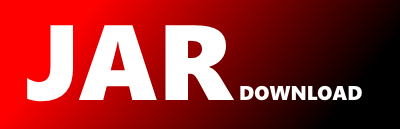
net.sf.aguacate.config.impl.ConfigurationImpl Maven / Gradle / Ivy
package net.sf.aguacate.config.impl;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.Map;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import net.sf.aguacate.config.Configuration;
import net.sf.aguacate.config.spi.PathInfoCompiler;
import net.sf.aguacate.context.ContextProcessor;
import net.sf.aguacate.context.ContextValidator;
import net.sf.aguacate.model.EntityInfo;
import net.sf.aguacate.model.FieldType;
import net.sf.aguacate.util.formatter.OutputFormater;
import net.sf.aguacate.util.json.JsonCodec;
import net.sf.aguacate.util.resource.ResourceLocator;
import net.sf.aguacate.validator.InputValidator;
import net.sf.aguacate.validator.ValidatorConverter;
public class ConfigurationImpl implements Configuration {
private static final Logger LOGGER = LogManager.getLogger(ConfigurationImpl.class);
private final ResourceLocator locator;
private final JsonCodec codec;
private final PathInfoCompiler compiler;
private final ValidatorConverter validatorConverter;
private Map cache;
public ConfigurationImpl(ResourceLocator locator, JsonCodec codec, PathInfoCompiler compiler,
ValidatorConverter validatorConverter) {
this.locator = locator;
this.codec = codec;
this.compiler = compiler;
this.validatorConverter = validatorConverter;
this.cache = new HashMap<>();
}
@Override
public boolean accepts(String entity, String method) {
return get(entity).accepts(method);
}
@Override
public InputValidator inputValidator(String entity, String method) {
return get(entity).getValidatorConverter(method);
}
@Override
public ContextValidator contextValidator(String entity, String method) {
return get(entity).getContextValidator();
}
@Override
public ContextProcessor contextProcessor(String entity, String method) {
return get(entity).getContextProcessor();
}
@Override
public OutputFormater outputFormater(String entity, String method) {
return get(entity).getOutputFormater();
}
@Override
public Map outputFields(String entity, String method) {
return get(entity).getOutputFields().get(method);
}
EntityInfo get(String entity) {
try {
EntityInfo info = cache.get(entity);
if (info == null) {
synchronized (this) {
info = cache.get(entity);
if (info == null) {
String resource = entity.concat(".json");
InputStream inputStream = locator.open(resource);
if (inputStream == null) {
LOGGER.warn("Not Found: {}", resource);
} else {
Map data;
try {
data = codec.decode(new InputStreamReader(inputStream));
} finally {
try {
inputStream.close();
} catch (IOException e) {
LOGGER.warn("when closing a resource", e);
}
}
LOGGER.trace("decoded: {}", data);
info = compiler.compile(validatorConverter, data);
LOGGER.trace("original: {}", cache);
Map temp = new HashMap<>(cache);
temp.put(entity, info);
LOGGER.trace("new: {}", temp);
cache = temp;
}
}
}
}
return info;
} catch (IOException e) {
throw new IllegalStateException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy