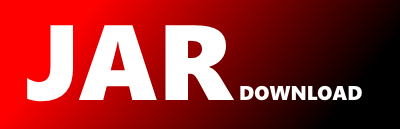
net.segoia.util.data.SetMap Maven / Gradle / Ivy
The newest version!
/**
* commons - Various Java Utils
* Copyright (C) 2009 Adrian Cristian Ionescu - https://github.com/acionescu
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.segoia.util.data;
import java.io.Serializable;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
import java.util.TreeSet;
public class SetMap implements Map>, Serializable {
private Map> nestedMap;
private SetFactory nestedSetFactory;
/**
*
*/
private static final long serialVersionUID = -7712790543550126181L;
public SetMap() {
nestedMap = new HashMap>();
nestedSetFactory = new HashSetFactory();
}
private SetMap(Map> nestedMap, SetFactory setFactory) {
this.nestedMap = nestedMap;
this.nestedSetFactory = setFactory;
}
public static SetMap createHashMapWithHashSet() {
return new SetMap(new HashMap>(),
new SetMap.HashSetFactory());
}
public static SetMap createTreeMapWithTreeSet() {
return new SetMap(new TreeMap>(),
new SetMap.TreeSetFactory());
}
public void add(K key, V value) {
Set nestedSet = getNestedSet(key);
nestedSet.add(value);
}
public Set getNestedSet(K key) {
Set nestedSet = get(key);
if (nestedSet == null) {
nestedSet = nestedSetFactory.createSet();
put(key, nestedSet);
}
return nestedSet;
}
public void putAllNestedValues(K key, Collection values) {
Set nestedSet = getNestedSet(key);
nestedSet.addAll(values);
}
public boolean containsNestedValue(K key, V value) {
Set nested = get(key);
if (nested == null) {
return false;
}
return nested.contains(value);
}
public Set getIntersection() {
Set intersection = new HashSet();
boolean first = true;
for (Set s : values()) {
if (first) {
intersection = s;
first = false;
} else {
intersection.retainAll(s);
}
}
return intersection;
}
public Set getUnion() {
Set union = new HashSet();
for (Set s : values()) {
union.addAll(s);
}
return union;
}
public Map> getAll(){
return nestedMap;
}
@Override
public int size() {
return nestedMap.size();
}
@Override
public boolean isEmpty() {
return nestedMap.isEmpty();
}
@Override
public boolean containsKey(Object key) {
return nestedMap.containsKey(key);
}
@Override
public boolean containsValue(Object value) {
return nestedMap.containsValue(value);
}
@Override
public Set get(Object key) {
return nestedMap.get(key);
}
@Override
public Set put(K key, Set value) {
return nestedMap.put(key, value);
}
@Override
public Set remove(Object key) {
return nestedMap.remove(key);
}
@Override
public void putAll(Map extends K, ? extends Set> m) {
nestedMap.putAll(m);
}
@Override
public void clear() {
nestedMap.clear();
}
@Override
public Set keySet() {
return nestedMap.keySet();
}
@Override
public Collection> values() {
return nestedMap.values();
}
@Override
public Set>> entrySet() {
return nestedMap.entrySet();
}
private static interface SetFactory extends Serializable{
Set createSet();
}
private static class HashSetFactory implements SetFactory {
/**
*
*/
private static final long serialVersionUID = 5493174035691826492L;
@Override
public Set createSet() {
return new HashSet();
}
}
private static class TreeSetFactory implements SetFactory {
/**
*
*/
private static final long serialVersionUID = 1403320248186453906L;
@Override
public Set createSet() {
return new TreeSet();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy