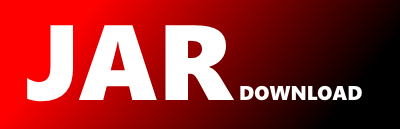
net.serenitybdd.screenplay.ensure.ComparableEnsure.kt Maven / Gradle / Ivy
@file:Suppress("UNCHECKED_CAST")
package net.serenitybdd.screenplay.ensure
import net.serenitybdd.screenplay.Actor
import net.serenitybdd.screenplay.ensure.CommonPreconditions.ensureActualAndExpectedNotNull
import net.serenitybdd.screenplay.ensure.CommonPreconditions.ensureActualAndRangeValues
import net.serenitybdd.screenplay.ensure.CommonPreconditions.ensureActualNotNull
/**
* Predicates about comparable types
*/
open class ComparableEnsure(override val value: KnowableValue>,
val comparator: Comparator? = null,
expectedDescription: String = descriptionOf { value }
) : CommonEnsure, A>(value, expectedDescription) {
constructor(value: Comparable, comparator: Comparator? = null):this(KnownValue(value, value.toString()), comparator)
/**
* Verifies that the actual value is equal to the given one.
*
* Example:
*
* actor.attemptsTo(Ensure.that("abc").isEqualTo("abc"));
*
*/
override fun isEqualTo(expected: A) =
PerformableExpectation(value, IS_EQUAL_TO_USING_COMPARATORS, expected, isNegated(), expectedDescription)
override fun isNotEqualTo(expected: A) =
PerformableExpectation(value, IS_NOT_EQUAL_TO_USING_COMPARATORS, expected, isNegated(), expectedDescription)
/**
* Verifies that the actual value is greater than the given one.
*
* Example:
*
// assertions will pass
* actor.attemptsTo(Ensure.that(2).isGreaterThan(1));
*
*/
fun isGreaterThan(expected: Comparable) = PerformableExpectation(value, IS_GREATER_THAN, expected, isNegated(), expectedDescription)
/**
* Verifies that the actual value is greater than or equal to the given one.
*
* Example:
*
// assertions will pass
* actor.attemptsTo(Ensure.that(2).isGreaterThanOrEqualTo(1));
* actor.attemptsTo(Ensure.that(1).isGreaterThanOrEqualTo(1));
*
*/
fun isGreaterThanOrEqualTo(expected: Comparable) = PerformableExpectation(value, IS_GREATER_THAN_OR_EQUAL_TO, expected, isNegated(), expectedDescription)
/**
* Verifies that the actual value is less than the given one.
*
* Example:
*
// assertions will pass
* actor.attemptsTo(Ensure.that(2).isLessThan(3));
*
*/
fun isLessThan(expected: Comparable) = PerformableExpectation(value, IS_LESS_THAN, expected, isNegated(), expectedDescription)
/**
* Verifies that the actual value is less than or equal to the given one.
*
* Example:
*
// assertions will pass
* actor.attemptsTo(Ensure.that(2).isLessThanOrEqualTo(2));
* actor.attemptsTo(Ensure.that(1).isLessThanOrEqualTo(2));
*
*/
fun isLessThanOrEqualTo(expected: Comparable) = PerformableExpectation(value, IS_LESS_THAN_OR_EQUAL_TO, expected, isNegated(), expectedDescription)
/**
* Verifies that the actual value is between two values
*
* Example:
*
// assertions will pass
* actor.attemptsTo(Ensure.that(2).isBetween(1,3));
*
*/
fun isBetween(lowerBound: Comparable, upperBound: Comparable) = BiPerformableExpectation(value, IS_BETWEEN, lowerBound, upperBound, isNegated(), expectedDescription)
/**
* Verifies that the actual value is between two values, excluding the boundaries
*
* Example:
*
// assertions will pass
* actor.attemptsTo(Ensure.that(2).isStrictlyBetween(1,3));
* // assertions will fail
* actor.attemptsTo(Ensure.that(1).isStrictlyBetween(1,3));
*
*/
fun isStrictlyBetween(lowerBound: Comparable, upperBound: Comparable) = BiPerformableExpectation(value, IS_STRICTLY_BETWEEN, lowerBound, upperBound, isNegated(), expectedDescription)
open fun hasValue(): ComparableEnsure = this
override fun not(): ComparableEnsure = negate() as ComparableEnsure
override fun silently(): ComparableEnsure = super.silently() as ComparableEnsure
open fun usingComparator(comparator: Comparator): ComparableEnsure {
return ComparableEnsure(value, comparator)
}
private val IS_EQUAL_TO_USING_COMPARATORS =
expectThatActualIs("equal to",
fun(actor: Actor?, actual: KnowableValue>?, expected: A): Boolean {
ensureActualAndExpectedNotNull(actual, expected)
val resolvedValue = actual!!(actor!!)
BlackBox.logAssertion(resolvedValue, expected)
return if (comparator != null) comparator.compare(resolvedValue as A, expected) == 0 else resolvedValue == expected
})
private val IS_NOT_EQUAL_TO_USING_COMPARATORS =
expectThatActualIs("not equal to",
fun(actor: Actor?, actual: KnowableValue>?, expected: A): Boolean {
ensureActualAndExpectedNotNull(actual, expected)
val resolvedValue = actual!!(actor!!)
BlackBox.logAssertion(resolvedValue, expected)
ensureActualNotNull(resolvedValue)
return if (comparator != null) comparator.compare(resolvedValue as A, expected) != 0 else resolvedValue != expected
})
private val IS_GREATER_THAN =
expectThatActualIs("greater than",
fun(actor: Actor?, actual: KnowableValue>?, expected: Comparable): Boolean {
ensureActualAndExpectedNotNull(actual, expected)
val resolvedValue = actual!!(actor!!)
BlackBox.logAssertion(resolvedValue, expected)
ensureActualNotNull(resolvedValue)
return if (comparator != null) comparator.compare(resolvedValue as A, expected as A) > 0 else resolvedValue!! > expected as A
})
private val IS_GREATER_THAN_OR_EQUAL_TO = expectThatActualIs("greater than or equal to",
fun(actor: Actor?, actual: KnowableValue>?, expected: Comparable): Boolean {
ensureActualAndExpectedNotNull(actual, expected)
val resolvedValue = actual!!(actor!!)
BlackBox.logAssertion(resolvedValue, expected)
ensureActualNotNull(resolvedValue)
return if (comparator != null) comparator.compare(resolvedValue as A, expected as A) >= 0 else resolvedValue!! >= expected as A
})
private val IS_LESS_THAN = expectThatActualIs("less than",
fun(actor: Actor?, actual: KnowableValue>?, expected: Comparable): Boolean {
ensureActualAndExpectedNotNull(actual, expected)
val resolvedValue = actual!!(actor!!)
BlackBox.logAssertion(resolvedValue, expected)
ensureActualNotNull(resolvedValue)
return if (comparator != null) comparator.compare(resolvedValue as A, expected as A) < 0 else resolvedValue!! < expected as A
})
private val IS_LESS_THAN_OR_EQUAL_TO =
expectThatActualIs("less than or equal to",
fun(actor: Actor?, actual: KnowableValue>?, expected: Comparable): Boolean {
ensureActualAndExpectedNotNull(actual, expected)
val resolvedValue = actual!!(actor!!)
BlackBox.logAssertion(resolvedValue, expected)
ensureActualNotNull(resolvedValue)
return if (comparator != null) comparator.compare(resolvedValue as A, expected as A) <= 0 else resolvedValue!! <= expected as A
})
private val IS_BETWEEN =
expectThatActualIs("between",
fun(actor: Actor?, actual: KnowableValue>?, startRange: Comparable, endRange: Comparable): Boolean {
ensureActualAndRangeValues(actual, startRange, endRange)
val resolvedValue = actual!!(actor!!)
BlackBox.logAssertion(resolvedValue, "between $startRange and $endRange")
ensureActualNotNull(resolvedValue)
return if (comparator != null)
comparator.compare(resolvedValue as A, startRange as A) >= 0 && comparator.compare(resolvedValue as A, endRange as A) <= 0
else
(resolvedValue!! >= startRange as A && resolvedValue <= endRange as A)
})
private val IS_STRICTLY_BETWEEN =
expectThatActualIs("strictly between",
fun(actor: Actor?, actual: KnowableValue>?, startRange: Comparable, endRange: Comparable): Boolean {
ensureActualAndRangeValues(actual, startRange, endRange)
val resolvedValue = actual!!(actor!!)
BlackBox.logAssertion(resolvedValue, "strictly between $startRange and $endRange")
ensureActualNotNull(resolvedValue)
return if (comparator != null)
comparator.compare(resolvedValue as A, startRange as A) > 0 && comparator.compare(resolvedValue as A, endRange as A) < 0
else
(resolvedValue!! > startRange as A && resolvedValue < endRange as A)
})
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy