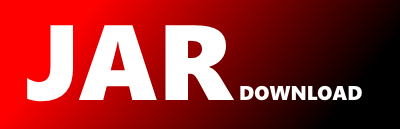
net.serenitybdd.core.pages.WebElementFacade Maven / Gradle / Ivy
package net.serenitybdd.core.pages;
import net.serenitybdd.core.Serenity;
import net.serenitybdd.core.annotations.ImplementedBy;
import net.serenitybdd.core.selectors.Selectors;
import net.thucydides.core.webdriver.ConfigurableTimeouts;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.WrapsElement;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.interactions.Locatable;
import org.openqa.selenium.support.ui.Wait;
import java.time.Duration;
import java.time.temporal.TemporalUnit;
import java.util.List;
import java.util.concurrent.TimeUnit;
import java.util.stream.Collectors;
@ImplementedBy(WebElementFacadeImpl.class)
public interface WebElementFacade extends WebElement, WrapsElement, WebElementState, Locatable, ConfigurableTimeouts {
T then(String xpathOrCssSelector);
T thenFind(String xpathOrCssSelector);
T then(String xpathOrCssSelector, Object... arguments);
T thenFind(String xpathOrCssSelector, Object... arguments);
T findBy(String xpathOrCssSelector);
T findBy(String xpathOrCssSelector, Object... arguments);
ListOfWebElementFacades thenFindAll(String xpathOrCssSelector);
ListOfWebElementFacades thenFindAll(String xpathOrCssSelector, Object... arguments);
T findBy(By selector);
T find(By bySelector);
T then(By bySelector);
String getAttribute(String name);
ListOfWebElementFacades thenFindAll(By... selector);
long getImplicitTimeoutInMilliseconds();
@Deprecated
T withTimeoutOf(int timeout, TimeUnit unit);
T withTimeoutOf(int timeout, TemporalUnit unit);
T withTimeoutOf(Duration duration);
/**
* Convenience method to chain method calls more fluently.
*/
T and();
/**
* Convenience method to chain method calls more fluently.
*/
T then();
List getSelectOptions();
List getSelectOptionValues();
String getFirstSelectedOptionVisibleText();
List getSelectedVisibleTexts();
String getFirstSelectedOptionValue();
List getSelectedValues();
/**
* Type a value into a field, making sure that the field is empty first.
*/
T type(CharSequence... keysToSend);
/**
* Type a value into a field and then press Enter, making sure that the field is empty first.
*/
T typeAndEnter(String value);
/**
* Type a value into a field and then press TAB, making sure that the field is empty first.
* This currently is not supported by all browsers, notably Firefox.
*/
T typeAndTab(String value);
void setWindowFocus();
FluentDropdownSelect select();
FluentDropdownDeselect deselect();
T deselectAll();
T deselectByVisibleText(String label);
T deselectByValue(String value);
T deselectByIndex(int indexValue);
T selectByVisibleText(String label);
T selectByValue(String value);
T selectByIndex(int indexValue);
T waitUntilVisible();
T waitUntilPresent();
Wait waitForCondition();
T waitUntilNotVisible();
String getValue();
String getText();
String getTextContent();
String getAriaLabel();
String getRole();
boolean isDisabled();
T waitUntilEnabled();
T waitUntilClickable();
T waitUntilDisabled();
/**
* Wait for an element to be visible and enabled, and then click on it.
*/
void click();
void click(ClickStrategy clickStrategy);
void doubleClick();
void contextClick();
void clear();
String toString();
boolean containsElements(By selector);
boolean containsElements(String xpathOrCssSelector);
WebElementState shouldContainElements(By selector);
WebElementState shouldContainElements(String xpathOrCssSelector);
boolean hasClass(String cssClassName);
WebElement getElement();
ListOfWebElementFacades findNestedElementsMatching(ResolvableElement nestedElement);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy