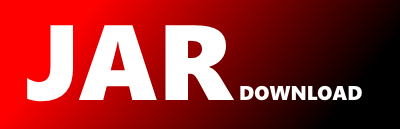
net.scattersphere.client.Main Maven / Gradle / Ivy
/*
* Scattersphere
* Copyright 2014-2015, Scattersphere Project.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.scattersphere.client;
import jline.console.ConsoleReader;
import java.util.concurrent.atomic.AtomicInteger;
/**
* This is the Scattersphere main command client.
*
* Created by kenji on 11/16/14.
*/
public class Main {
private static String username;
private static String password;
private static String connectHostname;
/**
* Main entrypoint to the CLI.
*
* @param args {@link String[]} of command line arguments, currently ignored.
* @throws Exception on any errors.
*/
public static void main(String args[]) throws Exception {
System.out.println(" _____ __ __ __ ");
System.out.println(" / ___/_________ _/ /_/ /____ ______________ / /_ ___ ________");
System.out.println(" \\__ \\/ ___/ __ `/ __/ __/ _ \\/ ___/ ___/ __ \\/ __ \\/ _ \\/ ___/ _ \\");
System.out.println(" ___/ / /__/ /_/ / /_/ /_/ __/ / (__ ) /_/ / / / / __/ / / __/");
System.out.println(" /____/\\___/\\__,_/\\__/\\__/\\___/_/ /____/ .___/_/ /_/\\___/_/ \\___/");
System.out.println(" /_/");
System.out.println();
System.out.println("Scattersphere Client: Command line interface (CLI)");
System.out.println("Type \"help\" for help. Use arrows to navigate command history.\n");
AtomicInteger commandCounter = new AtomicInteger(0);
ConsoleReader reader = new ConsoleReader();
connectHostname = null;
// Adds the ability to connect to a site by providing that address through the command line.
if (args.length > 0) {
processArgs(args);
}
String line;
ClientCommandHandler handler = new ClientCommandHandler(reader, username, password);
if (connectHostname != null) {
handler.handle("connect " + connectHostname);
}
reader.setPrompt(commandCounter.incrementAndGet() + "> ");
while ((line = reader.readLine()) != null) {
handler.handle(line);
reader.setPrompt(commandCounter.incrementAndGet() + "> ");
}
}
private static void processArgs(String args[]) {
for(int i = 0; i < args.length; i++) {
if ("-h".equals(args[i])) {
giveHelp();
System.exit(0);
} else if ("-c".equals(args[i])) {
i++;
if (i > args.length) {
System.out.println("-c requires a hostname.");
System.exit(-1);
}
connectHostname = args[i];
} else if ("-u".equals(args[i])) {
i++;
if (i > args.length) {
System.out.println("-u requires a username.");
System.exit(-1);
}
username = args[i];
} else if ("-p".equals(args[i])) {
i++;
if (i > args.length) {
System.out.println("-p requires a password.");
System.exit(-1);
}
password = args[i];
}
}
}
private static void giveHelp() {
System.out.println("Usage:");
System.out.println("------");
System.out.println(" -h Gives help.");
System.out.println(" -c [host] Connects to the specified host.");
System.out.println(" -u [user] Specifies a username for authentication.");
System.out.println(" -p [pass] Specifies a password for authentication.");
System.out.println();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy