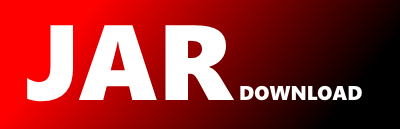
net.scattersphere.server.ClientConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scattersphere-core Show documentation
Show all versions of scattersphere-core Show documentation
Scattersphere Core library, used to run the main server.
The newest version!
/*
* Scattersphere
* Copyright 2014-2015, Scattersphere Project.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.scattersphere.server;
import net.scattersphere.util.PropertyUtil;
import org.vertx.java.core.buffer.Buffer;
import org.vertx.java.core.net.NetSocket;
/**
* This is a storage class that is used to store a read buffer for a client, and the client's {@link NetSocket} object.
*
* Created by kenji on 11/20/14.
*/
public class ClientConnection {
private final NetSocket endpoint;
private Buffer readBuffer;
private boolean authenticated;
private long expectedPayloadSize;
/** This is the maximum size of a read payload. */
public static final long MAX_PAYLOAD = Long.parseLong(PropertyUtil.get("server.payload.max", Main.serverProperties, "16384"));
/**
* Constructor, takes the {@link NetSocket} object.
*
* @param endpoint The {@link NetSocket} connected endpoint.
*/
public ClientConnection(NetSocket endpoint) {
if (endpoint == null) {
throw new NullPointerException("No NetSocket object provided.");
}
this.endpoint = endpoint;
this.readBuffer = new Buffer();
this.expectedPayloadSize = 0;
this.authenticated = false;
}
/**
* Retrieves the connected endpoint.
*
* @return {@link NetSocket} object.
*/
public NetSocket getEndpoint() {
return endpoint;
}
/**
* Retrieves the current read buffer.
*
* @return {@link Buffer} object.
*/
public Buffer getReadBuffer() {
return readBuffer;
}
/**
* Clears out the current read buffer, allowing for new data to be appended. Also clears out the expected payload size,
* as that information will be cleared out.
*/
public void clearReadBuffer() {
readBuffer = new Buffer();
setExpectedPayloadSize(0);
}
/**
* Sets the expected payload size.
*
* @param expectedPayloadSize The number of bytes expected for the next packet.
*/
public void setExpectedPayloadSize(long expectedPayloadSize) {
this.expectedPayloadSize = expectedPayloadSize;
}
/**
* Returns the current number of expected bytes from the read packet.
*
* @return {@code long} containing the number of bytes expected.
*/
public long getExpectedPayloadSize() {
return expectedPayloadSize;
}
/**
* Indicates whether or not the client connection is authenticated.
*
* @return {@code true} if authenticated, {@code false} otherwise.
*/
public boolean isAuthenticated() {
return authenticated;
}
/**
* Sets the authentication flag, indicating that the user is authenticated.
*
* @param authenticated {@code boolean} flag indicating whether or not authentication was successful.
*/
public void setAuthenticated(boolean authenticated) {
this.authenticated = authenticated;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy