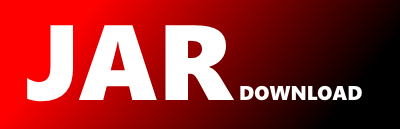
net.serenitybdd.jbehave.SerenityStepContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serenity-jbehave Show documentation
Show all versions of serenity-jbehave Show documentation
Serenity JBehave integration
package net.serenitybdd.jbehave;
import com.google.common.collect.Maps;
import net.thucydides.core.pages.Pages;
import net.thucydides.core.webdriver.ThucydidesWebDriverSupport;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.lang.reflect.Constructor;
import java.util.Map;
/**
* Keeps track of instantiated JBehave step libraries used in ThucydidesWebdriverIntegration tests.
*/
public class SerenityStepContext {
private static final Logger LOGGER = LoggerFactory.getLogger(SerenityStepContext.class);
private Map, Object> stepInstances = Maps.newHashMap();
public SerenityStepContext() {
}
public Object newInstanceOf(final Class> type) {
if (stepInstances.containsKey(type)) {
return stepInstances.get(type);
} else {
Object newInstance = null;
try {
ThucydidesWebDriverSupport.getPages();
if (hasConstructorWithPagesParameter(type)) {
newInstance = createNewPageEnabledStepCandidate(type);
} else {
newInstance = type.newInstance();
}
} catch (Exception e) {
throw new SerenityStepInitializationError(e);
}
stepInstances.put(type, newInstance);
return newInstance;
}
}
private boolean hasConstructorWithPagesParameter(Class> type) {
Class[] constructorArgs = new Class[1];
constructorArgs[0] = Pages.class;
try {
type.getConstructor(constructorArgs);
} catch (NoSuchMethodException e) {
return false;
}
return true;
}
private T createNewPageEnabledStepCandidate(final Class type) {
T newInstance = null;
try {
Pages pageFactory = ThucydidesWebDriverSupport.getPages();
Class[] constructorArgs = new Class[1];
constructorArgs[0] = Pages.class;
Constructor constructor = type.getConstructor(constructorArgs);
newInstance = constructor.newInstance(pageFactory);
} catch (Exception e) {
LOGGER.info("Failed to instantiate page of type {} ({})", type, e.getMessage());
}
return newInstance;
}
public void reset() {
stepInstances.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy