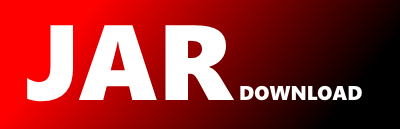
net.sf.aguacate.config.spi.PathInfoCompilerImpl Maven / Gradle / Ivy
package net.sf.aguacate.config.spi;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Pattern;
import javax.sql.DataSource;
import org.apache.commons.lang3.time.FastDateFormat;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import net.sf.aguacate.context.impl.ContextValidatorSql;
import net.sf.aguacate.context.spi.sql.ContextProcessorSql;
import net.sf.aguacate.context.spi.sql.SentenceSql;
import net.sf.aguacate.context.spi.sql.impl.SentenceSqlArrayIterator;
import net.sf.aguacate.context.spi.sql.impl.SentenceSqlConstant;
import net.sf.aguacate.context.spi.sql.impl.SentenceSqlInsert;
import net.sf.aguacate.context.spi.sql.impl.SentenceSqlSelectMultipleRow;
import net.sf.aguacate.context.spi.sql.impl.SentenceSqlSelectSingle;
import net.sf.aguacate.context.spi.sql.impl.SentenceSqlSelectSingleRow;
import net.sf.aguacate.context.spi.sql.impl.SentenceSqlupdate;
import net.sf.aguacate.function.Function;
import net.sf.aguacate.function.Parameter;
import net.sf.aguacate.function.spi.impl.FunctionArrayIterator;
import net.sf.aguacate.function.spi.impl.FunctionConstant;
import net.sf.aguacate.function.spi.impl.FunctionEquals;
import net.sf.aguacate.function.spi.impl.FunctionGreaterThan;
import net.sf.aguacate.function.spi.impl.FunctionGreaterThanToday;
import net.sf.aguacate.function.spi.impl.FunctionLessThan;
import net.sf.aguacate.function.spi.impl.FunctionNotEquals;
import net.sf.aguacate.function.spi.impl.FunctionRename;
import net.sf.aguacate.function.spi.impl.FunctionScript;
import net.sf.aguacate.function.spi.impl.FunctionSelectSingle;
import net.sf.aguacate.function.spi.impl.FunctionZero;
import net.sf.aguacate.model.EntityInfo;
import net.sf.aguacate.model.Field;
import net.sf.aguacate.model.FieldBoolean;
import net.sf.aguacate.model.FieldDynamicDate;
import net.sf.aguacate.model.FieldNumber;
import net.sf.aguacate.model.FieldStaticDateTime;
import net.sf.aguacate.model.FieldString;
import net.sf.aguacate.model.FieldStructureArray;
import net.sf.aguacate.model.FieldType;
import net.sf.aguacate.util.config.datasource.DataSourceCoupling;
import net.sf.aguacate.util.formatter.impl.OutputFormaterImpl;
import net.sf.aguacate.validator.ValidatorConverter;
public class PathInfoCompilerImpl implements PathInfoCompiler {
private static final Logger LOGGER = LogManager.getLogger(PathInfoCompilerImpl.class);
private static final String[] METHODS = new String[] { "GET", "POST", "PUT", "DELETE", "GET0", "PATCH" };
private static final FastDateFormat FMT_TIME;
private static final FastDateFormat FMT_DATE;
private static final FastDateFormat FMT_DATETIME;
static {
String time = "HH:mm:ss.SSS";
FMT_TIME = FastDateFormat.getInstance(time);
String date = "yyyy-MM-dd";
FMT_DATE = FastDateFormat.getInstance(date);
String datetime = date + "'T'" + time;
FMT_DATETIME = FastDateFormat.getInstance(datetime);
}
@Override
public EntityInfo compile(ValidatorConverter validatorConverter, Map data) {
return processFields(validatorConverter, data, processValidations(data), processSentences(data));
}
@SuppressWarnings("unchecked")
EntityInfo processFields(ValidatorConverter validatorConverter, Map data, List functions,
List sentences) {
Map fields = (Map) data.get("fields");
if (fields == null) {
return null;
} else {
Map> inputFields = new HashMap<>();
Map> outputFields = new HashMap<>();
for (Map.Entry entry : fields.entrySet()) {
String name = entry.getKey();
Map meta = (Map) entry.getValue();
parseInputField(name, meta, inputFields);
parseOutputField(name, meta, outputFields);
}
String primary = (String) data.get("primary");
DataSource dataSource = DataSourceCoupling.getDataSource((String) data.get("datasource"));
return new EntityInfo(validatorConverter, primary, inputFields, outputFields,
new ContextValidatorSql(dataSource, functions), new ContextProcessorSql(dataSource, sentences),
new OutputFormaterImpl());
}
}
@SuppressWarnings("unchecked")
void parseInputField(String name, Map meta, Map> methods) {
Map input = (Map) meta.get("input");
for (String method : METHODS) {
Map meta2 = (Map) input.get(method);
if (meta2 != null) {
Boolean mandatory = (Boolean) meta2.get("mandatory");
if (mandatory != null) {
Field field = toField(name, meta, !mandatory.booleanValue());
if (field != null) {
Map fields = methods.get(method);
if (fields == null) {
methods.put(method, fields = new HashMap<>());
}
fields.put(name, field);
}
}
}
}
}
Map toField(Map meta) {
Map fields = new HashMap<>();
for (Map.Entry entry : meta.entrySet()) {
String name = entry.getKey();
@SuppressWarnings("unchecked")
Map meta2 = (Map) entry.getValue();
// TODO: avoid optional with a hardcoded value (false)
fields.put(name, toField(name, meta2, false));
}
return fields;
}
Field toField(String name, Map meta, boolean optional) {
String string = (String) meta.get("type");
FieldType type = FieldType.valueOf(string);
switch (type) {
case TIME: {
Date minValue = parse(FMT_TIME, (String) meta.get("minval"));
Date maxValue = parse(FMT_TIME, (String) meta.get("maxval"));
return new FieldStaticDateTime(name, type, optional, minValue, maxValue);
}
case DYNAMIC_DATE:
return new FieldDynamicDate(name, type, optional, (String) meta.get("minval"), (String) meta.get("maxval"));
case DATE: {
Date minValue = parse(FMT_DATE, (String) meta.get("minval"));
Date maxValue = parse(FMT_DATE, (String) meta.get("maxval"));
return new FieldStaticDateTime(name, type, optional, minValue, maxValue);
}
case DATETIME: {
Date minValue = parse(FMT_DATETIME, (String) meta.get("minval"));
Date maxValue = parse(FMT_DATETIME, (String) meta.get("maxval"));
return new FieldStaticDateTime(name, type, optional, minValue, maxValue);
}
case FLOAT: {
BigDecimal minValue = new BigDecimal((String) meta.get("minval"));
BigDecimal maxValue = new BigDecimal((String) meta.get("maxval"));
return new FieldNumber(name, type, optional, minValue, maxValue);
}
case INTEGER: {
BigInteger minValue = new BigInteger((String) meta.get("minval"));
BigInteger maxValue = new BigInteger((String) meta.get("maxval"));
return new FieldNumber(name, type, optional, minValue, maxValue);
}
case STRING: {
int minLenth = Integer.parseInt((String) meta.get("minlen"));
int maxLength = Integer.parseInt((String) meta.get("maxlen"));
String regex = (String) meta.get("regex");
LOGGER.trace("{} regex to compile: {}", name, regex);
Pattern pattern = Pattern.compile(regex);
return new FieldString(name, type, optional, minLenth, maxLength, pattern);
}
case BOOLEAN: {
return new FieldBoolean(name, type, optional);
}
case STRUCTURE_ARRAY: {
@SuppressWarnings("unchecked")
Map sub = (Map) meta.get("data");
return new FieldStructureArray(name, type, optional, toField(sub));
}
default:
throw new IllegalArgumentException(string);
}
}
Date parse(FastDateFormat parser, String value) {
try {
return parser.parse(value);
} catch (ParseException e) {
throw new IllegalArgumentException(value);
}
}
@SuppressWarnings("unchecked")
List processValidations(Map data) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy