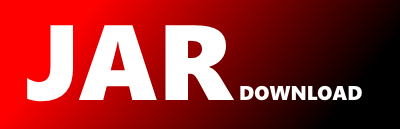
net.serenitybdd.screenplay.rest.questions.RestQuestionBuilder Maven / Gradle / Ivy
package net.serenitybdd.screenplay.rest.questions;
import net.serenitybdd.screenplay.Question;
import java.util.ArrayList;
import java.util.List;
public class RestQuestionBuilder implements To, Returning {
private String name;
private String endpoint;
private List queries = new ArrayList<>();
public To about(String name) {
this.name = name;
return this;
}
public Returning to(String endpoint) {
this.endpoint = endpoint;
return this;
}
public Returning with(RestQueryFunction query) {
this.queries.add(query);
return this;
}
public Returning withPathParameters(String param1, Object value1) {
queries.add(request -> request.pathParam(param1, value1));
return this;
}
public Returning withPathParameters(String param1, Object value1,
Object... otherParams) {
this.queries.add(request -> request.pathParam(param1, value1));
if (otherParams.length % 2 == 1) {
throw new IllegalArgumentException("Invalid number of path parameters");
}
int paramIndex = 0;
while (paramIndex < otherParams.length) {
String param = otherParams[paramIndex].toString();
Object value = otherParams[paramIndex + 1];
paramIndex = paramIndex + 2;
RestQueryFunction restQueryFunction = request -> request.pathParam(param, value);
this.queries.add(restQueryFunction);
}
return this;
}
public Returning withQueryParameters(String param1, Object value1) {
queries.add(request -> request.queryParam(param1, value1));
return this;
}
public Returning withQueryParameters(String param1, Object value1,
Object... otherParams) {
this.queries.add(request -> request.queryParam(param1, value1));
if (otherParams.length % 2 == 1) {
throw new IllegalArgumentException("Invalid number of query parameters");
}
int paramIndex = 0;
while (paramIndex < otherParams.length) {
String param = otherParams[paramIndex].toString();
Object value = otherParams[paramIndex + 1];
paramIndex = paramIndex + 2;
this.queries.add(request -> request.queryParam(param, value));
}
return this;
}
public Question returning(RestResponseFunction response) {
return new RestQuestion(name, endpoint, queries, response);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy