4j.office2010.word.StoryRanges storyRanges();
@VTID(75)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.StoryRanges.class})
net.rgielen.com4j.office2010.word.Range storyRanges(
net.rgielen.com4j.office2010.word.WdStoryType index);
/**
*
* Getter method for the COM property "CommandBars"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office._CommandBars
*/
@DISPID(57) //= 0x39. The runtime will prefer the VTID if present
@VTID(76)
net.rgielen.com4j.office2010.office._CommandBars commandBars();
/**
*
* Getter method for the COM property "IsSubdocument"
*
* @return Returns a value of type boolean
*/
@DISPID(58) //= 0x3a. The runtime will prefer the VTID if present
@VTID(77)
boolean isSubdocument();
/**
*
* Getter method for the COM property "SaveFormat"
*
* @return Returns a value of type int
*/
@DISPID(59) //= 0x3b. The runtime will prefer the VTID if present
@VTID(78)
int saveFormat();
/**
*
* Getter method for the COM property "ProtectionType"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdProtectionType
*/
@DISPID(60) //= 0x3c. The runtime will prefer the VTID if present
@VTID(79)
net.rgielen.com4j.office2010.word.WdProtectionType protectionType();
/**
*
* Getter method for the COM property "Hyperlinks"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Hyperlinks
*/
@DISPID(61) //= 0x3d. The runtime will prefer the VTID if present
@VTID(80)
net.rgielen.com4j.office2010.word.Hyperlinks hyperlinks();
@VTID(80)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Hyperlinks.class})
net.rgielen.com4j.office2010.word.Hyperlink hyperlinks(
java.lang.Object index);
/**
*
* Getter method for the COM property "Shapes"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Shapes
*/
@DISPID(62) //= 0x3e. The runtime will prefer the VTID if present
@VTID(81)
net.rgielen.com4j.office2010.word.Shapes shapes();
@VTID(81)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Shapes.class})
net.rgielen.com4j.office2010.word.Shape shapes(
java.lang.Object index);
/**
*
* Getter method for the COM property "ListTemplates"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.ListTemplates
*/
@DISPID(63) //= 0x3f. The runtime will prefer the VTID if present
@VTID(82)
net.rgielen.com4j.office2010.word.ListTemplates listTemplates();
@VTID(82)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.ListTemplates.class})
net.rgielen.com4j.office2010.word.ListTemplate listTemplates(
java.lang.Object index);
/**
*
* Getter method for the COM property "Lists"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Lists
*/
@DISPID(64) //= 0x40. The runtime will prefer the VTID if present
@VTID(83)
net.rgielen.com4j.office2010.word.Lists lists();
@VTID(83)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Lists.class})
net.rgielen.com4j.office2010.word.List lists(
int index);
/**
*
* Getter method for the COM property "UpdateStylesOnOpen"
*
* @return Returns a value of type boolean
*/
@DISPID(66) //= 0x42. The runtime will prefer the VTID if present
@VTID(84)
boolean updateStylesOnOpen();
/**
*
* Setter method for the COM property "UpdateStylesOnOpen"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(66) //= 0x42. The runtime will prefer the VTID if present
@VTID(85)
void updateStylesOnOpen(
boolean prop);
/**
*
* Getter method for the COM property "AttachedTemplate"
*
* @return Returns a value of type java.lang.Object
*/
@DISPID(67) //= 0x43. The runtime will prefer the VTID if present
@VTID(86)
@ReturnValue(type=NativeType.VARIANT)
java.lang.Object attachedTemplate();
/**
*
* Setter method for the COM property "AttachedTemplate"
*
* @param prop Mandatory java.lang.Object parameter.
*/
@DISPID(67) //= 0x43. The runtime will prefer the VTID if present
@VTID(87)
void attachedTemplate(
java.lang.Object prop);
/**
*
* Getter method for the COM property "InlineShapes"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.InlineShapes
*/
@DISPID(68) //= 0x44. The runtime will prefer the VTID if present
@VTID(88)
net.rgielen.com4j.office2010.word.InlineShapes inlineShapes();
@VTID(88)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.InlineShapes.class})
net.rgielen.com4j.office2010.word.InlineShape inlineShapes(
int index);
/**
*
* Getter method for the COM property "Background"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Shape
*/
@DISPID(69) //= 0x45. The runtime will prefer the VTID if present
@VTID(89)
net.rgielen.com4j.office2010.word.Shape background();
/**
*
* Setter method for the COM property "Background"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.Shape parameter.
*/
@DISPID(69) //= 0x45. The runtime will prefer the VTID if present
@VTID(90)
void background(
net.rgielen.com4j.office2010.word.Shape prop);
/**
*
* Getter method for the COM property "GrammarChecked"
*
* @return Returns a value of type boolean
*/
@DISPID(70) //= 0x46. The runtime will prefer the VTID if present
@VTID(91)
boolean grammarChecked();
/**
*
* Setter method for the COM property "GrammarChecked"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(70) //= 0x46. The runtime will prefer the VTID if present
@VTID(92)
void grammarChecked(
boolean prop);
/**
*
* Getter method for the COM property "SpellingChecked"
*
* @return Returns a value of type boolean
*/
@DISPID(71) //= 0x47. The runtime will prefer the VTID if present
@VTID(93)
boolean spellingChecked();
/**
*
* Setter method for the COM property "SpellingChecked"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(71) //= 0x47. The runtime will prefer the VTID if present
@VTID(94)
void spellingChecked(
boolean prop);
/**
*
* Getter method for the COM property "ShowGrammaticalErrors"
*
* @return Returns a value of type boolean
*/
@DISPID(72) //= 0x48. The runtime will prefer the VTID if present
@VTID(95)
boolean showGrammaticalErrors();
/**
*
* Setter method for the COM property "ShowGrammaticalErrors"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(72) //= 0x48. The runtime will prefer the VTID if present
@VTID(96)
void showGrammaticalErrors(
boolean prop);
/**
*
* Getter method for the COM property "ShowSpellingErrors"
*
* @return Returns a value of type boolean
*/
@DISPID(73) //= 0x49. The runtime will prefer the VTID if present
@VTID(97)
boolean showSpellingErrors();
/**
*
* Setter method for the COM property "ShowSpellingErrors"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(73) //= 0x49. The runtime will prefer the VTID if present
@VTID(98)
void showSpellingErrors(
boolean prop);
/**
*
* Getter method for the COM property "Versions"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Versions
*/
@DISPID(75) //= 0x4b. The runtime will prefer the VTID if present
@VTID(99)
net.rgielen.com4j.office2010.word.Versions versions();
@VTID(99)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.Versions.class})
net.rgielen.com4j.office2010.word.Version versions(
int index);
/**
*
* Getter method for the COM property "ShowSummary"
*
* @return Returns a value of type boolean
*/
@DISPID(76) //= 0x4c. The runtime will prefer the VTID if present
@VTID(100)
boolean showSummary();
/**
*
* Setter method for the COM property "ShowSummary"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(76) //= 0x4c. The runtime will prefer the VTID if present
@VTID(101)
void showSummary(
boolean prop);
/**
*
* Getter method for the COM property "SummaryViewMode"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdSummaryMode
*/
@DISPID(77) //= 0x4d. The runtime will prefer the VTID if present
@VTID(102)
net.rgielen.com4j.office2010.word.WdSummaryMode summaryViewMode();
/**
*
* Setter method for the COM property "SummaryViewMode"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdSummaryMode parameter.
*/
@DISPID(77) //= 0x4d. The runtime will prefer the VTID if present
@VTID(103)
void summaryViewMode(
net.rgielen.com4j.office2010.word.WdSummaryMode prop);
/**
*
* Getter method for the COM property "SummaryLength"
*
* @return Returns a value of type int
*/
@DISPID(78) //= 0x4e. The runtime will prefer the VTID if present
@VTID(104)
int summaryLength();
/**
*
* Setter method for the COM property "SummaryLength"
*
* @param prop Mandatory int parameter.
*/
@DISPID(78) //= 0x4e. The runtime will prefer the VTID if present
@VTID(105)
void summaryLength(
int prop);
/**
*
* Getter method for the COM property "PrintFractionalWidths"
*
* @return Returns a value of type boolean
*/
@DISPID(79) //= 0x4f. The runtime will prefer the VTID if present
@VTID(106)
boolean printFractionalWidths();
/**
*
* Setter method for the COM property "PrintFractionalWidths"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(79) //= 0x4f. The runtime will prefer the VTID if present
@VTID(107)
void printFractionalWidths(
boolean prop);
/**
*
* Getter method for the COM property "PrintPostScriptOverText"
*
* @return Returns a value of type boolean
*/
@DISPID(80) //= 0x50. The runtime will prefer the VTID if present
@VTID(108)
boolean printPostScriptOverText();
/**
*
* Setter method for the COM property "PrintPostScriptOverText"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(80) //= 0x50. The runtime will prefer the VTID if present
@VTID(109)
void printPostScriptOverText(
boolean prop);
/**
*
* Getter method for the COM property "Container"
*
* @return Returns a value of type com4j.Com4jObject
*/
@DISPID(82) //= 0x52. The runtime will prefer the VTID if present
@VTID(110)
@ReturnValue(type=NativeType.Dispatch)
com4j.Com4jObject container();
/**
*
* Getter method for the COM property "PrintFormsData"
*
* @return Returns a value of type boolean
*/
@DISPID(83) //= 0x53. The runtime will prefer the VTID if present
@VTID(111)
boolean printFormsData();
/**
*
* Setter method for the COM property "PrintFormsData"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(83) //= 0x53. The runtime will prefer the VTID if present
@VTID(112)
void printFormsData(
boolean prop);
/**
*
* Getter method for the COM property "ListParagraphs"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.ListParagraphs
*/
@DISPID(84) //= 0x54. The runtime will prefer the VTID if present
@VTID(113)
net.rgielen.com4j.office2010.word.ListParagraphs listParagraphs();
@VTID(113)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.ListParagraphs.class})
net.rgielen.com4j.office2010.word.Paragraph listParagraphs(
int index);
/**
*
* Setter method for the COM property "Password"
*
* @param rhs Mandatory java.lang.String parameter.
*/
@DISPID(85) //= 0x55. The runtime will prefer the VTID if present
@VTID(114)
void password(
java.lang.String rhs);
/**
*
* Setter method for the COM property "WritePassword"
*
* @param rhs Mandatory java.lang.String parameter.
*/
@DISPID(86) //= 0x56. The runtime will prefer the VTID if present
@VTID(115)
void writePassword(
java.lang.String rhs);
/**
*
* Getter method for the COM property "HasPassword"
*
* @return Returns a value of type boolean
*/
@DISPID(87) //= 0x57. The runtime will prefer the VTID if present
@VTID(116)
boolean hasPassword();
/**
*
* Getter method for the COM property "WriteReserved"
*
* @return Returns a value of type boolean
*/
@DISPID(88) //= 0x58. The runtime will prefer the VTID if present
@VTID(117)
boolean writeReserved();
/**
*
* Getter method for the COM property "ActiveWritingStyle"
*
* @param languageID Mandatory java.lang.Object parameter.
* @return Returns a value of type java.lang.String
*/
@DISPID(90) //= 0x5a. The runtime will prefer the VTID if present
@VTID(118)
java.lang.String activeWritingStyle(
java.lang.Object languageID);
/**
*
* Setter method for the COM property "ActiveWritingStyle"
*
* @param languageID Mandatory java.lang.Object parameter.
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(90) //= 0x5a. The runtime will prefer the VTID if present
@VTID(119)
void activeWritingStyle(
java.lang.Object languageID,
java.lang.String prop);
/**
*
* Getter method for the COM property "UserControl"
*
* @return Returns a value of type boolean
*/
@DISPID(92) //= 0x5c. The runtime will prefer the VTID if present
@VTID(120)
boolean userControl();
/**
*
* Setter method for the COM property "UserControl"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(92) //= 0x5c. The runtime will prefer the VTID if present
@VTID(121)
void userControl(
boolean prop);
/**
*
* Getter method for the COM property "HasMailer"
*
* @return Returns a value of type boolean
*/
@DISPID(93) //= 0x5d. The runtime will prefer the VTID if present
@VTID(122)
boolean hasMailer();
/**
*
* Setter method for the COM property "HasMailer"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(93) //= 0x5d. The runtime will prefer the VTID if present
@VTID(123)
void hasMailer(
boolean prop);
/**
*
* Getter method for the COM property "Mailer"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Mailer
*/
@DISPID(94) //= 0x5e. The runtime will prefer the VTID if present
@VTID(124)
net.rgielen.com4j.office2010.word.Mailer mailer();
/**
*
* Getter method for the COM property "ReadabilityStatistics"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.ReadabilityStatistics
*/
@DISPID(96) //= 0x60. The runtime will prefer the VTID if present
@VTID(125)
net.rgielen.com4j.office2010.word.ReadabilityStatistics readabilityStatistics();
@VTID(125)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.ReadabilityStatistics.class})
net.rgielen.com4j.office2010.word.ReadabilityStatistic readabilityStatistics(
java.lang.Object index);
/**
*
* Getter method for the COM property "GrammaticalErrors"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.ProofreadingErrors
*/
@DISPID(97) //= 0x61. The runtime will prefer the VTID if present
@VTID(126)
net.rgielen.com4j.office2010.word.ProofreadingErrors grammaticalErrors();
@VTID(126)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.ProofreadingErrors.class})
net.rgielen.com4j.office2010.word.Range grammaticalErrors(
int index);
/**
*
* Getter method for the COM property "SpellingErrors"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.ProofreadingErrors
*/
@DISPID(98) //= 0x62. The runtime will prefer the VTID if present
@VTID(127)
net.rgielen.com4j.office2010.word.ProofreadingErrors spellingErrors();
@VTID(127)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.ProofreadingErrors.class})
net.rgielen.com4j.office2010.word.Range spellingErrors(
int index);
/**
*
* Getter method for the COM property "VBProject"
*
* @return Returns a value of type net.rgielen.com4j.office2010.vba._VBProject
*/
@DISPID(99) //= 0x63. The runtime will prefer the VTID if present
@VTID(128)
net.rgielen.com4j.office2010.vba._VBProject vbProject();
/**
*
* Getter method for the COM property "FormsDesign"
*
* @return Returns a value of type boolean
*/
@DISPID(100) //= 0x64. The runtime will prefer the VTID if present
@VTID(129)
boolean formsDesign();
/**
*
* Getter method for the COM property "_CodeName"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(-2147418112) //= 0x80010000. The runtime will prefer the VTID if present
@VTID(130)
java.lang.String _CodeName();
/**
*
* Setter method for the COM property "_CodeName"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(-2147418112) //= 0x80010000. The runtime will prefer the VTID if present
@VTID(131)
void _CodeName(
java.lang.String prop);
/**
*
* Getter method for the COM property "CodeName"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(262) //= 0x106. The runtime will prefer the VTID if present
@VTID(132)
java.lang.String codeName();
/**
*
* Getter method for the COM property "SnapToGrid"
*
* @return Returns a value of type boolean
*/
@DISPID(300) //= 0x12c. The runtime will prefer the VTID if present
@VTID(133)
boolean snapToGrid();
/**
*
* Setter method for the COM property "SnapToGrid"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(300) //= 0x12c. The runtime will prefer the VTID if present
@VTID(134)
void snapToGrid(
boolean prop);
/**
*
* Getter method for the COM property "SnapToShapes"
*
* @return Returns a value of type boolean
*/
@DISPID(301) //= 0x12d. The runtime will prefer the VTID if present
@VTID(135)
boolean snapToShapes();
/**
*
* Setter method for the COM property "SnapToShapes"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(301) //= 0x12d. The runtime will prefer the VTID if present
@VTID(136)
void snapToShapes(
boolean prop);
/**
*
* Getter method for the COM property "GridDistanceHorizontal"
*
* @return Returns a value of type float
*/
@DISPID(302) //= 0x12e. The runtime will prefer the VTID if present
@VTID(137)
float gridDistanceHorizontal();
/**
*
* Setter method for the COM property "GridDistanceHorizontal"
*
* @param prop Mandatory float parameter.
*/
@DISPID(302) //= 0x12e. The runtime will prefer the VTID if present
@VTID(138)
void gridDistanceHorizontal(
float prop);
/**
*
* Getter method for the COM property "GridDistanceVertical"
*
* @return Returns a value of type float
*/
@DISPID(303) //= 0x12f. The runtime will prefer the VTID if present
@VTID(139)
float gridDistanceVertical();
/**
*
* Setter method for the COM property "GridDistanceVertical"
*
* @param prop Mandatory float parameter.
*/
@DISPID(303) //= 0x12f. The runtime will prefer the VTID if present
@VTID(140)
void gridDistanceVertical(
float prop);
/**
*
* Getter method for the COM property "GridOriginHorizontal"
*
* @return Returns a value of type float
*/
@DISPID(304) //= 0x130. The runtime will prefer the VTID if present
@VTID(141)
float gridOriginHorizontal();
/**
*
* Setter method for the COM property "GridOriginHorizontal"
*
* @param prop Mandatory float parameter.
*/
@DISPID(304) //= 0x130. The runtime will prefer the VTID if present
@VTID(142)
void gridOriginHorizontal(
float prop);
/**
*
* Getter method for the COM property "GridOriginVertical"
*
* @return Returns a value of type float
*/
@DISPID(305) //= 0x131. The runtime will prefer the VTID if present
@VTID(143)
float gridOriginVertical();
/**
*
* Setter method for the COM property "GridOriginVertical"
*
* @param prop Mandatory float parameter.
*/
@DISPID(305) //= 0x131. The runtime will prefer the VTID if present
@VTID(144)
void gridOriginVertical(
float prop);
/**
*
* Getter method for the COM property "GridSpaceBetweenHorizontalLines"
*
* @return Returns a value of type int
*/
@DISPID(306) //= 0x132. The runtime will prefer the VTID if present
@VTID(145)
int gridSpaceBetweenHorizontalLines();
/**
*
* Setter method for the COM property "GridSpaceBetweenHorizontalLines"
*
* @param prop Mandatory int parameter.
*/
@DISPID(306) //= 0x132. The runtime will prefer the VTID if present
@VTID(146)
void gridSpaceBetweenHorizontalLines(
int prop);
/**
*
* Getter method for the COM property "GridSpaceBetweenVerticalLines"
*
* @return Returns a value of type int
*/
@DISPID(307) //= 0x133. The runtime will prefer the VTID if present
@VTID(147)
int gridSpaceBetweenVerticalLines();
/**
*
* Setter method for the COM property "GridSpaceBetweenVerticalLines"
*
* @param prop Mandatory int parameter.
*/
@DISPID(307) //= 0x133. The runtime will prefer the VTID if present
@VTID(148)
void gridSpaceBetweenVerticalLines(
int prop);
/**
*
* Getter method for the COM property "GridOriginFromMargin"
*
* @return Returns a value of type boolean
*/
@DISPID(308) //= 0x134. The runtime will prefer the VTID if present
@VTID(149)
boolean gridOriginFromMargin();
/**
*
* Setter method for the COM property "GridOriginFromMargin"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(308) //= 0x134. The runtime will prefer the VTID if present
@VTID(150)
void gridOriginFromMargin(
boolean prop);
/**
*
* Getter method for the COM property "KerningByAlgorithm"
*
* @return Returns a value of type boolean
*/
@DISPID(309) //= 0x135. The runtime will prefer the VTID if present
@VTID(151)
boolean kerningByAlgorithm();
/**
*
* Setter method for the COM property "KerningByAlgorithm"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(309) //= 0x135. The runtime will prefer the VTID if present
@VTID(152)
void kerningByAlgorithm(
boolean prop);
/**
*
* Getter method for the COM property "JustificationMode"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdJustificationMode
*/
@DISPID(310) //= 0x136. The runtime will prefer the VTID if present
@VTID(153)
net.rgielen.com4j.office2010.word.WdJustificationMode justificationMode();
/**
*
* Setter method for the COM property "JustificationMode"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdJustificationMode parameter.
*/
@DISPID(310) //= 0x136. The runtime will prefer the VTID if present
@VTID(154)
void justificationMode(
net.rgielen.com4j.office2010.word.WdJustificationMode prop);
/**
*
* Getter method for the COM property "FarEastLineBreakLevel"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdFarEastLineBreakLevel
*/
@DISPID(311) //= 0x137. The runtime will prefer the VTID if present
@VTID(155)
net.rgielen.com4j.office2010.word.WdFarEastLineBreakLevel farEastLineBreakLevel();
/**
*
* Setter method for the COM property "FarEastLineBreakLevel"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdFarEastLineBreakLevel parameter.
*/
@DISPID(311) //= 0x137. The runtime will prefer the VTID if present
@VTID(156)
void farEastLineBreakLevel(
net.rgielen.com4j.office2010.word.WdFarEastLineBreakLevel prop);
/**
*
* Getter method for the COM property "NoLineBreakBefore"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(312) //= 0x138. The runtime will prefer the VTID if present
@VTID(157)
java.lang.String noLineBreakBefore();
/**
*
* Setter method for the COM property "NoLineBreakBefore"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(312) //= 0x138. The runtime will prefer the VTID if present
@VTID(158)
void noLineBreakBefore(
java.lang.String prop);
/**
*
* Getter method for the COM property "NoLineBreakAfter"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(313) //= 0x139. The runtime will prefer the VTID if present
@VTID(159)
java.lang.String noLineBreakAfter();
/**
*
* Setter method for the COM property "NoLineBreakAfter"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(313) //= 0x139. The runtime will prefer the VTID if present
@VTID(160)
void noLineBreakAfter(
java.lang.String prop);
/**
*
* Getter method for the COM property "TrackRevisions"
*
* @return Returns a value of type boolean
*/
@DISPID(314) //= 0x13a. The runtime will prefer the VTID if present
@VTID(161)
boolean trackRevisions();
/**
*
* Setter method for the COM property "TrackRevisions"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(314) //= 0x13a. The runtime will prefer the VTID if present
@VTID(162)
void trackRevisions(
boolean prop);
/**
*
* Getter method for the COM property "PrintRevisions"
*
* @return Returns a value of type boolean
*/
@DISPID(315) //= 0x13b. The runtime will prefer the VTID if present
@VTID(163)
boolean printRevisions();
/**
*
* Setter method for the COM property "PrintRevisions"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(315) //= 0x13b. The runtime will prefer the VTID if present
@VTID(164)
void printRevisions(
boolean prop);
/**
*
* Getter method for the COM property "ShowRevisions"
*
* @return Returns a value of type boolean
*/
@DISPID(316) //= 0x13c. The runtime will prefer the VTID if present
@VTID(165)
boolean showRevisions();
/**
*
* Setter method for the COM property "ShowRevisions"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(316) //= 0x13c. The runtime will prefer the VTID if present
@VTID(166)
void showRevisions(
boolean prop);
/**
* @param saveChanges Optional parameter. Default value is com4j.Variant.getMissing()
* @param originalFormat Optional parameter. Default value is com4j.Variant.getMissing()
* @param routeDocument Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(1105) //= 0x451. The runtime will prefer the VTID if present
@VTID(167)
void close(
@Optional java.lang.Object saveChanges,
@Optional java.lang.Object originalFormat,
@Optional java.lang.Object routeDocument);
/**
* @param fileName Optional parameter. Default value is com4j.Variant.getMissing()
* @param fileFormat Optional parameter. Default value is com4j.Variant.getMissing()
* @param lockComments Optional parameter. Default value is com4j.Variant.getMissing()
* @param password Optional parameter. Default value is com4j.Variant.getMissing()
* @param addToRecentFiles Optional parameter. Default value is com4j.Variant.getMissing()
* @param writePassword Optional parameter. Default value is com4j.Variant.getMissing()
* @param readOnlyRecommended Optional parameter. Default value is com4j.Variant.getMissing()
* @param embedTrueTypeFonts Optional parameter. Default value is com4j.Variant.getMissing()
* @param saveNativePictureFormat Optional parameter. Default value is com4j.Variant.getMissing()
* @param saveFormsData Optional parameter. Default value is com4j.Variant.getMissing()
* @param saveAsAOCELetter Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(102) //= 0x66. The runtime will prefer the VTID if present
@VTID(168)
void saveAs2000(
@Optional java.lang.Object fileName,
@Optional java.lang.Object fileFormat,
@Optional java.lang.Object lockComments,
@Optional java.lang.Object password,
@Optional java.lang.Object addToRecentFiles,
@Optional java.lang.Object writePassword,
@Optional java.lang.Object readOnlyRecommended,
@Optional java.lang.Object embedTrueTypeFonts,
@Optional java.lang.Object saveNativePictureFormat,
@Optional java.lang.Object saveFormsData,
@Optional java.lang.Object saveAsAOCELetter);
/**
*/
@DISPID(103) //= 0x67. The runtime will prefer the VTID if present
@VTID(169)
void repaginate();
/**
*/
@DISPID(104) //= 0x68. The runtime will prefer the VTID if present
@VTID(170)
void fitToPages();
/**
*/
@DISPID(105) //= 0x69. The runtime will prefer the VTID if present
@VTID(171)
void manualHyphenation();
/**
*/
@DISPID(65535) //= 0xffff. The runtime will prefer the VTID if present
@VTID(172)
void select();
/**
*/
@DISPID(106) //= 0x6a. The runtime will prefer the VTID if present
@VTID(173)
void dataForm();
/**
*/
@DISPID(107) //= 0x6b. The runtime will prefer the VTID if present
@VTID(174)
void route();
/**
*/
@DISPID(108) //= 0x6c. The runtime will prefer the VTID if present
@VTID(175)
void save();
/**
* @param background Optional parameter. Default value is com4j.Variant.getMissing()
* @param append Optional parameter. Default value is com4j.Variant.getMissing()
* @param range Optional parameter. Default value is com4j.Variant.getMissing()
* @param outputFileName Optional parameter. Default value is com4j.Variant.getMissing()
* @param from Optional parameter. Default value is com4j.Variant.getMissing()
* @param to Optional parameter. Default value is com4j.Variant.getMissing()
* @param item Optional parameter. Default value is com4j.Variant.getMissing()
* @param copies Optional parameter. Default value is com4j.Variant.getMissing()
* @param pages Optional parameter. Default value is com4j.Variant.getMissing()
* @param pageType Optional parameter. Default value is com4j.Variant.getMissing()
* @param printToFile Optional parameter. Default value is com4j.Variant.getMissing()
* @param collate Optional parameter. Default value is com4j.Variant.getMissing()
* @param activePrinterMacGX Optional parameter. Default value is com4j.Variant.getMissing()
* @param manualDuplexPrint Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(109) //= 0x6d. The runtime will prefer the VTID if present
@VTID(176)
void printOutOld(
@Optional java.lang.Object background,
@Optional java.lang.Object append,
@Optional java.lang.Object range,
@Optional java.lang.Object outputFileName,
@Optional java.lang.Object from,
@Optional java.lang.Object to,
@Optional java.lang.Object item,
@Optional java.lang.Object copies,
@Optional java.lang.Object pages,
@Optional java.lang.Object pageType,
@Optional java.lang.Object printToFile,
@Optional java.lang.Object collate,
@Optional java.lang.Object activePrinterMacGX,
@Optional java.lang.Object manualDuplexPrint);
/**
*/
@DISPID(110) //= 0x6e. The runtime will prefer the VTID if present
@VTID(177)
void sendMail();
/**
* @param start Optional parameter. Default value is com4j.Variant.getMissing()
* @param end Optional parameter. Default value is com4j.Variant.getMissing()
* @return Returns a value of type net.rgielen.com4j.office2010.word.Range
*/
@DISPID(2000) //= 0x7d0. The runtime will prefer the VTID if present
@VTID(178)
net.rgielen.com4j.office2010.word.Range range(
@Optional java.lang.Object start,
@Optional java.lang.Object end);
/**
* @param which Mandatory net.rgielen.com4j.office2010.word.WdAutoMacros parameter.
*/
@DISPID(112) //= 0x70. The runtime will prefer the VTID if present
@VTID(179)
void runAutoMacro(
net.rgielen.com4j.office2010.word.WdAutoMacros which);
/**
*/
@DISPID(113) //= 0x71. The runtime will prefer the VTID if present
@VTID(180)
void activate();
/**
*/
@DISPID(114) //= 0x72. The runtime will prefer the VTID if present
@VTID(181)
void printPreview();
/**
* @param what Optional parameter. Default value is com4j.Variant.getMissing()
* @param which Optional parameter. Default value is com4j.Variant.getMissing()
* @param count Optional parameter. Default value is com4j.Variant.getMissing()
* @param name Optional parameter. Default value is com4j.Variant.getMissing()
* @return Returns a value of type net.rgielen.com4j.office2010.word.Range
*/
@DISPID(115) //= 0x73. The runtime will prefer the VTID if present
@VTID(182)
net.rgielen.com4j.office2010.word.Range goTo(
@Optional java.lang.Object what,
@Optional java.lang.Object which,
@Optional java.lang.Object count,
@Optional java.lang.Object name);
/**
* @param times Optional parameter. Default value is com4j.Variant.getMissing()
* @return Returns a value of type boolean
*/
@DISPID(116) //= 0x74. The runtime will prefer the VTID if present
@VTID(183)
boolean undo(
@Optional java.lang.Object times);
/**
* @param times Optional parameter. Default value is com4j.Variant.getMissing()
* @return Returns a value of type boolean
*/
@DISPID(117) //= 0x75. The runtime will prefer the VTID if present
@VTID(184)
boolean redo(
@Optional java.lang.Object times);
/**
* @param statistic Mandatory net.rgielen.com4j.office2010.word.WdStatistic parameter.
* @param includeFootnotesAndEndnotes Optional parameter. Default value is com4j.Variant.getMissing()
* @return Returns a value of type int
*/
@DISPID(118) //= 0x76. The runtime will prefer the VTID if present
@VTID(185)
int computeStatistics(
net.rgielen.com4j.office2010.word.WdStatistic statistic,
@Optional java.lang.Object includeFootnotesAndEndnotes);
/**
*/
@DISPID(119) //= 0x77. The runtime will prefer the VTID if present
@VTID(186)
void makeCompatibilityDefault();
/**
* @param type Mandatory net.rgielen.com4j.office2010.word.WdProtectionType parameter.
* @param noReset Optional parameter. Default value is com4j.Variant.getMissing()
* @param password Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(120) //= 0x78. The runtime will prefer the VTID if present
@VTID(187)
void protect2002(
net.rgielen.com4j.office2010.word.WdProtectionType type,
@Optional java.lang.Object noReset,
@Optional java.lang.Object password);
/**
* @param password Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(121) //= 0x79. The runtime will prefer the VTID if present
@VTID(188)
void unprotect(
@Optional java.lang.Object password);
/**
* @param type Mandatory net.rgielen.com4j.office2010.word.WdEditionType parameter.
* @param option Mandatory net.rgielen.com4j.office2010.word.WdEditionOption parameter.
* @param name Mandatory java.lang.String parameter.
* @param format Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(122) //= 0x7a. The runtime will prefer the VTID if present
@VTID(189)
void editionOptions(
net.rgielen.com4j.office2010.word.WdEditionType type,
net.rgielen.com4j.office2010.word.WdEditionOption option,
java.lang.String name,
@Optional java.lang.Object format);
/**
* @param letterContent Optional parameter. Default value is com4j.Variant.getMissing()
* @param wizardMode Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(123) //= 0x7b. The runtime will prefer the VTID if present
@VTID(190)
void runLetterWizard(
@Optional java.lang.Object letterContent,
@Optional java.lang.Object wizardMode);
/**
* @return Returns a value of type net.rgielen.com4j.office2010.word._LetterContent
*/
@DISPID(124) //= 0x7c. The runtime will prefer the VTID if present
@VTID(191)
net.rgielen.com4j.office2010.word._LetterContent getLetterContent();
/**
* @param letterContent Mandatory java.lang.Object parameter.
*/
@DISPID(125) //= 0x7d. The runtime will prefer the VTID if present
@VTID(192)
void setLetterContent(
java.lang.Object letterContent);
/**
* @param template Mandatory java.lang.String parameter.
*/
@DISPID(126) //= 0x7e. The runtime will prefer the VTID if present
@VTID(193)
void copyStylesFromTemplate(
java.lang.String template);
/**
*/
@DISPID(127) //= 0x7f. The runtime will prefer the VTID if present
@VTID(194)
void updateStyles();
/**
*/
@DISPID(131) //= 0x83. The runtime will prefer the VTID if present
@VTID(195)
void checkGrammar();
/**
* @param customDictionary Optional parameter. Default value is com4j.Variant.getMissing()
* @param ignoreUppercase Optional parameter. Default value is com4j.Variant.getMissing()
* @param alwaysSuggest Optional parameter. Default value is com4j.Variant.getMissing()
* @param customDictionary2 Optional parameter. Default value is com4j.Variant.getMissing()
* @param customDictionary3 Optional parameter. Default value is com4j.Variant.getMissing()
* @param customDictionary4 Optional parameter. Default value is com4j.Variant.getMissing()
* @param customDictionary5 Optional parameter. Default value is com4j.Variant.getMissing()
* @param customDictionary6 Optional parameter. Default value is com4j.Variant.getMissing()
* @param customDictionary7 Optional parameter. Default value is com4j.Variant.getMissing()
* @param customDictionary8 Optional parameter. Default value is com4j.Variant.getMissing()
* @param customDictionary9 Optional parameter. Default value is com4j.Variant.getMissing()
* @param customDictionary10 Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(132) //= 0x84. The runtime will prefer the VTID if present
@VTID(196)
void checkSpelling(
@Optional java.lang.Object customDictionary,
@Optional java.lang.Object ignoreUppercase,
@Optional java.lang.Object alwaysSuggest,
@Optional java.lang.Object customDictionary2,
@Optional java.lang.Object customDictionary3,
@Optional java.lang.Object customDictionary4,
@Optional java.lang.Object customDictionary5,
@Optional java.lang.Object customDictionary6,
@Optional java.lang.Object customDictionary7,
@Optional java.lang.Object customDictionary8,
@Optional java.lang.Object customDictionary9,
@Optional java.lang.Object customDictionary10);
/**
* @param address Optional parameter. Default value is com4j.Variant.getMissing()
* @param subAddress Optional parameter. Default value is com4j.Variant.getMissing()
* @param newWindow Optional parameter. Default value is com4j.Variant.getMissing()
* @param addHistory Optional parameter. Default value is com4j.Variant.getMissing()
* @param extraInfo Optional parameter. Default value is com4j.Variant.getMissing()
* @param method Optional parameter. Default value is com4j.Variant.getMissing()
* @param headerInfo Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(135) //= 0x87. The runtime will prefer the VTID if present
@VTID(197)
void followHyperlink(
@Optional java.lang.Object address,
@Optional java.lang.Object subAddress,
@Optional java.lang.Object newWindow,
@Optional java.lang.Object addHistory,
@Optional java.lang.Object extraInfo,
@Optional java.lang.Object method,
@Optional java.lang.Object headerInfo);
/**
*/
@DISPID(136) //= 0x88. The runtime will prefer the VTID if present
@VTID(198)
void addToFavorites();
/**
*/
@DISPID(137) //= 0x89. The runtime will prefer the VTID if present
@VTID(199)
void reload();
/**
* @param length Optional parameter. Default value is com4j.Variant.getMissing()
* @param mode Optional parameter. Default value is com4j.Variant.getMissing()
* @param updateProperties Optional parameter. Default value is com4j.Variant.getMissing()
* @return Returns a value of type net.rgielen.com4j.office2010.word.Range
*/
@DISPID(138) //= 0x8a. The runtime will prefer the VTID if present
@VTID(200)
net.rgielen.com4j.office2010.word.Range autoSummarize(
@Optional java.lang.Object length,
@Optional java.lang.Object mode,
@Optional java.lang.Object updateProperties);
/**
* @param numberType Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(140) //= 0x8c. The runtime will prefer the VTID if present
@VTID(201)
void removeNumbers(
@Optional java.lang.Object numberType);
/**
* @param numberType Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(141) //= 0x8d. The runtime will prefer the VTID if present
@VTID(202)
void convertNumbersToText(
@Optional java.lang.Object numberType);
/**
* @param numberType Optional parameter. Default value is com4j.Variant.getMissing()
* @param level Optional parameter. Default value is com4j.Variant.getMissing()
* @return Returns a value of type int
*/
@DISPID(142) //= 0x8e. The runtime will prefer the VTID if present
@VTID(203)
int countNumberedItems(
@Optional java.lang.Object numberType,
@Optional java.lang.Object level);
/**
*/
@DISPID(143) //= 0x8f. The runtime will prefer the VTID if present
@VTID(204)
void post();
/**
*/
@DISPID(144) //= 0x90. The runtime will prefer the VTID if present
@VTID(205)
void toggleFormsDesign();
/**
* @param name Mandatory java.lang.String parameter.
*/
@DISPID(145) //= 0x91. The runtime will prefer the VTID if present
@VTID(206)
void compare2000(
java.lang.String name);
/**
*/
@DISPID(146) //= 0x92. The runtime will prefer the VTID if present
@VTID(207)
void updateSummaryProperties();
/**
* @param referenceType Mandatory java.lang.Object parameter.
* @return Returns a value of type java.lang.Object
*/
@DISPID(147) //= 0x93. The runtime will prefer the VTID if present
@VTID(208)
@ReturnValue(type=NativeType.VARIANT)
java.lang.Object getCrossReferenceItems(
java.lang.Object referenceType);
/**
*/
@DISPID(148) //= 0x94. The runtime will prefer the VTID if present
@VTID(209)
void autoFormat();
/**
*/
@DISPID(149) //= 0x95. The runtime will prefer the VTID if present
@VTID(210)
void viewCode();
/**
*/
@DISPID(150) //= 0x96. The runtime will prefer the VTID if present
@VTID(211)
void viewPropertyBrowser();
/**
*/
@DISPID(250) //= 0xfa. The runtime will prefer the VTID if present
@VTID(212)
void forwardMailer();
/**
*/
@DISPID(251) //= 0xfb. The runtime will prefer the VTID if present
@VTID(213)
void reply();
/**
*/
@DISPID(252) //= 0xfc. The runtime will prefer the VTID if present
@VTID(214)
void replyAll();
/**
* @param fileFormat Optional parameter. Default value is com4j.Variant.getMissing()
* @param priority Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(253) //= 0xfd. The runtime will prefer the VTID if present
@VTID(215)
void sendMailer(
@Optional java.lang.Object fileFormat,
@Optional java.lang.Object priority);
/**
*/
@DISPID(254) //= 0xfe. The runtime will prefer the VTID if present
@VTID(216)
void undoClear();
/**
*/
@DISPID(255) //= 0xff. The runtime will prefer the VTID if present
@VTID(217)
void presentIt();
/**
* @param address Mandatory java.lang.String parameter.
* @param subject Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(256) //= 0x100. The runtime will prefer the VTID if present
@VTID(218)
void sendFax(
java.lang.String address,
@Optional java.lang.Object subject);
/**
* @param fileName Mandatory java.lang.String parameter.
*/
@DISPID(257) //= 0x101. The runtime will prefer the VTID if present
@VTID(219)
void merge2000(
java.lang.String fileName);
/**
*/
@DISPID(258) //= 0x102. The runtime will prefer the VTID if present
@VTID(220)
void closePrintPreview();
/**
*/
@DISPID(259) //= 0x103. The runtime will prefer the VTID if present
@VTID(221)
void checkConsistency();
/**
* @param dateFormat Mandatory java.lang.String parameter.
* @param includeHeaderFooter Mandatory boolean parameter.
* @param pageDesign Mandatory java.lang.String parameter.
* @param letterStyle Mandatory net.rgielen.com4j.office2010.word.WdLetterStyle parameter.
* @param letterhead Mandatory boolean parameter.
* @param letterheadLocation Mandatory net.rgielen.com4j.office2010.word.WdLetterheadLocation parameter.
* @param letterheadSize Mandatory float parameter.
* @param recipientName Mandatory java.lang.String parameter.
* @param recipientAddress Mandatory java.lang.String parameter.
* @param salutation Mandatory java.lang.String parameter.
* @param salutationType Mandatory net.rgielen.com4j.office2010.word.WdSalutationType parameter.
* @param recipientReference Mandatory java.lang.String parameter.
* @param mailingInstructions Mandatory java.lang.String parameter.
* @param attentionLine Mandatory java.lang.String parameter.
* @param subject Mandatory java.lang.String parameter.
* @param ccList Mandatory java.lang.String parameter.
* @param returnAddress Mandatory java.lang.String parameter.
* @param senderName Mandatory java.lang.String parameter.
* @param closing Mandatory java.lang.String parameter.
* @param senderCompany Mandatory java.lang.String parameter.
* @param senderJobTitle Mandatory java.lang.String parameter.
* @param senderInitials Mandatory java.lang.String parameter.
* @param enclosureNumber Mandatory int parameter.
* @param infoBlock Optional parameter. Default value is com4j.Variant.getMissing()
* @param recipientCode Optional parameter. Default value is com4j.Variant.getMissing()
* @param recipientGender Optional parameter. Default value is com4j.Variant.getMissing()
* @param returnAddressShortForm Optional parameter. Default value is com4j.Variant.getMissing()
* @param senderCity Optional parameter. Default value is com4j.Variant.getMissing()
* @param senderCode Optional parameter. Default value is com4j.Variant.getMissing()
* @param senderGender Optional parameter. Default value is com4j.Variant.getMissing()
* @param senderReference Optional parameter. Default value is com4j.Variant.getMissing()
* @return Returns a value of type net.rgielen.com4j.office2010.word._LetterContent
*/
@DISPID(260) //= 0x104. The runtime will prefer the VTID if present
@VTID(222)
net.rgielen.com4j.office2010.word._LetterContent createLetterContent(
java.lang.String dateFormat,
boolean includeHeaderFooter,
java.lang.String pageDesign,
net.rgielen.com4j.office2010.word.WdLetterStyle letterStyle,
boolean letterhead,
net.rgielen.com4j.office2010.word.WdLetterheadLocation letterheadLocation,
float letterheadSize,
java.lang.String recipientName,
java.lang.String recipientAddress,
java.lang.String salutation,
net.rgielen.com4j.office2010.word.WdSalutationType salutationType,
java.lang.String recipientReference,
java.lang.String mailingInstructions,
java.lang.String attentionLine,
java.lang.String subject,
java.lang.String ccList,
java.lang.String returnAddress,
java.lang.String senderName,
java.lang.String closing,
java.lang.String senderCompany,
java.lang.String senderJobTitle,
java.lang.String senderInitials,
int enclosureNumber,
@Optional java.lang.Object infoBlock,
@Optional java.lang.Object recipientCode,
@Optional java.lang.Object recipientGender,
@Optional java.lang.Object returnAddressShortForm,
@Optional java.lang.Object senderCity,
@Optional java.lang.Object senderCode,
@Optional java.lang.Object senderGender,
@Optional java.lang.Object senderReference);
/**
*/
@DISPID(317) //= 0x13d. The runtime will prefer the VTID if present
@VTID(223)
void acceptAllRevisions();
/**
*/
@DISPID(318) //= 0x13e. The runtime will prefer the VTID if present
@VTID(224)
void rejectAllRevisions();
/**
*/
@DISPID(151) //= 0x97. The runtime will prefer the VTID if present
@VTID(225)
void detectLanguage();
/**
* @param name Mandatory java.lang.String parameter.
*/
@DISPID(322) //= 0x142. The runtime will prefer the VTID if present
@VTID(226)
void applyTheme(
java.lang.String name);
/**
*/
@DISPID(323) //= 0x143. The runtime will prefer the VTID if present
@VTID(227)
void removeTheme();
/**
*/
@DISPID(325) //= 0x145. The runtime will prefer the VTID if present
@VTID(228)
void webPagePreview();
/**
* @param encoding Mandatory net.rgielen.com4j.office2010.office.MsoEncoding parameter.
*/
@DISPID(331) //= 0x14b. The runtime will prefer the VTID if present
@VTID(229)
void reloadAs(
net.rgielen.com4j.office2010.office.MsoEncoding encoding);
/**
*
* Getter method for the COM property "ActiveTheme"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(540) //= 0x21c. The runtime will prefer the VTID if present
@VTID(230)
java.lang.String activeTheme();
/**
*
* Getter method for the COM property "ActiveThemeDisplayName"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(541) //= 0x21d. The runtime will prefer the VTID if present
@VTID(231)
java.lang.String activeThemeDisplayName();
/**
*
* Getter method for the COM property "Email"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Email
*/
@DISPID(319) //= 0x13f. The runtime will prefer the VTID if present
@VTID(232)
net.rgielen.com4j.office2010.word.Email email();
/**
*
* Getter method for the COM property "Scripts"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.Scripts
*/
@DISPID(320) //= 0x140. The runtime will prefer the VTID if present
@VTID(233)
net.rgielen.com4j.office2010.office.Scripts scripts();
@VTID(233)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.office.Scripts.class})
net.rgielen.com4j.office2010.office.Script scripts(
@MarshalAs(NativeType.VARIANT) java.lang.Object index);
/**
*
* Getter method for the COM property "LanguageDetected"
*
* @return Returns a value of type boolean
*/
@DISPID(321) //= 0x141. The runtime will prefer the VTID if present
@VTID(234)
boolean languageDetected();
/**
*
* Setter method for the COM property "LanguageDetected"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(321) //= 0x141. The runtime will prefer the VTID if present
@VTID(235)
void languageDetected(
boolean prop);
/**
*
* Getter method for the COM property "FarEastLineBreakLanguage"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdFarEastLineBreakLanguageID
*/
@DISPID(326) //= 0x146. The runtime will prefer the VTID if present
@VTID(236)
net.rgielen.com4j.office2010.word.WdFarEastLineBreakLanguageID farEastLineBreakLanguage();
/**
*
* Setter method for the COM property "FarEastLineBreakLanguage"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdFarEastLineBreakLanguageID parameter.
*/
@DISPID(326) //= 0x146. The runtime will prefer the VTID if present
@VTID(237)
void farEastLineBreakLanguage(
net.rgielen.com4j.office2010.word.WdFarEastLineBreakLanguageID prop);
/**
*
* Getter method for the COM property "Frameset"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Frameset
*/
@DISPID(327) //= 0x147. The runtime will prefer the VTID if present
@VTID(238)
net.rgielen.com4j.office2010.word.Frameset frameset();
/**
*
* Getter method for the COM property "ClickAndTypeParagraphStyle"
*
* @return Returns a value of type java.lang.Object
*/
@DISPID(328) //= 0x148. The runtime will prefer the VTID if present
@VTID(239)
@ReturnValue(type=NativeType.VARIANT)
java.lang.Object clickAndTypeParagraphStyle();
/**
*
* Setter method for the COM property "ClickAndTypeParagraphStyle"
*
* @param prop Mandatory java.lang.Object parameter.
*/
@DISPID(328) //= 0x148. The runtime will prefer the VTID if present
@VTID(240)
void clickAndTypeParagraphStyle(
java.lang.Object prop);
/**
*
* Getter method for the COM property "HTMLProject"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.HTMLProject
*/
@DISPID(329) //= 0x149. The runtime will prefer the VTID if present
@VTID(241)
net.rgielen.com4j.office2010.office.HTMLProject htmlProject();
/**
*
* Getter method for the COM property "WebOptions"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WebOptions
*/
@DISPID(330) //= 0x14a. The runtime will prefer the VTID if present
@VTID(242)
net.rgielen.com4j.office2010.word.WebOptions webOptions();
/**
*
* Getter method for the COM property "OpenEncoding"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.MsoEncoding
*/
@DISPID(332) //= 0x14c. The runtime will prefer the VTID if present
@VTID(243)
net.rgielen.com4j.office2010.office.MsoEncoding openEncoding();
/**
*
* Getter method for the COM property "SaveEncoding"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.MsoEncoding
*/
@DISPID(333) //= 0x14d. The runtime will prefer the VTID if present
@VTID(244)
net.rgielen.com4j.office2010.office.MsoEncoding saveEncoding();
/**
*
* Setter method for the COM property "SaveEncoding"
*
* @param prop Mandatory net.rgielen.com4j.office2010.office.MsoEncoding parameter.
*/
@DISPID(333) //= 0x14d. The runtime will prefer the VTID if present
@VTID(245)
void saveEncoding(
net.rgielen.com4j.office2010.office.MsoEncoding prop);
/**
*
* Getter method for the COM property "OptimizeForWord97"
*
* @return Returns a value of type boolean
*/
@DISPID(334) //= 0x14e. The runtime will prefer the VTID if present
@VTID(246)
boolean optimizeForWord97();
/**
*
* Setter method for the COM property "OptimizeForWord97"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(334) //= 0x14e. The runtime will prefer the VTID if present
@VTID(247)
void optimizeForWord97(
boolean prop);
/**
*
* Getter method for the COM property "VBASigned"
*
* @return Returns a value of type boolean
*/
@DISPID(335) //= 0x14f. The runtime will prefer the VTID if present
@VTID(248)
boolean vbaSigned();
/**
* @param background Optional parameter. Default value is com4j.Variant.getMissing()
* @param append Optional parameter. Default value is com4j.Variant.getMissing()
* @param range Optional parameter. Default value is com4j.Variant.getMissing()
* @param outputFileName Optional parameter. Default value is com4j.Variant.getMissing()
* @param from Optional parameter. Default value is com4j.Variant.getMissing()
* @param to Optional parameter. Default value is com4j.Variant.getMissing()
* @param item Optional parameter. Default value is com4j.Variant.getMissing()
* @param copies Optional parameter. Default value is com4j.Variant.getMissing()
* @param pages Optional parameter. Default value is com4j.Variant.getMissing()
* @param pageType Optional parameter. Default value is com4j.Variant.getMissing()
* @param printToFile Optional parameter. Default value is com4j.Variant.getMissing()
* @param collate Optional parameter. Default value is com4j.Variant.getMissing()
* @param activePrinterMacGX Optional parameter. Default value is com4j.Variant.getMissing()
* @param manualDuplexPrint Optional parameter. Default value is com4j.Variant.getMissing()
* @param printZoomColumn Optional parameter. Default value is com4j.Variant.getMissing()
* @param printZoomRow Optional parameter. Default value is com4j.Variant.getMissing()
* @param printZoomPaperWidth Optional parameter. Default value is com4j.Variant.getMissing()
* @param printZoomPaperHeight Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(444) //= 0x1bc. The runtime will prefer the VTID if present
@VTID(249)
void printOut2000(
@Optional java.lang.Object background,
@Optional java.lang.Object append,
@Optional java.lang.Object range,
@Optional java.lang.Object outputFileName,
@Optional java.lang.Object from,
@Optional java.lang.Object to,
@Optional java.lang.Object item,
@Optional java.lang.Object copies,
@Optional java.lang.Object pages,
@Optional java.lang.Object pageType,
@Optional java.lang.Object printToFile,
@Optional java.lang.Object collate,
@Optional java.lang.Object activePrinterMacGX,
@Optional java.lang.Object manualDuplexPrint,
@Optional java.lang.Object printZoomColumn,
@Optional java.lang.Object printZoomRow,
@Optional java.lang.Object printZoomPaperWidth,
@Optional java.lang.Object printZoomPaperHeight);
/**
* @param s Mandatory java.lang.String parameter.
*/
@DISPID(445) //= 0x1bd. The runtime will prefer the VTID if present
@VTID(250)
void sblt(
java.lang.String s);
/**
* @param codePageOrigin Mandatory int parameter.
*/
@DISPID(447) //= 0x1bf. The runtime will prefer the VTID if present
@VTID(251)
void convertVietDoc(
int codePageOrigin);
/**
* @param background Optional parameter. Default value is com4j.Variant.getMissing()
* @param append Optional parameter. Default value is com4j.Variant.getMissing()
* @param range Optional parameter. Default value is com4j.Variant.getMissing()
* @param outputFileName Optional parameter. Default value is com4j.Variant.getMissing()
* @param from Optional parameter. Default value is com4j.Variant.getMissing()
* @param to Optional parameter. Default value is com4j.Variant.getMissing()
* @param item Optional parameter. Default value is com4j.Variant.getMissing()
* @param copies Optional parameter. Default value is com4j.Variant.getMissing()
* @param pages Optional parameter. Default value is com4j.Variant.getMissing()
* @param pageType Optional parameter. Default value is com4j.Variant.getMissing()
* @param printToFile Optional parameter. Default value is com4j.Variant.getMissing()
* @param collate Optional parameter. Default value is com4j.Variant.getMissing()
* @param activePrinterMacGX Optional parameter. Default value is com4j.Variant.getMissing()
* @param manualDuplexPrint Optional parameter. Default value is com4j.Variant.getMissing()
* @param printZoomColumn Optional parameter. Default value is com4j.Variant.getMissing()
* @param printZoomRow Optional parameter. Default value is com4j.Variant.getMissing()
* @param printZoomPaperWidth Optional parameter. Default value is com4j.Variant.getMissing()
* @param printZoomPaperHeight Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(446) //= 0x1be. The runtime will prefer the VTID if present
@VTID(252)
void printOut(
@Optional java.lang.Object background,
@Optional java.lang.Object append,
@Optional java.lang.Object range,
@Optional java.lang.Object outputFileName,
@Optional java.lang.Object from,
@Optional java.lang.Object to,
@Optional java.lang.Object item,
@Optional java.lang.Object copies,
@Optional java.lang.Object pages,
@Optional java.lang.Object pageType,
@Optional java.lang.Object printToFile,
@Optional java.lang.Object collate,
@Optional java.lang.Object activePrinterMacGX,
@Optional java.lang.Object manualDuplexPrint,
@Optional java.lang.Object printZoomColumn,
@Optional java.lang.Object printZoomRow,
@Optional java.lang.Object printZoomPaperWidth,
@Optional java.lang.Object printZoomPaperHeight);
/**
*
* Getter method for the COM property "MailEnvelope"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.IMsoEnvelopeVB
*/
@DISPID(336) //= 0x150. The runtime will prefer the VTID if present
@VTID(253)
net.rgielen.com4j.office2010.office.IMsoEnvelopeVB mailEnvelope();
/**
*
* Getter method for the COM property "DisableFeatures"
*
* @return Returns a value of type boolean
*/
@DISPID(337) //= 0x151. The runtime will prefer the VTID if present
@VTID(254)
boolean disableFeatures();
/**
*
* Setter method for the COM property "DisableFeatures"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(337) //= 0x151. The runtime will prefer the VTID if present
@VTID(255)
void disableFeatures(
boolean prop);
/**
*
* Getter method for the COM property "DoNotEmbedSystemFonts"
*
* @return Returns a value of type boolean
*/
@DISPID(338) //= 0x152. The runtime will prefer the VTID if present
@VTID(256)
boolean doNotEmbedSystemFonts();
/**
*
* Setter method for the COM property "DoNotEmbedSystemFonts"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(338) //= 0x152. The runtime will prefer the VTID if present
@VTID(257)
void doNotEmbedSystemFonts(
boolean prop);
/**
*
* Getter method for the COM property "Signatures"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.SignatureSet
*/
@DISPID(339) //= 0x153. The runtime will prefer the VTID if present
@VTID(258)
net.rgielen.com4j.office2010.office.SignatureSet signatures();
@VTID(258)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.office.SignatureSet.class})
net.rgielen.com4j.office2010.office.Signature signatures(
int iSig);
/**
*
* Getter method for the COM property "DefaultTargetFrame"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(340) //= 0x154. The runtime will prefer the VTID if present
@VTID(259)
java.lang.String defaultTargetFrame();
/**
*
* Setter method for the COM property "DefaultTargetFrame"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(340) //= 0x154. The runtime will prefer the VTID if present
@VTID(260)
void defaultTargetFrame(
java.lang.String prop);
/**
*
* Getter method for the COM property "HTMLDivisions"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.HTMLDivisions
*/
@DISPID(342) //= 0x156. The runtime will prefer the VTID if present
@VTID(261)
net.rgielen.com4j.office2010.word.HTMLDivisions htmlDivisions();
@VTID(261)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.HTMLDivisions.class})
net.rgielen.com4j.office2010.word.HTMLDivision htmlDivisions(
int index);
/**
*
* Getter method for the COM property "DisableFeaturesIntroducedAfter"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdDisableFeaturesIntroducedAfter
*/
@DISPID(343) //= 0x157. The runtime will prefer the VTID if present
@VTID(262)
net.rgielen.com4j.office2010.word.WdDisableFeaturesIntroducedAfter disableFeaturesIntroducedAfter();
/**
*
* Setter method for the COM property "DisableFeaturesIntroducedAfter"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdDisableFeaturesIntroducedAfter parameter.
*/
@DISPID(343) //= 0x157. The runtime will prefer the VTID if present
@VTID(263)
void disableFeaturesIntroducedAfter(
net.rgielen.com4j.office2010.word.WdDisableFeaturesIntroducedAfter prop);
/**
*
* Getter method for the COM property "RemovePersonalInformation"
*
* @return Returns a value of type boolean
*/
@DISPID(344) //= 0x158. The runtime will prefer the VTID if present
@VTID(264)
boolean removePersonalInformation();
/**
*
* Setter method for the COM property "RemovePersonalInformation"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(344) //= 0x158. The runtime will prefer the VTID if present
@VTID(265)
void removePersonalInformation(
boolean prop);
/**
*
* Getter method for the COM property "SmartTags"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.SmartTags
*/
@DISPID(346) //= 0x15a. The runtime will prefer the VTID if present
@VTID(266)
net.rgielen.com4j.office2010.word.SmartTags smartTags();
@VTID(266)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.SmartTags.class})
net.rgielen.com4j.office2010.word.SmartTag smartTags(
java.lang.Object index);
/**
* @param name Mandatory java.lang.String parameter.
* @param authorName Optional parameter. Default value is com4j.Variant.getMissing()
* @param compareTarget Optional parameter. Default value is com4j.Variant.getMissing()
* @param detectFormatChanges Optional parameter. Default value is com4j.Variant.getMissing()
* @param ignoreAllComparisonWarnings Optional parameter. Default value is com4j.Variant.getMissing()
* @param addToRecentFiles Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(345) //= 0x159. The runtime will prefer the VTID if present
@VTID(267)
void compare2002(
java.lang.String name,
@Optional java.lang.Object authorName,
@Optional java.lang.Object compareTarget,
@Optional java.lang.Object detectFormatChanges,
@Optional java.lang.Object ignoreAllComparisonWarnings,
@Optional java.lang.Object addToRecentFiles);
/**
* @param saveChanges Optional parameter. Default value is false
* @param comments Optional parameter. Default value is com4j.Variant.getMissing()
* @param makePublic Optional parameter. Default value is false
*/
@DISPID(349) //= 0x15d. The runtime will prefer the VTID if present
@VTID(268)
void checkIn(
@Optional @DefaultValue("-1") boolean saveChanges,
@Optional java.lang.Object comments,
@Optional @DefaultValue("0") boolean makePublic);
/**
* @return Returns a value of type boolean
*/
@DISPID(351) //= 0x15f. The runtime will prefer the VTID if present
@VTID(269)
boolean canCheckin();
/**
* @param fileName Mandatory java.lang.String parameter.
* @param mergeTarget Optional parameter. Default value is com4j.Variant.getMissing()
* @param detectFormatChanges Optional parameter. Default value is com4j.Variant.getMissing()
* @param useFormattingFrom Optional parameter. Default value is com4j.Variant.getMissing()
* @param addToRecentFiles Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(362) //= 0x16a. The runtime will prefer the VTID if present
@VTID(270)
void merge(
java.lang.String fileName,
@Optional java.lang.Object mergeTarget,
@Optional java.lang.Object detectFormatChanges,
@Optional java.lang.Object useFormattingFrom,
@Optional java.lang.Object addToRecentFiles);
/**
*
* Getter method for the COM property "EmbedSmartTags"
*
* @return Returns a value of type boolean
*/
@DISPID(347) //= 0x15b. The runtime will prefer the VTID if present
@VTID(271)
boolean embedSmartTags();
/**
*
* Setter method for the COM property "EmbedSmartTags"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(347) //= 0x15b. The runtime will prefer the VTID if present
@VTID(272)
void embedSmartTags(
boolean prop);
/**
*
* Getter method for the COM property "SmartTagsAsXMLProps"
*
* @return Returns a value of type boolean
*/
@DISPID(348) //= 0x15c. The runtime will prefer the VTID if present
@VTID(273)
boolean smartTagsAsXMLProps();
/**
*
* Setter method for the COM property "SmartTagsAsXMLProps"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(348) //= 0x15c. The runtime will prefer the VTID if present
@VTID(274)
void smartTagsAsXMLProps(
boolean prop);
/**
*
* Getter method for the COM property "TextEncoding"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.MsoEncoding
*/
@DISPID(357) //= 0x165. The runtime will prefer the VTID if present
@VTID(275)
net.rgielen.com4j.office2010.office.MsoEncoding textEncoding();
/**
*
* Setter method for the COM property "TextEncoding"
*
* @param prop Mandatory net.rgielen.com4j.office2010.office.MsoEncoding parameter.
*/
@DISPID(357) //= 0x165. The runtime will prefer the VTID if present
@VTID(276)
void textEncoding(
net.rgielen.com4j.office2010.office.MsoEncoding prop);
/**
*
* Getter method for the COM property "TextLineEnding"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdLineEndingType
*/
@DISPID(358) //= 0x166. The runtime will prefer the VTID if present
@VTID(277)
net.rgielen.com4j.office2010.word.WdLineEndingType textLineEnding();
/**
*
* Setter method for the COM property "TextLineEnding"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdLineEndingType parameter.
*/
@DISPID(358) //= 0x166. The runtime will prefer the VTID if present
@VTID(278)
void textLineEnding(
net.rgielen.com4j.office2010.word.WdLineEndingType prop);
/**
* @param recipients Optional parameter. Default value is com4j.Variant.getMissing()
* @param subject Optional parameter. Default value is com4j.Variant.getMissing()
* @param showMessage Optional parameter. Default value is com4j.Variant.getMissing()
* @param includeAttachment Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(353) //= 0x161. The runtime will prefer the VTID if present
@VTID(279)
void sendForReview(
@Optional java.lang.Object recipients,
@Optional java.lang.Object subject,
@Optional java.lang.Object showMessage,
@Optional java.lang.Object includeAttachment);
/**
* @param showMessage Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(354) //= 0x162. The runtime will prefer the VTID if present
@VTID(280)
void replyWithChanges(
@Optional java.lang.Object showMessage);
/**
*/
@DISPID(356) //= 0x164. The runtime will prefer the VTID if present
@VTID(281)
void endReview();
/**
*
* Getter method for the COM property "StyleSheets"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.StyleSheets
*/
@DISPID(360) //= 0x168. The runtime will prefer the VTID if present
@VTID(282)
net.rgielen.com4j.office2010.word.StyleSheets styleSheets();
@VTID(282)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.StyleSheets.class})
net.rgielen.com4j.office2010.word.StyleSheet styleSheets(
java.lang.Object index);
/**
*
* Getter method for the COM property "DefaultTableStyle"
*
* @return Returns a value of type java.lang.Object
*/
@DISPID(365) //= 0x16d. The runtime will prefer the VTID if present
@VTID(283)
@ReturnValue(type=NativeType.VARIANT)
java.lang.Object defaultTableStyle();
/**
*
* Getter method for the COM property "PasswordEncryptionProvider"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(367) //= 0x16f. The runtime will prefer the VTID if present
@VTID(284)
java.lang.String passwordEncryptionProvider();
/**
*
* Getter method for the COM property "PasswordEncryptionAlgorithm"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(368) //= 0x170. The runtime will prefer the VTID if present
@VTID(285)
java.lang.String passwordEncryptionAlgorithm();
/**
*
* Getter method for the COM property "PasswordEncryptionKeyLength"
*
* @return Returns a value of type int
*/
@DISPID(369) //= 0x171. The runtime will prefer the VTID if present
@VTID(286)
int passwordEncryptionKeyLength();
/**
*
* Getter method for the COM property "PasswordEncryptionFileProperties"
*
* @return Returns a value of type boolean
*/
@DISPID(370) //= 0x172. The runtime will prefer the VTID if present
@VTID(287)
boolean passwordEncryptionFileProperties();
/**
* @param passwordEncryptionProvider Mandatory java.lang.String parameter.
* @param passwordEncryptionAlgorithm Mandatory java.lang.String parameter.
* @param passwordEncryptionKeyLength Mandatory int parameter.
* @param passwordEncryptionFileProperties Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(361) //= 0x169. The runtime will prefer the VTID if present
@VTID(288)
void setPasswordEncryptionOptions(
java.lang.String passwordEncryptionProvider,
java.lang.String passwordEncryptionAlgorithm,
int passwordEncryptionKeyLength,
@Optional java.lang.Object passwordEncryptionFileProperties);
/**
*/
@DISPID(363) //= 0x16b. The runtime will prefer the VTID if present
@VTID(289)
void recheckSmartTags();
/**
*/
@DISPID(364) //= 0x16c. The runtime will prefer the VTID if present
@VTID(290)
void removeSmartTags();
/**
* @param style Mandatory java.lang.Object parameter.
* @param setInTemplate Mandatory boolean parameter.
*/
@DISPID(366) //= 0x16e. The runtime will prefer the VTID if present
@VTID(291)
void setDefaultTableStyle(
java.lang.Object style,
boolean setInTemplate);
/**
*/
@DISPID(371) //= 0x173. The runtime will prefer the VTID if present
@VTID(292)
void deleteAllComments();
/**
*/
@DISPID(372) //= 0x174. The runtime will prefer the VTID if present
@VTID(293)
void acceptAllRevisionsShown();
/**
*/
@DISPID(373) //= 0x175. The runtime will prefer the VTID if present
@VTID(294)
void rejectAllRevisionsShown();
/**
*/
@DISPID(374) //= 0x176. The runtime will prefer the VTID if present
@VTID(295)
void deleteAllCommentsShown();
/**
*/
@DISPID(375) //= 0x177. The runtime will prefer the VTID if present
@VTID(296)
void resetFormFields();
/**
* @param fileName Optional parameter. Default value is com4j.Variant.getMissing()
* @param fileFormat Optional parameter. Default value is com4j.Variant.getMissing()
* @param lockComments Optional parameter. Default value is com4j.Variant.getMissing()
* @param password Optional parameter. Default value is com4j.Variant.getMissing()
* @param addToRecentFiles Optional parameter. Default value is com4j.Variant.getMissing()
* @param writePassword Optional parameter. Default value is com4j.Variant.getMissing()
* @param readOnlyRecommended Optional parameter. Default value is com4j.Variant.getMissing()
* @param embedTrueTypeFonts Optional parameter. Default value is com4j.Variant.getMissing()
* @param saveNativePictureFormat Optional parameter. Default value is com4j.Variant.getMissing()
* @param saveFormsData Optional parameter. Default value is com4j.Variant.getMissing()
* @param saveAsAOCELetter Optional parameter. Default value is com4j.Variant.getMissing()
* @param encoding Optional parameter. Default value is com4j.Variant.getMissing()
* @param insertLineBreaks Optional parameter. Default value is com4j.Variant.getMissing()
* @param allowSubstitutions Optional parameter. Default value is com4j.Variant.getMissing()
* @param lineEnding Optional parameter. Default value is com4j.Variant.getMissing()
* @param addBiDiMarks Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(376) //= 0x178. The runtime will prefer the VTID if present
@VTID(297)
void saveAs(
@Optional java.lang.Object fileName,
@Optional java.lang.Object fileFormat,
@Optional java.lang.Object lockComments,
@Optional java.lang.Object password,
@Optional java.lang.Object addToRecentFiles,
@Optional java.lang.Object writePassword,
@Optional java.lang.Object readOnlyRecommended,
@Optional java.lang.Object embedTrueTypeFonts,
@Optional java.lang.Object saveNativePictureFormat,
@Optional java.lang.Object saveFormsData,
@Optional java.lang.Object saveAsAOCELetter,
@Optional java.lang.Object encoding,
@Optional java.lang.Object insertLineBreaks,
@Optional java.lang.Object allowSubstitutions,
@Optional java.lang.Object lineEnding,
@Optional java.lang.Object addBiDiMarks);
/**
*
* Getter method for the COM property "EmbedLinguisticData"
*
* @return Returns a value of type boolean
*/
@DISPID(377) //= 0x179. The runtime will prefer the VTID if present
@VTID(298)
boolean embedLinguisticData();
/**
*
* Setter method for the COM property "EmbedLinguisticData"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(377) //= 0x179. The runtime will prefer the VTID if present
@VTID(299)
void embedLinguisticData(
boolean prop);
/**
*
* Getter method for the COM property "FormattingShowFont"
*
* @return Returns a value of type boolean
*/
@DISPID(448) //= 0x1c0. The runtime will prefer the VTID if present
@VTID(300)
boolean formattingShowFont();
/**
*
* Setter method for the COM property "FormattingShowFont"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(448) //= 0x1c0. The runtime will prefer the VTID if present
@VTID(301)
void formattingShowFont(
boolean prop);
/**
*
* Getter method for the COM property "FormattingShowClear"
*
* @return Returns a value of type boolean
*/
@DISPID(449) //= 0x1c1. The runtime will prefer the VTID if present
@VTID(302)
boolean formattingShowClear();
/**
*
* Setter method for the COM property "FormattingShowClear"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(449) //= 0x1c1. The runtime will prefer the VTID if present
@VTID(303)
void formattingShowClear(
boolean prop);
/**
*
* Getter method for the COM property "FormattingShowParagraph"
*
* @return Returns a value of type boolean
*/
@DISPID(450) //= 0x1c2. The runtime will prefer the VTID if present
@VTID(304)
boolean formattingShowParagraph();
/**
*
* Setter method for the COM property "FormattingShowParagraph"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(450) //= 0x1c2. The runtime will prefer the VTID if present
@VTID(305)
void formattingShowParagraph(
boolean prop);
/**
*
* Getter method for the COM property "FormattingShowNumbering"
*
* @return Returns a value of type boolean
*/
@DISPID(451) //= 0x1c3. The runtime will prefer the VTID if present
@VTID(306)
boolean formattingShowNumbering();
/**
*
* Setter method for the COM property "FormattingShowNumbering"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(451) //= 0x1c3. The runtime will prefer the VTID if present
@VTID(307)
void formattingShowNumbering(
boolean prop);
/**
*
* Getter method for the COM property "FormattingShowFilter"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdShowFilter
*/
@DISPID(452) //= 0x1c4. The runtime will prefer the VTID if present
@VTID(308)
net.rgielen.com4j.office2010.word.WdShowFilter formattingShowFilter();
/**
*
* Setter method for the COM property "FormattingShowFilter"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdShowFilter parameter.
*/
@DISPID(452) //= 0x1c4. The runtime will prefer the VTID if present
@VTID(309)
void formattingShowFilter(
net.rgielen.com4j.office2010.word.WdShowFilter prop);
/**
*/
@DISPID(378) //= 0x17a. The runtime will prefer the VTID if present
@VTID(310)
void checkNewSmartTags();
/**
*
* Getter method for the COM property "Permission"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.Permission
*/
@DISPID(453) //= 0x1c5. The runtime will prefer the VTID if present
@VTID(311)
net.rgielen.com4j.office2010.office.Permission permission();
@VTID(311)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.office.Permission.class})
net.rgielen.com4j.office2010.office.UserPermission permission(
@MarshalAs(NativeType.VARIANT) java.lang.Object index);
/**
*
* Getter method for the COM property "XMLNodes"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.XMLNodes
*/
@DISPID(460) //= 0x1cc. The runtime will prefer the VTID if present
@VTID(312)
net.rgielen.com4j.office2010.word.XMLNodes xmlNodes();
@VTID(312)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.XMLNodes.class})
net.rgielen.com4j.office2010.word.XMLNode xmlNodes(
int index);
/**
*
* Getter method for the COM property "XMLSchemaReferences"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.XMLSchemaReferences
*/
@DISPID(461) //= 0x1cd. The runtime will prefer the VTID if present
@VTID(313)
net.rgielen.com4j.office2010.word.XMLSchemaReferences xmlSchemaReferences();
@VTID(313)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.XMLSchemaReferences.class})
net.rgielen.com4j.office2010.word.XMLSchemaReference xmlSchemaReferences(
java.lang.Object index);
/**
*
* Getter method for the COM property "SmartDocument"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.SmartDocument
*/
@DISPID(462) //= 0x1ce. The runtime will prefer the VTID if present
@VTID(314)
net.rgielen.com4j.office2010.office.SmartDocument smartDocument();
/**
*
* Getter method for the COM property "SharedWorkspace"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.SharedWorkspace
*/
@DISPID(463) //= 0x1cf. The runtime will prefer the VTID if present
@VTID(315)
net.rgielen.com4j.office2010.office.SharedWorkspace sharedWorkspace();
/**
*
* Getter method for the COM property "Sync"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.Sync
*/
@DISPID(466) //= 0x1d2. The runtime will prefer the VTID if present
@VTID(316)
net.rgielen.com4j.office2010.office.Sync sync();
/**
*
* Getter method for the COM property "EnforceStyle"
*
* @return Returns a value of type boolean
*/
@DISPID(471) //= 0x1d7. The runtime will prefer the VTID if present
@VTID(317)
boolean enforceStyle();
/**
*
* Setter method for the COM property "EnforceStyle"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(471) //= 0x1d7. The runtime will prefer the VTID if present
@VTID(318)
void enforceStyle(
boolean prop);
/**
*
* Getter method for the COM property "AutoFormatOverride"
*
* @return Returns a value of type boolean
*/
@DISPID(472) //= 0x1d8. The runtime will prefer the VTID if present
@VTID(319)
boolean autoFormatOverride();
/**
*
* Setter method for the COM property "AutoFormatOverride"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(472) //= 0x1d8. The runtime will prefer the VTID if present
@VTID(320)
void autoFormatOverride(
boolean prop);
/**
*
* Getter method for the COM property "XMLSaveDataOnly"
*
* @return Returns a value of type boolean
*/
@DISPID(473) //= 0x1d9. The runtime will prefer the VTID if present
@VTID(321)
boolean xmlSaveDataOnly();
/**
*
* Setter method for the COM property "XMLSaveDataOnly"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(473) //= 0x1d9. The runtime will prefer the VTID if present
@VTID(322)
void xmlSaveDataOnly(
boolean prop);
/**
*
* Getter method for the COM property "XMLHideNamespaces"
*
* @return Returns a value of type boolean
*/
@DISPID(477) //= 0x1dd. The runtime will prefer the VTID if present
@VTID(323)
boolean xmlHideNamespaces();
/**
*
* Setter method for the COM property "XMLHideNamespaces"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(477) //= 0x1dd. The runtime will prefer the VTID if present
@VTID(324)
void xmlHideNamespaces(
boolean prop);
/**
*
* Getter method for the COM property "XMLShowAdvancedErrors"
*
* @return Returns a value of type boolean
*/
@DISPID(478) //= 0x1de. The runtime will prefer the VTID if present
@VTID(325)
boolean xmlShowAdvancedErrors();
/**
*
* Setter method for the COM property "XMLShowAdvancedErrors"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(478) //= 0x1de. The runtime will prefer the VTID if present
@VTID(326)
void xmlShowAdvancedErrors(
boolean prop);
/**
*
* Getter method for the COM property "XMLUseXSLTWhenSaving"
*
* @return Returns a value of type boolean
*/
@DISPID(474) //= 0x1da. The runtime will prefer the VTID if present
@VTID(327)
boolean xmlUseXSLTWhenSaving();
/**
*
* Setter method for the COM property "XMLUseXSLTWhenSaving"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(474) //= 0x1da. The runtime will prefer the VTID if present
@VTID(328)
void xmlUseXSLTWhenSaving(
boolean prop);
/**
*
* Getter method for the COM property "XMLSaveThroughXSLT"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(475) //= 0x1db. The runtime will prefer the VTID if present
@VTID(329)
java.lang.String xmlSaveThroughXSLT();
/**
*
* Setter method for the COM property "XMLSaveThroughXSLT"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(475) //= 0x1db. The runtime will prefer the VTID if present
@VTID(330)
void xmlSaveThroughXSLT(
java.lang.String prop);
/**
*
* Getter method for the COM property "DocumentLibraryVersions"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.DocumentLibraryVersions
*/
@DISPID(476) //= 0x1dc. The runtime will prefer the VTID if present
@VTID(331)
net.rgielen.com4j.office2010.office.DocumentLibraryVersions documentLibraryVersions();
@VTID(331)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.office.DocumentLibraryVersions.class})
net.rgielen.com4j.office2010.office.DocumentLibraryVersion documentLibraryVersions(
int lIndex);
/**
*
* Getter method for the COM property "ReadingModeLayoutFrozen"
*
* @return Returns a value of type boolean
*/
@DISPID(481) //= 0x1e1. The runtime will prefer the VTID if present
@VTID(332)
boolean readingModeLayoutFrozen();
/**
*
* Setter method for the COM property "ReadingModeLayoutFrozen"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(481) //= 0x1e1. The runtime will prefer the VTID if present
@VTID(333)
void readingModeLayoutFrozen(
boolean prop);
/**
*
* Getter method for the COM property "RemoveDateAndTime"
*
* @return Returns a value of type boolean
*/
@DISPID(484) //= 0x1e4. The runtime will prefer the VTID if present
@VTID(334)
boolean removeDateAndTime();
/**
*
* Setter method for the COM property "RemoveDateAndTime"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(484) //= 0x1e4. The runtime will prefer the VTID if present
@VTID(335)
void removeDateAndTime(
boolean prop);
/**
* @param recipients Optional parameter. Default value is com4j.Variant.getMissing()
* @param subject Optional parameter. Default value is com4j.Variant.getMissing()
* @param showMessage Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(464) //= 0x1d0. The runtime will prefer the VTID if present
@VTID(336)
void sendFaxOverInternet(
@Optional java.lang.Object recipients,
@Optional java.lang.Object subject,
@Optional java.lang.Object showMessage);
/**
* @param path Mandatory java.lang.String parameter.
* @param dataOnly Optional parameter. Default value is false
*/
@DISPID(500) //= 0x1f4. The runtime will prefer the VTID if present
@VTID(337)
void transformDocument(
java.lang.String path,
@Optional @DefaultValue("-1") boolean dataOnly);
/**
* @param type Mandatory net.rgielen.com4j.office2010.word.WdProtectionType parameter.
* @param noReset Optional parameter. Default value is com4j.Variant.getMissing()
* @param password Optional parameter. Default value is com4j.Variant.getMissing()
* @param useIRM Optional parameter. Default value is com4j.Variant.getMissing()
* @param enforceStyleLock Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(467) //= 0x1d3. The runtime will prefer the VTID if present
@VTID(338)
void protect(
net.rgielen.com4j.office2010.word.WdProtectionType type,
@Optional java.lang.Object noReset,
@Optional java.lang.Object password,
@Optional java.lang.Object useIRM,
@Optional java.lang.Object enforceStyleLock);
/**
* @param editorID Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(468) //= 0x1d4. The runtime will prefer the VTID if present
@VTID(339)
void selectAllEditableRanges(
@Optional java.lang.Object editorID);
/**
* @param editorID Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(469) //= 0x1d5. The runtime will prefer the VTID if present
@VTID(340)
void deleteAllEditableRanges(
@Optional java.lang.Object editorID);
/**
*/
@DISPID(479) //= 0x1df. The runtime will prefer the VTID if present
@VTID(341)
void deleteAllInkAnnotations();
/**
* @param richFormat Mandatory boolean parameter.
* @param url Mandatory java.lang.String parameter.
* @param title Mandatory java.lang.String parameter.
* @param description Mandatory java.lang.String parameter.
* @param id Mandatory java.lang.String parameter.
*/
@DISPID(482) //= 0x1e2. The runtime will prefer the VTID if present
@VTID(342)
void addDocumentWorkspaceHeader(
boolean richFormat,
java.lang.String url,
java.lang.String title,
java.lang.String description,
java.lang.String id);
/**
* @param id Mandatory java.lang.String parameter.
*/
@DISPID(483) //= 0x1e3. The runtime will prefer the VTID if present
@VTID(343)
void removeDocumentWorkspaceHeader(
java.lang.String id);
/**
* @param name Mandatory java.lang.String parameter.
* @param authorName Optional parameter. Default value is com4j.Variant.getMissing()
* @param compareTarget Optional parameter. Default value is com4j.Variant.getMissing()
* @param detectFormatChanges Optional parameter. Default value is com4j.Variant.getMissing()
* @param ignoreAllComparisonWarnings Optional parameter. Default value is com4j.Variant.getMissing()
* @param addToRecentFiles Optional parameter. Default value is com4j.Variant.getMissing()
* @param removePersonalInformation Optional parameter. Default value is com4j.Variant.getMissing()
* @param removeDateAndTime Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(485) //= 0x1e5. The runtime will prefer the VTID if present
@VTID(344)
void compare(
java.lang.String name,
@Optional java.lang.Object authorName,
@Optional java.lang.Object compareTarget,
@Optional java.lang.Object detectFormatChanges,
@Optional java.lang.Object ignoreAllComparisonWarnings,
@Optional java.lang.Object addToRecentFiles,
@Optional java.lang.Object removePersonalInformation,
@Optional java.lang.Object removeDateAndTime);
/**
*/
@DISPID(487) //= 0x1e7. The runtime will prefer the VTID if present
@VTID(345)
void removeLockedStyles();
/**
*
* Getter method for the COM property "ChildNodeSuggestions"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.XMLChildNodeSuggestions
*/
@DISPID(486) //= 0x1e6. The runtime will prefer the VTID if present
@VTID(346)
net.rgielen.com4j.office2010.word.XMLChildNodeSuggestions childNodeSuggestions();
@VTID(346)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.XMLChildNodeSuggestions.class})
net.rgielen.com4j.office2010.word.XMLChildNodeSuggestion childNodeSuggestions(
java.lang.Object index);
/**
* @param xPath Mandatory java.lang.String parameter.
* @param prefixMapping Optional parameter. Default value is ""
* @param fastSearchSkippingTextNodes Optional parameter. Default value is false
* @return Returns a value of type net.rgielen.com4j.office2010.word.XMLNode
*/
@DISPID(488) //= 0x1e8. The runtime will prefer the VTID if present
@VTID(347)
net.rgielen.com4j.office2010.word.XMLNode selectSingleNode(
java.lang.String xPath,
@Optional @DefaultValue("") java.lang.String prefixMapping,
@Optional @DefaultValue("-1") boolean fastSearchSkippingTextNodes);
/**
* @param xPath Mandatory java.lang.String parameter.
* @param prefixMapping Optional parameter. Default value is ""
* @param fastSearchSkippingTextNodes Optional parameter. Default value is false
* @return Returns a value of type net.rgielen.com4j.office2010.word.XMLNodes
*/
@DISPID(489) //= 0x1e9. The runtime will prefer the VTID if present
@VTID(348)
net.rgielen.com4j.office2010.word.XMLNodes selectNodes(
java.lang.String xPath,
@Optional @DefaultValue("") java.lang.String prefixMapping,
@Optional @DefaultValue("-1") boolean fastSearchSkippingTextNodes);
/**
*
* Getter method for the COM property "XMLSchemaViolations"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.XMLNodes
*/
@DISPID(490) //= 0x1ea. The runtime will prefer the VTID if present
@VTID(349)
net.rgielen.com4j.office2010.word.XMLNodes xmlSchemaViolations();
@VTID(349)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.XMLNodes.class})
net.rgielen.com4j.office2010.word.XMLNode xmlSchemaViolations(
int index);
/**
*
* Getter method for the COM property "ReadingLayoutSizeX"
*
* @return Returns a value of type int
*/
@DISPID(491) //= 0x1eb. The runtime will prefer the VTID if present
@VTID(350)
int readingLayoutSizeX();
/**
*
* Setter method for the COM property "ReadingLayoutSizeX"
*
* @param prop Mandatory int parameter.
*/
@DISPID(491) //= 0x1eb. The runtime will prefer the VTID if present
@VTID(351)
void readingLayoutSizeX(
int prop);
/**
*
* Getter method for the COM property "ReadingLayoutSizeY"
*
* @return Returns a value of type int
*/
@DISPID(492) //= 0x1ec. The runtime will prefer the VTID if present
@VTID(352)
int readingLayoutSizeY();
/**
*
* Setter method for the COM property "ReadingLayoutSizeY"
*
* @param prop Mandatory int parameter.
*/
@DISPID(492) //= 0x1ec. The runtime will prefer the VTID if present
@VTID(353)
void readingLayoutSizeY(
int prop);
/**
*
* Getter method for the COM property "StyleSortMethod"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdStyleSort
*/
@DISPID(493) //= 0x1ed. The runtime will prefer the VTID if present
@VTID(354)
net.rgielen.com4j.office2010.word.WdStyleSort styleSortMethod();
/**
*
* Setter method for the COM property "StyleSortMethod"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdStyleSort parameter.
*/
@DISPID(493) //= 0x1ed. The runtime will prefer the VTID if present
@VTID(355)
void styleSortMethod(
net.rgielen.com4j.office2010.word.WdStyleSort prop);
/**
*
* Getter method for the COM property "ContentTypeProperties"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.MetaProperties
*/
@DISPID(496) //= 0x1f0. The runtime will prefer the VTID if present
@VTID(356)
net.rgielen.com4j.office2010.office.MetaProperties contentTypeProperties();
@VTID(356)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.office.MetaProperties.class})
net.rgielen.com4j.office2010.office.MetaProperty contentTypeProperties(
@MarshalAs(NativeType.VARIANT) java.lang.Object index);
/**
*
* Getter method for the COM property "TrackMoves"
*
* @return Returns a value of type boolean
*/
@DISPID(499) //= 0x1f3. The runtime will prefer the VTID if present
@VTID(357)
boolean trackMoves();
/**
*
* Setter method for the COM property "TrackMoves"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(499) //= 0x1f3. The runtime will prefer the VTID if present
@VTID(358)
void trackMoves(
boolean prop);
/**
*
* Getter method for the COM property "TrackFormatting"
*
* @return Returns a value of type boolean
*/
@DISPID(502) //= 0x1f6. The runtime will prefer the VTID if present
@VTID(359)
boolean trackFormatting();
/**
*
* Setter method for the COM property "TrackFormatting"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(502) //= 0x1f6. The runtime will prefer the VTID if present
@VTID(360)
void trackFormatting(
boolean prop);
/**
*
* Getter method for the COM property "Dummy1"
*
*/
@DISPID(503) //= 0x1f7. The runtime will prefer the VTID if present
@VTID(361)
void dummy1();
/**
*
* Getter method for the COM property "OMaths"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.OMaths
*/
@DISPID(504) //= 0x1f8. The runtime will prefer the VTID if present
@VTID(362)
net.rgielen.com4j.office2010.word.OMaths oMaths();
@VTID(362)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.OMaths.class})
net.rgielen.com4j.office2010.word.OMath oMaths(
int index);
/**
* @param removeDocInfoType Mandatory net.rgielen.com4j.office2010.word.WdRemoveDocInfoType parameter.
*/
@DISPID(495) //= 0x1ef. The runtime will prefer the VTID if present
@VTID(363)
void removeDocumentInformation(
net.rgielen.com4j.office2010.word.WdRemoveDocInfoType removeDocInfoType);
/**
* @param saveChanges Optional parameter. Default value is false
* @param comments Optional parameter. Default value is com4j.Variant.getMissing()
* @param makePublic Optional parameter. Default value is false
* @param versionType Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(501) //= 0x1f5. The runtime will prefer the VTID if present
@VTID(364)
void checkInWithVersion(
@Optional @DefaultValue("-1") boolean saveChanges,
@Optional java.lang.Object comments,
@Optional @DefaultValue("0") boolean makePublic,
@Optional java.lang.Object versionType);
/**
*/
@DISPID(505) //= 0x1f9. The runtime will prefer the VTID if present
@VTID(365)
void dummy2();
/**
*
* Getter method for the COM property "Dummy3"
*
*/
@DISPID(506) //= 0x1fa. The runtime will prefer the VTID if present
@VTID(366)
void dummy3();
/**
*
* Getter method for the COM property "ServerPolicy"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.ServerPolicy
*/
@DISPID(507) //= 0x1fb. The runtime will prefer the VTID if present
@VTID(367)
net.rgielen.com4j.office2010.office.ServerPolicy serverPolicy();
@VTID(367)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.office.ServerPolicy.class})
net.rgielen.com4j.office2010.office.PolicyItem serverPolicy(
@MarshalAs(NativeType.VARIANT) java.lang.Object index);
/**
*
* Getter method for the COM property "ContentControls"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.ContentControls
*/
@DISPID(508) //= 0x1fc. The runtime will prefer the VTID if present
@VTID(368)
net.rgielen.com4j.office2010.word.ContentControls contentControls();
@VTID(368)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.word.ContentControls.class})
net.rgielen.com4j.office2010.word.ContentControl contentControls(
java.lang.Object index);
/**
*
* Getter method for the COM property "DocumentInspectors"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.DocumentInspectors
*/
@DISPID(510) //= 0x1fe. The runtime will prefer the VTID if present
@VTID(369)
net.rgielen.com4j.office2010.office.DocumentInspectors documentInspectors();
@VTID(369)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.office.DocumentInspectors.class})
net.rgielen.com4j.office2010.office.DocumentInspector documentInspectors(
int index);
/**
*/
@DISPID(509) //= 0x1fd. The runtime will prefer the VTID if present
@VTID(370)
void lockServerFile();
/**
* @return Returns a value of type net.rgielen.com4j.office2010.office.WorkflowTasks
*/
@DISPID(511) //= 0x1ff. The runtime will prefer the VTID if present
@VTID(371)
net.rgielen.com4j.office2010.office.WorkflowTasks getWorkflowTasks();
@VTID(371)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.office.WorkflowTasks.class})
net.rgielen.com4j.office2010.office.WorkflowTask getWorkflowTasks(
int index);
/**
* @return Returns a value of type net.rgielen.com4j.office2010.office.WorkflowTemplates
*/
@DISPID(512) //= 0x200. The runtime will prefer the VTID if present
@VTID(372)
net.rgielen.com4j.office2010.office.WorkflowTemplates getWorkflowTemplates();
@VTID(372)
@ReturnValue(defaultPropertyThrough={net.rgielen.com4j.office2010.office.WorkflowTemplates.class})
net.rgielen.com4j.office2010.office.WorkflowTemplate getWorkflowTemplates(
int index);
/**
*/
@DISPID(514) //= 0x202. The runtime will prefer the VTID if present
@VTID(373)
void dummy4();
/**
* @param skipIfAbsent Mandatory boolean parameter.
* @param url Mandatory java.lang.String parameter.
* @param title Mandatory java.lang.String parameter.
* @param description Mandatory java.lang.String parameter.
* @param id Mandatory java.lang.String parameter.
*/
@DISPID(515) //= 0x203. The runtime will prefer the VTID if present
@VTID(374)
void addMeetingWorkspaceHeader(
boolean skipIfAbsent,
java.lang.String url,
java.lang.String title,
java.lang.String description,
java.lang.String id);
/**
*
* Getter method for the COM property "Bibliography"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Bibliography
*/
@DISPID(516) //= 0x204. The runtime will prefer the VTID if present
@VTID(375)
net.rgielen.com4j.office2010.word.Bibliography bibliography();
/**
*
* Getter method for the COM property "LockTheme"
*
* @return Returns a value of type boolean
*/
@DISPID(517) //= 0x205. The runtime will prefer the VTID if present
@VTID(376)
boolean lockTheme();
/**
*
* Setter method for the COM property "LockTheme"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(517) //= 0x205. The runtime will prefer the VTID if present
@VTID(377)
void lockTheme(
boolean prop);
/**
*
* Getter method for the COM property "LockQuickStyleSet"
*
* @return Returns a value of type boolean
*/
@DISPID(518) //= 0x206. The runtime will prefer the VTID if present
@VTID(378)
boolean lockQuickStyleSet();
/**
*
* Setter method for the COM property "LockQuickStyleSet"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(518) //= 0x206. The runtime will prefer the VTID if present
@VTID(379)
void lockQuickStyleSet(
boolean prop);
/**
*
* Getter method for the COM property "OriginalDocumentTitle"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(519) //= 0x207. The runtime will prefer the VTID if present
@VTID(380)
java.lang.String originalDocumentTitle();
/**
*
* Getter method for the COM property "RevisedDocumentTitle"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(520) //= 0x208. The runtime will prefer the VTID if present
@VTID(381)
java.lang.String revisedDocumentTitle();
/**
*
* Getter method for the COM property "CustomXMLParts"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office._CustomXMLParts
*/
@DISPID(521) //= 0x209. The runtime will prefer the VTID if present
@VTID(382)
net.rgielen.com4j.office2010.office._CustomXMLParts customXMLParts();
/**
*
* Getter method for the COM property "FormattingShowNextLevel"
*
* @return Returns a value of type boolean
*/
@DISPID(522) //= 0x20a. The runtime will prefer the VTID if present
@VTID(383)
boolean formattingShowNextLevel();
/**
*
* Setter method for the COM property "FormattingShowNextLevel"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(522) //= 0x20a. The runtime will prefer the VTID if present
@VTID(384)
void formattingShowNextLevel(
boolean prop);
/**
*
* Getter method for the COM property "FormattingShowUserStyleName"
*
* @return Returns a value of type boolean
*/
@DISPID(523) //= 0x20b. The runtime will prefer the VTID if present
@VTID(385)
boolean formattingShowUserStyleName();
/**
*
* Setter method for the COM property "FormattingShowUserStyleName"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(523) //= 0x20b. The runtime will prefer the VTID if present
@VTID(386)
void formattingShowUserStyleName(
boolean prop);
/**
* @param fileName Mandatory java.lang.String parameter.
*/
@DISPID(524) //= 0x20c. The runtime will prefer the VTID if present
@VTID(387)
void saveAsQuickStyleSet(
java.lang.String fileName);
/**
* @param name Mandatory java.lang.String parameter.
*/
@DISPID(525) //= 0x20d. The runtime will prefer the VTID if present
@VTID(388)
void applyQuickStyleSet(
java.lang.String name);
/**
*
* Getter method for the COM property "Research"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.Research
*/
@DISPID(526) //= 0x20e. The runtime will prefer the VTID if present
@VTID(389)
net.rgielen.com4j.office2010.word.Research research();
/**
*
* Getter method for the COM property "Final"
*
* @return Returns a value of type boolean
*/
@DISPID(527) //= 0x20f. The runtime will prefer the VTID if present
@VTID(390)
boolean _final();
/**
*
* Setter method for the COM property "Final"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(527) //= 0x20f. The runtime will prefer the VTID if present
@VTID(391)
void _final(
boolean prop);
/**
*
* Getter method for the COM property "OMathBreakBin"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdOMathBreakBin
*/
@DISPID(528) //= 0x210. The runtime will prefer the VTID if present
@VTID(392)
net.rgielen.com4j.office2010.word.WdOMathBreakBin oMathBreakBin();
/**
*
* Setter method for the COM property "OMathBreakBin"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdOMathBreakBin parameter.
*/
@DISPID(528) //= 0x210. The runtime will prefer the VTID if present
@VTID(393)
void oMathBreakBin(
net.rgielen.com4j.office2010.word.WdOMathBreakBin prop);
/**
*
* Getter method for the COM property "OMathBreakSub"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdOMathBreakSub
*/
@DISPID(529) //= 0x211. The runtime will prefer the VTID if present
@VTID(394)
net.rgielen.com4j.office2010.word.WdOMathBreakSub oMathBreakSub();
/**
*
* Setter method for the COM property "OMathBreakSub"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdOMathBreakSub parameter.
*/
@DISPID(529) //= 0x211. The runtime will prefer the VTID if present
@VTID(395)
void oMathBreakSub(
net.rgielen.com4j.office2010.word.WdOMathBreakSub prop);
/**
*
* Getter method for the COM property "OMathJc"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.WdOMathJc
*/
@DISPID(530) //= 0x212. The runtime will prefer the VTID if present
@VTID(396)
net.rgielen.com4j.office2010.word.WdOMathJc oMathJc();
/**
*
* Setter method for the COM property "OMathJc"
*
* @param prop Mandatory net.rgielen.com4j.office2010.word.WdOMathJc parameter.
*/
@DISPID(530) //= 0x212. The runtime will prefer the VTID if present
@VTID(397)
void oMathJc(
net.rgielen.com4j.office2010.word.WdOMathJc prop);
/**
*
* Getter method for the COM property "OMathLeftMargin"
*
* @return Returns a value of type float
*/
@DISPID(531) //= 0x213. The runtime will prefer the VTID if present
@VTID(398)
float oMathLeftMargin();
/**
*
* Setter method for the COM property "OMathLeftMargin"
*
* @param prop Mandatory float parameter.
*/
@DISPID(531) //= 0x213. The runtime will prefer the VTID if present
@VTID(399)
void oMathLeftMargin(
float prop);
/**
*
* Getter method for the COM property "OMathRightMargin"
*
* @return Returns a value of type float
*/
@DISPID(532) //= 0x214. The runtime will prefer the VTID if present
@VTID(400)
float oMathRightMargin();
/**
*
* Setter method for the COM property "OMathRightMargin"
*
* @param prop Mandatory float parameter.
*/
@DISPID(532) //= 0x214. The runtime will prefer the VTID if present
@VTID(401)
void oMathRightMargin(
float prop);
/**
*
* Getter method for the COM property "OMathWrap"
*
* @return Returns a value of type float
*/
@DISPID(535) //= 0x217. The runtime will prefer the VTID if present
@VTID(402)
float oMathWrap();
/**
*
* Setter method for the COM property "OMathWrap"
*
* @param prop Mandatory float parameter.
*/
@DISPID(535) //= 0x217. The runtime will prefer the VTID if present
@VTID(403)
void oMathWrap(
float prop);
/**
*
* Getter method for the COM property "OMathIntSubSupLim"
*
* @return Returns a value of type boolean
*/
@DISPID(536) //= 0x218. The runtime will prefer the VTID if present
@VTID(404)
boolean oMathIntSubSupLim();
/**
*
* Setter method for the COM property "OMathIntSubSupLim"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(536) //= 0x218. The runtime will prefer the VTID if present
@VTID(405)
void oMathIntSubSupLim(
boolean prop);
/**
*
* Getter method for the COM property "OMathNarySupSubLim"
*
* @return Returns a value of type boolean
*/
@DISPID(537) //= 0x219. The runtime will prefer the VTID if present
@VTID(406)
boolean oMathNarySupSubLim();
/**
*
* Setter method for the COM property "OMathNarySupSubLim"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(537) //= 0x219. The runtime will prefer the VTID if present
@VTID(407)
void oMathNarySupSubLim(
boolean prop);
/**
*
* Getter method for the COM property "OMathSmallFrac"
*
* @return Returns a value of type boolean
*/
@DISPID(539) //= 0x21b. The runtime will prefer the VTID if present
@VTID(408)
boolean oMathSmallFrac();
/**
*
* Setter method for the COM property "OMathSmallFrac"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(539) //= 0x21b. The runtime will prefer the VTID if present
@VTID(409)
void oMathSmallFrac(
boolean prop);
/**
*
* Getter method for the COM property "WordOpenXML"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(542) //= 0x21e. The runtime will prefer the VTID if present
@VTID(410)
java.lang.String wordOpenXML();
/**
*
* Getter method for the COM property "DocumentTheme"
*
* @return Returns a value of type net.rgielen.com4j.office2010.office.OfficeTheme
*/
@DISPID(545) //= 0x221. The runtime will prefer the VTID if present
@VTID(411)
net.rgielen.com4j.office2010.office.OfficeTheme documentTheme();
/**
* @param fileName Mandatory java.lang.String parameter.
*/
@DISPID(546) //= 0x222. The runtime will prefer the VTID if present
@VTID(412)
void applyDocumentTheme(
java.lang.String fileName);
/**
*
* Getter method for the COM property "HasVBProject"
*
* @return Returns a value of type boolean
*/
@DISPID(548) //= 0x224. The runtime will prefer the VTID if present
@VTID(413)
boolean hasVBProject();
/**
* @param node Mandatory net.rgielen.com4j.office2010.office.CustomXMLNode parameter.
* @return Returns a value of type net.rgielen.com4j.office2010.word.ContentControls
*/
@DISPID(549) //= 0x225. The runtime will prefer the VTID if present
@VTID(414)
net.rgielen.com4j.office2010.word.ContentControls selectLinkedControls(
net.rgielen.com4j.office2010.office.CustomXMLNode node);
/**
* @param stream Optional parameter. Default value is 0
* @return Returns a value of type net.rgielen.com4j.office2010.word.ContentControls
*/
@DISPID(550) //= 0x226. The runtime will prefer the VTID if present
@VTID(415)
net.rgielen.com4j.office2010.word.ContentControls selectUnlinkedControls(
@Optional @DefaultValue("0") net.rgielen.com4j.office2010.office._CustomXMLPart stream);
/**
* @param title Mandatory java.lang.String parameter.
* @return Returns a value of type net.rgielen.com4j.office2010.word.ContentControls
*/
@DISPID(551) //= 0x227. The runtime will prefer the VTID if present
@VTID(416)
net.rgielen.com4j.office2010.word.ContentControls selectContentControlsByTitle(
java.lang.String title);
/**
* @param outputFileName Mandatory java.lang.String parameter.
* @param exportFormat Mandatory net.rgielen.com4j.office2010.word.WdExportFormat parameter.
* @param openAfterExport Optional parameter. Default value is false
* @param optimizeFor Optional parameter. Default value is 0
* @param range Optional parameter. Default value is 0
* @param from Optional parameter. Default value is 1
* @param to Optional parameter. Default value is 1
* @param item Optional parameter. Default value is 0
* @param includeDocProps Optional parameter. Default value is false
* @param keepIRM Optional parameter. Default value is false
* @param createBookmarks Optional parameter. Default value is 0
* @param docStructureTags Optional parameter. Default value is false
* @param bitmapMissingFonts Optional parameter. Default value is false
* @param useISO19005_1 Optional parameter. Default value is false
* @param fixedFormatExtClassPtr Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(552) //= 0x228. The runtime will prefer the VTID if present
@VTID(417)
void exportAsFixedFormat(
java.lang.String outputFileName,
net.rgielen.com4j.office2010.word.WdExportFormat exportFormat,
@Optional @DefaultValue("0") boolean openAfterExport,
@Optional @DefaultValue("0") net.rgielen.com4j.office2010.word.WdExportOptimizeFor optimizeFor,
@Optional @DefaultValue("0") net.rgielen.com4j.office2010.word.WdExportRange range,
@Optional @DefaultValue("1") int from,
@Optional @DefaultValue("1") int to,
@Optional @DefaultValue("0") net.rgielen.com4j.office2010.word.WdExportItem item,
@Optional @DefaultValue("0") boolean includeDocProps,
@Optional @DefaultValue("-1") boolean keepIRM,
@Optional @DefaultValue("0") net.rgielen.com4j.office2010.word.WdExportCreateBookmarks createBookmarks,
@Optional @DefaultValue("-1") boolean docStructureTags,
@Optional @DefaultValue("-1") boolean bitmapMissingFonts,
@Optional @DefaultValue("0") boolean useISO19005_1,
@Optional java.lang.Object fixedFormatExtClassPtr);
/**
*/
@DISPID(553) //= 0x229. The runtime will prefer the VTID if present
@VTID(418)
void freezeLayout();
/**
*/
@DISPID(554) //= 0x22a. The runtime will prefer the VTID if present
@VTID(419)
void unfreezeLayout();
/**
*
* Getter method for the COM property "OMathFontName"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(555) //= 0x22b. The runtime will prefer the VTID if present
@VTID(420)
java.lang.String oMathFontName();
/**
*
* Setter method for the COM property "OMathFontName"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(555) //= 0x22b. The runtime will prefer the VTID if present
@VTID(421)
void oMathFontName(
java.lang.String prop);
/**
*/
@DISPID(558) //= 0x22e. The runtime will prefer the VTID if present
@VTID(422)
void downgradeDocument();
/**
*
* Getter method for the COM property "EncryptionProvider"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(559) //= 0x22f. The runtime will prefer the VTID if present
@VTID(423)
java.lang.String encryptionProvider();
/**
*
* Setter method for the COM property "EncryptionProvider"
*
* @param prop Mandatory java.lang.String parameter.
*/
@DISPID(559) //= 0x22f. The runtime will prefer the VTID if present
@VTID(424)
void encryptionProvider(
java.lang.String prop);
/**
*
* Getter method for the COM property "UseMathDefaults"
*
* @return Returns a value of type boolean
*/
@DISPID(560) //= 0x230. The runtime will prefer the VTID if present
@VTID(425)
boolean useMathDefaults();
/**
*
* Setter method for the COM property "UseMathDefaults"
*
* @param prop Mandatory boolean parameter.
*/
@DISPID(560) //= 0x230. The runtime will prefer the VTID if present
@VTID(426)
void useMathDefaults(
boolean prop);
/**
*
* Getter method for the COM property "CurrentRsid"
*
* @return Returns a value of type int
*/
@DISPID(563) //= 0x233. The runtime will prefer the VTID if present
@VTID(427)
int currentRsid();
/**
*/
@DISPID(561) //= 0x231. The runtime will prefer the VTID if present
@VTID(428)
void convert();
/**
* @param tag Mandatory java.lang.String parameter.
* @return Returns a value of type net.rgielen.com4j.office2010.word.ContentControls
*/
@DISPID(562) //= 0x232. The runtime will prefer the VTID if present
@VTID(429)
net.rgielen.com4j.office2010.word.ContentControls selectContentControlsByTag(
java.lang.String tag);
/**
*/
@DISPID(650) //= 0x28a. The runtime will prefer the VTID if present
@VTID(430)
void convertAutoHyphens();
/**
*
* Getter method for the COM property "DocID"
*
* @return Returns a value of type int
*/
@DISPID(564) //= 0x234. The runtime will prefer the VTID if present
@VTID(431)
int docID();
/**
* @param style Mandatory java.lang.Object parameter.
*/
@DISPID(566) //= 0x236. The runtime will prefer the VTID if present
@VTID(432)
void applyQuickStyleSet2(
java.lang.Object style);
/**
*
* Getter method for the COM property "CompatibilityMode"
*
* @return Returns a value of type int
*/
@DISPID(567) //= 0x237. The runtime will prefer the VTID if present
@VTID(433)
int compatibilityMode();
/**
* @param fileName Optional parameter. Default value is com4j.Variant.getMissing()
* @param fileFormat Optional parameter. Default value is com4j.Variant.getMissing()
* @param lockComments Optional parameter. Default value is com4j.Variant.getMissing()
* @param password Optional parameter. Default value is com4j.Variant.getMissing()
* @param addToRecentFiles Optional parameter. Default value is com4j.Variant.getMissing()
* @param writePassword Optional parameter. Default value is com4j.Variant.getMissing()
* @param readOnlyRecommended Optional parameter. Default value is com4j.Variant.getMissing()
* @param embedTrueTypeFonts Optional parameter. Default value is com4j.Variant.getMissing()
* @param saveNativePictureFormat Optional parameter. Default value is com4j.Variant.getMissing()
* @param saveFormsData Optional parameter. Default value is com4j.Variant.getMissing()
* @param saveAsAOCELetter Optional parameter. Default value is com4j.Variant.getMissing()
* @param encoding Optional parameter. Default value is com4j.Variant.getMissing()
* @param insertLineBreaks Optional parameter. Default value is com4j.Variant.getMissing()
* @param allowSubstitutions Optional parameter. Default value is com4j.Variant.getMissing()
* @param lineEnding Optional parameter. Default value is com4j.Variant.getMissing()
* @param addBiDiMarks Optional parameter. Default value is com4j.Variant.getMissing()
* @param compatibilityMode Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(568) //= 0x238. The runtime will prefer the VTID if present
@VTID(434)
void saveAs2(
@Optional java.lang.Object fileName,
@Optional java.lang.Object fileFormat,
@Optional java.lang.Object lockComments,
@Optional java.lang.Object password,
@Optional java.lang.Object addToRecentFiles,
@Optional java.lang.Object writePassword,
@Optional java.lang.Object readOnlyRecommended,
@Optional java.lang.Object embedTrueTypeFonts,
@Optional java.lang.Object saveNativePictureFormat,
@Optional java.lang.Object saveFormsData,
@Optional java.lang.Object saveAsAOCELetter,
@Optional java.lang.Object encoding,
@Optional java.lang.Object insertLineBreaks,
@Optional java.lang.Object allowSubstitutions,
@Optional java.lang.Object lineEnding,
@Optional java.lang.Object addBiDiMarks,
@Optional java.lang.Object compatibilityMode);
/**
*
* Getter method for the COM property "CoAuthoring"
*
* @return Returns a value of type net.rgielen.com4j.office2010.word.CoAuthoring
*/
@DISPID(600) //= 0x258. The runtime will prefer the VTID if present
@VTID(435)
net.rgielen.com4j.office2010.word.CoAuthoring coAuthoring();
/**
* @param mode Mandatory int parameter.
*/
@DISPID(571) //= 0x23b. The runtime will prefer the VTID if present
@VTID(436)
void setCompatibilityMode(
int mode);
// Properties:
}