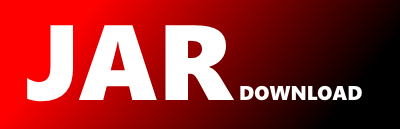
net.scattersphere.client.StreamClientCache Maven / Gradle / Ivy
package net.scattersphere.client;
import net.scattersphere.api.StreamClient;
import java.io.PrintWriter;
import java.util.HashMap;
import java.util.Map;
/**
* This is a class that caches {@link StreamClient} connections.
*
* Created by kenji on 2/14/15.
*/
public class StreamClientCache {
private static final StreamClientCache instance = new StreamClientCache();
private final Map clients = new HashMap<>();
/**
* Returns the singleton instance of the {@link StreamClientCache} object.
*
* @return {@link net.scattersphere.client.StreamClientCache} object.
*/
public static StreamClientCache instance() {
return instance;
}
/**
* Opens a stream, connecting to localhost, pulling in stream data for the specified ID.
*
* @param streamId The stream ID to retrieve.
* @param out The active {@link PrintWriter} object.
*/
public void openStream(String streamId, PrintWriter out) {
if (clients.get(streamId) != null) {
out.println("Stream already open for stream ID " + streamId);
return;
}
StreamClient streamClient = new StreamClient();
streamClient.onConnect(x -> {
streamClient.openStream(streamId, streamId);
out.println("[" + streamId + "] Connected to " + x);
out.flush();
}).onDisconnect(x -> {
out.println("[" + streamId + "] Disconnected from " + x);
out.flush();
clients.remove(streamId);
}).onStream((name, data) -> {
out.println("[" + name + "] data=" + new String(data));
out.flush();
});
streamClient.addClient(streamId, "localhost");
clients.put(streamId, streamClient);
}
/**
* Closes a stream connection.
*
* @param streamId The stream ID to disconnect from.
* @param out The currently active {@link PrintWriter} object.
*/
public void closeStream(String streamId, PrintWriter out) {
if (clients.get(streamId) == null) {
out.println("Stream not open by ID " + streamId);
out.flush();
return;
}
StreamClient streamClient = clients.get(streamId);
streamClient.closeStream(streamId, streamId);
out.println("Stream closed for stream ID " + streamId);
out.flush();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy