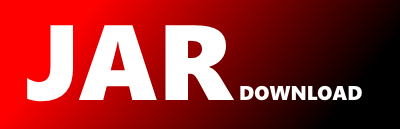
net.segoia.util.data.ListMap Maven / Gradle / Ivy
/**
* commons - Various Java Utils
* Copyright (C) 2009 Adrian Cristian Ionescu - https://github.com/acionescu
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.segoia.util.data;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
public class ListMap implements Serializable, Cloneable{
/**
*
*/
private static final long serialVersionUID = -1703006626599612175L;
private Map> backedMap = new LinkedHashMap>();
public ListMap() {
}
public ListMap(ListMapFactory factory) {
backedMap = factory.createMap();
}
public void add(K key, T value){
List defList = backedMap.get(key);
if(defList == null){
defList = new ArrayList();
backedMap.put(key, defList);
}
if(!defList.contains(value)){
defList.add(value);
}
}
public void add(K key, List values){
for(T value : values){
add(key,value);
}
}
public void add(Map> map){
if(map == null || map.size() <= 0) {
return;
}
for(Map.Entry> e : map.entrySet()){
add(e.getKey(),e.getValue());
}
}
public List remove(K key){
return backedMap.remove(key);
}
public boolean remove(K key, T value) {
List values = get(key);
if(values != null) {
return values.remove(value);
}
return false;
}
public List get(K key){
return backedMap.get(key);
}
public Set keySet(){
return backedMap.keySet();
}
public Map> getAll(){
return backedMap;
}
public Collection> values(){
return backedMap.values();
}
public void clear(){
backedMap.clear();
}
public int getTotalSize() {
int sum = 0;
for(List list : backedMap.values()) {
sum += list.size();
}
return sum;
}
public int size() {
return backedMap.size();
}
public Set>> entrySet(){
return backedMap.entrySet();
}
public boolean containsValueForKey(K key, T value) {
List values = get(key);
if(values == null) {
return false;
}
return values.contains(value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy