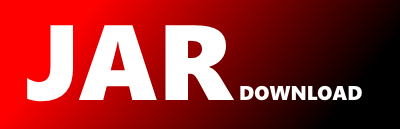
net.scattersphere.server.handler.stream.SubscribedStreamWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scattersphere-core Show documentation
Show all versions of scattersphere-core Show documentation
Scattersphere Core library, used to run the main server.
The newest version!
package net.scattersphere.server.handler.stream;
import net.scattersphere.data.DataSerializer;
import net.scattersphere.registry.StreamRegistry;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.vertx.java.core.buffer.Buffer;
import org.vertx.java.core.net.NetSocket;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.atomic.AtomicBoolean;
/**
* This is a thread that runs to send data to a connection. Once the data pump has been fully sent, the
* connection is closed. This current implementation is uninterruptible.
*
* Created by kenji on 2/13/15.
*/
public class SubscribedStreamWriter implements Runnable {
private final NetSocket endpoint;
private final String streamId;
private final BlockingQueue stream;
private final AtomicBoolean isRunning;
private final Logger LOG = LoggerFactory.getLogger(SubscribedStreamWriter.class);
public SubscribedStreamWriter(NetSocket endpoint, String streamId) {
this.endpoint = endpoint;
this.streamId = streamId;
this.isRunning = new AtomicBoolean(false);
this.stream = StreamRegistry.instance().getStream(streamId);
}
public void run() {
isRunning.set(true);
while(isRunning.get()) {
byte[] buffer = null;
if (endpoint == null) {
LOG.debug("Stream {} endpoint is null", streamId);
break;
}
try {
buffer = stream.take();
StreamRegistry.instance().decrementStreamSize(streamId);
} catch(InterruptedException ex) {
LOG.debug("Stream {} interrupted, closing connection to {}", streamId, endpoint.toString());
} catch(NullPointerException ex) {
LOG.debug("Stream {} empty, closing connection to {}", streamId, endpoint.toString());
endpoint.close();
break;
}
Buffer outBuffer = new Buffer(DataSerializer.packetize(buffer));
LOG.debug("Writing packet: destination={} length={} status={} size={}", endpoint.toString(), buffer.length,
StreamRegistry.instance().isClosed(streamId), StreamRegistry.instance().getSize(streamId));
endpoint.write(outBuffer);
if (StreamRegistry.instance().isClosed(streamId) && StreamRegistry.instance().isEmpty(streamId)) {
endpoint.close();
LOG.debug("Stream closed to {}", endpoint.toString());
break;
}
}
if (isRunning.get()) {
LOG.info("Thread shutdown normally.");
} else {
LOG.info("Thread shutdown due to disconnect or error.");
}
}
public void stop() {
this.isRunning.set(false);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy