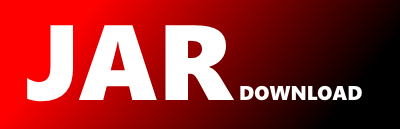
net.scattersphere.server.Server Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scattersphere-core Show documentation
Show all versions of scattersphere-core Show documentation
Scattersphere Core library, used to run the main server.
The newest version!
/*
* Scattersphere
* Copyright 2014-2015, Scattersphere Project.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.scattersphere.server;
import net.scattersphere.registry.AuthRegistry;
import net.scattersphere.registry.JobRegistry;
import net.scattersphere.server.handler.core.ConnectionCloseHandler;
import net.scattersphere.server.handler.core.ConnectionReadHandler;
import net.scattersphere.util.AuthenticationHelper;
import net.scattersphere.util.PropertyUtil;
import net.scattersphere.util.thread.JobManager;
import org.slf4j.LoggerFactory;
import org.vertx.java.core.Handler;
import org.vertx.java.core.VertxFactory;
import org.vertx.java.core.net.NetServer;
import org.vertx.java.core.net.NetSocket;
import java.io.File;
import java.io.FileInputStream;
import java.util.Properties;
/**
* Main server code.
*
* Created by kenji on 11/16/14.
*/
public class Server {
private final NetServer controllerServer;
private final Properties properties = new Properties();
private final org.slf4j.Logger LOG = LoggerFactory.getLogger(Server.class);
/**
* As a new connection is received by the server, read and close handlers are assigned to each connection. This way,
* new messages can be handled through their respective handlers by the server.
*/
public class ServerConnectionHandler implements Handler {
@Override
public void handle(NetSocket netSocket) {
ClientConnection client = new ClientConnection(netSocket);
ConnectionReadHandler readHandler = new ConnectionReadHandler(client);
ConnectionCloseHandler closeHandler = new ConnectionCloseHandler(client);
netSocket.dataHandler(readHandler);
netSocket.closeHandler(closeHandler);
// Check registry to see if authentication is required. If not, set the user as authenticated by default.
if (!AuthenticationHelper.requiresAuthentication()) {
AuthenticationHelper.sendAuthSuccessful(client);
} else {
AuthenticationHelper.sendAuthRequired(client);
}
LOG.info("New connection: {}", netSocket);
}
}
/**
* Instantiates a new server.
*
* @param args An array of {@code String} objects provided at command line.
*/
public Server(String args[]) {
if (args.length > 0) {
parseArguments(args);
}
// Initializes the job registry.
JobRegistry.instance();
// Initializes the job manager thread pool.
JobManager.instance();
controllerServer = VertxFactory.newVertx().createNetServer();
String serverBindAddress = PropertyUtil.get("server.bind.main.address", Main.serverProperties, "localhost");
int serverBindPort = Integer.parseInt(PropertyUtil.get("server.bind.main.port", Main.serverProperties, "10001"));
controllerServer.connectHandler(new ServerConnectionHandler())
.setTCPKeepAlive(true)
.listen(serverBindPort, serverBindAddress);
System.err.println("--- Main server listening for connections on " + serverBindAddress + ":" + serverBindPort);
}
private void showHelp() {
System.err.println("Usage: server [arguments]");
System.err.println(" -p [file] Loads the specified properties file.");
System.err.println(" -u [file] Loads the specified user auth properties file.");
System.err.println(" -h/--help Shows help.");
System.err.println(" -v/--version Shows version information.");
}
private void showVersion() {
System.err.println("Version: " + Main.getRelease());
}
private void loadProperties(String file) throws Exception {
properties.load(new FileInputStream(new File(file)));
JobRegistry.instance().loadFromProperties(properties);
}
private void loadUsers(String file) throws Exception {
Properties props = new Properties();
props.load(new FileInputStream(new File(file)));
AuthRegistry.instance().loadFromProperties(props);
}
private void parseArguments(String args[]) {
for(int i = 0; i < args.length; i++) {
String arg = args[i].toLowerCase();
switch(arg) {
case "-h":
case "--help":
showHelp();
System.exit(0);
case "-v":
case "--version":
showVersion();
System.exit(0);
case "-p":
if (++i >= args.length) {
System.err.println("-p requires a filename.");
System.exit(-1);
}
try {
loadProperties(args[i]);
} catch(Exception ex) {
System.err.println("*** Properties file could not be loaded: " + ex.getMessage());
ex.printStackTrace();
System.exit(-1);
}
break;
case "-u":
if (++i >= args.length) {
System.err.println("-u requires a filename.");
System.exit(-1);
}
try {
loadUsers(args[i]);
} catch(Exception ex) {
System.err.println("*** Properties file could not be loaded: " + ex.getMessage());
ex.printStackTrace();
System.exit(-1);
}
break;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy