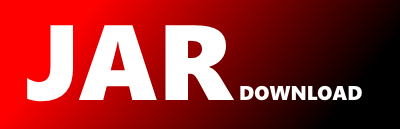
net.sf.aguacate.context.spi.sql.impl.AbstractSentenceSqlSelect Maven / Gradle / Ivy
package net.sf.aguacate.context.spi.sql.impl;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.List;
import java.util.Map;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import net.sf.aguacate.context.spi.sql.SentenceExecutionResult;
import net.sf.aguacate.context.spi.sql.SentenceSqlType;
public abstract class AbstractSentenceSqlSelect extends AbstractSentenceSql {
private static final Logger LOGGER = LogManager.getLogger(AbstractSentenceSqlSelect.class);
private static final String STR_AND = " AND ";
private final String[] required;
private final String[] optional;
private final String outputName;
public AbstractSentenceSqlSelect(String name, String table, List methods, List required,
List optional, String outputName) {
super(name, table, methods);
this.required = required.toArray(new String[required.size()]);
this.optional = optional.toArray(new String[optional.size()]);
this.outputName = outputName;
}
@Override
public SentenceSqlType getType() {
return SentenceSqlType.SELECT;
}
@Override
public String outputName() {
return outputName;
}
@Override
protected String buildSqlSentence(String table, Map context) {
StringBuilder builder = new StringBuilder("SELECT ");
for (String parameter : optional) {
builder.append(parameter).append(',');
}
int position = builder.length() - 1;
if (builder.charAt(position) == ',') {
builder.setLength(position);
} else {
LOGGER.warn("No parameters");
return null;
}
builder.append(" FROM ").append(table);
if (required.length == 0) {
return builder.toString();
} else {
builder.append(" WHERE ");
for (String parameter : required) {
if (context.containsKey(parameter)) {
builder.append(parameter).append("=?").append(STR_AND);
} else {
LOGGER.warn("Invalid parameter: {}", parameter);
return null;
}
}
int size = STR_AND.length();
position = builder.length() - size;
int count = 0;
for (count = 0; count < size; count++) {
if (STR_AND.charAt(count) != builder.charAt(position + count)) {
break;
}
}
if (count == size) {
return builder.substring(0, position);
} else {
LOGGER.warn("Size mismatch");
return null;
}
}
}
@Override
protected SentenceExecutionResult execute(PreparedStatement statement, Map context)
throws SQLException {
for (String parameter : required) {
statement.setObject(1, context.get(parameter));
}
ResultSet resultSet = statement.executeQuery();
try {
Object data = extract(optional, resultSet);
if (LOGGER.isTraceEnabled()) {
LOGGER.trace("execution {} result: {}", getName(), data);
}
return new SentenceExecutionResult(true, data);
} finally {
try {
resultSet.close();
} catch (SQLException e) {
LOGGER.warn("On ResultSet.close()", e);
}
}
}
abstract Object extract(String[] optional, ResultSet resultSet) throws SQLException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy