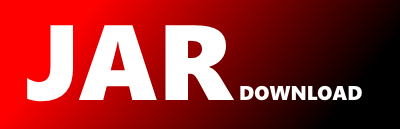
net.serenitybdd.screenplay.EventualConsequence Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serenity-screenplay Show documentation
Show all versions of serenity-screenplay Show documentation
Support for the User Journey pattern in Serenity
package net.serenitybdd.screenplay;
import net.serenitybdd.core.time.Stopwatch;
import net.thucydides.core.guice.Injectors;
import net.thucydides.core.webdriver.Configuration;
public class EventualConsequence implements Consequence {
public static final int A_SHORT_PERIOD_BETWEEN_TRIES = 100;
private final Consequence consequenceThatMightTakeSomeTime;
private final long timeout;
private AssertionError caughtAssertionError = null;
private RuntimeException caughtRuntimeException = null;
public EventualConsequence(Consequence consequenceThatMightTakeSomeTime, long timeout) {
this.consequenceThatMightTakeSomeTime = consequenceThatMightTakeSomeTime;
this.timeout = timeout;
}
public EventualConsequence(Consequence consequenceThatMightTakeSomeTime) {
this(consequenceThatMightTakeSomeTime,
Injectors.getInjector().getInstance(Configuration.class).getElementTimeout() * 1000);
}
public static EventualConsequence eventually(Consequence consequenceThatMightTakeSomeTime) {
return new EventualConsequence(consequenceThatMightTakeSomeTime);
}
public EventualConsequenceBuilder waitingForNoLongerThan(long amount) {
return new EventualConsequenceBuilder(consequenceThatMightTakeSomeTime, amount);
}
@Override
public void evaluateFor(Actor actor) {
Stopwatch stopwatch = new Stopwatch();
stopwatch.start();
do {
try {
consequenceThatMightTakeSomeTime.evaluateFor(actor);
return;
} catch (AssertionError assertionError) {
caughtAssertionError = assertionError;
} catch (RuntimeException runtimeException) {
caughtRuntimeException = runtimeException;
}
pauseBeforeNextAttempt();
} while (stopwatch.lapTime() < timeout);
throwAnyCaughtErrors();
}
private void pauseBeforeNextAttempt() {
try {
Thread.sleep(A_SHORT_PERIOD_BETWEEN_TRIES);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
private void throwAnyCaughtErrors() {
if (caughtAssertionError != null) {
throw caughtAssertionError;
}
if (caughtRuntimeException != null) {
throw caughtRuntimeException;
}
}
@Override
public String toString() {
return consequenceThatMightTakeSomeTime.toString();
}
@Override
public Consequence orComplainWith(Class extends Error> complaintType) {
return new EventualConsequence(consequenceThatMightTakeSomeTime.orComplainWith(complaintType));
}
@Override
public Consequence orComplainWith(Class extends Error> complaintType, String complaintDetails) {
return new EventualConsequence(consequenceThatMightTakeSomeTime.orComplainWith(complaintType, complaintDetails));
}
@Override
public Consequence whenAttemptingTo(Performable performable) {
return new EventualConsequence(consequenceThatMightTakeSomeTime.whenAttemptingTo(performable));
}
@Override
public Consequence because(String explanation) {
return new EventualConsequence(consequenceThatMightTakeSomeTime.because(explanation));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy