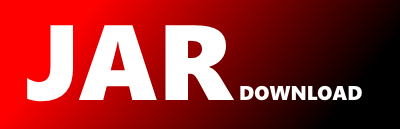
net.scattersphere.job.JobContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scattersphere-core Show documentation
Show all versions of scattersphere-core Show documentation
Scattersphere Core library, used to run the main server.
The newest version!
/*
* Scattersphere
* Copyright 2014-2015, Scattersphere Project.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.scattersphere.job;
import net.scattersphere.util.thread.JobExecutionResult;
import java.io.Serializable;
/**
* {@code JobContext} is a context class that is used to job, and some simple metrics.
*
* @author kenji
*/
public class JobContext implements Serializable {
private static final long serialVersionUID = 2837394854983379966L;
protected String jobName;
protected String jobId;
protected String jobStopReason;
protected JobExecutionResult jobResult;
protected Exception jobException;
protected long jobStart;
protected long jobEnd;
/**
* Sets the context for the job. Job start time is set as {@link System#nanoTime()}.
*
* @param jobName The job name specified.
* @param jobId The associated job ID.
* @param jobResult The result of the job run.
*/
public JobContext( String jobName, String jobId, JobExecutionResult jobResult ) {
this.jobName = jobName;
this.jobId = jobId;
this.jobStopReason = null;
this.jobResult = jobResult;
this.jobException = null;
this.jobStart = System.nanoTime();
this.jobEnd = 0L;
}
/**
* Retrieves the job name.
*
* @return {@code String} containing the job name.
*/
public String getJobName() {
return jobName;
}
/**
* Retrieves the job ID.
*
* @return {@code String} containing the job ID.
*/
public String getJobId() {
return jobId;
}
/**
* Returns the current job result.
*
* @return {@link net.scattersphere.util.thread.JobExecutionResult} containing the current result status.
*/
public JobExecutionResult getJobResult() {
return jobResult;
}
/**
* Sets the job result.
*
* @param jobResult {@link net.scattersphere.util.thread.JobExecutionResult} containing the new result status to set.
*/
public void setJobResult(JobExecutionResult jobResult) {
this.jobResult = jobResult;
}
/**
* Sets the job result with a {@link Exception} exception cause.
*
* @param jobResult {@link net.scattersphere.util.thread.JobExecutionResult} containing the new result status to set.
* @param cause The {@link Exception} cause.
*/
public void setJobResult(JobExecutionResult jobResult, Exception cause) {
this.jobResult = jobResult;
this.jobException = cause;
}
/**
* Returns the {@link Throwable} exception state if one exists.
*
* @return The {@link Throwable} exception that occurred, {@code null} if none was specified.
*/
public Exception getJobException() {
return jobException;
}
/**
* Sets the job stop reason.
*
* @param jobStopReason {@code String} containing the reason to stop the job.
*/
public void setJobStopReason(String jobStopReason) {
this.jobStopReason = jobStopReason;
}
/**
* Returns the reason the job was stopped.
*
* @return {@code String} containing the reason, {@code null} if none was set.
*/
public String getJobStopReason() {
return jobStopReason;
}
/**
* Sets the current start time to system time in milliseconds.
*/
public void setJobStart() {
setJobStart(System.currentTimeMillis());
}
/**
* Sets the start time of the job.
*
* @param jobStart {@code long} containing the job start time in nano or milliseconds.
*/
public void setJobStart(long jobStart) {
this.jobStart = jobStart;
}
/**
* Retrieves the job start time.
*
* @return {@code long} containing the job start time in nano or milliseconds.
*/
public long getJobStart() {
return jobStart;
}
/**
* Sets the current end time to the current time in milliseconds.
*/
public void setJobEnd() {
setJobEnd(System.currentTimeMillis());
}
/**
* Sets the time in which the job ended.
*
* @param jobEnd {@code long} containing the job end time in nano or milliseconds.
*/
public void setJobEnd(long jobEnd) {
this.jobEnd = jobEnd;
}
/**
* Retrieves the job end time.
*
* @return {@code long} containing the time in which the job ended in nano or milliseconds.
*/
public long getJobEnd() {
return jobEnd;
}
@Override
public String toString() {
return "JobContext{" +
"jobName='" + jobName + '\'' +
", jobId='" + jobId + '\'' +
", jobStopReason='" + jobStopReason + '\'' +
", jobResult=" + jobResult +
", jobException=" + jobException +
", jobStart=" + jobStart +
", jobEnd=" + jobEnd +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy