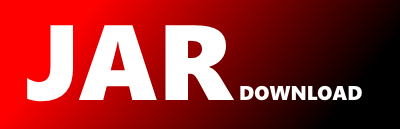
net.scattersphere.util.thread.StrictBlockingQueue Maven / Gradle / Ivy
Show all versions of scattersphere-core Show documentation
/*
* Scattersphere
* Copyright 2014-2015, Scattersphere Project.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.scattersphere.util.thread;
import java.util.LinkedList;
import java.util.List;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.TimeUnit;
/**
* This is an extension of the {@link java.util.concurrent.LinkedBlockingQueue} class. It behaves exactly like a standard blocking
* queue, except that it will restrict the type of objects that are added to the queue. The classes that are added
* must be of type {@code T} as the base class, however, any objects that are to be added to the queue must extend
* or implement type {@code T}.
*
* The entry point {@link net.scattersphere.util.thread.StrictBlockingQueue#addAllowedClass(Class)} is used to identify which classes can be injected
* into the queue. Any classes that do not extend or implement type {@code E} will throw an {@link IllegalArgumentException}.
*
* @author [email protected]
* @param Any typed class, typically a class that is extended as a base class, interface, or a raw {@code Object}.
*/
public class StrictBlockingQueue extends LinkedBlockingQueue implements BlockingQueue {
private static final long serialVersionUID = -8767657443214194248L;
private List> _allowedClasses;
public StrictBlockingQueue(int capacity) {
super(capacity);
_allowedClasses = new LinkedList<>();
}
/**
* Identifies a {@link Class} object that can be injected into this queue.
*
* @param c Any {@link Class} object.
*/
public void addAllowedClass(Class extends T> c) {
_allowedClasses.add(c);
}
// TODO
// Convert this into a Lambda.
private boolean allowed(T e) {
for(Class> c : _allowedClasses) {
if (c.isInstance(e)) {
return true;
}
}
return false;
}
/**
* Adds an object to the queue.
*
* @param e Object of type {@code T}.
* @throws IllegalArgumentException if the class type is not allowed.
*/
public boolean add(T e) {
if (allowed(e)) {
return super.add(e);
}
throw new IllegalArgumentException("Class type not allowed: " + e.getClass().toString());
}
/**
* Offers an object for the queue.
*
* @param e Object of type {@code T}.
* @throws IllegalArgumentException if the class type is not allowed.
*/
public boolean offer(T e) {
if (allowed(e)) {
return super.offer(e);
}
throw new IllegalArgumentException("Class type not allowed: " + e.getClass().toString());
}
/**
* Offers an object for the queue.
*
* @param e Object of type {@code T}.
* @param timeout The time period to wait.
* @param unit The {@link java.util.concurrent.TimeUnit} of time to wait.
* @throws IllegalArgumentException if the class type is not allowed.
*/
public boolean offer(T e, long timeout, TimeUnit unit) throws InterruptedException {
if (allowed(e)) {
return super.offer(e, timeout, unit);
}
throw new IllegalArgumentException("Class type not allowed: " + e.getClass().toString());
}
/**
* Puts an object into the queue.
*
* @param e Object of type {@code T}.
* @throws IllegalArgumentException if the class type is not allowed.
*/
public void put(T e) throws InterruptedException {
if (allowed(e)) {
super.put(e);
}
throw new IllegalArgumentException("Class type not allowed: " + e.getClass().toString());
}
}