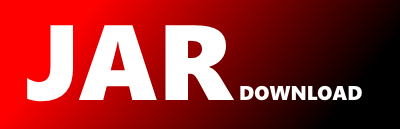
net.segoia.util.data.repository.ObjectsRepository Maven / Gradle / Ivy
The newest version!
/**
* commons - Various Java Utils
* Copyright (C) 2009 Adrian Cristian Ionescu - https://github.com/acionescu
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.segoia.util.data.repository;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
import net.segoia.util.data.ObjectsUtil;
import net.segoia.util.data.SetMap;
import net.segoia.util.data.reflection.ReflectionUtility;
/**
* Implements a repository for a certain type of object (all the objects should
* share a common interface) this can also be used as a repository of
* repositories
*
* @author adi
*
* @param
*/
public class ObjectsRepository implements Serializable {
/**
*
*/
private static final long serialVersionUID = -2852851812850022179L;
/**
* keeps a map with all the maps used to search objects by certain
* properties key - the name of the property used to search
* value - the map with all the mapped values for the property
*/
private Map> indexMap = new TreeMap>();
/**
* the list of searchable properties
*/
private List indexProperties = new ArrayList();
private Set allObjects = new HashSet();
public ObjectsRepository() {
}
/**
* @return the indexProperties
*/
public List getIndexProperties() {
return indexProperties;
}
/**
* @param indexProperties
* the indexProperties to set
*/
public void setIndexProperties(List indexProperties) {
this.indexProperties = indexProperties;
}
public void addIndexProperty(String property) {
if (!indexProperties.contains(property)) {
indexProperties.add(property);
}
}
public boolean addObject(O obj) {
boolean added = allObjects.add(obj);
if (added) {
buildIndexesForObject(obj);
}
return added;
}
public int addObjects(Collection c) {
int addedCount = 0;
for (O o : c) {
boolean added = addObject(o);
if (added) {
addedCount++;
}
}
return addedCount;
}
/**
* adds the current object to the indexes according to the specified index
* properties
*
* @param obj
*/
private void buildIndexesForObject(O obj) {
for (String prop : indexProperties) {
try {
Object value = ReflectionUtility.getValueForField(obj, prop);
/* get the map for the current property */
SetMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy