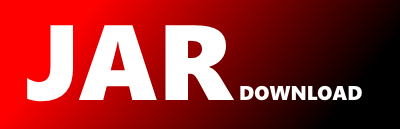
net.sf.aguacate.function.spi.impl.FunctionSelectSingle Maven / Gradle / Ivy
package net.sf.aguacate.function.spi.impl;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.text.MessageFormat;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import javax.sql.DataSource;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import net.sf.aguacate.function.FunctionEvalResult;
import net.sf.aguacate.function.Parameter;
import net.sf.aguacate.function.spi.AbstractFunction;
public class FunctionSelectSingle extends AbstractFunction {
private static final Logger LOGGER = LogManager.getLogger(FunctionSelectSingle.class);
private final String table;
public FunctionSelectSingle(Collection methods, String name, List parameters, String outputName,
String table) {
super(methods, name, parameters, outputName);
this.table = table;
}
@Override
public FunctionEvalResult evaluate(DataSource dataSource, Map context) {
String column = getParameters()[0].getName();
try {
Connection connection = dataSource.getConnection();
try {
String sql = MessageFormat.format("SELECT {0} FROM {1} WHERE {2} = ?", getOutputName(), table, column);
LOGGER.trace(sql);
PreparedStatement statement = connection.prepareStatement(sql);
try {
Object value = context.get(column);
statement.setObject(1, value);
ResultSet resultSet = statement.executeQuery();
try {
if (resultSet.next()) {
Object data = resultSet.getObject(1);
LOGGER.debug("found: {}", data);
if (resultSet.next()) {
LOGGER.warn("Multiple results for {} & {} = {}", table, column, value);
}
return new FunctionEvalResult(null, data);
} else {
LOGGER.debug("not found");
return new FunctionEvalResult(null, null);
}
} finally {
try {
resultSet.close();
} catch (SQLException e) {
LOGGER.error("On close resultSet", e);
}
}
} finally {
try {
statement.close();
} catch (SQLException e) {
LOGGER.error("", e);
}
}
} finally {
try {
connection.close();
} catch (SQLException e) {
LOGGER.error("", e);
}
}
} catch (SQLException e) {
throw new IllegalStateException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy