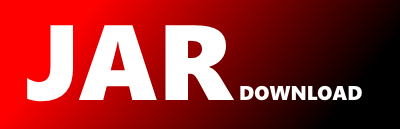
net.scattersphere.job.AbstractJob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scattersphere-core Show documentation
Show all versions of scattersphere-core Show documentation
Scattersphere Core library, used to run the main server.
The newest version!
package net.scattersphere.job;
import net.scattersphere.registry.StreamRegistry;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* This is the abstract implementation of the {@link Job} interface. {@link Job}s should extend this class, and
* override the methods provided, if necessary. At the very least, they must implement the {@code run()} method.
*
* Created by kenji on 1/1/15.
*/
public abstract class AbstractJob implements Job {
private String[] arguments;
private String jobId;
private final Logger LOG = LoggerFactory.getLogger(AbstractJob.class);
/**
* Constructor.
*/
public AbstractJob() {
this.arguments = null;
}
/**
* Prepares the abstract job, settings its parameters.
*
* @param arguments {@link String[]} containing a list of arguments.
*/
public void prepare(String[] arguments) {
this.arguments = arguments;
LOG.info("Prepare: Set arguments={}", arguments);
// Open the stream as soon as the prepare is called.
StreamRegistry.instance().openStream(jobId);
}
/**
* Retrieves the arguments that were sent.
*
* @return {@code String[]} list of arguments.
*/
public String[] getArguments() {
return arguments;
}
/**
* Sets the job ID.
*
* @param jobId {@code String} containing the job ID.
*/
public void setJobId(String jobId) {
this.jobId = jobId;
}
/**
* Retrieves the current job ID.
*
* @return {@code String} containing the job ID.
*/
public String getJobId() {
return jobId;
}
/**
* Writes data to the job's stream.
*
* @param data {@code byte[]} array of data to write as a packet.
*/
public void writeToStream(byte[] data) {
StreamRegistry.instance().write(jobId, data);
}
/**
* Closes the buffer stream.
*/
public void closeStream() {
StreamRegistry.instance().closeStream(jobId);
}
/**
* Retrieves a description for this job.
*
* @return {@code String} containing the job description.
*/
public String getDescription() {
return "No description provided.";
}
public abstract void run();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy