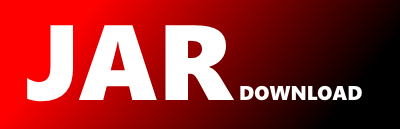
net.serenitybdd.assertions.CollectionMatchers Maven / Gradle / Ivy
package net.serenitybdd.assertions;
import org.hamcrest.Description;
import org.hamcrest.Matcher;
import org.hamcrest.TypeSafeMatcher;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Collection;
import java.util.function.Consumer;
import java.util.function.Predicate;
public class CollectionMatchers {
private final static Logger LOGGER = LoggerFactory.getLogger(CollectionMatchers.class);
public static Matcher> containsAll(Collection expectedListEntries) {
return new TypeSafeMatcher>() {
@Override
protected boolean matchesSafely(Collection actualValues) {
return actualValues.containsAll(expectedListEntries);
}
@Override
public void describeTo(Description description) {
description.appendText("contains ").appendValue(expectedListEntries);
}
};
}
public static Matcher> allMatch(Predicate predicate) {
return allMatch(predicate, "match predicate");
}
public static Matcher> allMatch(Predicate predicate, String predicateDescription) {
return new TypeSafeMatcher>() {
@Override
protected boolean matchesSafely(Collection actualValues) {
return actualValues.stream().allMatch(predicate);
}
@Override
public void describeTo(Description description) {
description.appendText("all elements ").appendText(predicateDescription);
}
};
}
public static Matcher> anyMatch(Predicate predicate) {
return anyMatch(predicate,"match predicate");
}
public static Matcher> anyMatch(Predicate predicate, String predicateDescription) {
return new TypeSafeMatcher>() {
@Override
protected boolean matchesSafely(Collection actualValues) {
return actualValues.stream().anyMatch(predicate);
}
@Override
public void describeTo(Description description) {
description.appendText("at least one element ").appendText(predicateDescription);
}
};
}
public static Matcher> allSatisfy(Consumer consumer) {
return allSatisfy(consumer, "all satisfy the expected condition");
}
public static Matcher> allSatisfy(Consumer consumer, String consumerDescription) {
return new TypeSafeMatcher>() {
@Override
protected boolean matchesSafely(Collection actualValues) {
try {
actualValues.forEach(consumer);
} catch (AssertionError assertionError) {
LOGGER.error("Assertion error", assertionError);
return false;
}
return true;
}
@Override
public void describeTo(Description description) {
description.appendText("for all elements ").appendText(consumerDescription);
}
};
}
public static Matcher> satisfies(Consumer> consumer) {
return satisfies(consumer, "satisfies the expected condition");
}
public static Matcher> satisfies(Consumer> consumer, String consumerDescription) {
return new TypeSafeMatcher>() {
@Override
protected boolean matchesSafely(Collection actualValues) {
try {
consumer.accept(actualValues);
} catch (AssertionError assertionError) {
LOGGER.error("Assertion error", assertionError);
return false;
}
return true;
}
@Override
public void describeTo(Description description) {
description.appendText("the collection ").appendText(consumerDescription);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy