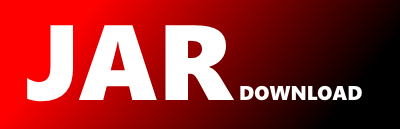
org.ar4k.agent.hazelcast.InternalMessageHandler Maven / Gradle / Ivy
package org.ar4k.agent.hazelcast;
import org.ar4k.agent.core.data.AbstractChannel;
import org.springframework.messaging.Message;
import org.springframework.messaging.MessageHandler;
import org.springframework.messaging.MessagingException;
import com.hazelcast.core.ITopic;
public class InternalMessageHandler implements MessageHandler {
private ITopic
© 2015 - 2025 Weber Informatics LLC | Privacy Policy