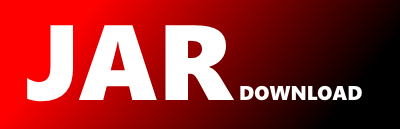
net.sf.aguacate.context.spi.sql.impl.SentenceSqlSelectMultipleRow Maven / Gradle / Ivy
package net.sf.aguacate.context.spi.sql.impl;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class SentenceSqlSelectMultipleRow extends AbstractSentenceSqlSelect {
private static final Logger LOGGER = LogManager.getLogger(SentenceSqlSelectMultipleRow.class);
public SentenceSqlSelectMultipleRow(String name, String table, List methods, List required,
List optional, String outputName) {
super(name, table, methods, required, optional, outputName);
}
@Override
Object extract(String[] optional, ResultSet resultSet) throws SQLException {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy