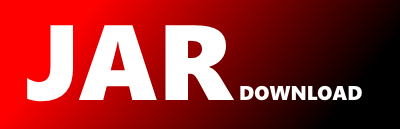
net.serenitybdd.screenplay.ensure.PerformableExpectations.kt Maven / Gradle / Ivy
package net.serenitybdd.screenplay.ensure
import net.serenitybdd.screenplay.Actor
import net.serenitybdd.screenplay.Performable
import net.thucydides.core.annotations.Step
import net.thucydides.core.model.TestResult
import net.thucydides.core.steps.StepEventBus
open class PerformableExpectation(private val actual: A?,
private val expectation: Expectation,
private val expected: E?,
private val isNegated: Boolean = false,
private val expectedDescription: String = "a value") : Performable {
private val description = expectation.describe(expected, isNegated, expectedDescription);
@Step("{0} should see #description")
override fun performAs(actor: T) {
BlackBox.reset()
val result = expectation.apply(actual, expected!!, actor)
if (isAFailure(result, isNegated)) {
val exceptionMessage = expectation.compareActualWithExpected(
actual,
expected,
isNegated,
expectedDescription
);
if (BlackBox.isUsingSoftAssertions()) {
BlackBox.softlyAssert(exceptionMessage)
StepEventBus.getEventBus().baseStepListener.updateCurrentStepFailureCause(AssertionError(exceptionMessage))
} else {
takeScreenshot()
throw AssertionError(exceptionMessage)
}
}
}
/**
* Internal use only - DO NOT USE
*/
protected constructor() : this(null,
Expectation(
"placeholder",
"placeholder",
fun(_: Actor?, _: A?, _: E): Boolean = true
),
null) {
}
}
open class BiPerformableExpectation(private val actual: A?,
private val expectation: DoubleValueExpectation,
private val startRange: E?,
private val endRange: E?,
private val isNegated: Boolean = false,
private val expectedDescription: String) : Performable {
private val description = expectation.describeRange(startRange, endRange, isNegated, expectedDescription);
@Step("{0} should see #description")
override fun performAs(actor: T) {
BlackBox.reset()
val result = expectation.apply(actual, startRange, endRange, actor)
if (isAFailure(result, isNegated)) {
takeScreenshot()
throw AssertionError(expectation.compareActualWithExpected(actual, startRange, endRange, isNegated, expectedDescription))
}
}
/**
* Internal use only - DO NOT USE
*/
protected constructor() : this(null,
DoubleValueExpectation(
"placeholder",
fun(_: Actor?, _: A?, _: E, _: E): Boolean = true
),
null,
null,
false,
"") {
}
}
fun takeScreenshot() {
if (StepEventBus.getEventBus().isBaseStepListenerRegistered) {
StepEventBus.getEventBus().takeScreenshot()
}
}
open class PerformablePredicate(private val actual: A?,
private val expectation: PredicateExpectation,
private val isNegated: Boolean = false,
private val expectedDescription: String,
private val exception: Throwable? = null) : Performable {
private val description = expectation.describe(isNegated, expectedDescription);
@Step("{0} should see #description")
override fun performAs(actor: T) {
BlackBox.reset()
val result = expectation.apply(actual, actor)
if (isAFailure(result, isNegated)) {
if (exception != null) {
throw exception
} else {
takeScreenshot()
throw AssertionError(expectation.compareActualWithExpected(actual, isNegated, expectedDescription))
}
}
}
fun orElseThrow(exception: Throwable): PerformablePredicate {
return PerformablePredicate(actual, expectation, isNegated, expectedDescription, exception)
}
/**
* Internal use only - DO NOT USE
*/
protected constructor() : this(null,
PredicateExpectation(
"placeholder", "placeholder",
fun(_: Actor?, _: A?): Boolean = true
),
false,
"placeholder") {
}
}
private fun isAFailure(result: Boolean, isNegated: Boolean) = (!isNegated && !result || isNegated && result)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy