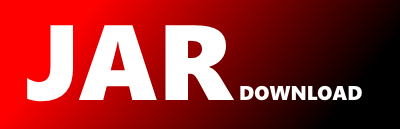
net.scattersphere.api.Client Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scattersphere-api Show documentation
Show all versions of scattersphere-api Show documentation
Scattersphere API, used for clients to connect to servers and control them through code.
package net.scattersphere.api;
import net.scattersphere.data.DataSerializer;
import net.scattersphere.data.message.JobMessage;
import net.scattersphere.data.message.JobParametersMessage;
import org.slf4j.LoggerFactory;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
/**
* This portion of the API handles commands sent to a client endpoint. This API is designed to make it easier to
* programmatically control jobs on servers, and control servers.
*
* Created by kenji on 1/2/15.
*/
public class Client {
private Consumer onConnect;
private Consumer onDisconnect;
private BiConsumer onMessage;
private final org.slf4j.Logger LOG = LoggerFactory.getLogger(Client.class);
/**
* This is a storage class used to store connections to servers.
*/
private class ClientConnectionPair {
private String host;
private String pairName;
private ClientConnection client;
public ClientConnectionPair(String pairName, String host) {
this.pairName = pairName;
this.host = host;
this.client = new ClientConnection();
}
public String getHost() {
return host;
}
public void connect() {
client.onConnect(x -> {
if (x == ClientConnectionState.CONNECTED) {
onConnect.accept(pairName);
LOG.info("New connection state: {}", x);
}
if (x == ClientConnectionState.CLOSED) {
onDisconnect.accept(pairName);
LOG.info("Connection closed: {}", x);
}
if (x == ClientConnectionState.FAILED) {
onDisconnect.accept(pairName);
LOG.info("Connection failed: {}", host);
}
})
.messageReceived(msg -> onMessage.accept(pairName, msg))
.connect(host);
}
public ClientConnection getClientConnection() {
return client;
}
}
private Map connections;
/**
* Constructor.
*/
public Client() {
this.connections = new HashMap<>();
this.onConnect = null;
}
/**
* Adds a connection to a server, assigning a name to that connection.
*
* @param clientName The name of the connection to assign.
* @param clientAddress The address to connect to.
*/
public void addClient(String clientName, String clientAddress) {
Objects.requireNonNull(clientName);
Objects.requireNonNull(clientAddress);
ClientConnectionPair clientConnectionPair = new ClientConnectionPair(clientName, clientAddress);
clientConnectionPair.connect();
connections.put(clientName, clientConnectionPair);
}
/**
* Retrieves the underlying {@link ClientConnection}, which is the communication layer to the endpoint. This is available
* for use if required.
*
* @param clientName The name of the connection to retrieve.
* @return {@link ClientConnection} if found.
*/
public ClientConnection getClientConnection(String clientName) {
Objects.requireNonNull(clientName);
if (connections.get(clientName) == null) {
throw new NullPointerException("Connection for client by name does not exist: " + clientName);
}
return connections.get(clientName).getClientConnection();
}
/**
* Requests a list of jobs from the specified server.
*
* @param clientName The server to request jobs from.
*/
public void listJobs(String clientName) {
getClientConnection(clientName).sendMessage(DataSerializer.packetize((new JobMessage("LIST")).toByteArray()));
}
public void listJobs(String clientName, String jobType) {
JobParametersMessage parameters = new JobParametersMessage(jobType, new String[0]);
JobMessage message = new JobMessage("LIST", parameters.toByteArray());
getClientConnection(clientName).sendMessage(DataSerializer.packetize(message.toByteArray()));
}
/**
* Starts a job by name, with specified arguments.
*
* @param clientName The server to start a job on.
* @param jobName The name of the job to start.
* @param arguments The arguments to send to the job.
*/
public void startJob(String clientName, String jobName, String[] arguments) {
JobParametersMessage parameters = new JobParametersMessage(jobName, arguments);
JobMessage message = new JobMessage("START", parameters.toByteArray());
getClientConnection(clientName).sendMessage(DataSerializer.packetize(message.toByteArray()));
}
/**
* Stops a job by its ID.
*
* @param clientName The server to stop a job on.
* @param jobId The ID of the job to stop.
*/
public void stopJob(String clientName, String jobId, String reason) {
JobParametersMessage parameters = new JobParametersMessage(jobId, reason, null);
JobMessage message = new JobMessage("STOP", parameters.toByteArray());
getClientConnection(clientName).sendMessage(DataSerializer.packetize(message.toByteArray()));
}
/**
* Retrieves status for a job by ID.
*
* @param clientName The server to get a job status from.
* @param jobId The ID of the job to retrieve status for.
*/
public void statusJob(String clientName, String jobId) {
getClientConnection(clientName).sendMessage(DataSerializer.packetize((new JobMessage("STATUS", jobId)).toByteArray()));
}
/**
* Lambda function called when a connection is successful.
*
* @param function The {@link Consumer} function to set.
*/
public void onConnect(Consumer function) {
this.onConnect = function;
}
/**
* Lambda function called when a connection is lost, or dropped.
*
* @param function The {@link Consumer} function to set.
*/
public void onDisconnect(Consumer function) {
this.onDisconnect = function;
}
/**
* Lambda function called when a message is received from a server.
*
* @param function The {@link BiConsumer} function to set.
*/
public void onMessage(BiConsumer function) {
this.onMessage = function;
}
/**
* Sends a message to the specified server.
*
* @param clientName The server to send a message to.
* @param message The message to send.
*/
public void send(String clientName, JobMessage message) {
connections.get(clientName).getClientConnection().sendMessage(message.toByteArray());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy