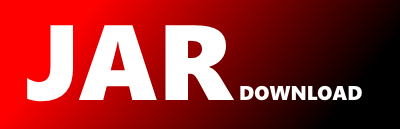
net.scattersphere.registry.JobRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scattersphere-core Show documentation
Show all versions of scattersphere-core Show documentation
Scattersphere Core library, used to run the main server.
The newest version!
/*
* Scattersphere
* Copyright 2014-2015, Scattersphere Project.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.scattersphere.registry;
import net.scattersphere.job.Job;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.*;
/**
* This is a registry that is used to store known {@link Job}s that can be referenced by Scattersphere.
*
* Created by kenji on 11/26/14.
*/
public class JobRegistry {
private static final JobRegistry instance = new JobRegistry();
private final Map> registry = new HashMap<>();
private final Logger LOG = LoggerFactory.getLogger(JobRegistry.class);
/**
* Returns the default instance of this class.
*
* @return Default {@link JobRegistry} object.
*/
public static JobRegistry instance() {
return instance;
}
/**
* This function loads in the registry of registered jobs from a properties file. Each registered job is then stored
* in a cache, and instantiated as required.
*
* @param props The properties to load job definitions from.
*/
public void loadFromProperties(Properties props) {
if (props.getProperty("jobs.count") == null) {
LOG.error("Unable to initialize: job registry missing key \"jobs.count\"");
System.exit(-1);
}
int jobsCount = Integer.parseInt(props.getProperty("jobs.count"));
if (jobsCount <= 0) {
LOG.error("Unable to initialize: invalid number of jobs: {}", jobsCount);
System.exit(-1);
}
LOG.debug("Initializing job registry: jobsCount={}", jobsCount);
int jobsRegistered = 0;
int jobsWarned = 0;
for(int i = 1; i < (jobsCount + 1); i++) {
String jobClassname = props.getProperty("jobs." + i + ".classname");
String jobName = props.getProperty("jobs." + i + ".name");
if (jobClassname == null) {
LOG.warn("Unable to start job at position {}: class name missing.", i);
jobsWarned++;
continue;
}
if (jobName == null) {
LOG.warn("Unable to start job at position {}: job name missing.", i);
jobsWarned++;
continue;
}
Class jobClass = null;
try {
jobClass = Class.forName(jobClassname);
} catch(Exception ex) {
LOG.error("Unable to start job at position {}: unable to find job in classpath: {}", i, jobClassname, ex);
continue;
}
registry.put(jobName, jobClass);
jobsRegistered++;
}
LOG.info("*** Job Registry: registered={} skipped={}", jobsRegistered, jobsWarned);
}
/**
* Retrieves a {@link Job} by the name specified.
*
* @param job The name o f the job to retrieve.
* @return {@link Job} object if found, {@code null} if not found.
*/
public Job get(String job) {
if (registry.get(job) == null) {
LOG.debug("Unable to find job by name: {}", job);
return null;
}
Class jobClass = registry.get(job);
Job jobObject = null;
try {
jobObject = (Job) jobClass.newInstance();
} catch(Exception ex) {
LOG.warn("Unable to create new instance of job: {}", job, ex);
ex.printStackTrace();
}
return jobObject;
}
/**
* Returns a list of the names of jobs available. Any jobs that could not be registered or instantiated are omitted
* from this list.
*
* @return {@code Map} of {@link String}, {@link Job}s containing the names of the registered jobs.
*/
public Map> getJobs() {
return registry;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy